To retrieve all files in a directory using PowerShell, you can utilize the `Get-ChildItem` cmdlet, which lists the contents of a specified directory.
Get-ChildItem -Path "C:\Your\Directory\Path" -File
Understanding PowerShell Commands
What is PowerShell?
PowerShell is a powerful task automation and configuration management tool designed specifically for system administrators. It combines a command-line shell with an associated scripting language, allowing for automation of diverse system management tasks through scripts written in its own syntax.
At the core of PowerShell are cmdlets (pronounced "command-lets"), which are specialized .NET classes that perform specific functions. The cmdlets are designed to be both simple to use and flexible enough to handle complex tasks, making them an excellent choice for managing Windows environments.
Importance of Directory Management
Effective directory management is crucial in any computing environment. Whether you’re organizing reports, scripts, or any other files, knowing how to quickly retrieve file information can boost your productivity. For instance:
- Troubleshooting: When you need to locate files swiftly due to a software issue.
- Data Management: Keeping track of backups and ensuring files are in their proper locations.
- Reporting: Quickly generating lists of files for audit trails or project management.
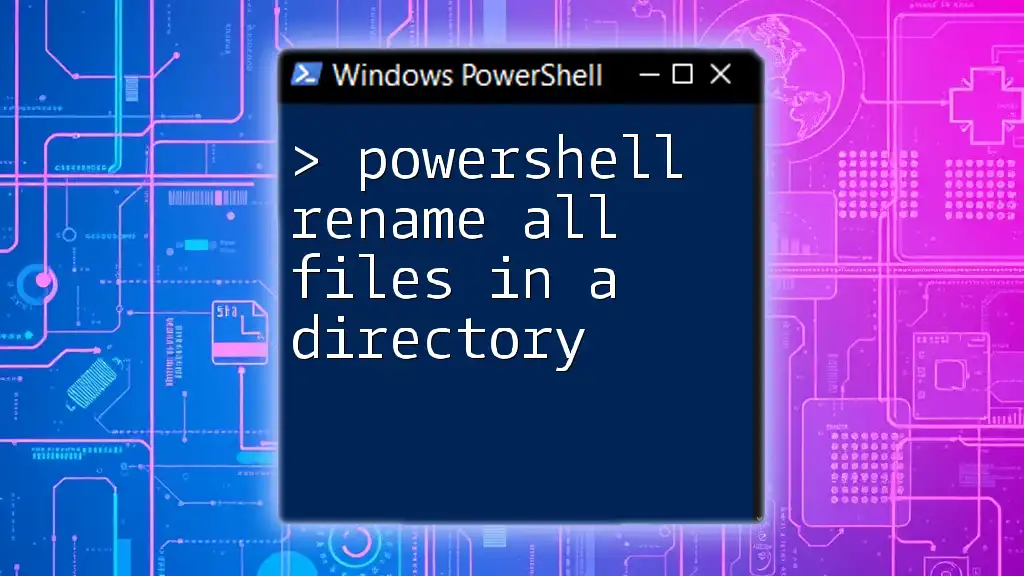
Getting Started with the Basic Command
The `Get-ChildItem` Cmdlet
One of the primary cmdlets for interacting with the filesystem in PowerShell is `Get-ChildItem`. This command is essential for retrieving items from a specified location. The syntax is straightforward, making it user-friendly for beginners.
An example of a basic command to retrieve all files from a specific directory would be:
Get-ChildItem -Path "C:\YourDirectoryPath"
This command lists all the files and directories within "C:\YourDirectoryPath", giving users a complete overview of what's stored there.
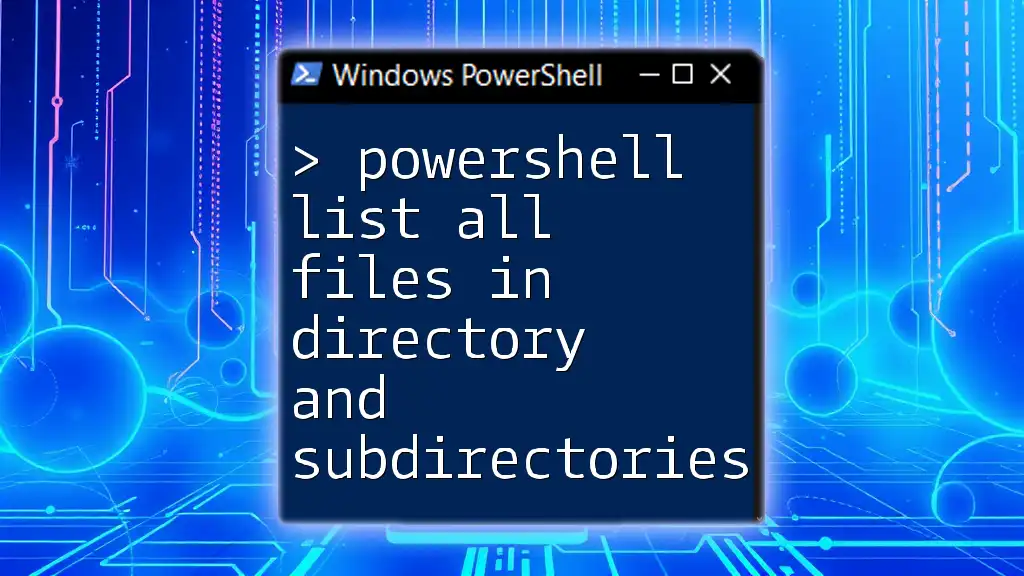
Filtering Files in a Directory
Retrieving Specific File Types
Sometimes, you may only need to retrieve files of a particular type, such as `.txt` or `.jpg`. The `-Filter` parameter comes in handy for such requirements.
For example, if you want to gather only text files, you'd use:
Get-ChildItem -Path "C:\YourDirectoryPath" -Filter "*.txt"
This command focuses only on files that have the `.txt` extension, allowing you to manage specific file types efficiently.
Using Wildcards
Wildcards offer additional flexibility in file retrieval. The asterisk (`*`) can be used to represent any number of characters.
For instance, to retrieve all files that start with "Report", you would execute the command:
Get-ChildItem -Path "C:\YourDirectoryPath\Report*"
This command will return any file that begins with "Report", promoting a more granular approach to file management.
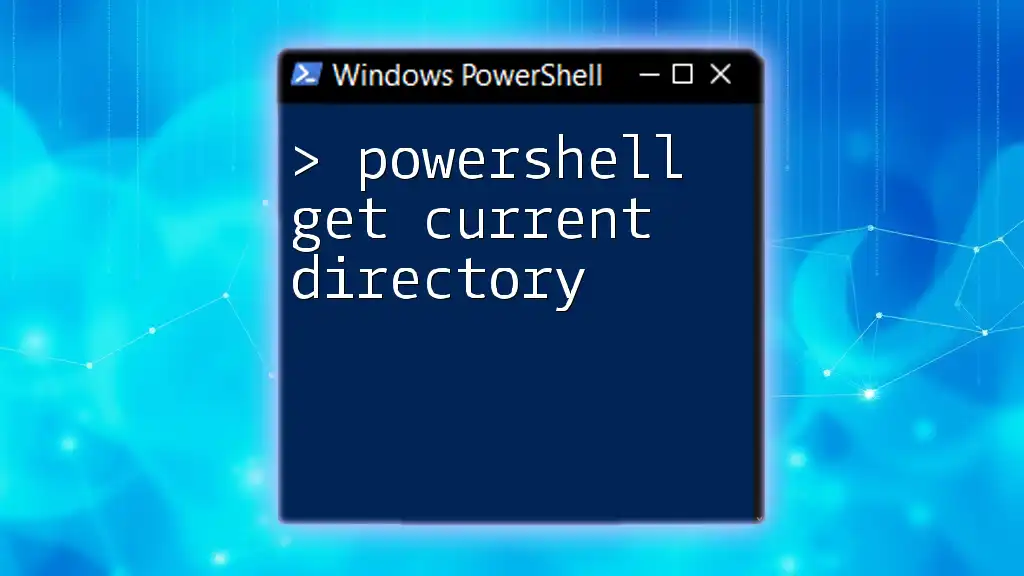
In-depth Exploration of Parameters
Common Parameters with `Get-ChildItem`
PowerShell offers several parameters with `Get-ChildItem` that enhance its functionality. Key parameters include `-Recurse`, `-File`, and `-Directory`, which modify the way files are retrieved.
Using `-Recurse`
The `-Recurse` parameter expands the search to include all subdirectories and their contents. This is particularly useful when you're unsure of where certain files are located within a directory tree.
To fetch files from the specified directory and all its subdirectories, you can use:
Get-ChildItem -Path "C:\YourDirectoryPath" -Recurse
This command collects all items, regardless of their depth within the directory hierarchy.
Fetching Only Files or Directories
You might also want to filter your results further to show either files or directories exclusively. You can use the `-File` and `-Directory` parameters accordingly.
To get only files within a directory, use:
Get-ChildItem -Path "C:\YourDirectoryPath" -File
Conversely, to list only directories, the command would be:
Get-ChildItem -Path "C:\YourDirectoryPath" -Directory
These commands are essential for managing your workspace and ensuring you are working with the correct item types.
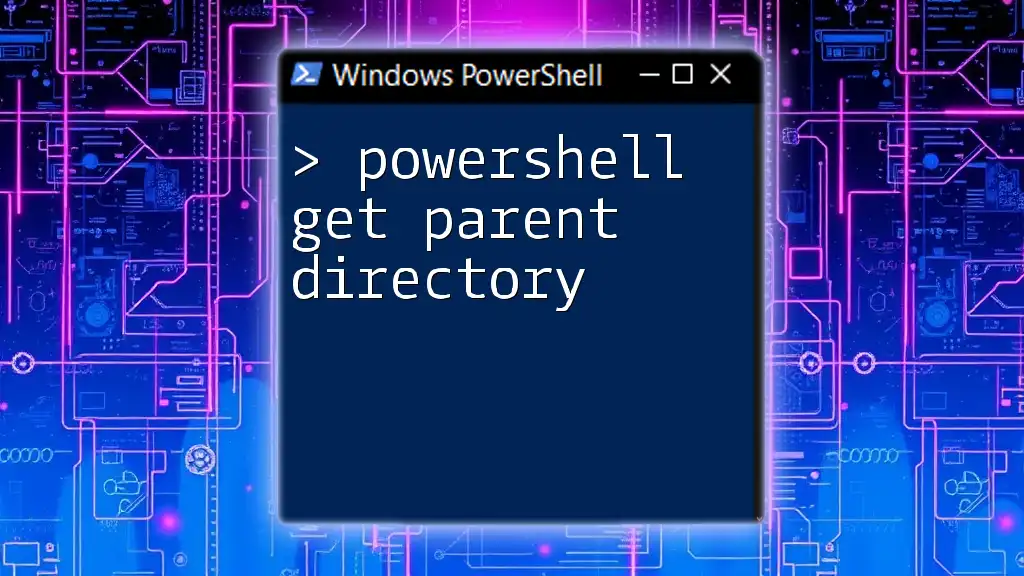
Advanced File Listing Techniques
Sort and Select
As you collect your files, you may want to sort them based on specific criteria, such as modification date or file size. You can sort the files retrieved and limit the displayed results using the `Sort-Object` and `Select-Object` cmdlets.
For example, to sort files by their last modified date and select just the top five most recent files, you would run:
Get-ChildItem -Path "C:\YourDirectoryPath" | Sort-Object LastWriteTime | Select-Object -First 5
This command provides a quick glimpse at the most recently altered files in the directory.
Exporting the List of Files
Once you've gathered your files, you might want to document them for future reference. PowerShell allows you to export the list of files to formats like CSV or plain text.
For instance, to export a list of files to a CSV file, you would use:
Get-ChildItem -Path "C:\YourDirectoryPath" | Export-Csv -Path "C:\FileList.csv" -NoTypeInformation
This command creates a CSV file with the details of the files within the specified directory, making them easy to share or analyze.
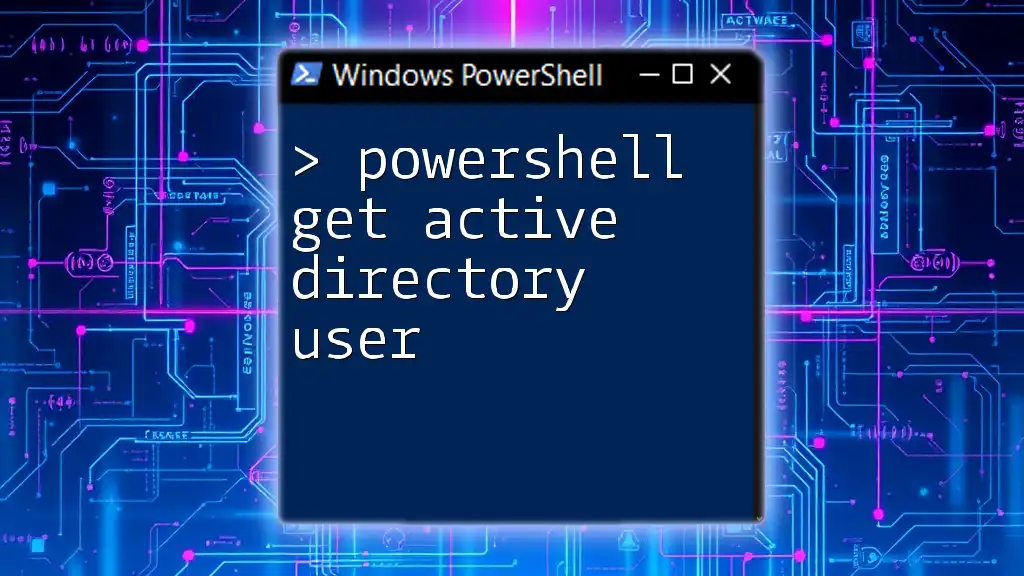
Troubleshooting Common Issues
Permission Denied Errors
One common issue when using PowerShell is receiving permission errors when attempting to access certain directories. This typically happens if the PowerShell session does not have adequate permissions.
To resolve this, consider running PowerShell as an administrator. Right-click the PowerShell icon and select "Run as administrator". This grants elevated permissions for accessing secured directories.
No Files Found
If your command returns no files, it may be due to an incorrect path or file extension. Always double-check your directory path for typos and ensure that the file type you are looking for exists in that directory.
Using the `-Recurse` option can also help in locating files that are nested within subdirectories.
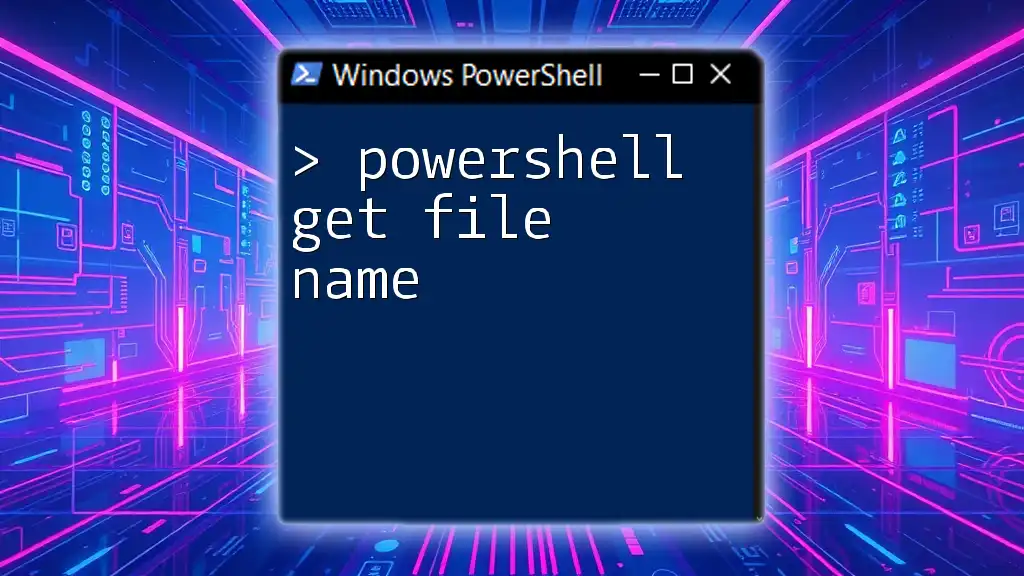
Conclusion
By mastering the `Get-ChildItem` cmdlet, you can effectively manage files within your directories using PowerShell. This powerful tool simplifies the process of retrieving, sorting, and exporting file information, enhancing your overall productivity.
Feel empowered to experiment with these commands and explore additional PowerShell functionalities to streamline your workflow further. Embrace the capabilities of PowerShell to become more effective in your file management tasks.
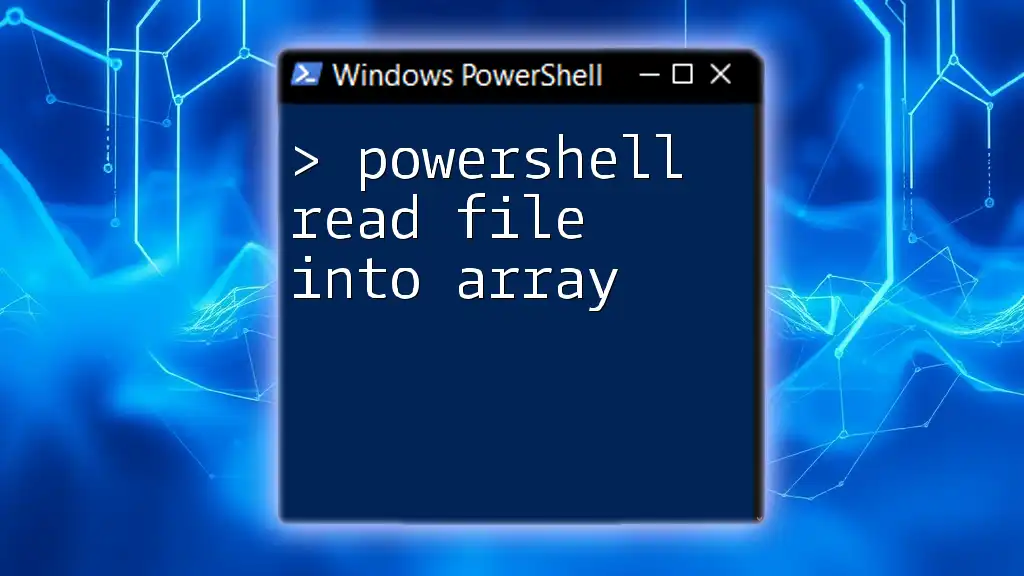
Additional Resources
For further learning, consider exploring PowerShell’s official documentation and other tutorials available online. These resources can provide you with additional insights and advanced techniques for mastering filesystem management using PowerShell.