To add a directory to the system PATH environment variable in PowerShell, you can use the following command:
$env:Path += ";C:\Your\Directory\Path"
This command appends `C:\Your\Directory\Path` to the existing PATH variable, allowing you to execute scripts or programs located in that directory from any command prompt.
Understanding the PATH Variable
What is the PATH Variable?
The PATH variable is an essential component of the operating system that dictates where executable files are located. When you enter a command in PowerShell or any command-line interface, the operating system searches through the directories listed in the PATH variable to find the corresponding executable. If it isn't found, you'll receive an error indicating that the command is unrecognized.
How PowerShell Interacts with the PATH Variable
PowerShell serves as a powerful tool that allows users to interact with and manipulate system environment variables, including the PATH variable. PowerShell can work with environment variables on both a user level and a system level, enhancing flexibility in managing computing environments.
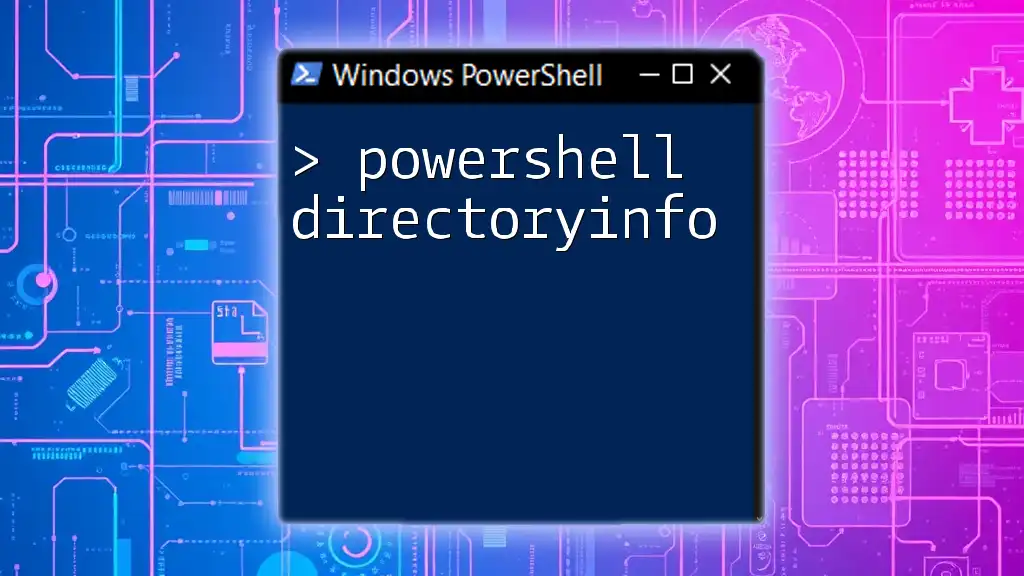
The Basics of Appending a Directory to the PATH
Reasons to Append Directories
Appending directories to the PATH variable can significantly enhance your workflow. For instance, if you regularly use scripts or executables from a specific folder, adding that directory to your PATH allows you to run them without needing to specify the full path every time. This can save time and reduce clutter in your command line.
Terminology: Appending vs. Overwriting
It's important to understand the difference between appending to the PATH and overwriting it. Appending adds a new directory to the existing list, while overwriting would replace the entire list with new values. Overwriting can lead to loss of access to commands if existing paths are removed, making appending the safer choice in most cases.
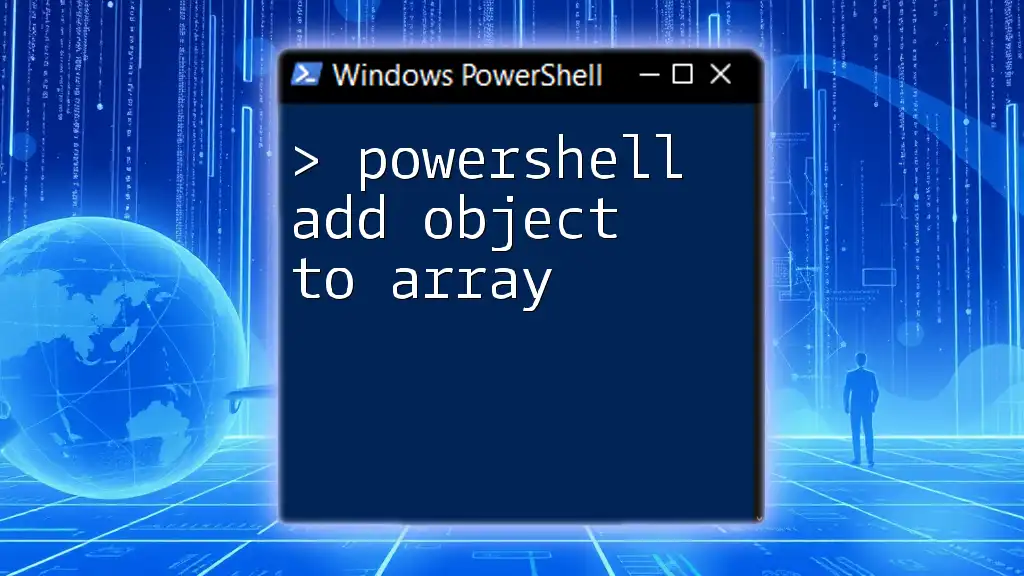
Steps to Add a Directory to PATH Using PowerShell
Accessing PowerShell
Before you begin modifying the PATH variable, open PowerShell with administrative privileges, as modifying system variables often requires elevated permissions. You can find PowerShell by searching in the Start Menu, right-clicking, and selecting "Run as administrator."
To ensure compatibility, check your PowerShell version with the following command:
$PSVersionTable.PSVersion
Viewing the Current PATH
To view the current directories included in your PATH variable, execute the following command:
$env:Path -split ";"
This command will split the PATH string into an array format, making it easy to read and understand which directories are currently included.
Appending a New Directory to PATH
Temporary Session Append
If you want to add a directory temporarily for the current PowerShell session, you can do so easily:
$env:Path += ";C:\NewDirectory"
This change will be effective only for the duration of the session. Once you close PowerShell, the addition will be lost.
Permanent PATH Modification
To permanently add a directory to your PATH, utilize the `System.Environment` class, allowing you to set the PATH variable more efficiently:
[System.Environment]::SetEnvironmentVariable("Path", $env:Path + ";C:\NewDirectory", [System.EnvironmentVariableTarget]::User)
This command appends `C:\NewDirectory` to the user-specific PATH variable. If you want to apply this change system-wide, replace `User` with `Machine` in the command. However, be cautious when modifying the Machine PATH as it affects all users on the system.
Verification of the Added Directory
Confirming the Change
To verify that the directory has been successfully added to your PATH, open a new PowerShell session and run:
$env:Path -split ";"
This will again show the current PATH variable. If the new directory is listed, you've successfully added it!
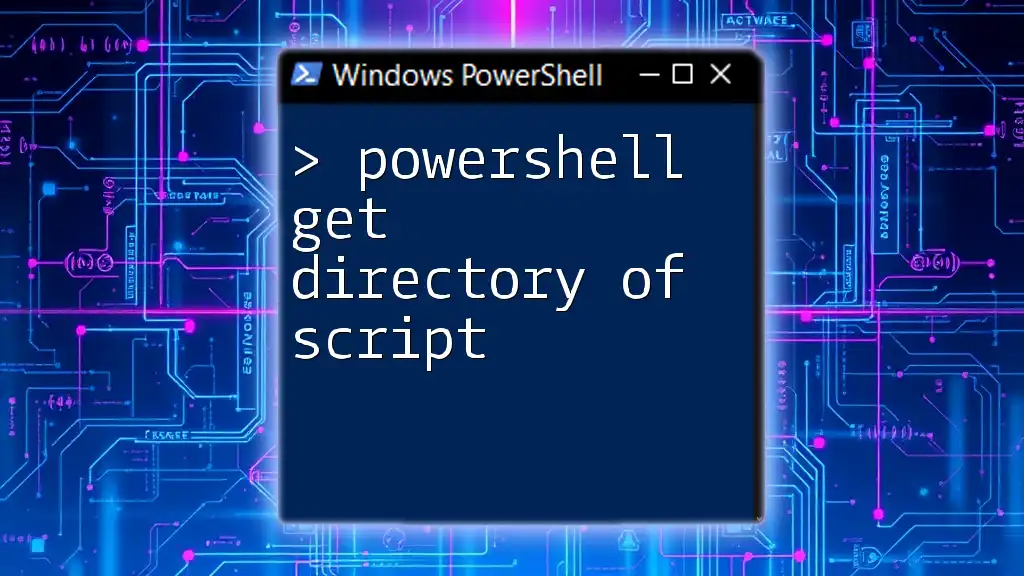
Troubleshooting Common Issues
Directory Not Found Errors
After adding a directory to your PATH, you may encounter issues where the command or script isn't recognized. This could be due to a typo in the directory path or the directory not existing at all. Double-check the path you added to ensure it is valid and properly formatted.
PATH Length Limitations
Keep in mind that the PATH variable has a maximum length, dependent on the operating system version. If you've added numerous directories and experience issues, consider cleaning up your PATH by removing any directories that are no longer needed. Doing so will help you avoid truncation and maintain efficient command access.
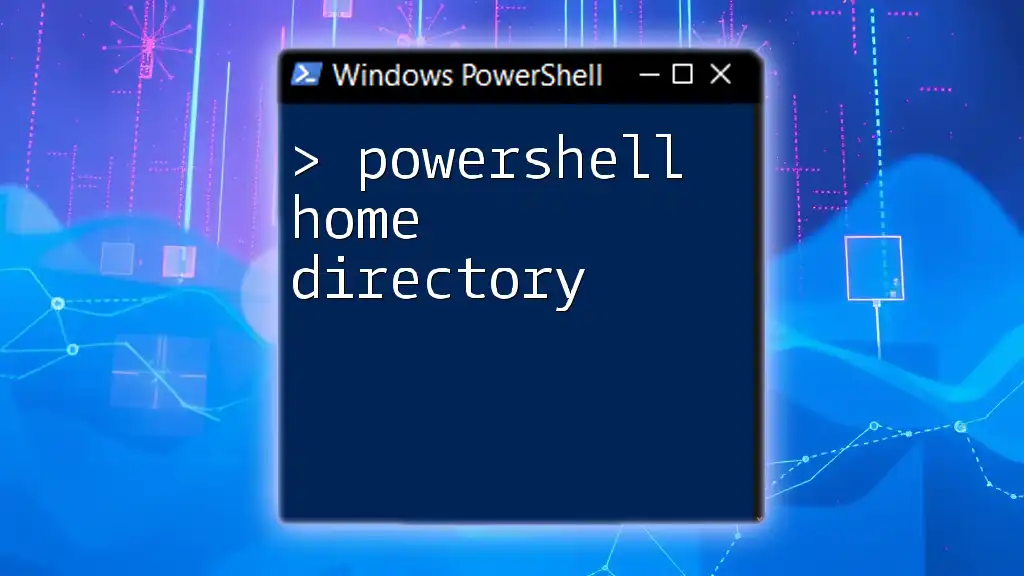
Advanced Tips and Best Practices
Keeping it Clean
To ensure that your PATH variable remains usable and efficient, regularly review and clean it. Delete any obsolete directories or paths that are no longer relevant. This helps maintain a tidy environment, minimizing confusion when executing commands.
Using PowerShell Scripts for PATH Management
For users frequently adding multiple directories, consider writing a PowerShell script to automate the process. Below is a sample script featuring a list of directories that can be added to your PATH:
$dirs = @("C:\NewDirectory1", "C:\NewDirectory2")
foreach ($dir in $dirs) {
[System.Environment]::SetEnvironmentVariable("Path", $env:Path + ";$dir", [System.EnvironmentVariableTarget]::User)
}
This script iterates through an array of directories and appends each one to the user's PATH permanently. It streamlines the addition process, especially useful for automation enthusiasts.
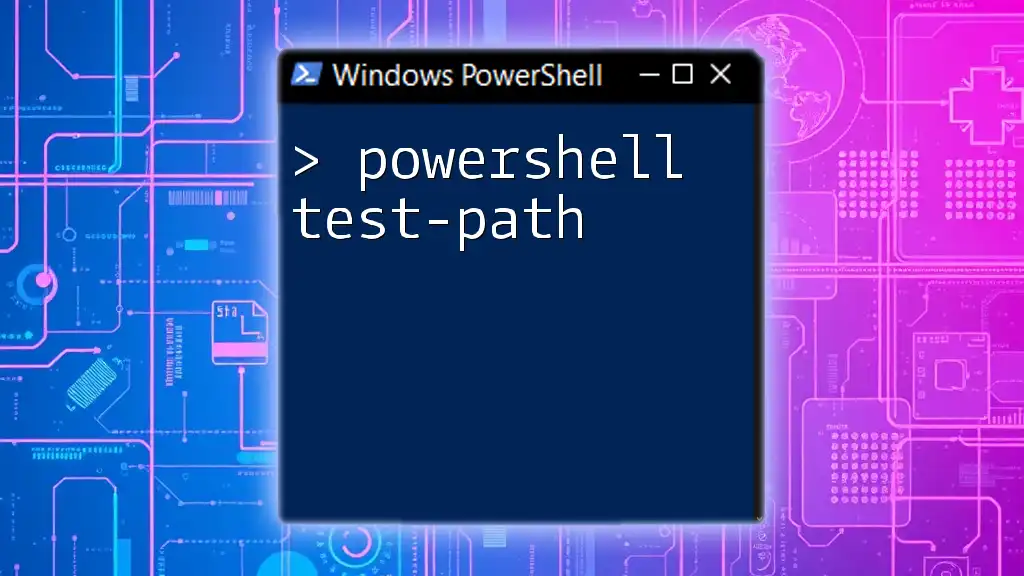
Conclusion
Understanding how to use PowerShell to add a directory to the PATH variable is an essential skill for enhancing your command-line efficiency. The ability to control which directories can be accessed directly via commands not only saves time but also simplifies your workflow dramatically. With the guidance provided in this article, you can confidently manage your PATH settings and optimize your PowerShell experience.
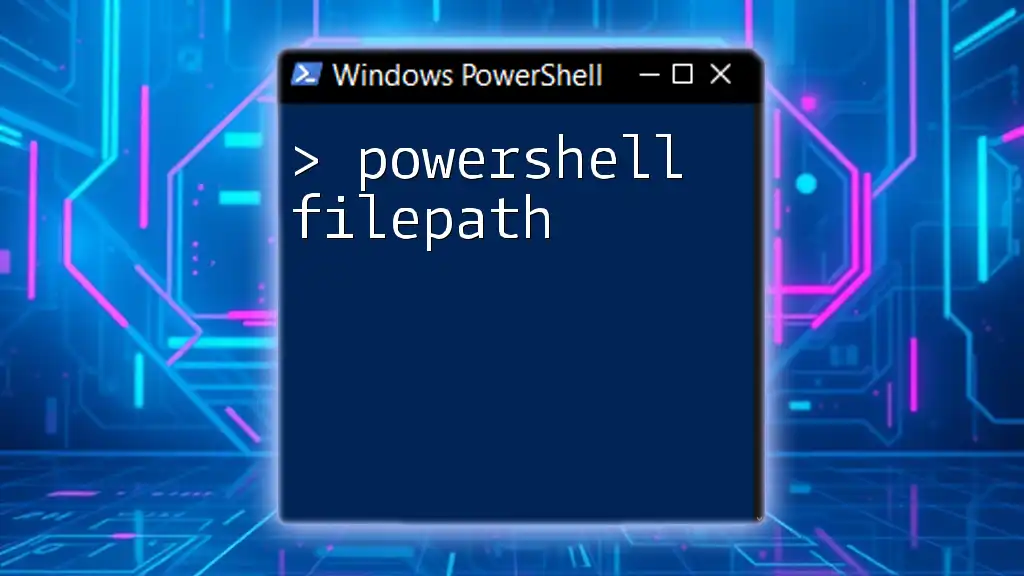
Additional Resources
For further reading, consider exploring the official PowerShell documentation or websites dedicated to scripting tutorials. This will broaden your knowledge and empower you to leverage PowerShell for robust system management.