In PowerShell, you can easily add days to a date using the `AddDays` method of the `DateTime` class, as shown in the following code snippet:
$originalDate = Get-Date
$newDate = $originalDate.AddDays(10)
Write-Host "The new date is: $newDate"
Understanding DateTime in PowerShell
What is DateTime?
In PowerShell, the DateTime object represents dates and times as a single point in time. Understanding this object is crucial for any tasks involving date manipulations as it allows for both straightforward and complex operations. The DateTime object encompasses various properties and methods, which enable you to work with dates effectively.
Common DateTime Properties
Some of the common properties of the DateTime object include:
- Year: Retrieves the year component of the date.
- Month: Retrieves the month component, with values from 1 (January) to 12 (December).
- Day: Retrieves the day of the date.
One particularly important method in PowerShell is AddDays(). This method allows you to add a specified number of days to a DateTime object, opening up a range of possibilities for date manipulation.
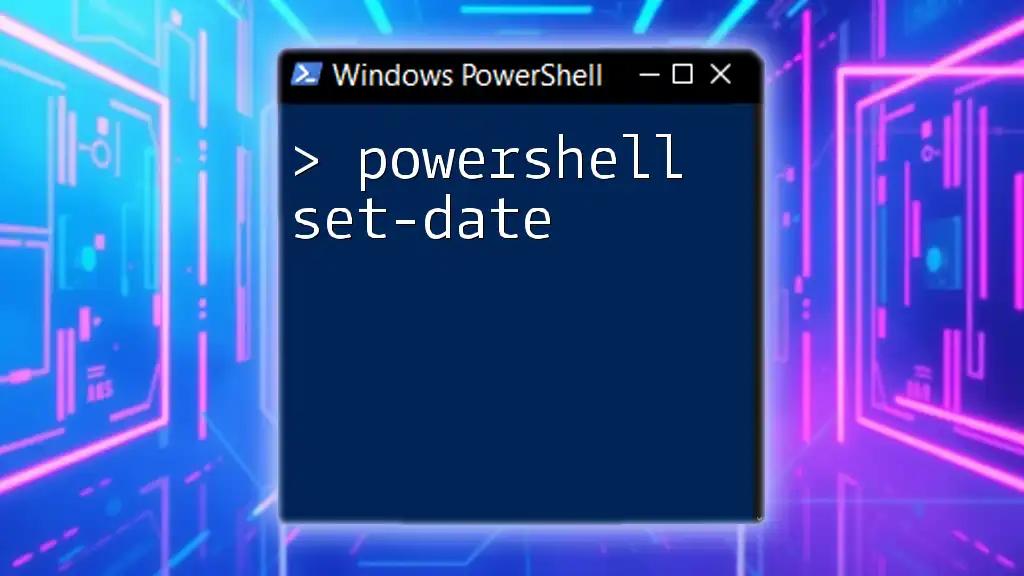
Getting Started with PowerShell Get-Date
Using Get-Date
The `Get-Date` cmdlet is your gateway to accessing and working with date and time in PowerShell. It returns a DateTime object for the current date and time.
To assign the current date to a variable, you would use:
$currentDate = Get-Date
You can also format the output easily. For example, if you want the date in the "yyyy-MM-dd" format, you can achieve it as follows:
$formattedDate = Get-Date -Format "yyyy-MM-dd"
Example: Fetching the Current Date
A basic but essential example of using `Get-Date` is fetching today's date:
$today = Get-Date
Write-Host "Today's date is: $today"
This simple code snippet outputs the current date and time, allowing you to see what you're working with.
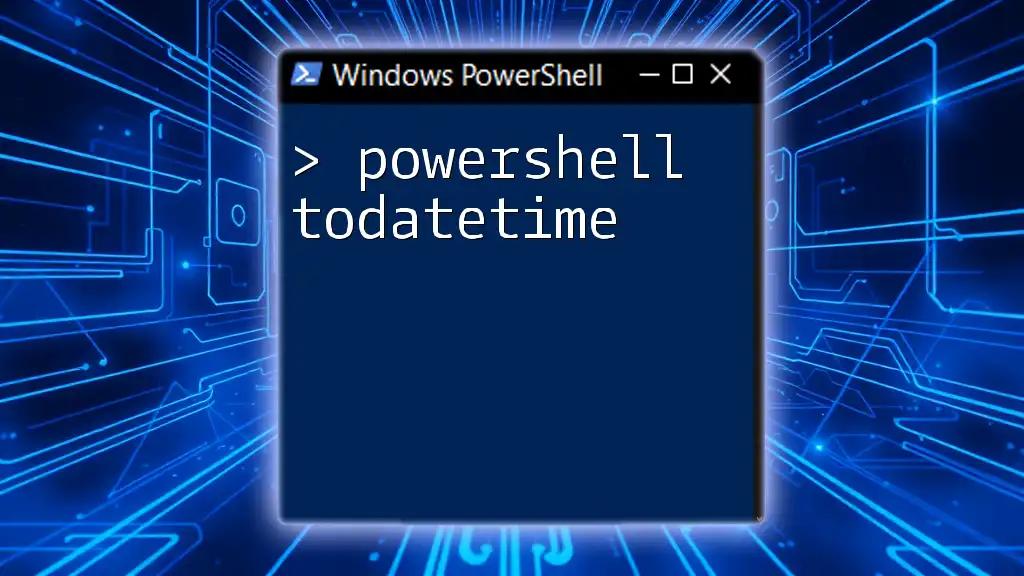
Adding Days to a Date
Using Get-Date with AddDays
You can add days to the current date using the `AddDays()` method with the DateTime object. This is where the magic of PowerShell’s date manipulation comes into play.
Here’s a straightforward example of how to add 10 days to the current date:
$futureDate = $today.AddDays(10)
Write-Host "The date 10 days from today will be: $futureDate"
Adding Days to a Specific Date
You can also use specific dates instead of relying solely on the current date. Suppose you want to add days to a predetermined date, such as January 1, 2023. You would do this:
$specificDate = Get-Date "2023-01-01"
$newDate = $specificDate.AddDays(15)
Write-Host "The date 15 days after January 1, 2023, is: $newDate"
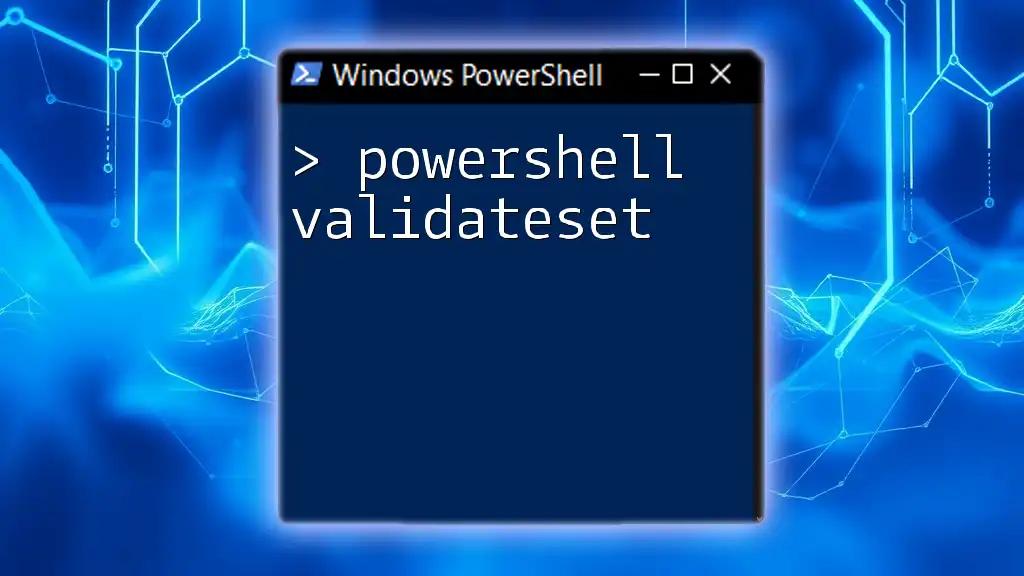
Leveraging PowerShell AddDays
Use Case: Scheduling Events or Reminders
One practical use case is scheduling events or reminders. By using date manipulation, you can easily calculate when you need to prepare for an event.
Here's an example:
$eventDate = Get-Date "2023-05-15"
$reminderDate = $eventDate.AddDays(-7)
Write-Host "Remember to prepare for your event by: $reminderDate"
This snippet helps in planning ahead by setting a reminder a week before a specified event.
Use Case: Creating Dynamic Reports
Another application of adding days is in creating dynamic reports. Often, reports need to cover specific periods, and understanding how days increment can create versatility in reporting.
For example:
$reportStartDate = Get-Date
$reportEndDate = $reportStartDate.AddDays(30)
Write-Host "Report period: From $reportStartDate to $reportEndDate"
This code calculates the reporting period starting from today for the next month, showcasing how effortlessly PowerShell can adapt to dynamic requirements.
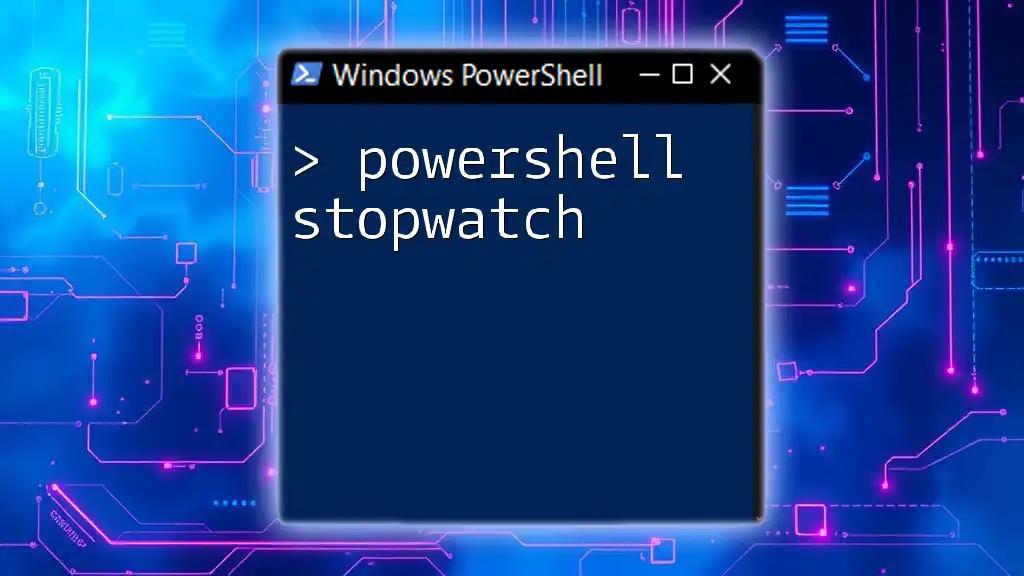
Error Handling and Best Practices
Common Errors with Date Manipulation
As you delve into date manipulations using PowerShell, be aware of common pitfalls. A prevalent mistake is passing non-integer values to the AddDays() method, which will generate an error. Always ensure that your inputs for date addition are integers.
Best Practices for Using AddDays
When working with date manipulations, clarity and readability in your code are paramount. Some best practices include:
- Commenting your code: This allows others (and yourself) to understand what your code does when they revisit it later.
- Naming conventions: Use meaningful variable names that reflect their content.
- Error checking: Integrate checks to handle unexpected input gracefully.
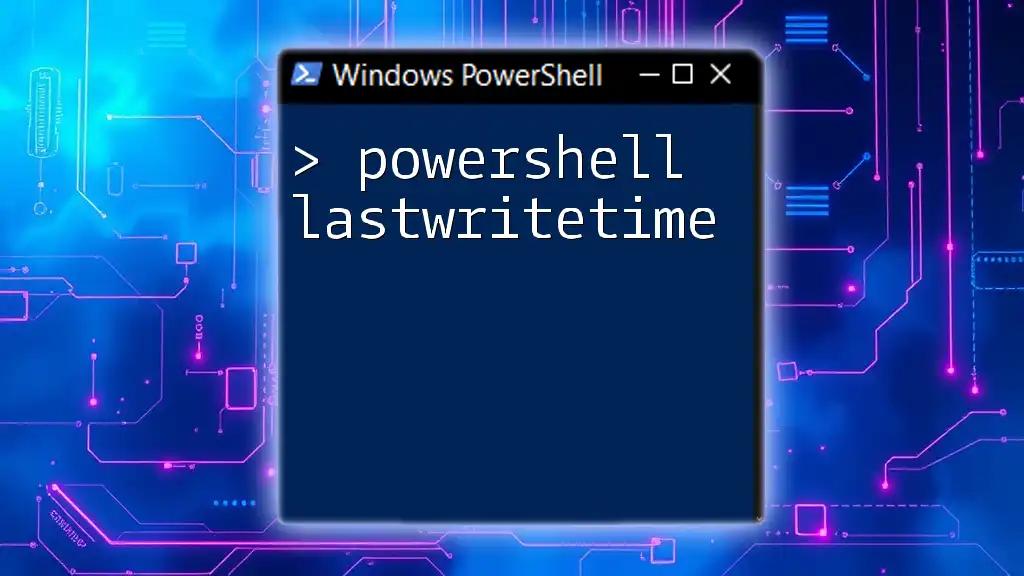
Conclusion
Mastering how to add days to a date in PowerShell enhances your scripting capabilities significantly. By embracing tools like `Get-Date` and the AddDays() method, you equip yourself for a myriad of tasks, from notifications to reports. Practice regularly with different scenarios to solidify your skills and navigate PowerShell's powerful date capabilities confidently.
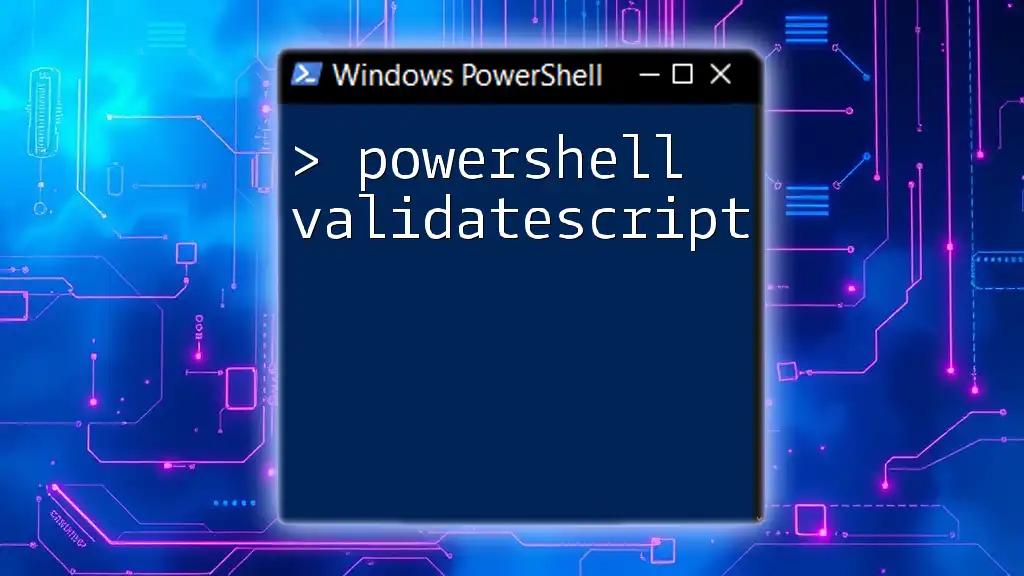
Further Learning Resources
PowerShell Documentation
For those looking to dive deeper into date manipulations and other functionalities within PowerShell, official documentation is a treasure trove of information.
Community Forums and Tutorials
Engaging with community forums and tutorials can provide diverse perspectives and solutions. Various platforms offer discussions and shared experiences that can enhance your learning journey.
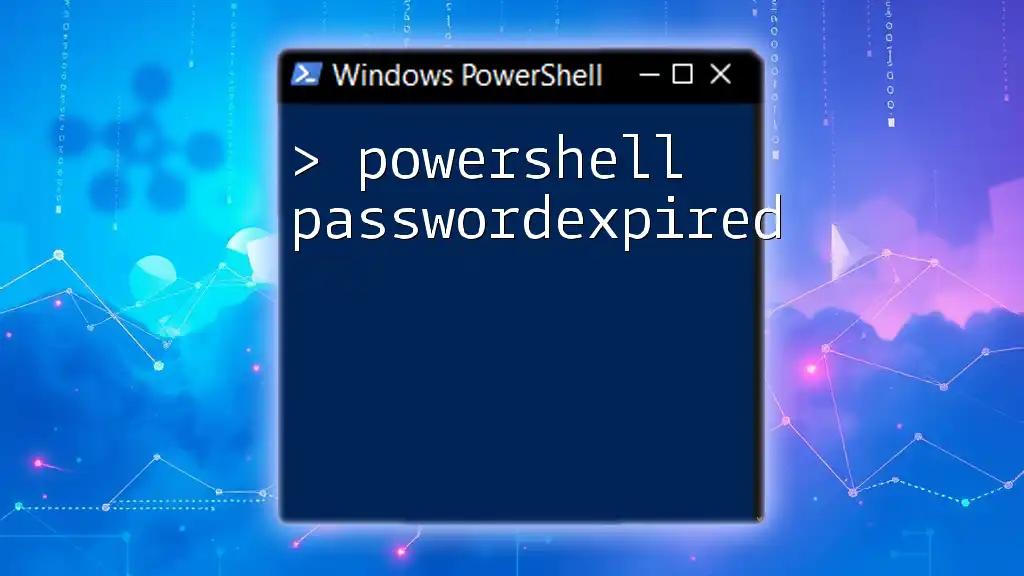
Call to Action
Share your own experiences with PowerShell date manipulations! If you have questions or want to explore more PowerShell topics, consider joining our upcoming courses or checking out our articles for further learning. Each new script enhances your skill set and opens up new possibilities!