To add a user to multiple Active Directory groups using PowerShell, you can use the `Add-ADGroupMember` cmdlet in a loop or array format.
Here's a code snippet for that purpose:
$user = "username"
$groups = "Group1", "Group2", "Group3"
foreach ($group in $groups) { Add-ADGroupMember -Identity $group -Members $user }
Understanding User Groups in PowerShell
What Are User Groups?
User groups in Windows environments are collections of user accounts that simplify permissions management. By grouping users, system administrators can easily assign or deny access to resources based on a collective identifier rather than managing individual accounts. This practice not only streamlines operations but also enhances security by minimizing the chances of misconfiguration.
Common Use Cases for Adding Users to Groups
There are several scenarios where adding users to multiple groups can be beneficial:
- Organizational Structure: Aligning employees with specific departments (e.g., Sales, IT, HR) ensures that access permissions reflect company hierarchy.
- Project-Based Access Management: For short-term projects, users can be added to project-specific groups, facilitating easy access to related resources.
- Role-Based Access Control: Users can be assigned to groups that reflect their job roles, ensuring they have the necessary access without compromising security.
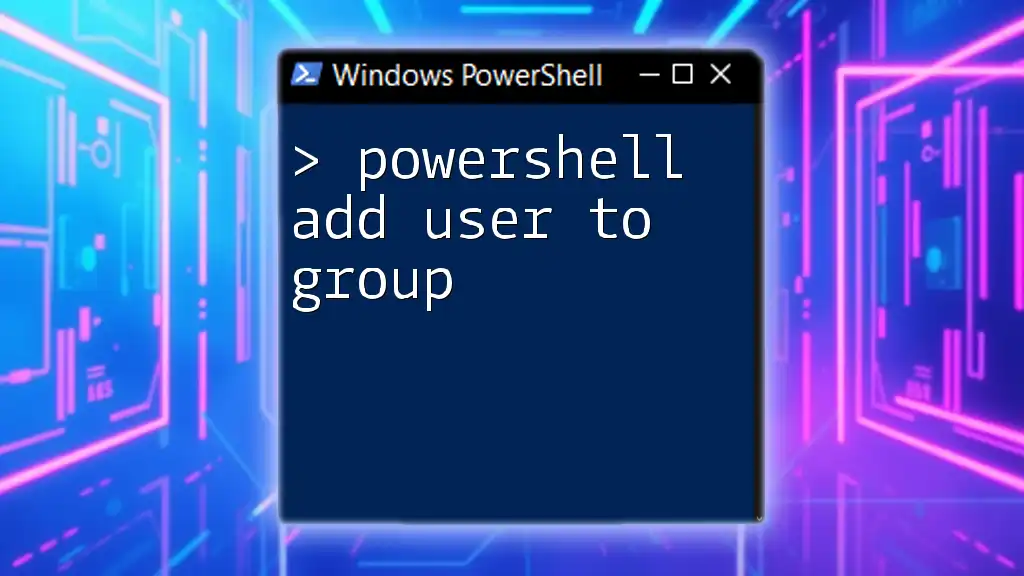
Prerequisites for Adding Users to Groups
Required Permissions
Before you can successfully add a user to multiple groups, it's crucial to have the appropriate permissions. Administrative privileges are often required to modify group memberships, so ensure you're logged in as a user with these permissions.
Understanding the PowerShell Environment
PowerShell must be properly configured for group management. To check if the Active Directory module is installed, you can run:
Get-Module -ListAvailable | Where-Object { $_.Name -eq "ActiveDirectory" }
If the module is not available, you may need to install it as part of the Remote Server Administration Tools (RSAT).
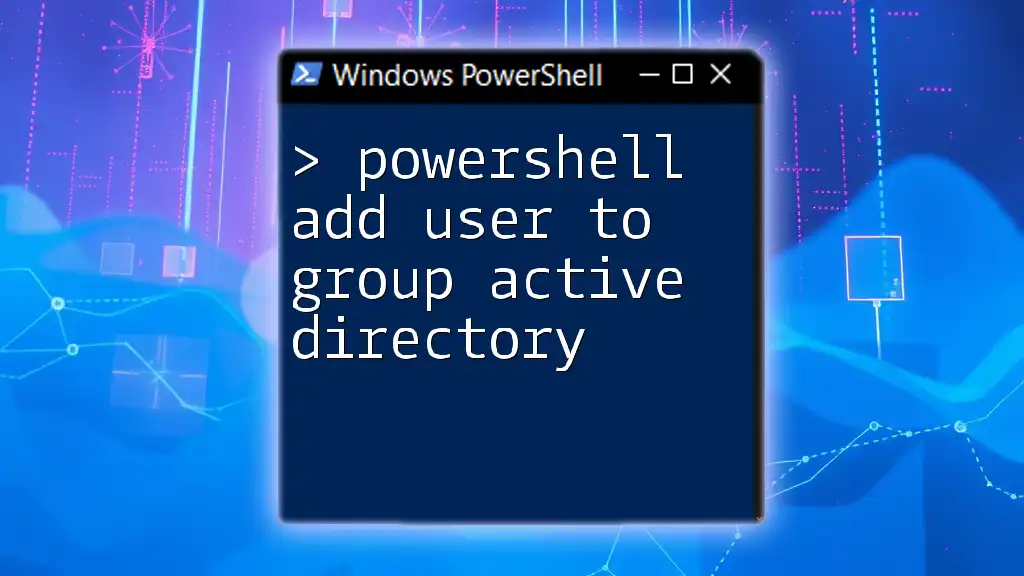
Adding a User to Multiple Groups in PowerShell
Basic Syntax for Adding a User to a Single Group
To add a user to a single group, the `Add-ADGroupMember` cmdlet is used. The basic syntax is:
Add-ADGroupMember -Identity "GroupName" -Members "UserName"
In this command:
- `-Identity` specifies the target group.
- `-Members` identifies the user being added.
Adding a User to Multiple Groups
Using a Simple Array
To add a single user to multiple groups in one go, you can use an array. This method is highly efficient for organizations where one user needs access to several groups.
Example Code Snippet:
$groups = "Group1", "Group2", "Group3"
foreach ($group in $groups) {
Add-ADGroupMember -Identity $group -Members "UserName"
}
In this example, we define an array called `$groups`, which includes the names of multiple groups. The `foreach` loop iterates over each group, executing the `Add-ADGroupMember` command for the specified user.
Leveraging a CSV File for Bulk Operations
For bulk operations, especially in larger environments, using a CSV file is incredibly efficient. CSV files allow you to list multiple group and user combinations which can be processed in a loop.
Example Format of a CSV File:
UserName,GroupName
User1,Group1
User1,Group2
User2,Group1
Example Code Snippet:
Import-Csv "C:\Users\yourusername\Desktop\groups.csv" | ForEach-Object {
Add-ADGroupMember -Identity $_.GroupName -Members $_.UserName
}
In this example, we import the CSV file using `Import-Csv`. The `ForEach-Object` cmdlet processes each row, adding the corresponding user to the designated group as defined in the CSV.
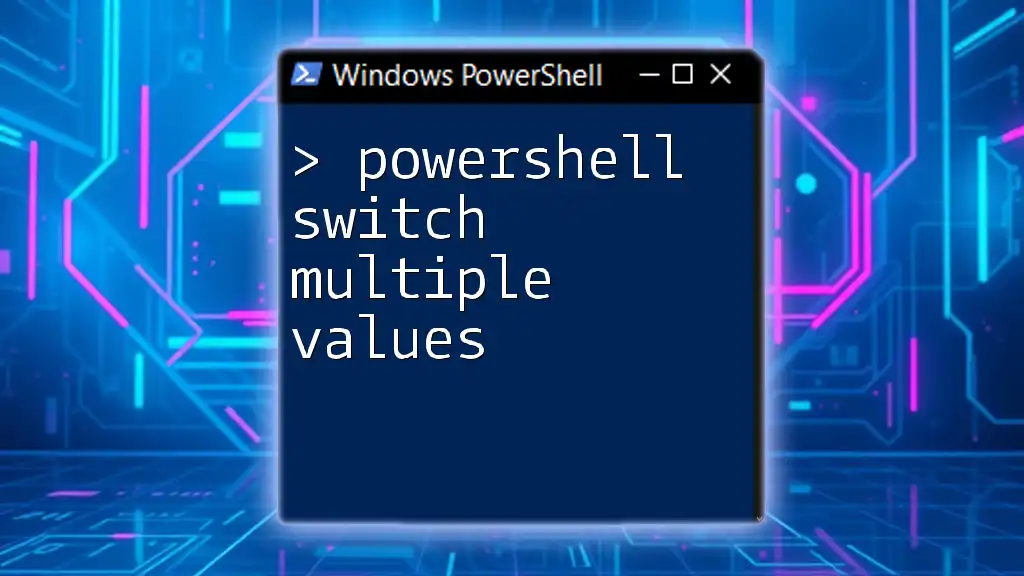
Checking User Membership in Groups
Verifying Group Membership
After adding users to groups, you may want to confirm those changes. The `Get-ADGroupMember` cmdlet allows you to verify group membership.
Code Snippet:
Get-ADGroupMember -Identity "GroupName"
This command will list all members of the specified group, allowing you to visually confirm that the desired users have been added successfully.
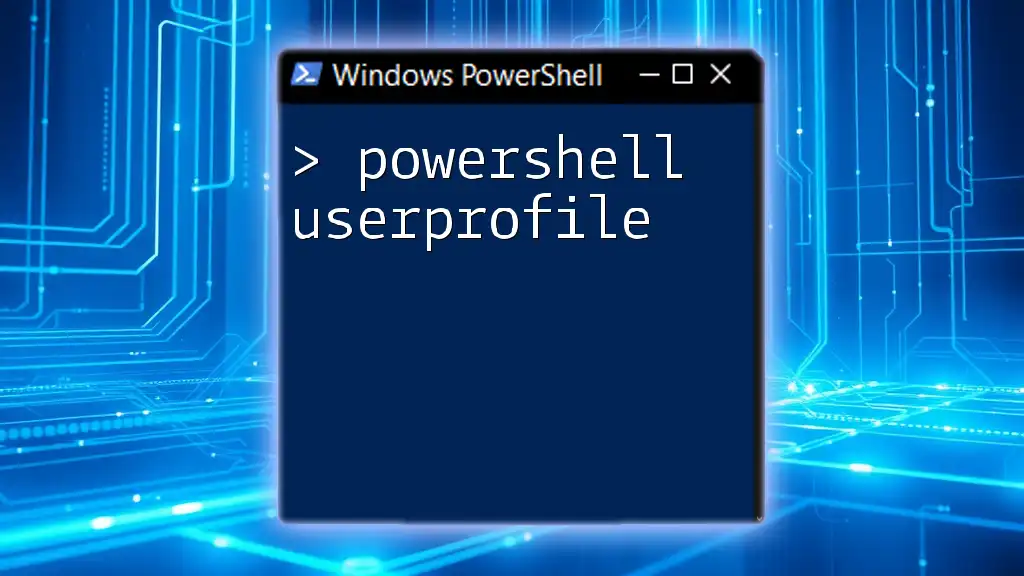
Handling Errors and Troubleshooting
Common Errors When Adding Users to Groups
Adding users to groups can sometimes result in errors, such as:
- User Not Found: This happens if the username specified does not exist in the Active Directory.
- Insufficient Permissions: This error arises when the current user does not have administrative rights.
Using Try-Catch for Error Handling
Incorporating error handling into your scripts is critical for troubleshooting mistakes gracefully.
Code Snippet:
try {
Add-ADGroupMember -Identity $group -Members "UserName"
} catch {
Write-Host "Error adding user to group: $_"
}
In this example, the `try-catch` block attempts to add the user to the group. If an error occurs, it catches the exception and outputs a relevant message, allowing the script to continue executing.
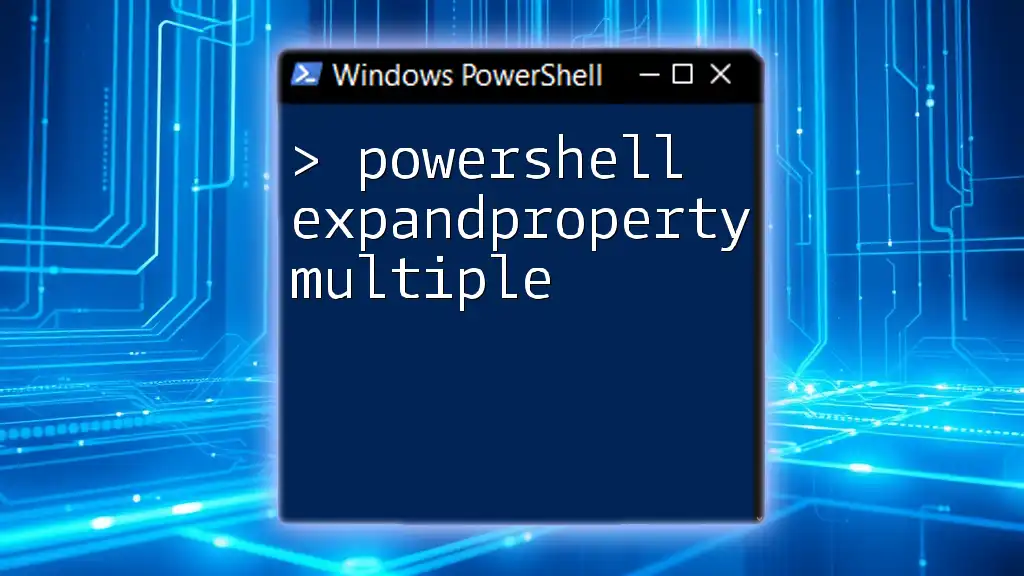
Best Practices for Managing Users in PowerShell
Regular Audits of Group Membership
Maintaining accurate group membership is fundamental for security and compliance. Periodically reviewing group memberships helps avoid unnecessary access and ensures that only the right users have privileges.
Documentation and Script Management
Keeping a log of permissions changes, script executions, and modifications to user groups is crucial. Implement version control and backup strategies to track changes effectively, which aids in understanding past configurations and future planning.
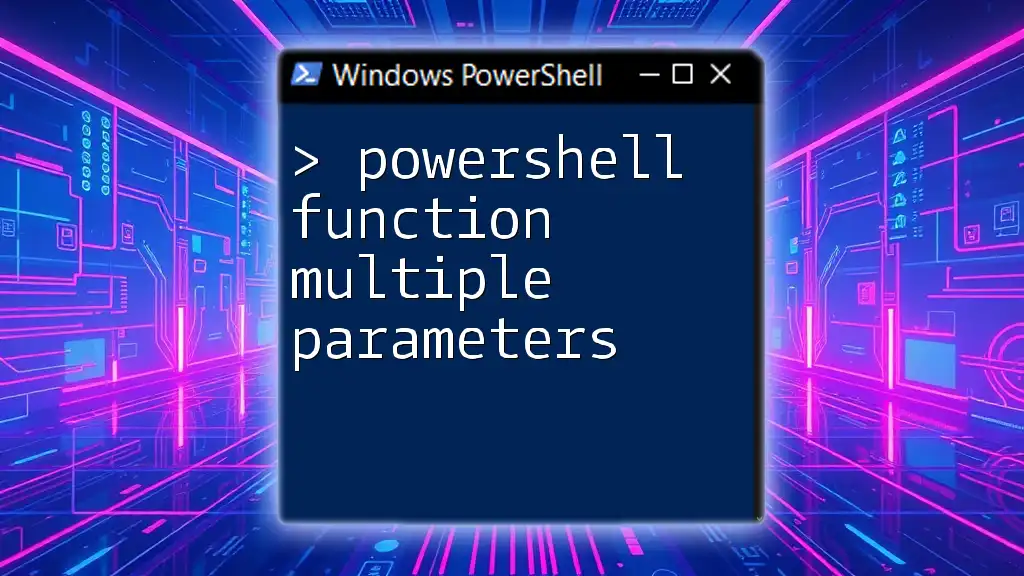
Conclusion
Adding a user to multiple groups in PowerShell can be accomplished efficiently with proper scripting techniques. By leveraging the power of PowerShell and following best practices, you can streamline user management and enhance your organization’s access control. With ongoing learning and practice, you can transform how your organization manages its resources through effective group membership strategies.
Additional Resources
For those looking to deepen their understanding of PowerShell and group management, consider exploring the official Microsoft documentation and other reputable online courses dedicated to mastering PowerShell. Support options and community forums can also provide valuable assistance as you continue your learning journey.