You can easily add users to a group from a CSV file in PowerShell by importing the CSV and using a loop to execute the `Add-LocalGroupMember` command for each user.
Here’s a concise example:
$users = Import-Csv -Path 'C:\path\to\your\file.csv'; foreach ($user in $users) { Add-LocalGroupMember -Group 'YourGroupName' -Member $user.Username }
Understanding CSV Files for User Data
What is a CSV File?
A Comma-Separated Values (CSV) file is a simple text format used to store tabular data, such as user accounts for Active Directory. This format is favored for its ease of use and universal compatibility across various applications, which makes it ideal for batch operations.
Structuring the CSV File
To effectively use a CSV file for adding users to an AD group, ensure that it has the correct structure. A well-structured CSV file might look like this:
Username,GroupName
jdoe,Marketing
asmith,Sales
bparker,Engineering
Each row contains the username and the corresponding group to which you wish to add the user. Pay attention to formatting: headers must exactly match the property names you will reference in your PowerShell script.
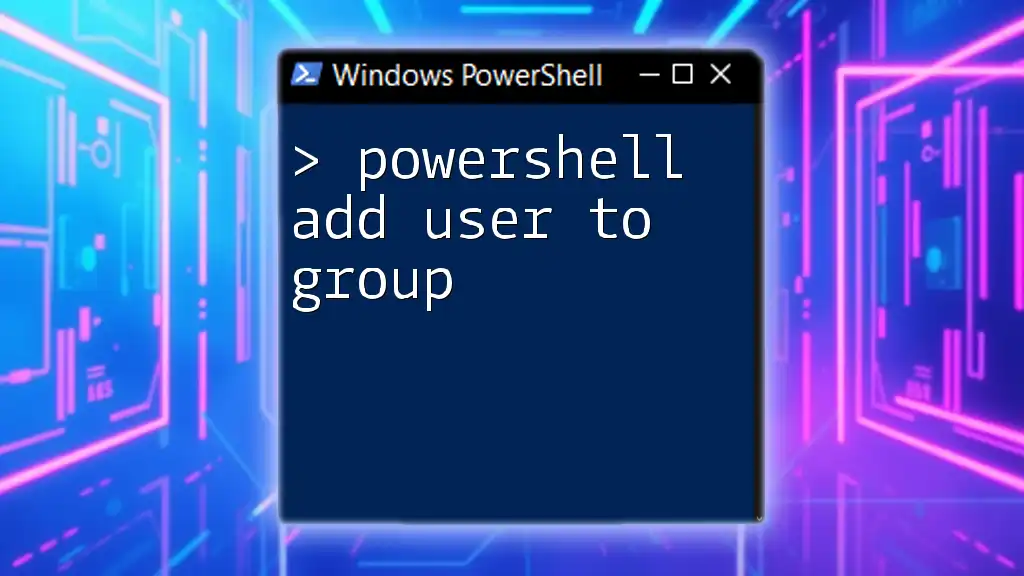
Prerequisites for PowerShell Commands
Required Permissions
Before you start adding users to an AD group, you must ensure that your account has the necessary permissions. Typically, you need to be a member of the Domain Admins group or have specific delegated permissions to modify the group memberships in Active Directory.
Installing the Necessary PowerShell Modules
For working with Active Directory, you need to have the Active Directory Module for Windows PowerShell installed. You can import the module using the following command:
Import-Module ActiveDirectory
This command loads the required cmdlets that allow you to manipulate AD objects, including groups and users.
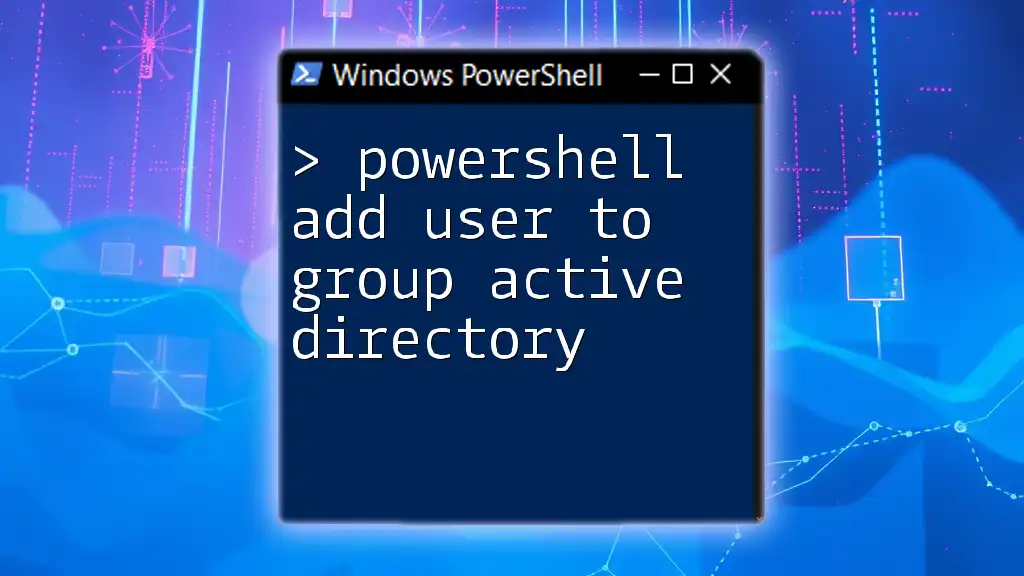
Writing the PowerShell Script to Add Users
Basic Template for the Script
The main task is to write a script that reads user data from a CSV file and adds each user to the specified group. Here's a quick template you can use:
# Import the CSV file
$users = Import-Csv -Path "C:\Path\To\Your\File.csv"
# Iterate through each user and add to specified group
foreach ($user in $users) {
$username = $user.Username
$groupname = $user.GroupName
# Adding user to group
Add-ADGroupMember -Identity $groupname -Members $username
}
Detailed Explanation of Each Component of the Script
Import-Csv Command
The `Import-Csv` cmdlet reads the contents of the CSV file and converts it into an array of PSObjects. Each row becomes an object, with the properties corresponding to the column headers. This cmdlet is essential for processing the user and group data efficiently.
The Foreach Loop
The `foreach` loop is a critical component of the script, allowing you to iterate through each user object created by `Import-Csv`. Within the loop, you assign the values from the CSV to variables (`$username` and `$groupname`), which are then used in the next step of the process. This approach ensures that each user is processed individually.
Add-ADGroupMember Cmdlet
The `Add-ADGroupMember` cmdlet is what actually performs the action of adding a user to the specified group. It takes two main parameters:
- `-Identity`: This specifies the group you want to add users to.
- `-Members`: This indicates which user(s) you want to add.
It's crucial to ensure that both the username and group name are valid; otherwise, you'll encounter errors.
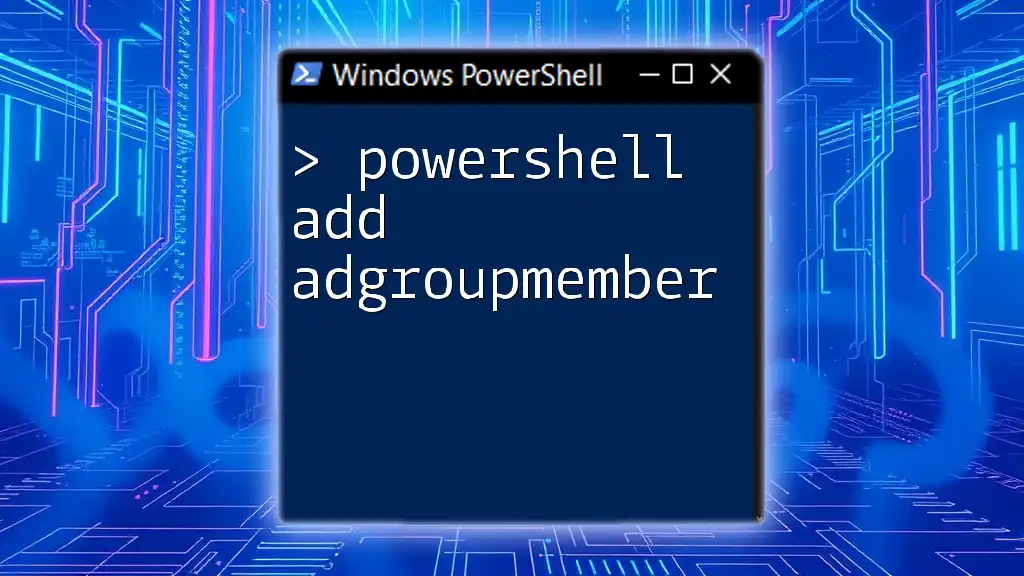
Error Handling and Troubleshooting
Common Errors When Adding Users to Groups
While running the script, you might encounter some prevalent issues, such as:
- User Not Found: This may happen if the username in the CSV doesn't match any user account in Active Directory.
- Group Does Not Exist: If the group specified doesn't exist in AD, the script will fail to add the user.
Implementing Error Handling in the Script
To make your script more robust, you can incorporate Try-Catch blocks for error handling. This allows you to gracefully handle exceptions and log errors for further review. Here’s how you can extend your script:
try {
Add-ADGroupMember -Identity $groupname -Members $username -ErrorAction Stop
} catch {
Write-Host "Error adding user $username to group $groupname: $_"
}
The `-ErrorAction Stop` parameter forces the cmdlet to throw an error when it encounters an issue, which is then caught by the catch block. The error message will display the user and group that caused the problem.
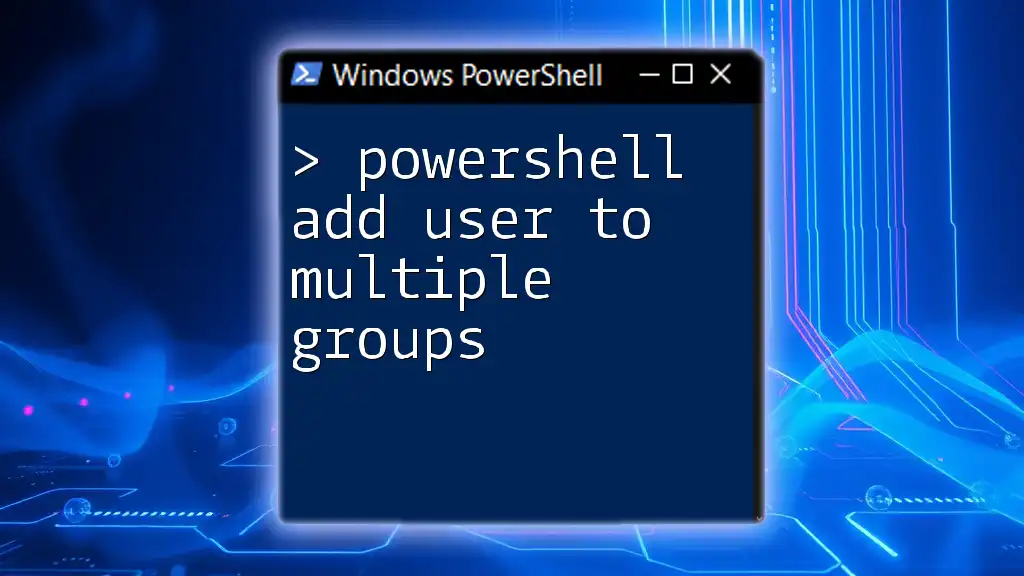
Testing the Script
Running the Script in a Test Environment
Before running your script in a production environment, it's advisable to test it in a controlled setting. You can replicate your setup on a test server or use a set of dummy accounts and groups to see how the script performs without the risk of impacting live data.
Verifying User Addition
After running your script, it’s critical to verify that the users were successfully added to the respective groups. You can do this using the `Get-ADGroupMember` cmdlet:
Get-ADGroupMember -Identity $groupname
This command lists all members of the specified group, allowing you to confirm the additions.
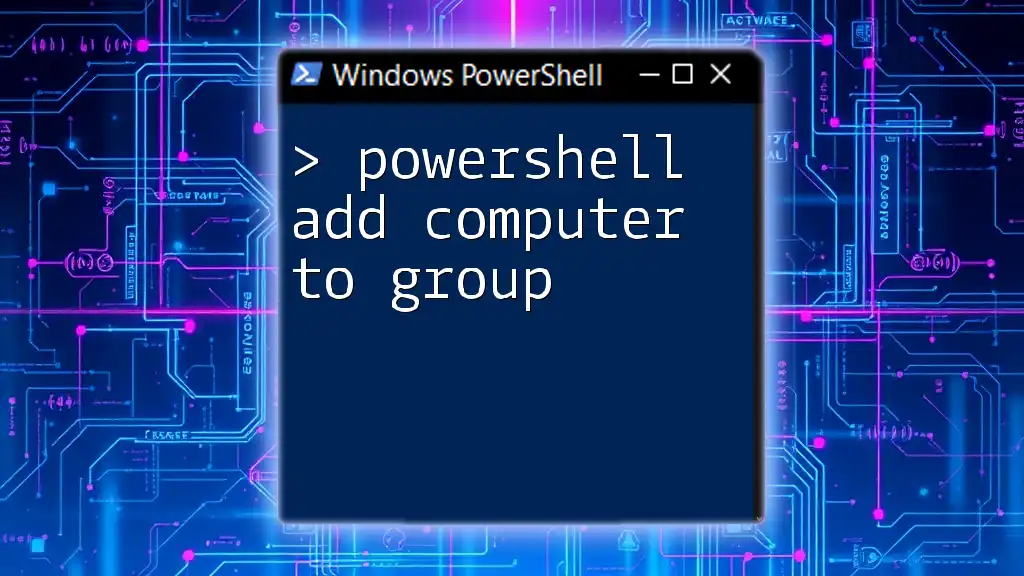
Conclusion
Using PowerShell to add users to an Active Directory group from a CSV file streamlines the management of user accounts and group memberships. By following the outlined steps and employing best practices, you can effectively perform bulk operations, saving time and reducing administrative overhead.
Feel free to adapt the script to suit your needs, and as you become comfortable, explore additional features and parameters available in PowerShell to enhance your user management capabilities.