To add a user to a group in Active Directory using PowerShell, you can utilize the `Add-ADGroupMember` cmdlet with the user's username and the target group's name specified.
Here's a code snippet to demonstrate this:
Add-ADGroupMember -Identity "GroupName" -Members "Username"
Replace `"GroupName"` with the actual name of the Active Directory group and `"Username"` with the user's username you want to add.
Understanding Active Directory Groups
What is Active Directory?
Active Directory (AD) is a directory service developed by Microsoft that manages permissions and access to network resources. It stores information about users, groups, computers, and other objects within a network, providing a centralized location for security and resource management. Within AD, groups serve as a mechanism to efficiently manage permissions and access rights for multiple users.
Types of AD Groups
Active Directory features several types of groups:
-
Security Groups: These groups are primarily used to manage permissions. They can be assigned rights to resources and simplify the process of granting access to large numbers of users.
-
Distribution Groups: Unlike security groups, distribution groups are used for email distribution lists and have no associated security permissions.
Understanding these distinctions can help you choose the appropriate group type when using PowerShell to manage user permissions.
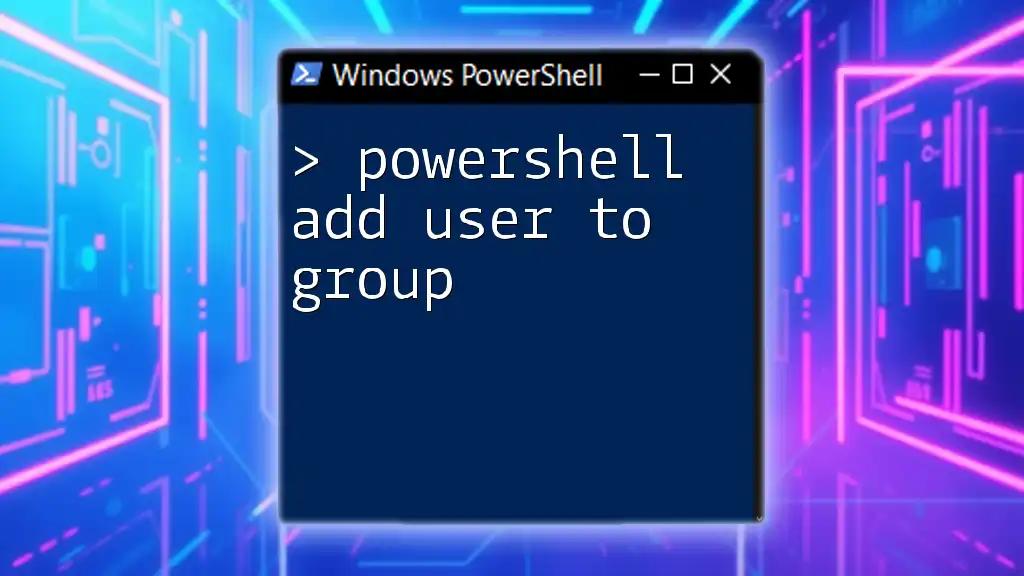
Why Use PowerShell for Adding Users to AD Groups?
PowerShell is an invaluable tool for system administrators managing Active Directory. Leveraging PowerShell to add users to AD groups offers several advantages:
-
Efficiency: With PowerShell scripts, you can automate the repetitive process of adding users, saving time and reducing workload.
-
Flexibility: PowerShell allows for easy scripting and the ability to schedule tasks, making it adaptable for various administrative needs.
-
Error Reduction: By using scripts, you minimize the chances of human error that can occur during manual entry, ensuring accuracy in your user management.

Prerequisites for Using PowerShell with Active Directory
Required Permissions
To add users to Active Directory groups, you must have the necessary permissions. Typically, you need to be a member of a group that has permission to manage group memberships, such as Domain Admins or Account Operators.
PowerShell Environment Setup
Before executing PowerShell commands for Active Directory, ensure that the Active Directory module is installed. This module is part of the Remote Server Administration Tools (RSAT) and is required to run commands related to AD.
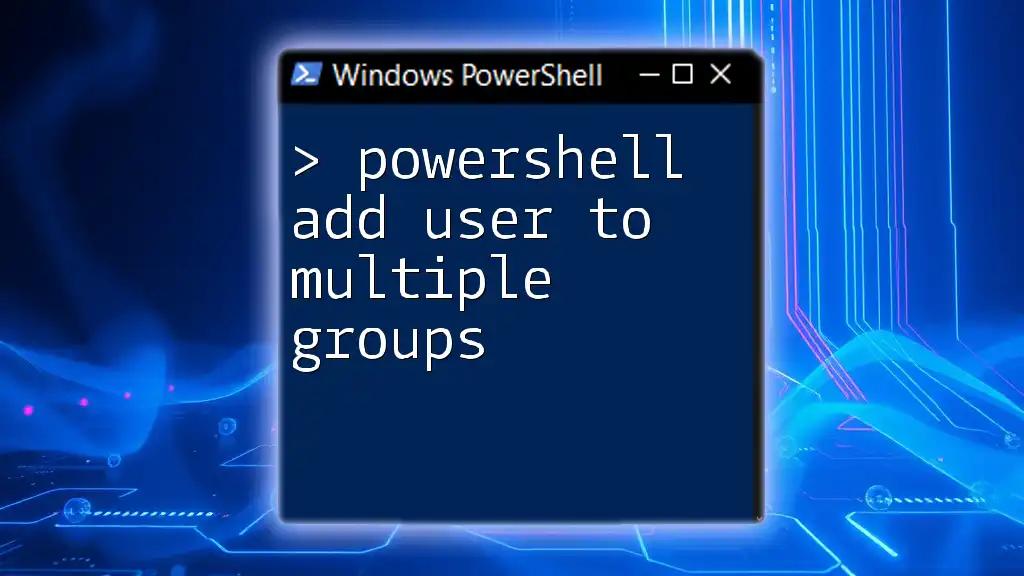
Basic PowerShell Commands for Managing AD Groups
Importing the Active Directory Module
To start working with AD commands, you need to import the Active Directory module:
Import-Module ActiveDirectory
This command loads the necessary cmdlets that will allow you to interact with Active Directory.
Checking Existing AD Groups
To see the groups available in your Active Directory, you can use the following command:
Get-ADGroup -Filter *
This command retrieves all AD groups, enabling you to verify existing group names before adding users.
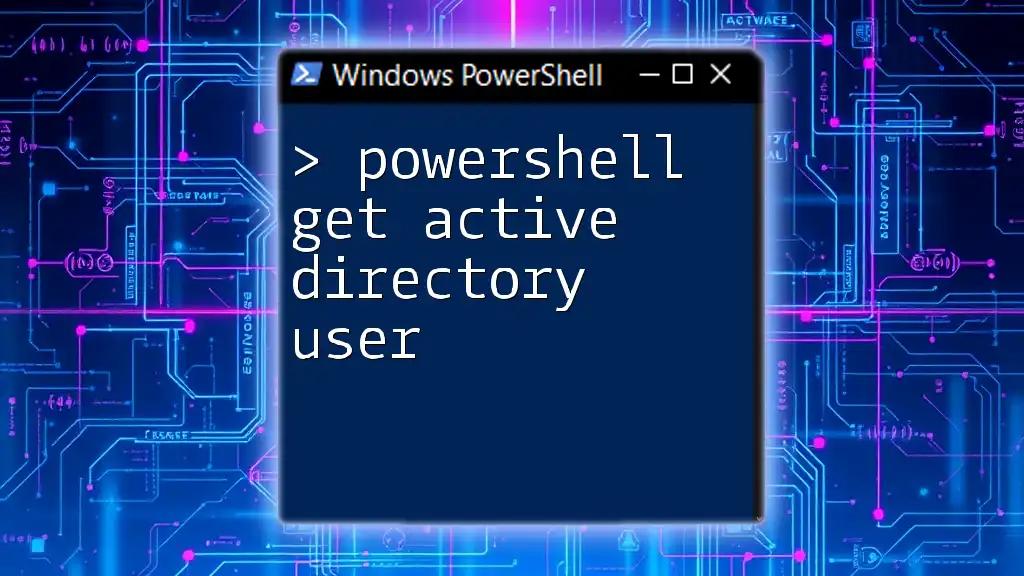
Adding Users to AD Groups Using PowerShell
Using the Add-ADGroupMember Command
The main command you will use to powershell add user to group active directory is `Add-ADGroupMember`. The syntax is simple:
Add-ADGroupMember -Identity "GroupName" -Members "UserName"
- -Identity: This parameter specifies the AD group to which you want to add users.
- -Members: This parameter identifies the user(s) you wish to add to the specified group.
Example: Adding a Single User
To add a user named "jdoe" to the "Sales" group, you would use:
Add-ADGroupMember -Identity "Sales" -Members "jdoe"
This command effectively adds "jdoe" as a member of the "Sales" group.
Example: Adding Multiple Users
Adding multiple users at once is also straightforward. For example, to add "jdoe" and "asmith" to the "Sales" group:
Add-ADGroupMember -Identity "Sales" -Members "jdoe", "asmith"
Using commas to separate user names allows you to add several users in a single command, streamlining your administrative tasks.
Using Variables for Group and User Name
Improving your PowerShell script's flexibility can be achieved by using variables. Here's how:
$groupName = "Sales"
$userName = "jdoe"
Add-ADGroupMember -Identity $groupName -Members $userName
By utilizing variables, you can easily change the group or user name without rewriting the entire command.
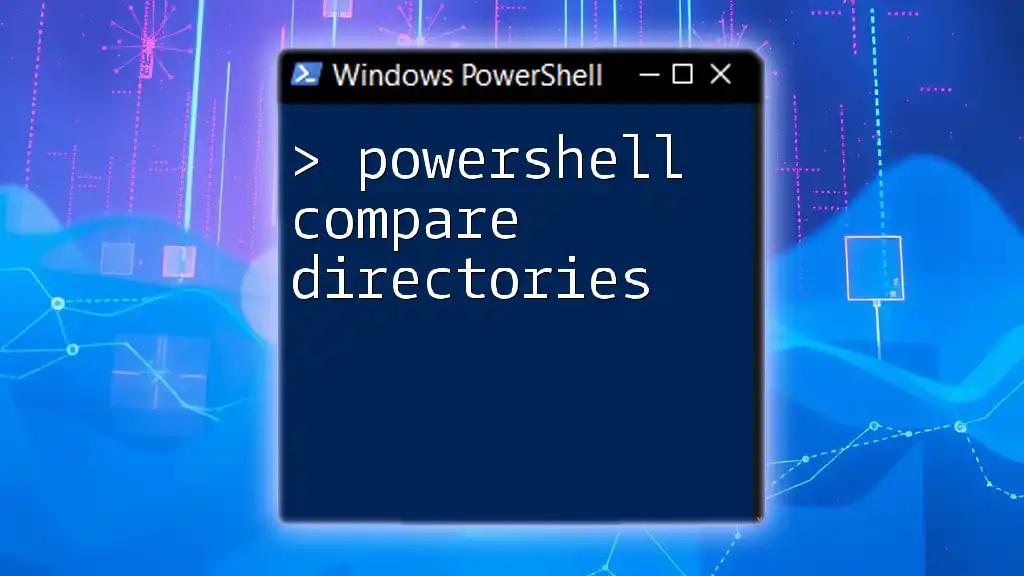
Adding Dynamic User Lists to AD Groups
Using CSV Files
For organizations that need to add many users, leveraging a CSV file is a powerful solution. Ensure that your CSV file is formatted correctly, including a header with user identifiers such as `UserName`.
Importing Users from CSV
To add users from a CSV file named "Users.csv" to the "Sales" group, you can run the following command:
Import-Csv "Users.csv" | ForEach-Object {
Add-ADGroupMember -Identity "Sales" -Members $_.UserName
}
This command imports user data from the CSV and adds each listed user to the specified group. The `ForEach-Object` cmdlet iterates through each user in the CSV, making bulk operations straightforward and efficient.

Troubleshooting Common Issues
Permission Errors
When you run into permission errors, verify that your user account has the appropriate privileges to modify group memberships. You can check user roles and permissions in Active Directory Users and Computers (ADUC) if necessary.
User Not Found Errors
It's common to encounter user not found errors when the username is incorrect or doesn't exist in Active Directory. Always double-check the spelling and confirm that the user is part of the domain before executing commands.
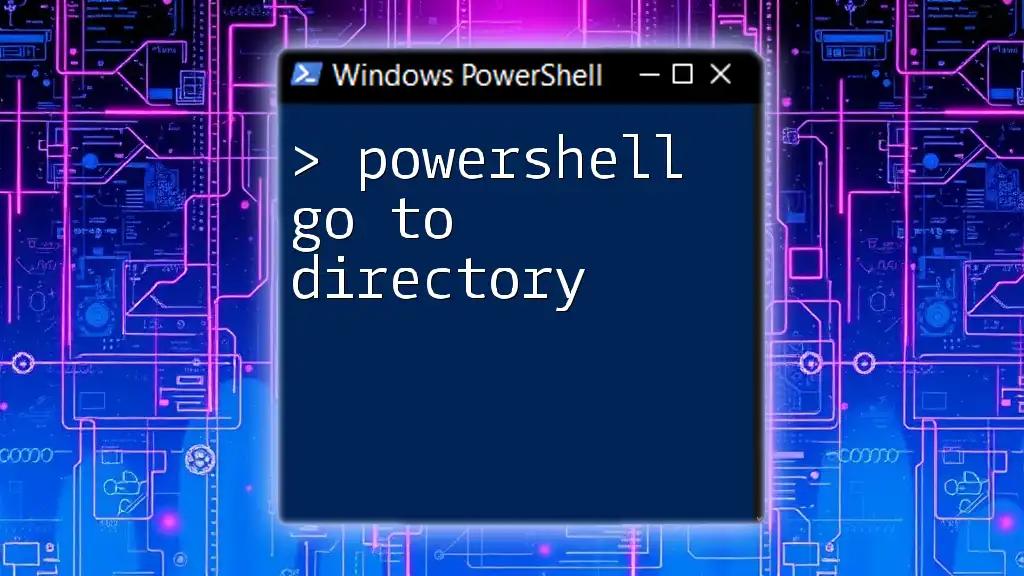
Best Practices for Using PowerShell with AD Groups
Utilizing PowerShell effectively requires following best practices:
-
Scripting: Conduct all your actions through scripts. This fosters reusability and helps maintain consistency across operations. Commenting your code can make it easier to understand for future reference or other administrators.
-
Testing: Always test your scripts in a safe environment before applying them in production. This helps prevent accidental changes or issues that could arise from unforeseen circumstances.
-
Documentation: Maintain clear documentation of scripts and processes. Good documentation can facilitate compliance and serve as a reference for troubleshooting and future modifications.
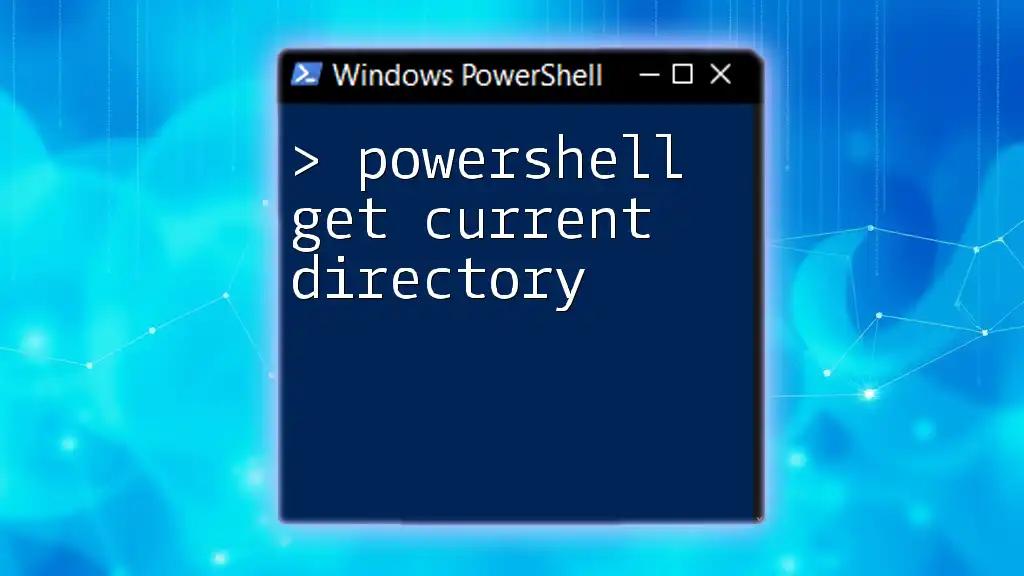
Conclusion
This guide has provided you with the essentials to add users to Active Directory groups using PowerShell. By practicing these commands, utilizing scripts, and adhering to best practices, you'll enhance your skills in managing AD effectively. Don’t hesitate to explore more advanced PowerShell functionalities as your knowledge grows! Implement the methods outlined here in a safe testing environment to build your confidence and proficiency with Active Directory management.
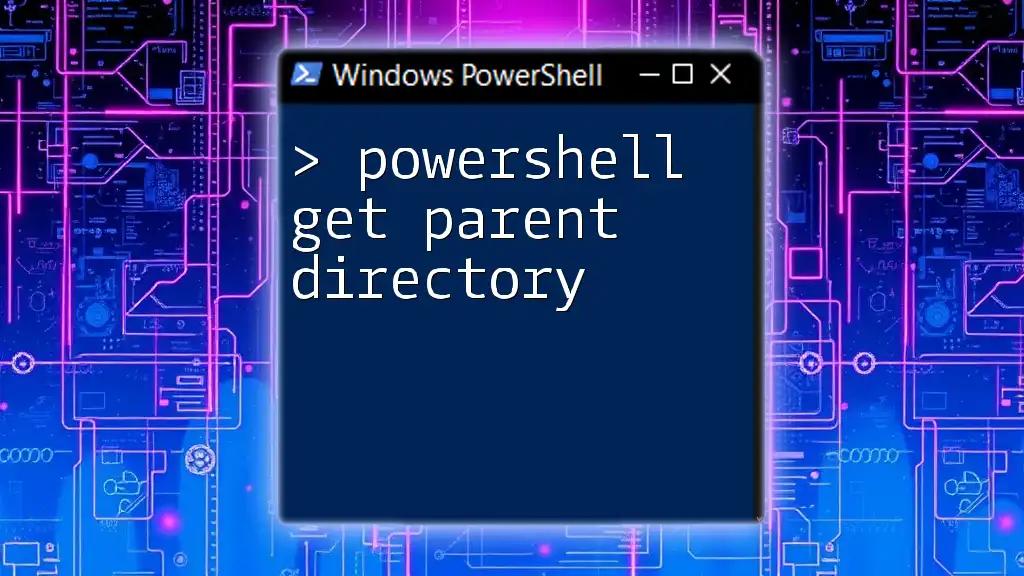
Additional Resources
For further information, check out Microsoft’s official documentation on PowerShell and Active Directory. Additionally, consider investing time in thorough learning resources like books or online courses to expand your PowerShell skill set.