To add a DNS record using PowerShell, you can utilize the `Add-DnsServerResourceRecordA` cmdlet to create an 'A' record that maps a hostname to an IP address.
Here's a code snippet demonstrating how to add a DNS record:
Add-DnsServerResourceRecordA -Name "example" -IPv4Address "192.168.1.1" -ZoneName "yourdomain.com"
Understanding DNS Records
What is a DNS Record?
DNS (Domain Name System) is like the phonebook of the internet, translating human-friendly domain names (like example.com) into machine-readable IP addresses (like 192.168.1.1). DNS records tell the internet how to route requests to the correct destination based on the queried domain name.
Common types of DNS records include:
- A Record: Maps a domain to an IPv4 address.
- AAAA Record: Maps a domain to an IPv6 address.
- CNAME Record: Alias for another domain.
- MX Record: Mail exchange for email routing.
- TXT Record: Used for various text information, often for verification purposes.
Why Manage DNS Records through PowerShell?
PowerShell offers a powerful way to automate DNS management tasks. Some advantages include:
- Efficiency: Use commands to add, edit, or delete records quickly.
- Automation: Create scripts to handle repetitive tasks or bulk changes.
- Control: Execute commands remotely and manage DNS in a structured way.
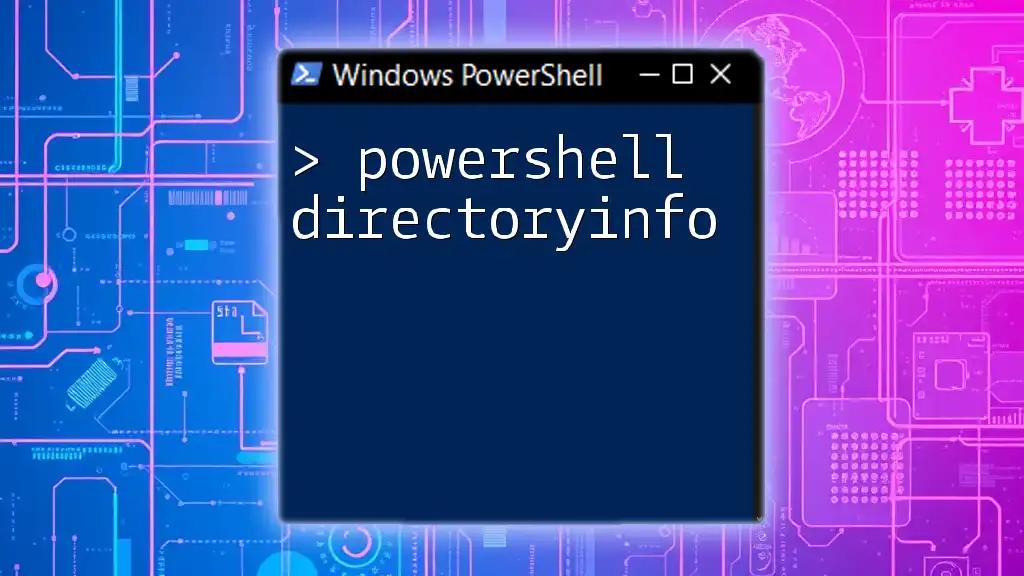
Prerequisites
System Requirements
Before diving into DNS record management with PowerShell, ensure your system meets the following requirements:
- A Windows version that supports PowerShell (Windows 10/Server 2016 and later).
- A compatible version of PowerShell (5.1 or higher recommended).
Necessary Permissions
To manage DNS records, administrative privileges are essential. Ensure your user account has the required permissions; otherwise, you'll encounter access denied errors. Always refer to your organization's network policies for any additional restrictions.
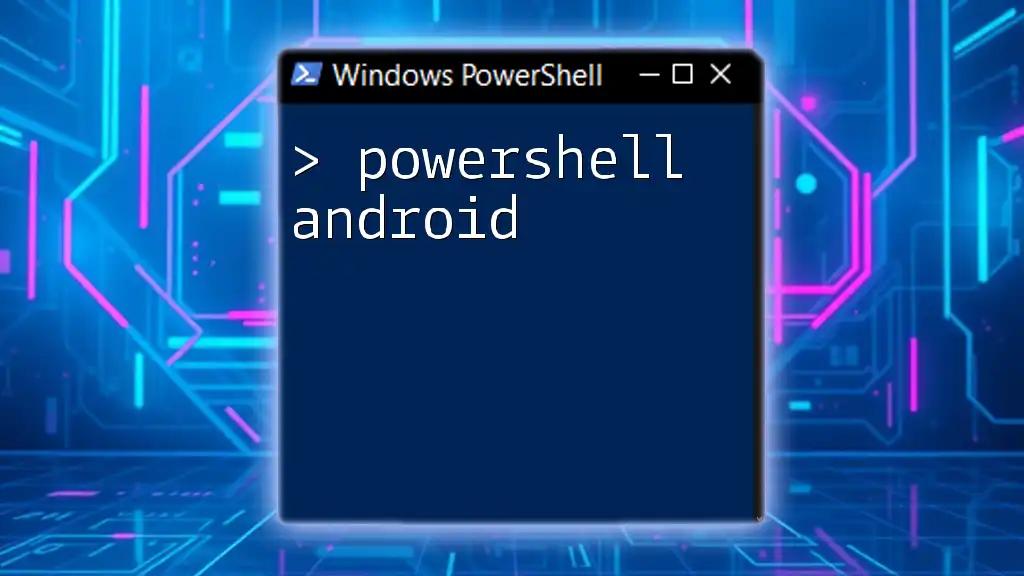
Getting Started with PowerShell DNS Commands
Installing the Required Modules
PowerShell requires specific modules to execute DNS commands. To install the necessary module, use the following command:
Install-WindowsFeature -Name RSAT-DNS-Server
This command ensures that the DNS Server tools are available on your system.
Loading the DNS Cmdlets
Before executing any DNS commands, ensure that the DNS cmdlets are loaded into your session with the following command:
Import-Module DnsServer
With the module imported, you're ready to start adding DNS records.
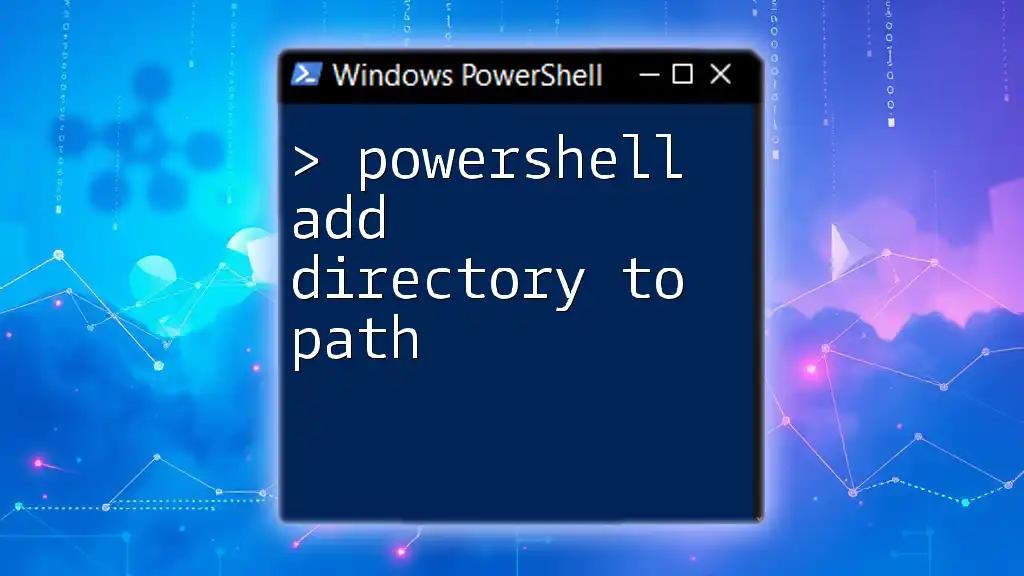
Adding a DNS Record
Using `Add-DnsServerResourceRecordA` to Add an A Record
An A Record is one of the most fundamental DNS record types. It maps a domain name directly to an IPv4 address, allowing users to access your site using its domain name.
To add an A Record, use the command:
Add-DnsServerResourceRecordA -Name "example" -IPv4Address "192.168.1.1" -ZoneName "example.com"
In this command:
- `-Name`: Specifies the subdomain you are adding (e.g., `example`).
- `-IPv4Address`: The IP address you want the domain to resolve to (e.g., `192.168.1.1`).
- `-ZoneName`: The zone in which you are adding the record, typically the domain name (e.g., `example.com`).
Adding Different Types of DNS Records
-
Adding a CNAME Record
A CNAME (Canonical Name) record allows you to alias one domain name to another, making it easier to manage services that may change over time.Example command to add a CNAME Record:
Add-DnsServerResourceRecordCName -Name "www" -HostNameAlias "example.com" -ZoneName "example.com"
Here, `-HostNameAlias` specifies the canonical domain name that your CNAME will point to.
-
Adding an MX Record
An MX (Mail Exchange) record directs email to the correct mail server based on the domain name.Use the following command to add an MX Record:
Add-DnsServerResourceRecordMX -Name "@" -MailExchange "mail.example.com" -Preference 10 -ZoneName "example.com"
In this case:
- `-MailExchange`: Specifies the mail server for routing emails.
- `-Preference`: Indicates the priority of the mail server (lower values mean higher priority).
-
Adding a TXT Record
TXT (Text) records can hold arbitrary text and are often used for verification or security policies.To add a TXT Record, you can use:
Add-DnsServerResourceRecordTXT -Name "@" -Text "v=spf1 include:_spf.example.com ~all" -ZoneName "example.com"
The `-Text` parameter allows you to input the necessary text, like SPF records for email security.
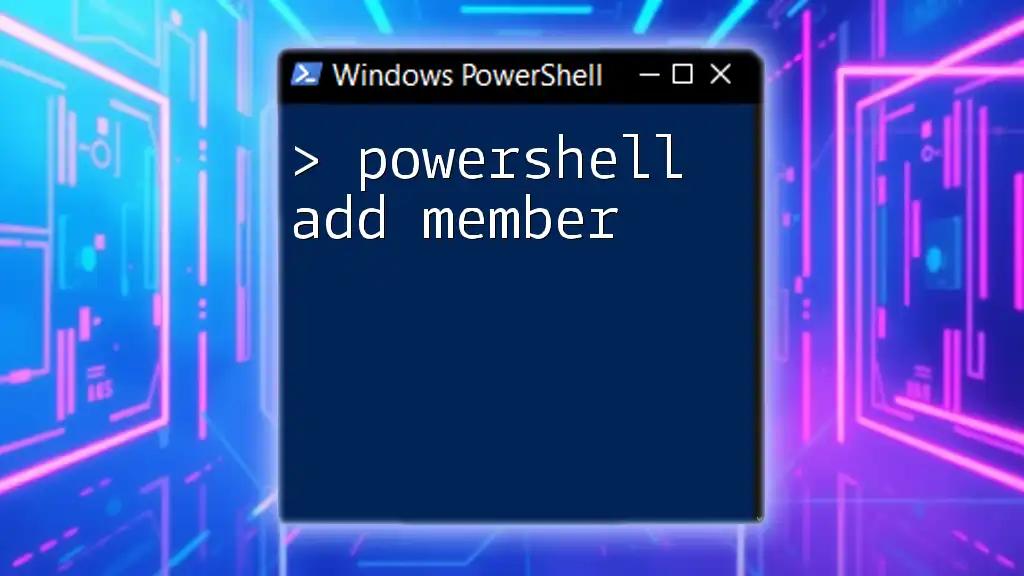
Verifying Added DNS Records
After adding DNS records, it's crucial to verify their existence and correctness. You can accomplish this using the command:
Get-DnsServerResourceRecord -ZoneName "example.com"
This command will list all DNS records within the specified zone. Look for your entries in the output to ensure they were added successfully.
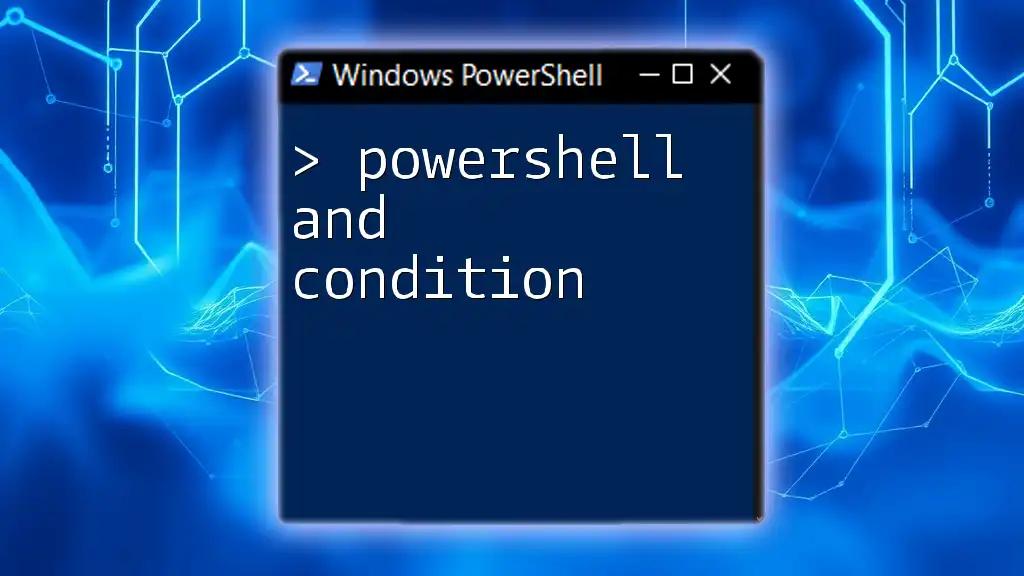
Troubleshooting Common Issues
Common Errors When Adding DNS Records
Sometimes you'll encounter errors when trying to add records. Common issues include:
- Access Denied: Make sure you have administrative permissions.
- Record Already Exists: Verify if the record is already in place using `Get-DnsServerResourceRecord`.
Validating DNS Changes
To confirm if your DNS changes have propagated, use command-line tools like `nslookup`:
nslookup example.com
This command queries the DNS to return the corresponding IP address and verifies if your records are active.
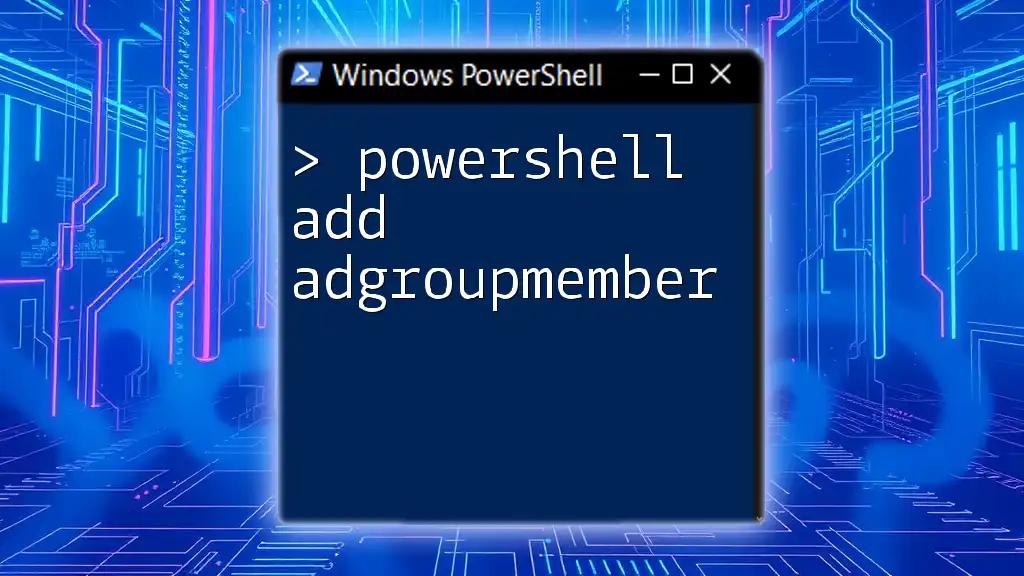
Best Practices for Managing DNS with PowerShell
Schedule Regular Backups of DNS Records
To maintain a stable DNS environment, backing up your DNS records is essential. Use the following command to export your DNS zone records:
Export-DnsServerZone -Name "example.com" -File "C:\Backup\example.com.dns"
This command creates a backup file, allowing you to restore records as needed.
Scripting for Automation
For repetitive tasks, consider scripting your commands. Below is a simple example that adds multiple A Records in a batch:
$records = @(
@{Name="example1"; IP="192.168.1.2"},
@{Name="example2"; IP="192.168.1.3"}
)
foreach ($record in $records) {
Add-DnsServerResourceRecordA -Name $record.Name -IPv4Address $record.IP -ZoneName "example.com"
}
This snippet creates an array of records and iterates through each entry, allowing for efficient DNS management.
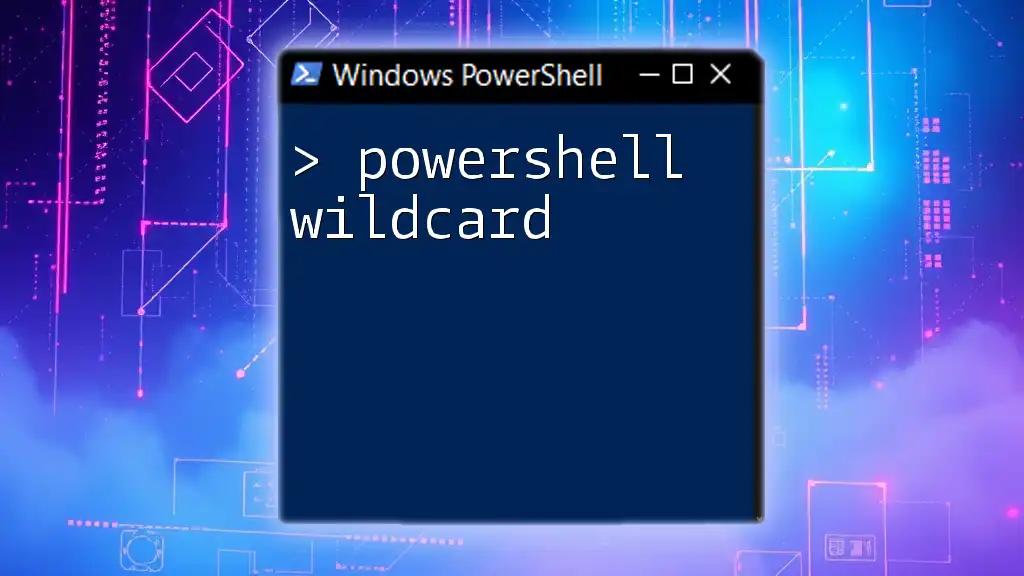
Conclusion
PowerShell provides a versatile and efficient way to manage DNS records, facilitating easier modifications and control. By leveraging the commands and understanding the underlying DNS concepts, you can ensure your domain-related tasks are handled proficiently. Embrace the power of scripting and automation to minimize manual efforts and enhance your DNS management capabilities.
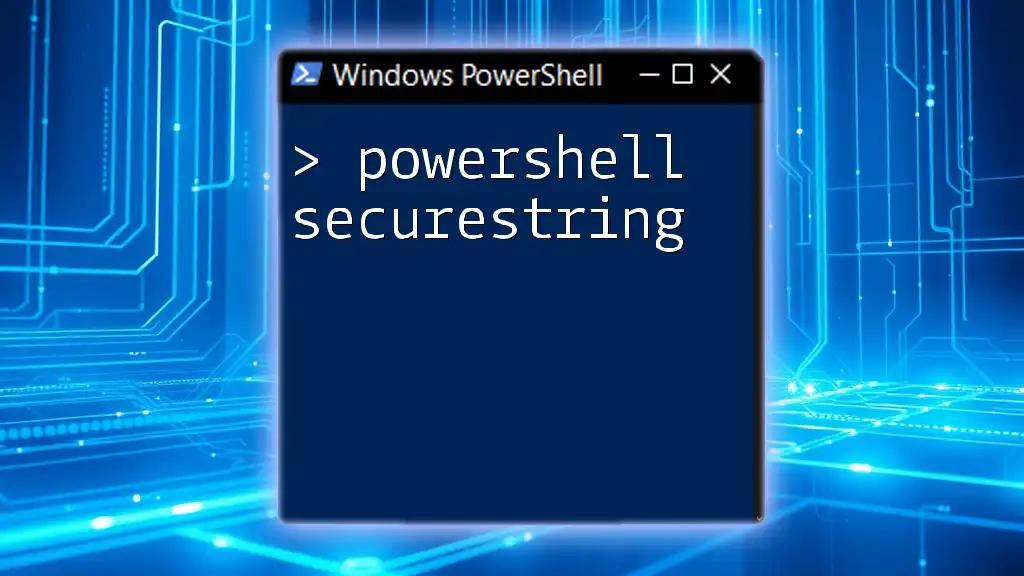
Additional Resources
- For more in-depth information, check Microsoft's official documentation on PowerShell DNS cmdlets.
- Engage in community forums or blogs dedicated to PowerShell for discussions and additional learning materials.