The `foreach` loop in PowerShell allows you to iterate through each file in a specified folder, enabling you to perform actions on those files efficiently.
Get-ChildItem "C:\Path\To\Your\Folder" | ForEach-Object { Write-Host $_.Name }
Understanding Foreach in PowerShell
What is the Foreach Statement?
The `foreach` statement in PowerShell is a powerful construct used to iterate over collections. This means that you can run a series of commands for each item in a set, such as files in a directory. The basic syntax of `foreach` allows for clear and straightforward coding, making it a favored choice among script developers.
Differences Between Foreach and ForEach-Object
While both `foreach` and `ForEach-Object` serve the purpose of iteration, they differ in their implementation and use cases. The `foreach` statement is an explicit looping structure that operates in-memory, and it evaluates its entire collection before executing the loops. In contrast, `ForEach-Object` operates in a pipeline, processing each item as it comes through. Typically, `ForEach-Object` is better suited for large datasets when combined with cmdlets, as it can begin processing before loading the entire collection into memory.
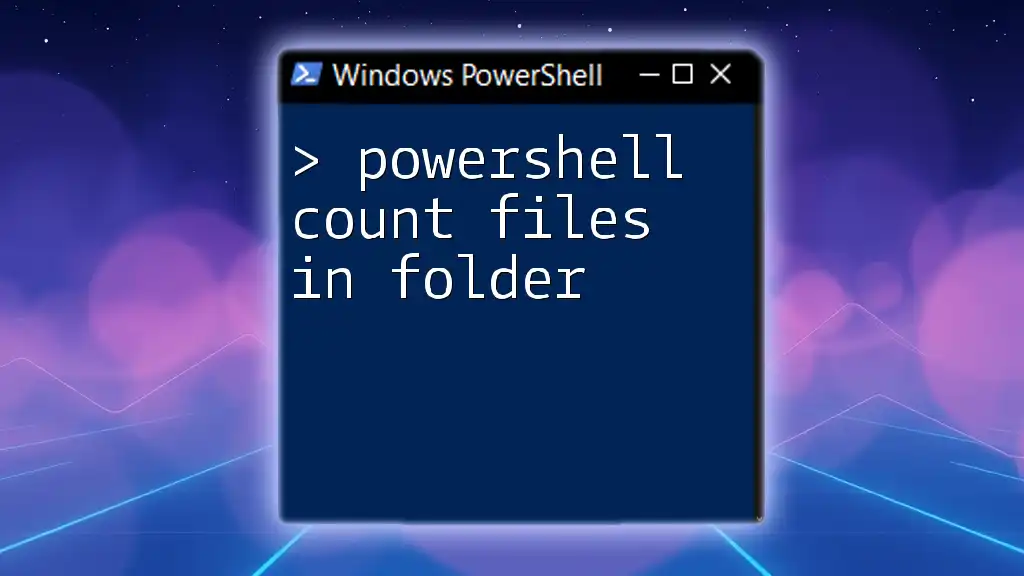
Setting Up Your Environment for File Manipulation
Installing PowerShell
Before diving into file manipulation, ensure that you have PowerShell installed. Most Windows operating systems come equipped with PowerShell pre-installed, but if you are using Linux or Mac, you can install PowerShell through the official package manager. Simply follow the guidelines in the PowerShell documentation for your respective operating system.
Basic Concepts to Familiarize Yourself With
Understanding file paths is crucial for effectively using PowerShell. There are two primary types of paths:
- Absolute Path: A complete path from the root of the file system to the target file or folder (e.g., `C:\Users\Name\Documents\File.txt`).
- Relative Path: A path relative to the current working directory (e.g., `.\Documents\File.txt`).
You can access the command line interface in PowerShell by simply searching for "PowerShell" in your Windows Start Menu or using the Terminal on Linux/Mac.
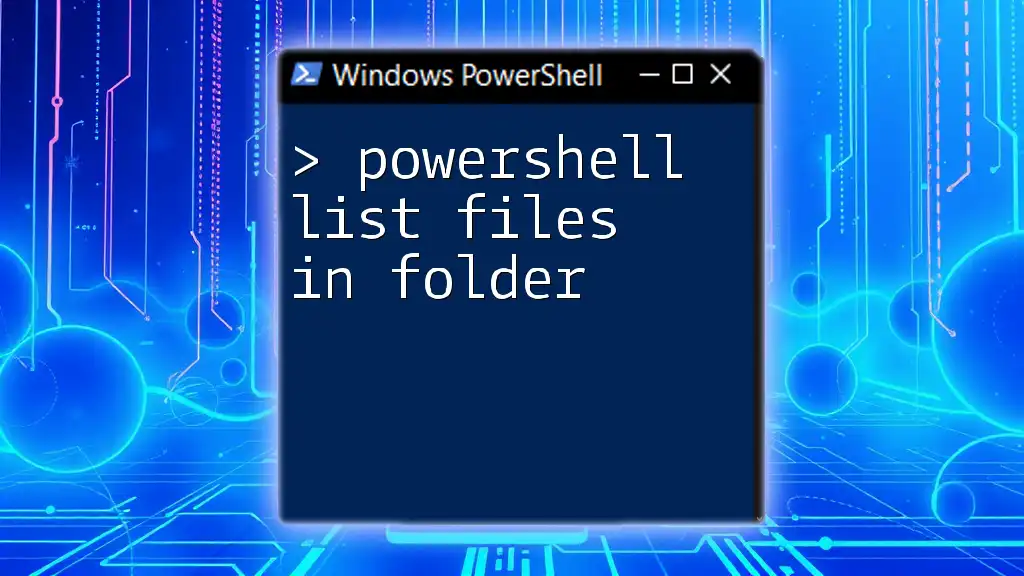
Using PowerShell to Loop Through Files
Syntax for Looping Through Files in a Directory
The basic structure of the `foreach` statement is quite simple. You will typically start by obtaining a list of files using the `Get-ChildItem` cmdlet followed by the `foreach` loop:
$files = Get-ChildItem -Path "C:\Your\Folder\Path"
foreach ($file in $files) {
Write-Output $file.Name
}
In this example, `Get-ChildItem` retrieves all items in the specified directory, and the `foreach` statement processes each file (obj) in `$files`, outputting its name.
Iterating Over Specific Types of Files
To filter files by extension while looping, you can use the `-Filter` parameter of the `Get-ChildItem` cmdlet. This helps you focus on specific file types. For instance, if you want to loop through only `.txt` files in a folder:
$files = Get-ChildItem -Path "C:\Your\Folder\Path" -Filter "*.txt"
foreach ($file in $files) {
Write-Output "Processing file: $($file.Name)"
}
Using Full Pathnames in the Loop
Accessing the full pathname of each file can be useful for various tasks like logging or for further manipulation. To display full paths of files, you can modify your loop as follows:
foreach ($file in $files) {
Write-Output $file.FullName
}
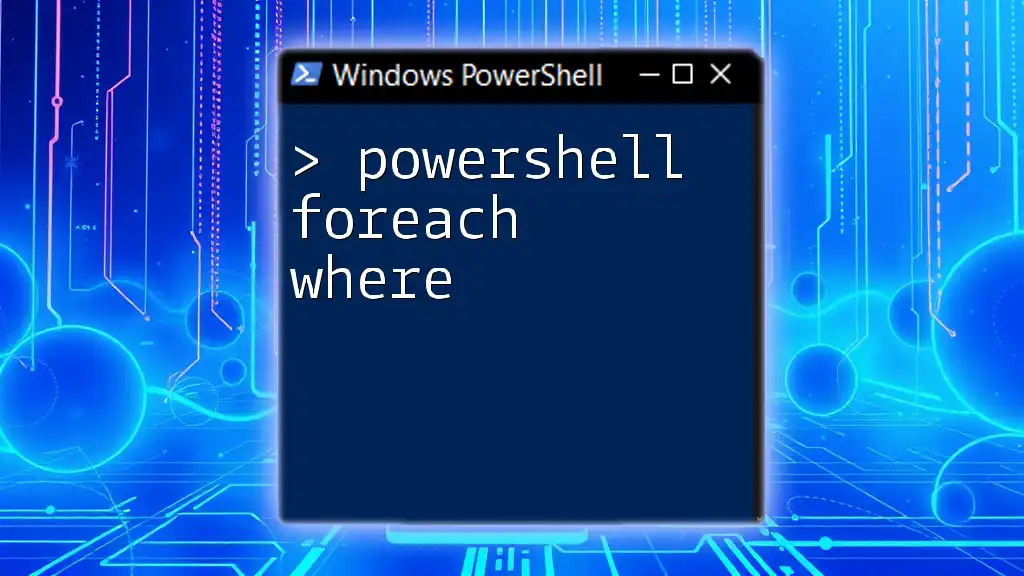
Advanced Iteration Techniques
Working with Nested Directories
Sometimes, you may want to iterate through not just the files in a directory but also the files in its subdirectories. For this purpose, the `-Recurse` parameter of `Get-ChildItem` is invaluable. Here's an example:
$files = Get-ChildItem -Path "C:\Your\Folder\Path" -Recurse
foreach ($file in $files) {
Write-Output "Found file: $($file.FullName)"
}
This command goes through every subfolder and retrieves their contents as well, allowing for comprehensive file handling.
Leveraging Pipeline with ForEach-Object
Inline processing can be considerably faster and more memory-efficient using `ForEach-Object`, especially with pipeline commands. Combine it with `Get-ChildItem` for a powerful one-liner:
Get-ChildItem -Path "C:\Your\Folder\Path" | ForEach-Object {
Write-Output "Processing: $($_.Name)"
}
With this approach, each file object is processed as it is read, reducing memory overhead.
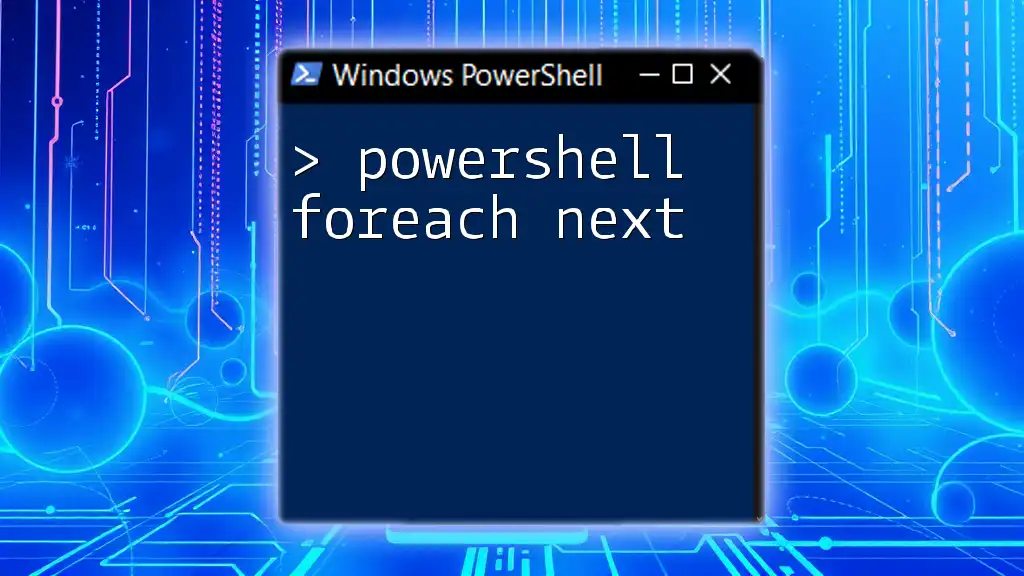
Practical Examples of Looping Through Files
Example: Renaming Files in a Directory
Bulk renaming files can be achieved seamlessly using a `foreach` loop. The following code snippet demonstrates how to add a prefix “New_” to each file in the specified directory:
$files = Get-ChildItem -Path "C:\Your\Folder\Path"
foreach ($file in $files) {
$newName = "New_" + $file.Name
Rename-Item -Path $file.FullName -NewName $newName
}
This command retrieves all files, generates a new name by concatenating "New_" with the original file name, and renames each file accordingly.
Example: Copying Files to Another Directory
You can also use the `foreach` loop to copy files to another directory. Here is a straightforward approach to accomplish this task:
$sourceFiles = Get-ChildItem -Path "C:\Your\Folder\Path"
$destination = "C:\Your\Destination\Path"
foreach ($file in $sourceFiles) {
Copy-Item -Path $file.FullName -Destination $destination
}
This code retrieves files from the source directory and copies them to the destination directory specified.
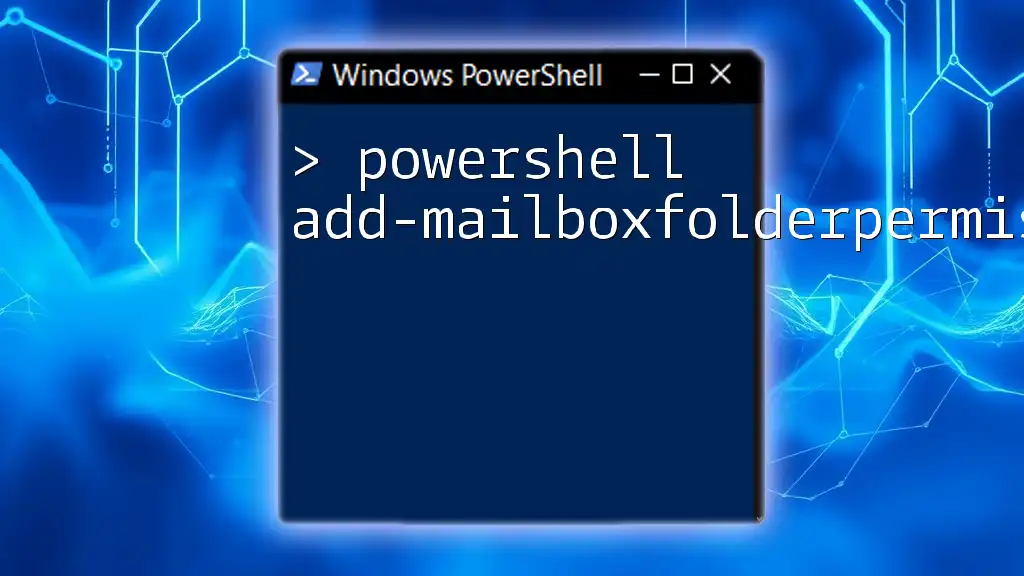
Common Errors and Troubleshooting
Handling Errors in PowerShell Scripts
In any programming environment, errors are inevitable. To handle errors gracefully, you can employ `try` and `catch` blocks. An example of this in a file processing context is:
foreach ($file in $files) {
try {
# Processing logic
} catch {
Write-Output "Error processing $($file.Name): $_"
}
}
This way, if an error occurs during processing, the script continues and logs the issue rather than terminating abruptly.
Permissions and Access Issues
When dealing with file operations, you may encounter permission-related errors, especially if trying to modify or access files you don’t have access to. It is important to run PowerShell with administrative privileges when required and adjust or check file permissions as necessary.
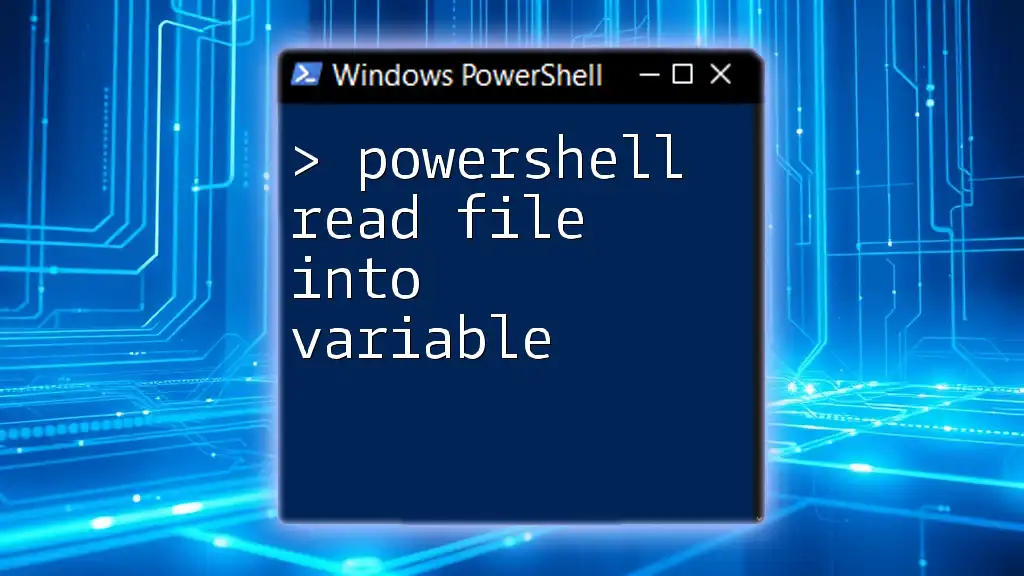
Conclusion
Leveraging `PowerShell foreach file in folder` commands can dramatically enhance your productivity by automating file management tasks effectively. By mastering these techniques, you can harness the full power of PowerShell for file manipulation and various other automation tasks.
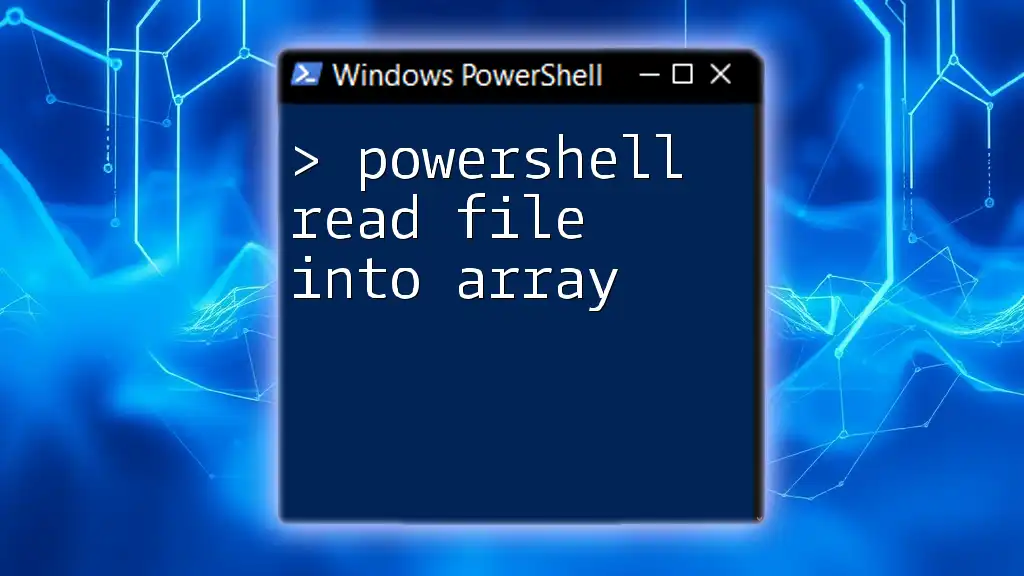
Additional Resources
To further your expertise in PowerShell, consider exploring the official PowerShell documentation, which offers rich insights and examples. Additionally, delve into recommended books and online courses that cover advanced PowerShell scripting. Engaging with community forums can also provide support, allowing you to explore shared experiences and solutions from fellow PowerShell users.
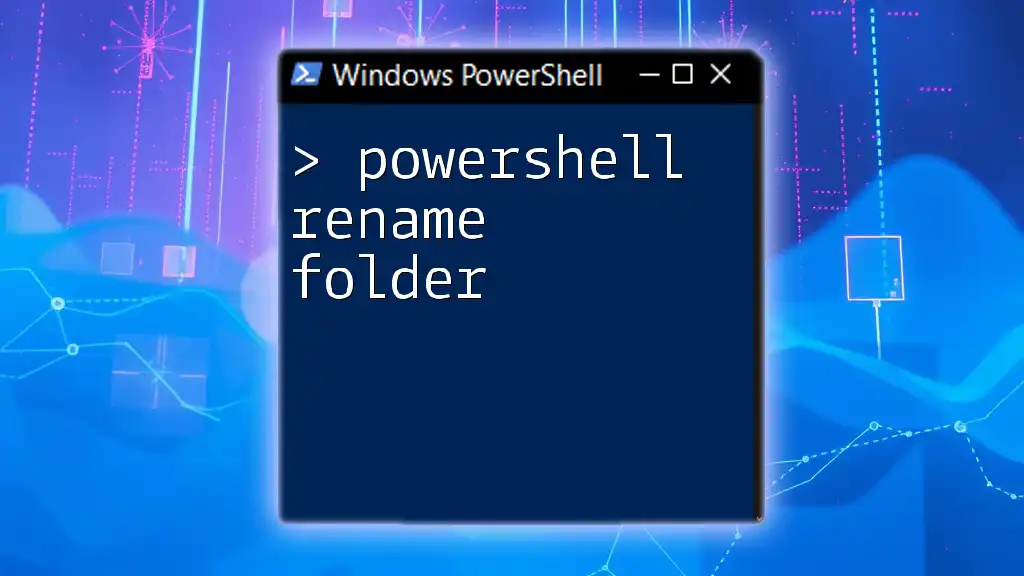
Call to Action
Join our PowerShell training sessions to gain hands-on experience and become proficient in using PowerShell for file manipulation and beyond. Subscribe for updates and tips to keep your PowerShell skills sharp and relevant!