PowerShell can read INI files by utilizing the `Get-Content` command combined with parsing logic to extract key-value pairs.
Here's a simple code snippet that demonstrates how to read an INI file in PowerShell:
$iniPath = "C:\path\to\your\file.ini"
$iniContent = Get-Content $iniPath | Where-Object { $_ -and $_ -notmatch '^\s*;' }
$iniHashTable = @{}
foreach ($line in $iniContent) {
$key, $value = $line -split '=', 2
$iniHashTable[$key.Trim()] = $value.Trim()
}
$iniHashTable
Understanding INI Files
Definition of INI Files
INI files, or initialization files, are simple text files used for configuration settings. They are particularly popular among applications in Windows environments due to their lightweight structure. An INI file generally consists of sections indicated by headers in square brackets, with keys and values defined as key=value pairs.
A typical INI file structure looks like this:
[Database]
User=root
Password=1234
[Server]
Host=localhost
Port=8080
Common Use Cases of INI Files
INI files serve various purposes in computer systems:
- Configuration settings for applications, allowing users to change behavior without modifying code.
- Storing user preferences, which can help create a more personalized experience.
- Simple data storage that doesn't require complex databases or data structures.

The Challenge of Reading INI Files in PowerShell
While INI files are relatively straightforward, parsing them can pose challenges, particularly if one is unfamiliar with their format. The main difficulty lies in that PowerShell does not natively support INI files; hence, you may need to resort to using string manipulation techniques, custom functions, or even .NET classes to read them effectively.
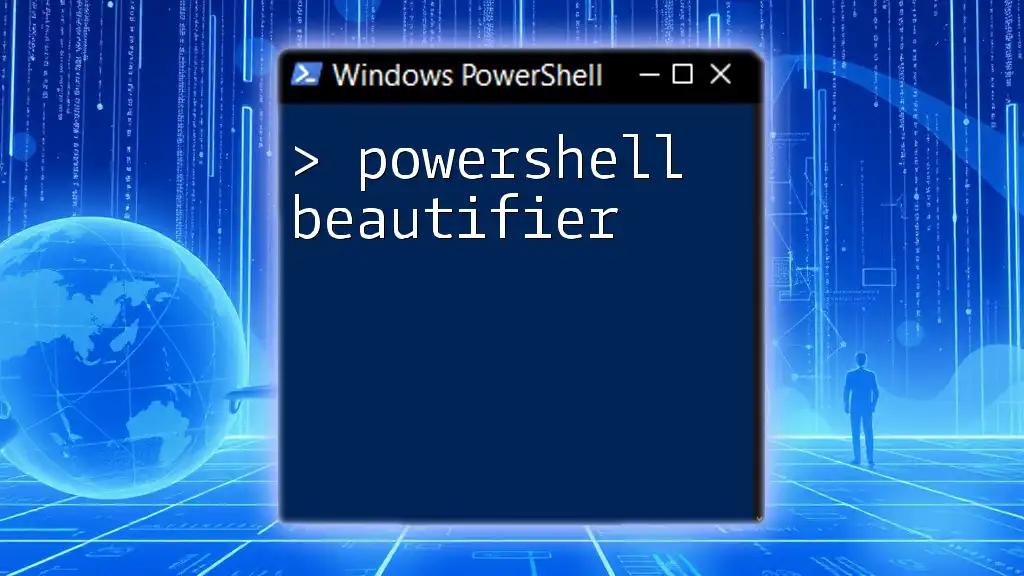
Reading INI Files in PowerShell
Overview of Reading Methods
You can read INI files in PowerShell using various methods:
- Manual string manipulation: Involves direct parsing of the INI file into a usable data structure.
- Creating custom functions: Offers reusability and cleaner code for reading INI files.
- Utilizing .NET classes: Provides advanced parsing capabilities suited for more complex needs.
Method 1: Manual String Manipulation
In this approach, you read the INI file and manually process its content. Below is the code snippet that demonstrates this method:
$iniContent = Get-Content "path\to\file.ini"
$iniData = @{}
foreach ($line in $iniContent) {
if ($line -match '^\[(.+?)\]') {
$section = $matches[1]
$iniData[$section] = @{}
} elseif ($line -match '^(.*?)=(.*)$') {
$key = $matches[1].Trim()
$value = $matches[2].Trim()
$iniData[$section][$key] = $value
}
}
In this code:
- `Get-Content` retrieves the file's content as an array of lines.
- A loop processes each line, identifying sections and key-value pairs using regular expressions.
- The resulting `$iniData` variable will be a nested hash table that reflects the INI file structure.
Method 2: Creating a Custom Function for Reusability
Creating a custom function allows you to reuse your parsing logic without rewriting code each time you need it. Here’s an example:
function Read-IniFile {
param(
[string]$path
)
$iniContent = Get-Content $path
$iniData = @{}
foreach ($line in $iniContent) {
if ($line -match '^\[(.+?)\]') {
$section = $matches[1]
$iniData[$section] = @{}
} elseif ($line -match '^(.*?)=(.*)$') {
$key = $matches[1].Trim()
$value = $matches[2].Trim()
$iniData[$section][$key] = $value
}
}
return $iniData
}
$iniFile = Read-IniFile "path\to\file.ini"
In this example:
- Parameters: The function accepts a file path, making it flexible.
- Return value: It returns a structured hashtable that can be easily manipulated in your script.
Method 3: Utilizing .NET Classes for Advanced Parsing
If you anticipate working with complex INI files, leveraging .NET for parsing could be beneficial. Here’s how you can create a parser class in PowerShell:
Add-Type @"
using System;
using System.Collections.Generic;
using System.IO;
public class IniFileParser {
private Dictionary<string, Dictionary<string, string>> _data;
public IniFileParser(string path) {
_data = new Dictionary<string, Dictionary<string, string>>();
ParseFile(path);
}
private void ParseFile(string path) {
string currentSection = "";
foreach (var line in File.ReadAllLines(path)) {
if (line.StartsWith("["))
currentSection = line.Trim('[', ']');
else if (line.Contains("=")) {
var parts = line.Split(new char[] { '=' }, 2);
var key = parts[0].Trim();
var value = parts[1].Trim();
if (!_data.ContainsKey(currentSection))
_data[currentSection] = new Dictionary<string, string>();
_data[currentSection][key] = value;
}
}
}
public Dictionary<string, Dictionary<string, string>> GetData() {
return _data;
}
}
"@
$parser = New-Object IniFileParser "path\to\file.ini"
$iniData = $parser.GetData()
In this approach:
- Custom parser class: This allows for modular code and adheres to object-oriented principles.
- Error handling: You may extend it with error-handling capabilities for better robustness.

Practical Use Cases: How to Use Read INI File Functionality
Fetching Specific Values from the Data Structure
Once you have your data parsed, retrieving specific values is straightforward. For instance:
$value = $iniData["Database"]["User"]
Write-Output "The database user is: $value"
In this example, you can quickly fetch values using section and key names. It's advisable to implement error handling logic to manage cases where a section or key may not exist, which will enhance your script's robustness.
Writing Back to INI Files
If your script modifies the contents and you need to write them back, consider maintaining a structure for saving changes. Here’s a simple outline for how you could implement this:
function Write-IniFile {
param(
[string]$path,
[hashtable]$data
)
$output = ""
foreach ($section in $data.Keys) {
$output += "[$section]`n"
foreach ($key in $data[$section].Keys) {
$output += "$key=$($data[$section][$key])`n"
}
}
Set-Content -Path $path -Value $output
}
Write-IniFile "path\to\file.ini" $iniData
This function constructs a string representing the INI file and writes it back to disk. Care must be taken to ensure that the formatting remains consistent.

Conclusion
Understanding how to read INI files in PowerShell enables you to leverage these simple configuration files effectively within your scripts. Whether using manual methods, custom functions, or .NET classes, you have several options at your disposal that cater to your specific needs.
Explore the sample codes provided and experiment with them in your environment. Mastering these skills can enhance your PowerShell expertise and enable you to handle configuration settings dynamically.
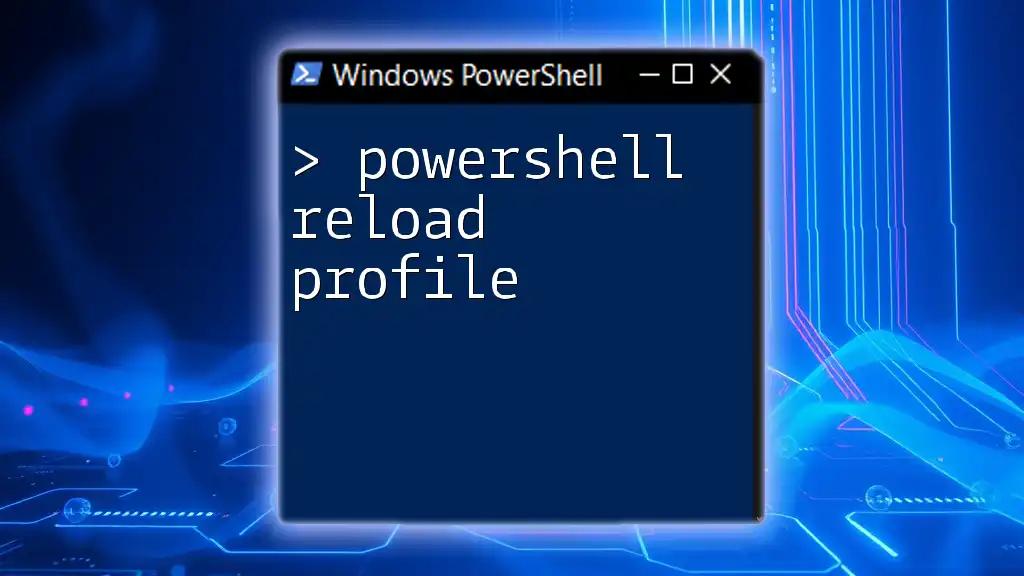
Additional Resources
Consider checking out community forums or online courses to further deepen your understanding of PowerShell scripting and broaden your knowledge of its capabilities.