To rename all files in a directory using PowerShell, you can use the following command which employs a loop to rename each file based on your specified format.
Get-ChildItem "C:\YourDirectoryPath" | Rename-Item -NewName { "NewPrefix_" + $_.Name }
Replace `C:\YourDirectoryPath` with the path of your directory and `NewPrefix_` with your desired prefix for the renamed files.
Understanding File Renaming in PowerShell
File renaming is a fundamental task in both individual and business workflows, often necessary for organization, clarity, and efficiency. Whether you're sorting photos, updating batch reports, or changing file extensions, renaming files in bulk helps you maintain a well-organized digital workspace.
Bulk renaming saves time and minimizes errors typical with manual renaming, making it an essential skill for anyone working with multiple files.
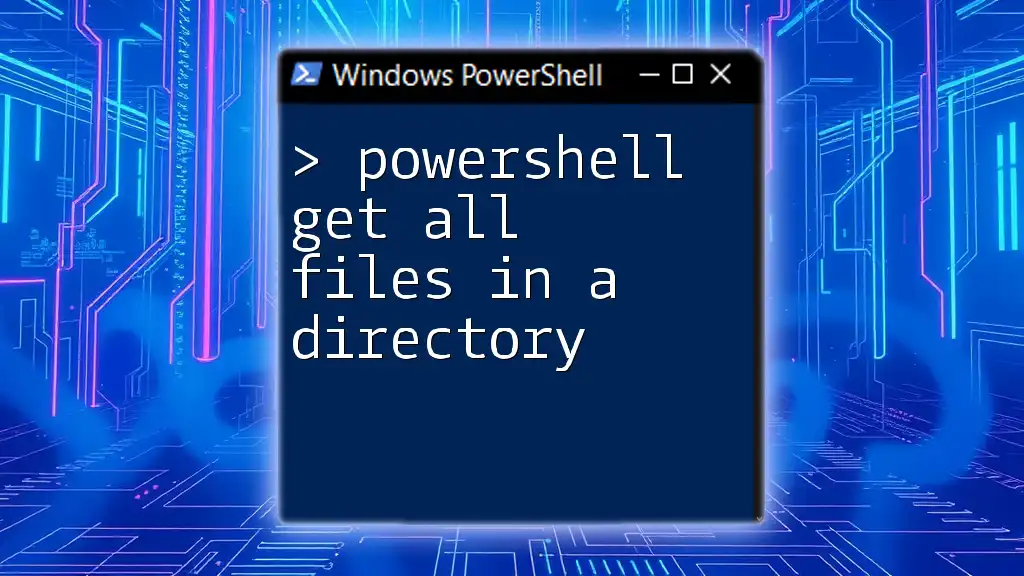
Prerequisites for Using PowerShell
To successfully use PowerShell, you should have a basic understanding of how PowerShell commands operate. Familiarity with file systems and the command line can significantly ease your experience.
Accessing PowerShell
You can launch PowerShell through various methods depending on your Windows version. For most users, right-clicking the Start button and selecting "Windows PowerShell" or "Windows Terminal" is the fastest option.
Permissions Consideration
Ensure you have the necessary permissions to modify files within your chosen directory. Lack of appropriate permissions can lead to errors and hinder your renaming process.
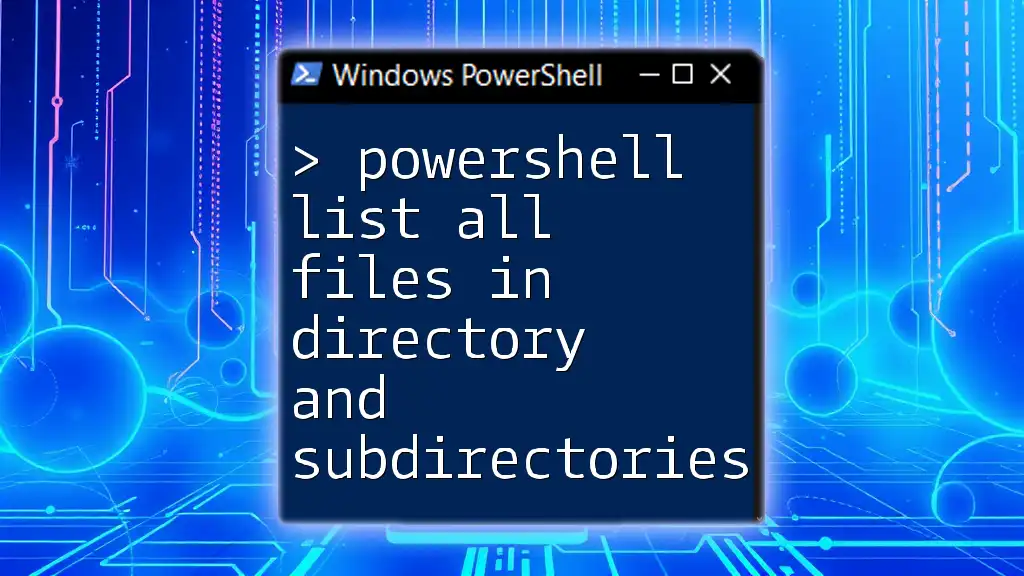
Setting Up Your PowerShell Environment
Choosing the Right Directory
Start by navigating to the directory containing the files you intend to rename. Use the following command to set your location:
Set-Location -Path "C:\Your\Target\Directory"
This command points PowerShell to the specified directory, enabling you to perform operations on its contents.
Confirming Files to Rename
Before committing to a renaming task, it's important to see what files currently exist in the directory. You can list the files with:
Get-ChildItem
This command displays all the files, allowing you to verify the contents and ensure you’re targeting the right files for renaming.
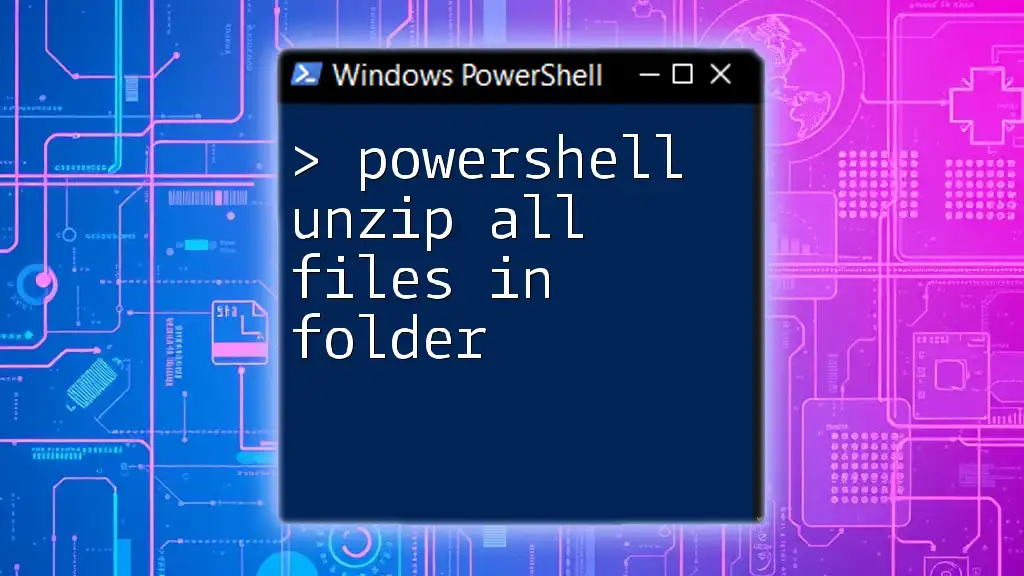
Techniques for Renaming Files in PowerShell
Renaming a Single File
Sometimes you may need to rename a single file. PowerShell makes this task straightforward. Here’s how you can rename a file:
Rename-Item -Path "oldFilename.txt" -NewName "newFilename.txt"
This command effectively renames `oldFilename.txt` to `newFilename.txt`.
Renaming Multiple Files: Basic Approach
For bulk operations, you can utilize a loop. A common scenario is to rename all files by adding a prefix to their filenames. Here’s a simple example:
Get-ChildItem -File | ForEach-Object { Rename-Item $_.FullName -NewName ("newPrefix_" + $_.Name) }
In this command, the `Get-ChildItem -File` retrieves all files in the directory. The `ForEach-Object` iterates over each file, and `Rename-Item` renames it by appending "newPrefix_" to the original file name. This is an efficient way to bulk rename files with minimal effort.
Advanced Renaming Techniques
Renaming Based on File Properties
You can take it a step further by renaming files based on their file properties, such as their creation date. Consider the following example to rename files by prefixing them with the creation date:
Get-ChildItem -File | ForEach-Object { Rename-Item $_.FullName -NewName ($_.CreationTime.ToString("yyyyMMdd") + "_" + $_.Name) }
In this snippet, `CreationTime.ToString("yyyyMMdd")` formats the file’s creation date in a Year-Month-Day format and prepends it to the original file name, which is useful for archiving purposes.
Using Regular Expressions for Complex Renaming Patterns
Regular expressions offer powerful pattern matching for more complex renaming tasks. Let’s say you want to change the file extension of all `.txt` files to `.docx`. You can achieve this with:
Get-ChildItem -File | Where-Object { $_.Name -match "\.txt$" } | ForEach-Object { Rename-Item $_.FullName -NewName ($_.Name -replace "\.txt$", ".docx") }
Here, `Where-Object` filters files that end with `.txt`, and `-replace` updates the extension. This example highlights the flexibility of PowerShell by allowing you to manipulate filenames based on specific criteria, facilitating intricate bulk operations.
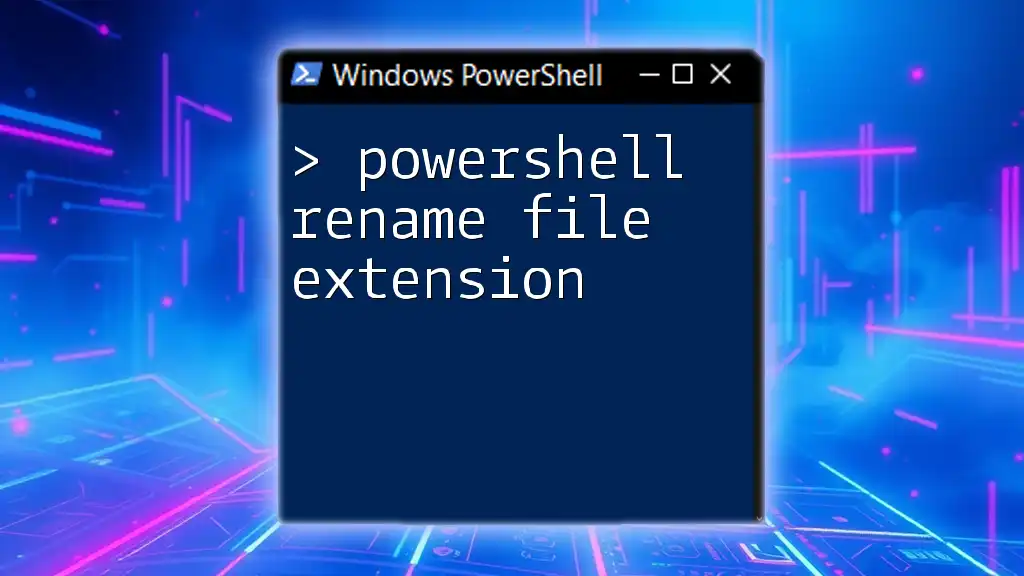
Best Practices for Renaming Files
Backup Files Before Renaming
Before performing any bulk renaming operation, always backup your files. Creating a duplicate of your directory or using file versioning can prevent data loss and provide a fallback if your changes don’t yield the expected results.
Testing Commands Before Execution
To decrease the risk of errors and ensure that the commands perform correctly, make use of the `-WhatIf` parameter. This parameter lets you preview the changes without executing them. Here’s how it's done:
Get-ChildItem -File | ForEach-Object { Rename-Item $_.FullName -NewName ("newName_" + $_.Name) -WhatIf }
This command will display what would happen if you ran it, allowing you to confirm that only the intended files would be affected.
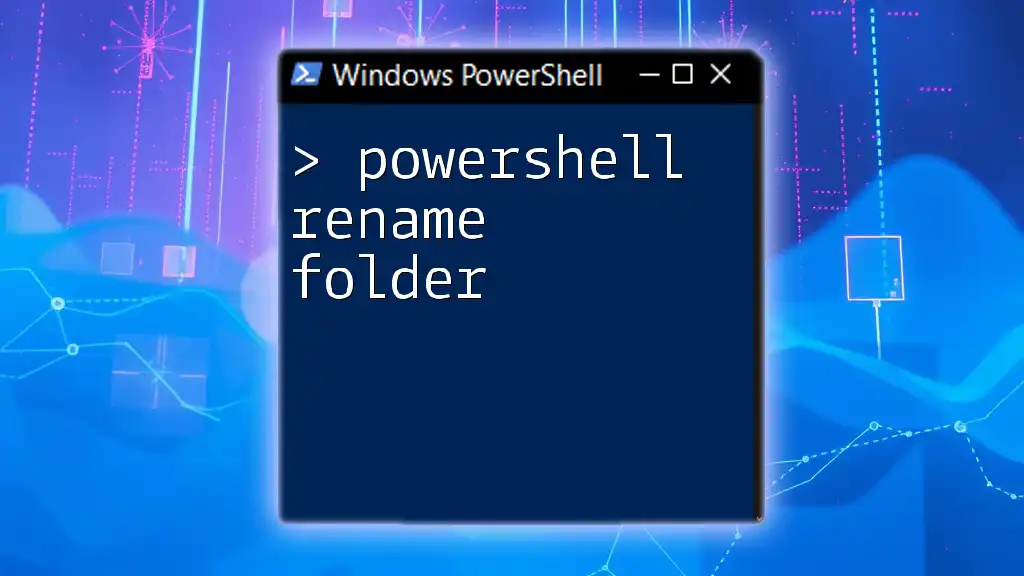
Common Errors and Troubleshooting
File In Use or Locked Errors
Errors involving files being in use will typically arise when you attempt to rename a file currently open in another application. To resolve this, ensure that all relevant applications are closed before executing your renaming commands.
Access Denied Errors
If you encounter permission-related errors, ensure that your account has the necessary access rights to modify the files within the directory. You may need to run PowerShell as an administrator or adjust the file permissions accordingly.
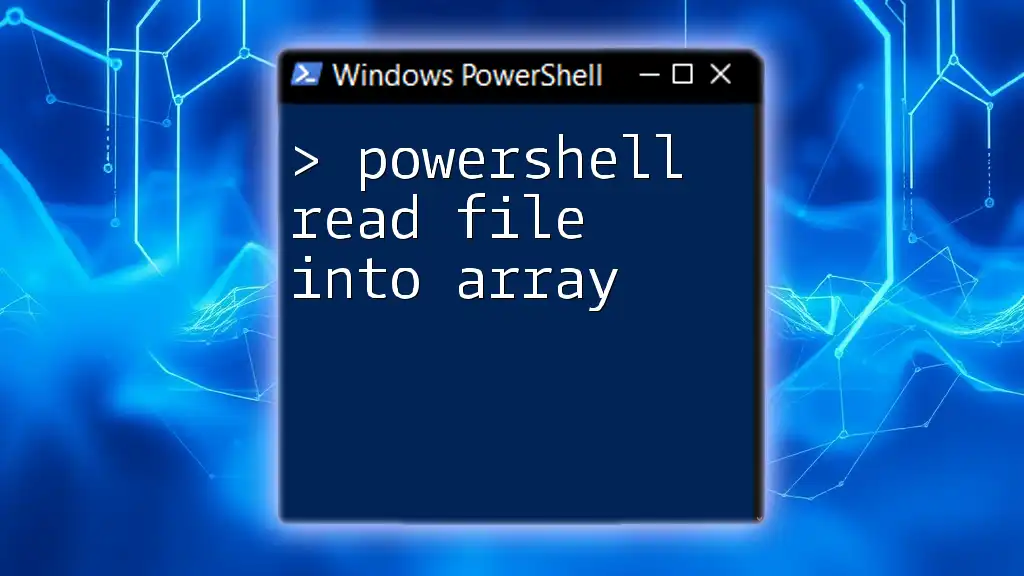
Conclusion
By mastering the PowerShell rename all files in a directory command, you gain powerful capabilities to streamline your file management tasks. From simple renaming to complex pattern-based transformations, PowerShell offers a flexible and efficient solution for bulk operations.
As you practice, don't hesitate to explore advanced functionalities, and remember to backup your files to prevent loss during renaming. Engage with the PowerShell community or online tutorials for ongoing learning and improved efficiency in your file management tasks.