To add members to a distribution group in PowerShell, you can use the `Add-DistributionGroupMember` cmdlet, as shown in the following code snippet:
Add-DistributionGroupMember -Identity "YourDistributionGroup" -Member "user1@example.com", "user2@example.com"
Understanding Distribution Groups
What is a Distribution Group?
A distribution group is a collection of email addresses that allows users to send emails to multiple recipients without having to add each address individually. This is particularly useful in workplace communication, where announcements, newsletters, and collaborative information need to be shared with several users at once.
Types of Distribution Groups
There are two main types of groups you might encounter in a Microsoft environment: security groups and distribution groups.
- Security Groups are used to manage user rights and access to resources. They can also be used for email purposes but are primarily focused on granting permissions.
- Distribution Groups, on the other hand, are specifically designed for email communication and do not grant any access rights.
Choosing the correct type of group for your needs is crucial to ensure that communications are directed properly.
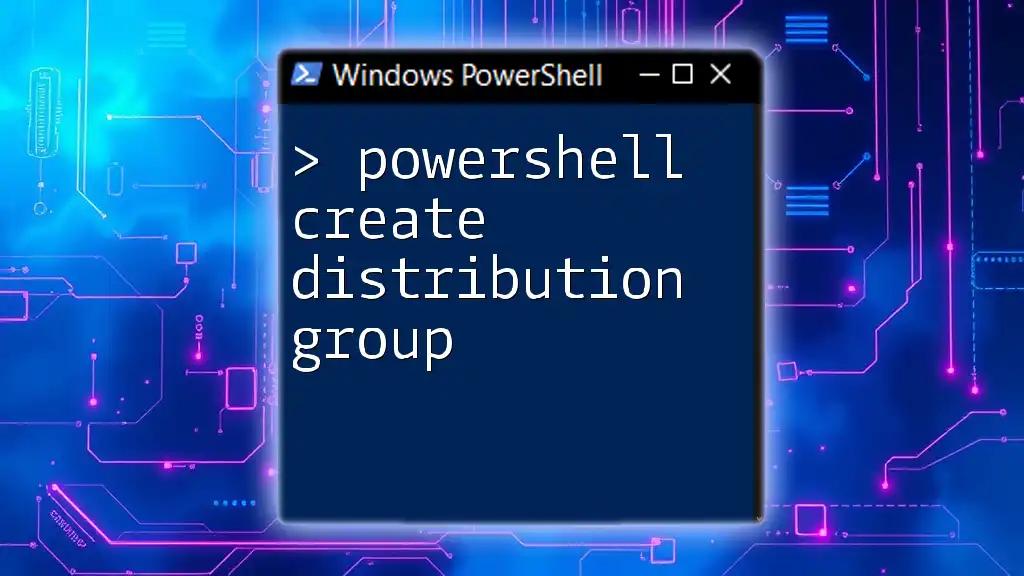
Getting Started with PowerShell
Prerequisites for Using PowerShell
Before you dive into adding members to distribution groups, it’s essential to understand the required permissions. Typically, you will need to be a member of the Exchange Administrators or Organization Management role group to manage these groups in Exchange Online or on-premises Exchange.
Installing Required Modules
To effectively use PowerShell for managing your distribution groups, you might need to install specific modules. The Exchange Online Management module is one of the critical tools you'll require.
To install this module, run the following command in PowerShell:
Install-Module -Name ExchangeOnlineManagement
This command will allow you to access a variety of Exchange-related commands, facilitating your work with distribution groups.
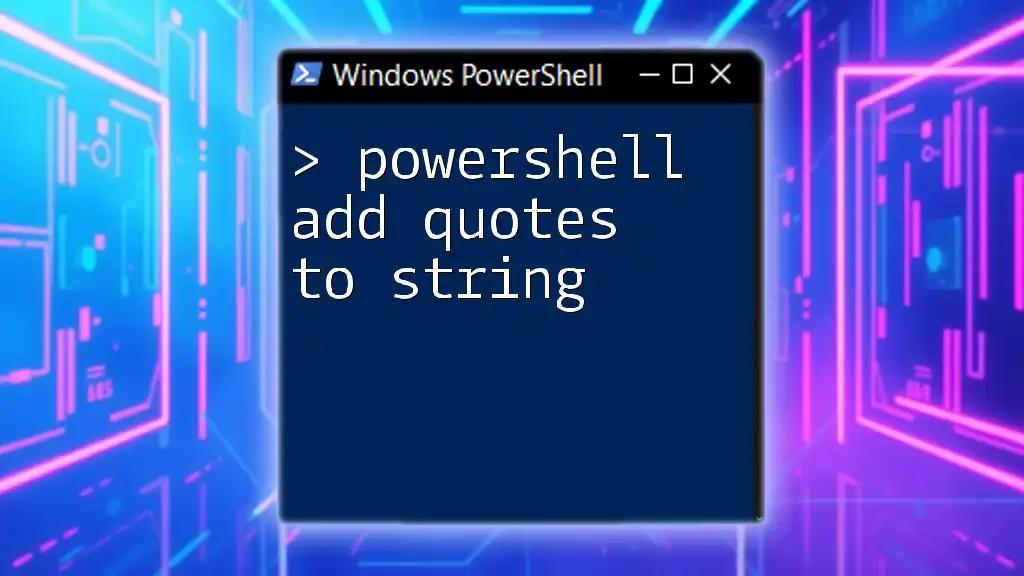
Basic Commands for Managing Distribution Groups
Finding Distribution Groups
To manage distribution groups effectively, you first need to identify the groups you wish to work with. You can list all distribution groups in your environment using the following command:
Get-DistributionGroup
This command will return a list of all distribution groups, including their names, email addresses, and other vital information. Understanding this output is critical for selecting the appropriate group when adding members.
Adding Members to a Distribution Group
Using PowerShell
The core command for adding users to a distribution group is straightforward. You can use the following syntax:
Add-DistributionGroupMember -Identity "GroupName" -Member "UserEmail"
- `-Identity` specifies the distribution group you want to modify.
- `-Member` is the user you wish to add.
For example, if you wanted to add a user named "john.doe@example.com" to a group called "MarketingTeam," your command would look like this:
Add-DistributionGroupMember -Identity "MarketingTeam" -Member "john.doe@example.com"
This simple command will immediately update the group’s membership.
Bulk Adding Members
If you need to add multiple users at once, PowerShell can streamline this for you. By using a CSV file containing the user email addresses, you can quickly bulk-add members to a distribution group.
Here’s how to do it:
- Prepare a CSV file (`users.csv`) with an EmailAddress header:
EmailAddress
john.doe@example.com
jane.smith@example.com
- Use the following PowerShell command to import and add all listed users to the desired group:
Import-Csv "C:\Path\To\users.csv" | ForEach-Object {
Add-DistributionGroupMember -Identity "MarketingTeam" -Member $_.EmailAddress
}
This command will read each email address from the CSV and add them to the specified distribution group.
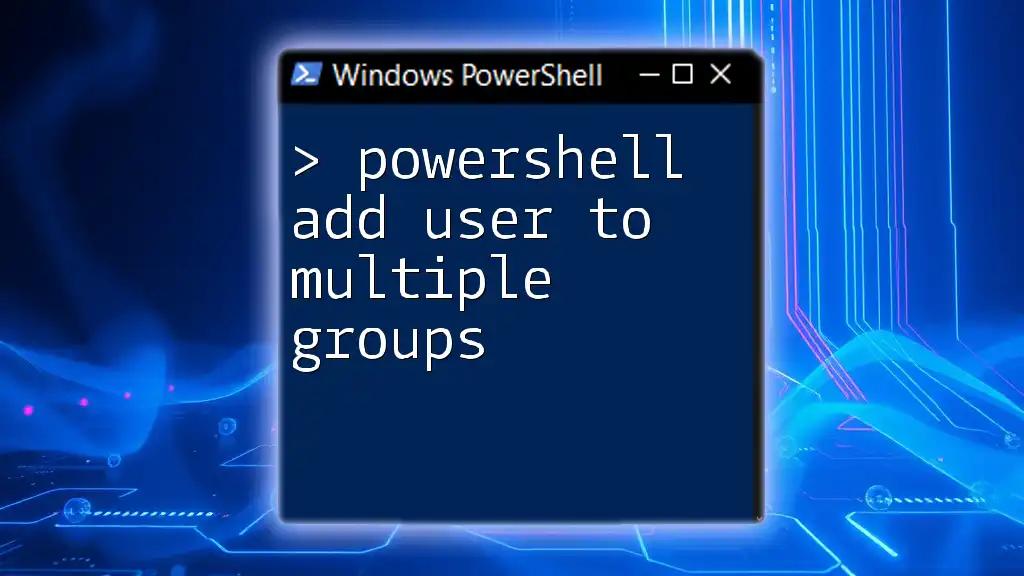
Handling Common Issues
Troubleshooting Errors
During the process of adding members to distribution groups, various errors may arise. One common error is when a user cannot be found. This typically occurs if the entered email address is incorrect or the user does not exist in the organization.
To troubleshoot, double-check the email address for any typos or check the user’s existence using:
Get-Mailbox "UserEmail"
Permissions Issues
Another common problem can stem from permissions. If you don’t have the necessary rights to add users to a distribution group, PowerShell will return an error. In such cases, you may need to contact your IT administrator to ensure that your role encompasses the required permissions.

Best Practices for Managing Distribution Groups
Regular Updates
To maintain effective communication through distribution groups, it's important to keep the membership updated regularly. Each quarter or after significant organizational changes, take the time to review the group memberships for relevancy and accuracy.
Documentation
Documenting changes made to distribution groups is a best practice that helps in maintaining clarity and accountability within your organization. Keep a log that includes changes such as users added or removed, the date of modification, and the reason for these changes.
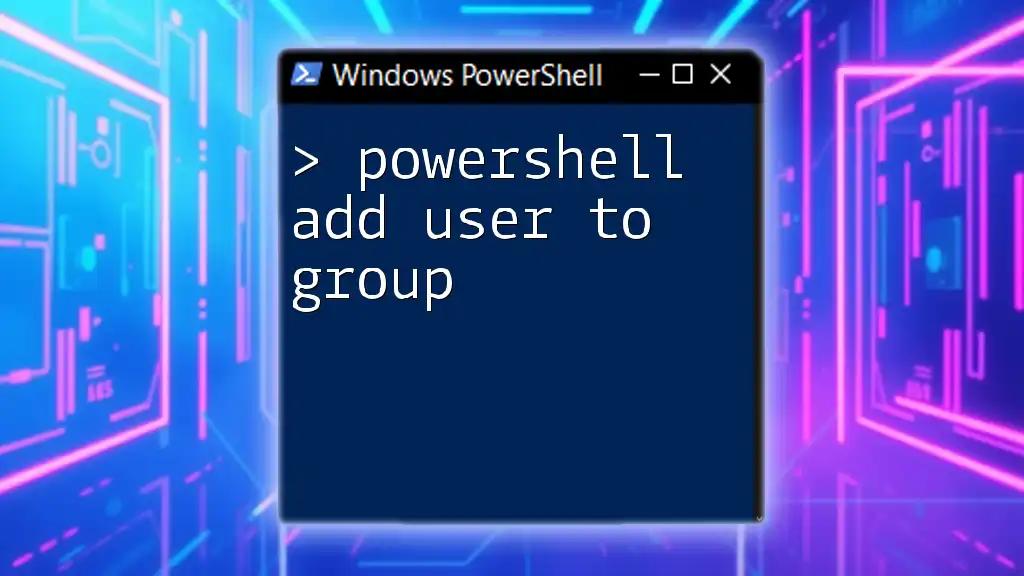
Summary
In summary, effectively managing distribution groups through PowerShell can significantly streamline communication within an organization. By understanding the commands and best practices highlighted in this guide, you can enhance your administrative skills and ensure that your teams are communicating effectively.
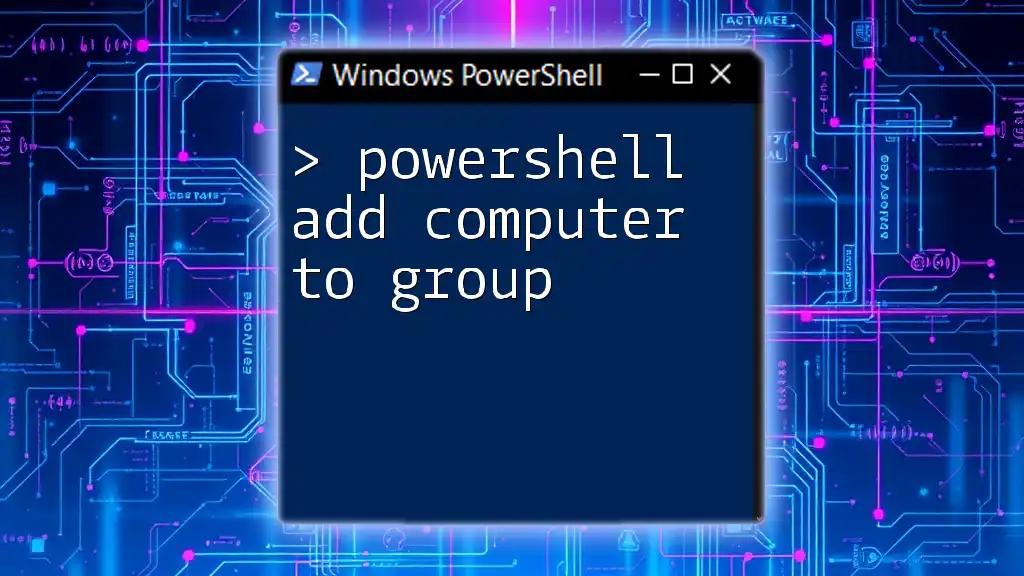
Additional Resources
For further learning and development, consider delving into PowerShell documentation, forums, and community resources. These platforms offer valuable insights and can aid in troubleshooting when issues arise.

Conclusion
Mastering PowerShell for adding members to distribution groups is not only beneficial for your professional skill set but is also essential for promoting seamless communication in any organization. As you continue to practice and refine your skills, you will become more proficient in utilizing PowerShell effectively for various administrative tasks. Don't hesitate to share your newfound knowledge with peers or explore more advanced features of PowerShell for greater productivity.