The `Add-Member` cmdlet in PowerShell is used to add properties and methods to an object, enhancing its functionality.
Here’s a simple code snippet demonstrating how to use `Add-Member`:
$myObject = New-Object PSObject -Property @{ Name = 'John'; Age = 30 }
$myObject | Add-Member -MemberType NoteProperty -Name 'City' -Value 'New York'
$myObject
This code creates a new object with properties 'Name' and 'Age', and then adds a new property 'City'.
Understanding the Add-Member Cmdlet
The Add-Member cmdlet is a powerful tool in PowerShell that allows users to add properties, methods, and events to existing objects. Understanding how to use this cmdlet effectively can significantly enhance your ability to manipulate and extend the functionality of objects within PowerShell.
Key Features of Add-Member
- Flexibility: You can add custom properties and methods to any object.
- Object-Oriented Programming: It supports the creation of complex objects, encapsulating data and functionality together.
- Enhancement of Output: Adding members can make the output of your scripts more meaningful and tailored to specific use cases.

Basic Syntax of Add-Member
The syntax of the Add-Member cmdlet can be summarized as follows:
Add-Member -InputObject <PSObject> -MemberType <String> -Name <String> -Value <Object>
Here’s a breakdown of the parameters:
- `-InputObject`: The object to which you want to add a member.
- `-MemberType`: Specifies the type of member you are adding (e.g., NoteProperty, ScriptMethod, etc.).
- `-Name`: The name of the member.
- `-Value`: The value for the new member.
For a basic example, you can create an object and add a new property to it:
$obj = New-Object PSObject
Add-Member -InputObject $obj -MemberType NoteProperty -Name "NewProperty" -Value "Hello, World!"
This code creates an object and adds a property named `NewProperty` with a value of `"Hello, World!"`.
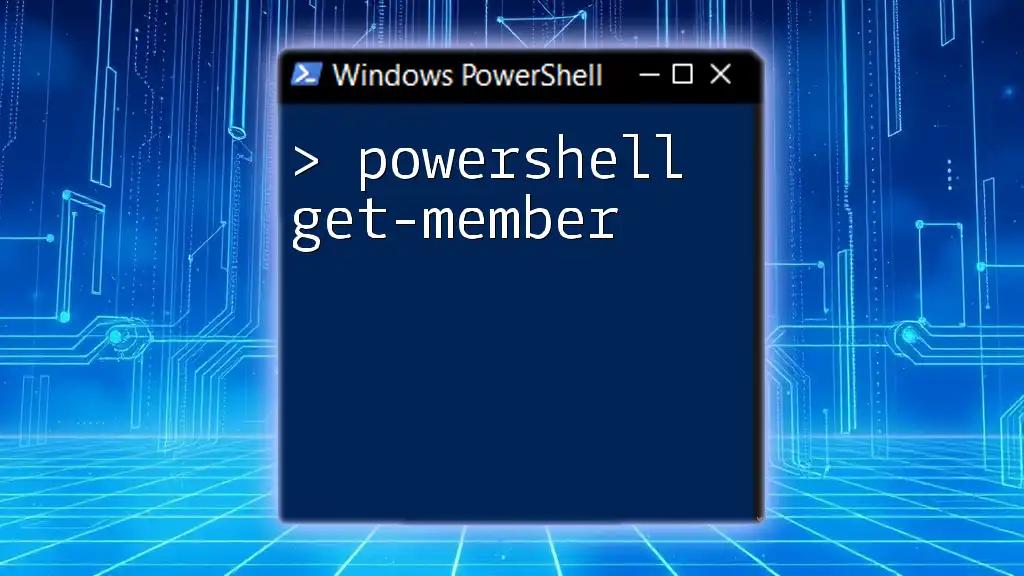
Different Member Types in Add-Member
NoteProperty
A NoteProperty is a simple property that holds data. It is commonly used when you want to store key-value pairs.
Example usage:
$person = New-Object PSObject
Add-Member -InputObject $person -MemberType NoteProperty -Name "FirstName" -Value "John"
In this case, we created a `person` object and added a property `FirstName` with a value of `John`.
Property
A Property is somewhat similar to a NoteProperty but is designed to encapsulate more advanced behaviors.
Example:
$car = New-Object PSObject
Add-Member -InputObject $car -MemberType Property -Name "Color" -Value "Red"
Here we demonstrate how to use a `Property` type member, which allows for further extensibility.
Method
Method members allow you to embed functionality directly into your objects. This is key for object-oriented programming.
When adding a method, the syntax looks like:
$math = New-Object PSObject
Add-Member -InputObject $math -MemberType ScriptMethod -Name "Add" -Value { param($x, $y) return $x + $y }
In this case, we've added a method `Add` that takes two parameters and returns their sum.
ScriptProperty
A ScriptProperty provides a way to calculate a value on-the-fly whenever the property is accessed. This is useful for computed values that depend on current object state.
Example:
$item = New-Object PSObject
Add-Member -InputObject $item -MemberType ScriptProperty -Name "Square" -Value { return $this.Value * $this.Value }
In this example, the `Square` property will execute the script provided to compute its value whenever accessed.
AliasProperty
An AliasProperty allows you to bind multiple member names to the same value. It can be useful for maintaining backward compatibility or offering multiple names for the same data.

Practical Examples of Using Add-Member
Building Custom Objects with Properties
Creating complex objects with multiple properties can help to organize your data effectively.
$user = New-Object PSObject
Add-Member -InputObject $user -MemberType NoteProperty -Name "Username" -Value "alice"
Add-Member -InputObject $user -MemberType NoteProperty -Name "Email" -Value "alice@example.com"
In this example, you have created a user object containing both a username and email property.
Creating Complex Objects with Methods
We can also encapsulate functionalities directly into our objects through methods.
$counter = New-Object PSObject
Add-Member -InputObject $counter -MemberType ScriptMethod -Name "Increment" -Value { $this.Count++ }
This allows the `counter` object to increment its `Count` property through an encapsulated method.
Combining Add-Member with Other Cmdlets
You can create more efficient scripts by combining Add-Member with other cmdlets.
For example, consider using it in a pipeline:
Get-Process | ForEach-Object {
Add-Member -InputObject $_ -MemberType NoteProperty -Name "Timestamp" -Value (Get-Date)
}
This approach enriches each process object with a `Timestamp` property, providing additional context.
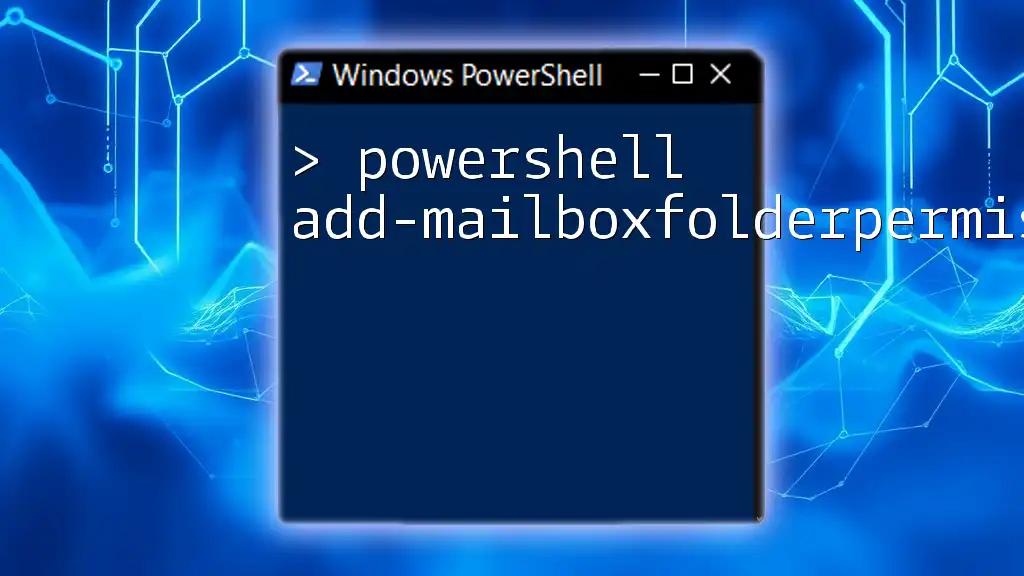
Best Practices When Using Add-Member
When using Add-Member, adhering to best practices is crucial for clarity and maintainability:
- Use descriptive names for added members to clarify their purpose.
- Choose appropriate member types thoughtfully, based on functionality requirements.
- In cases of large datasets, be aware of performance implications when adding properties dynamically.
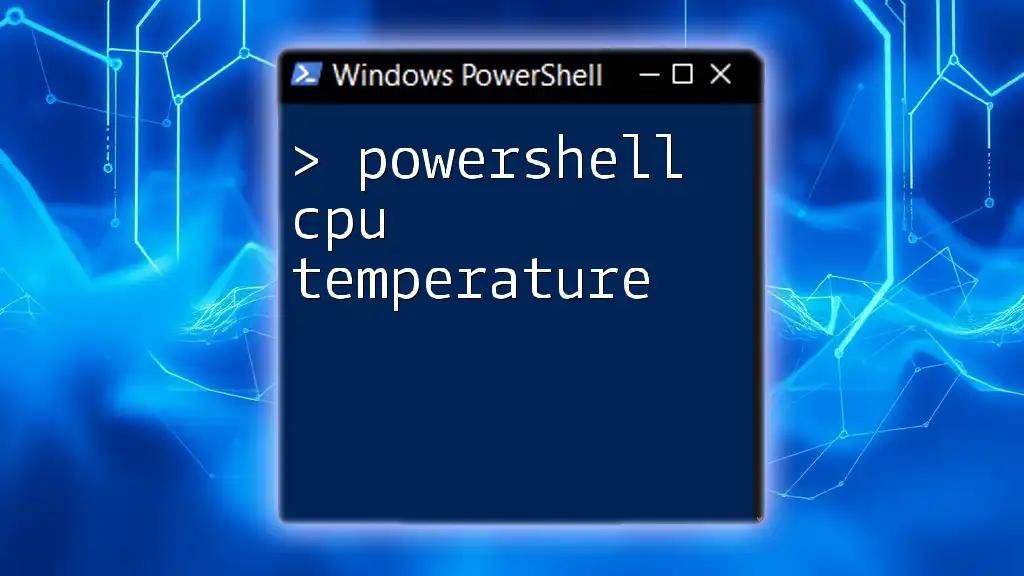
Common Errors and Troubleshooting Tips
PowerShell's flexibility can sometimes lead to mistakes. Here are some common pitfalls:
- Duplicate member names: Adding a member with a name that already exists can lead to errors. Always check existing members first.
- Incorrect member types: Trying to add a value of the wrong type for a specified member can cause issues. Make sure to choose a member type that matches your data requirements.
To debug issues, utilize `Get-Member` to inspect the members present on objects, assisting in identifying what might be wrong.
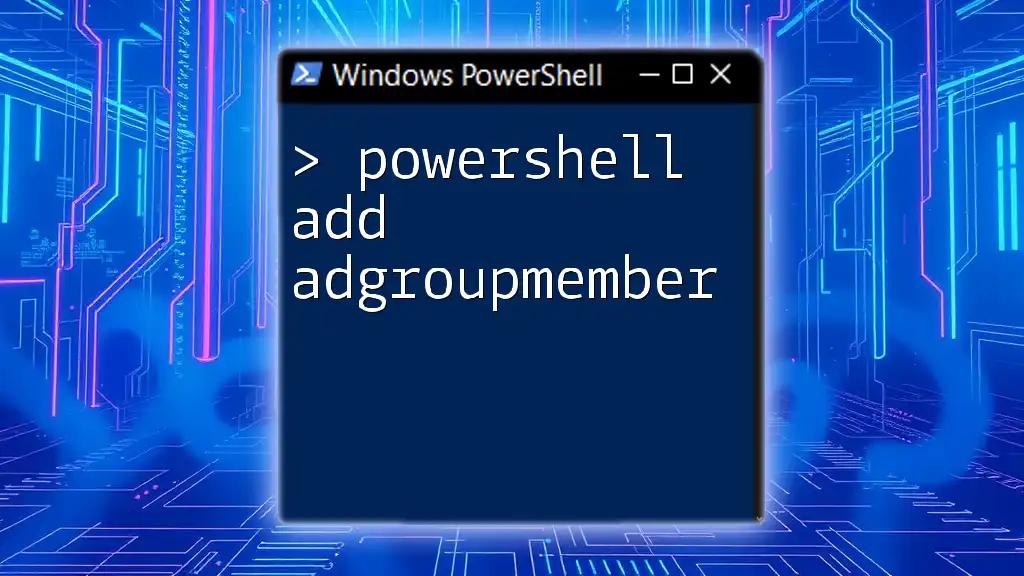
Conclusion
The PowerShell Add-Member cmdlet is an essential feature for anyone looking to extend the capabilities of objects within their scripts. From simple property additions to complex methods, mastering this cmdlet can significantly enhance your PowerShell scripting capabilities. By practicing and exploring the various member types, you will be better equipped to create dynamic, custom objects that meet your unique requirements.
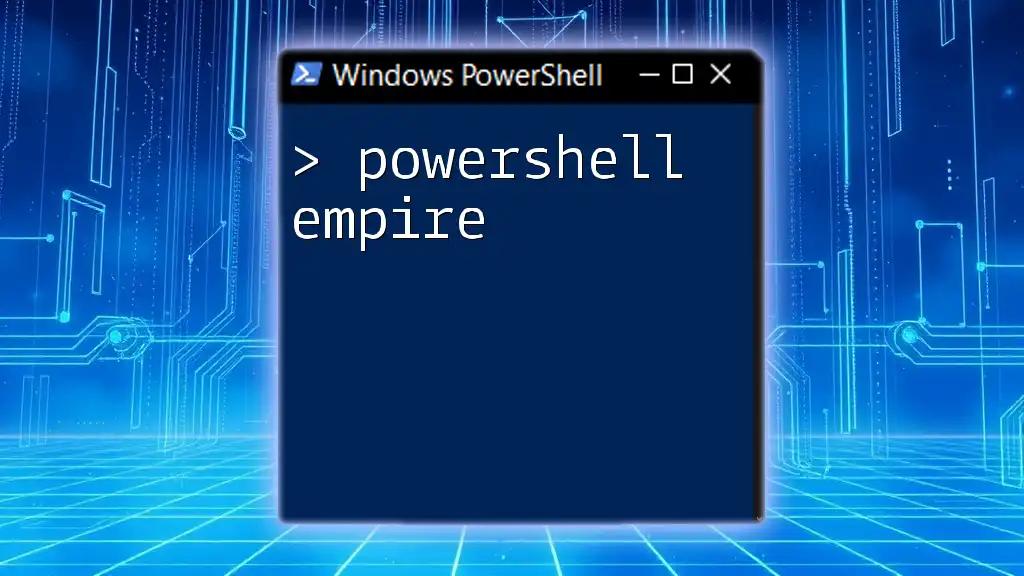
Additional Resources
For further learning, consider checking the official PowerShell documentation, community forums, dedicated blogs, and recommended books geared towards enhancing your PowerShell skills. Engaging with these resources will help solidify your understanding and application of key concepts like Add-Member.