Reading a file line by line in PowerShell can be accomplished easily using the `Get-Content` cmdlet, which allows you to process each line individually.
Get-Content "C:\path\to\your\file.txt" | ForEach-Object { Write-Host $_ }
Understanding Text Files in PowerShell
Text files play a crucial role in scripting and automation with PowerShell. They can vary widely in format, such as plain text (.txt) or formatted data (.csv), but their basic structure remains relatively simple. Understanding the types of text files at your disposal allows you to select the appropriate methods for data manipulation and processing.
Text files are ubiquitous throughout IT and programming environments. You might encounter them in log files, configuration files, or even as part of larger workflows. The ability to read text files line by line is essential for extracting relevant data and automating tasks effectively.
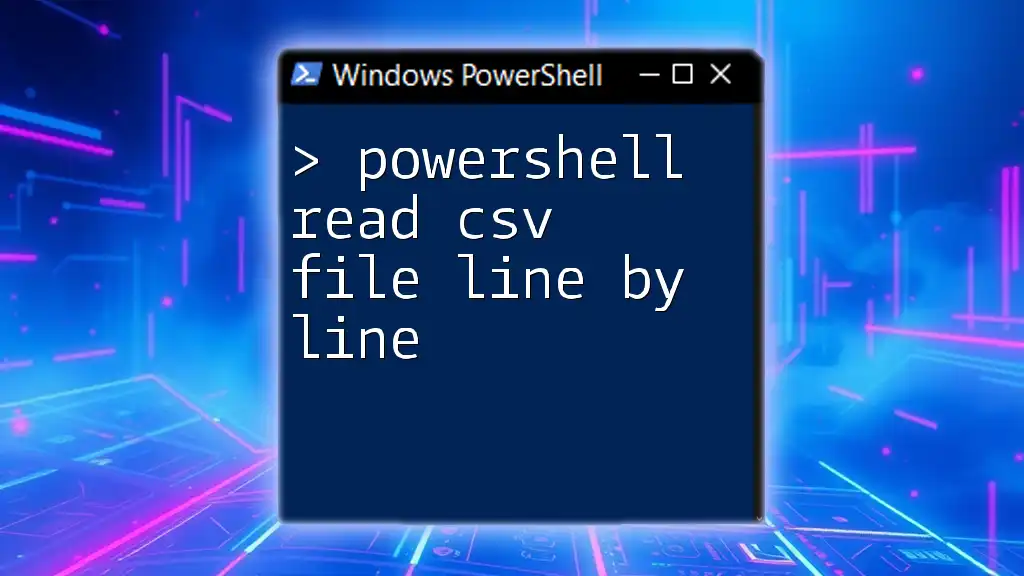
PowerShell Cmdlets for File Operations
PowerShell provides a robust set of cmdlets specifically designed for file operations. Familiarity with these cmdlets is vital for performing effective data operations.
Key Cmdlets to Know:
- `Get-Content`: This cmdlet retrieves the contents of a file, making it the primary command for reading text files.
- `Set-Content`: Use this cmdlet to write or replace the contents of a file. While it doesn't specifically focus on reading data, it's invaluable when you're updating text files.
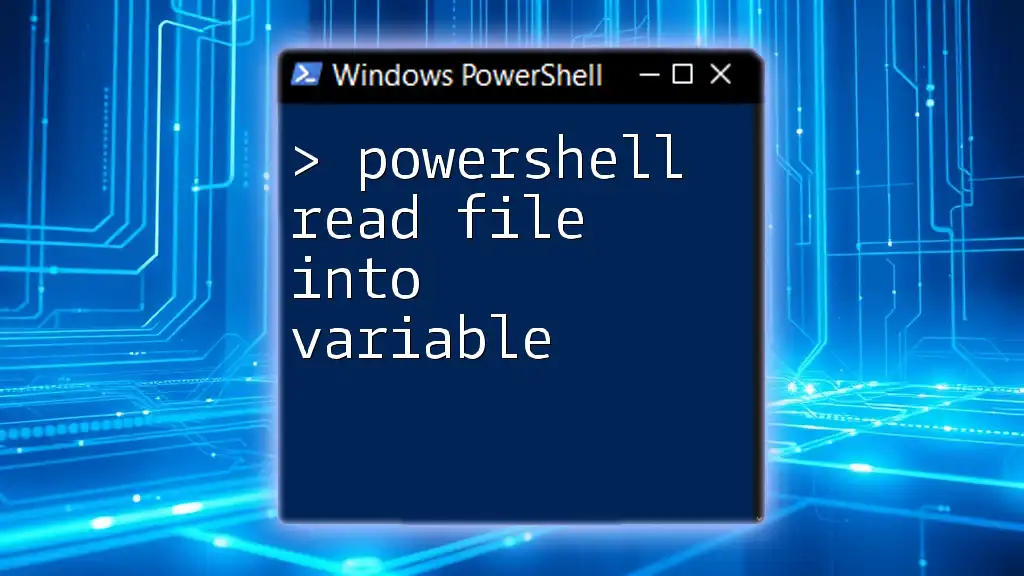
Reading Files Line by Line
Using `Get-Content`
The foundation for powerfully reading files in PowerShell lies within the `Get-Content` cmdlet. The basic syntax for this cmdlet is straightforward:
Get-Content -Path "C:\path\to\your\file.txt"
This command reads the entire file located at the specified path and outputs the content to the console.
Example of Reading a File:
Imagine you have a text file that lists server names. You can read and print each line with the following code snippet:
$lines = Get-Content -Path "C:\path\to\your\file.txt"
foreach ($line in $lines) {
Write-Output $line
}
In this example, `$lines` is an array that stores each line in the text file. The `foreach` loop then iterates through each line, allowing us to perform operations, such as displaying or modifying the data.
PowerShell `ForEach` with Line by Line Processing
You can also leverage the `ForEach-Object` cmdlet for line-by-line processing directly from the pipeline. Here’s how you can do it:
Get-Content -Path "C:\path\to\your\file.txt" | ForEach-Object {
$_
}
Here, `$_` represents the current object in the pipeline (which, in this case, is each line of the text file). This method is particularly useful for chaining commands and performing operations on each line without creating a separate array.
Example Scenario:
Suppose you need to filter lines containing a specific keyword (e.g., "error"). You can combine these commands as follows:
Get-Content -Path "C:\path\to\your\file.txt" | Where-Object { $_ -like "*error*" } | ForEach-Object {
Write-Output $_
}
This snippet reads through the file, filters out lines containing "error," and outputs them.
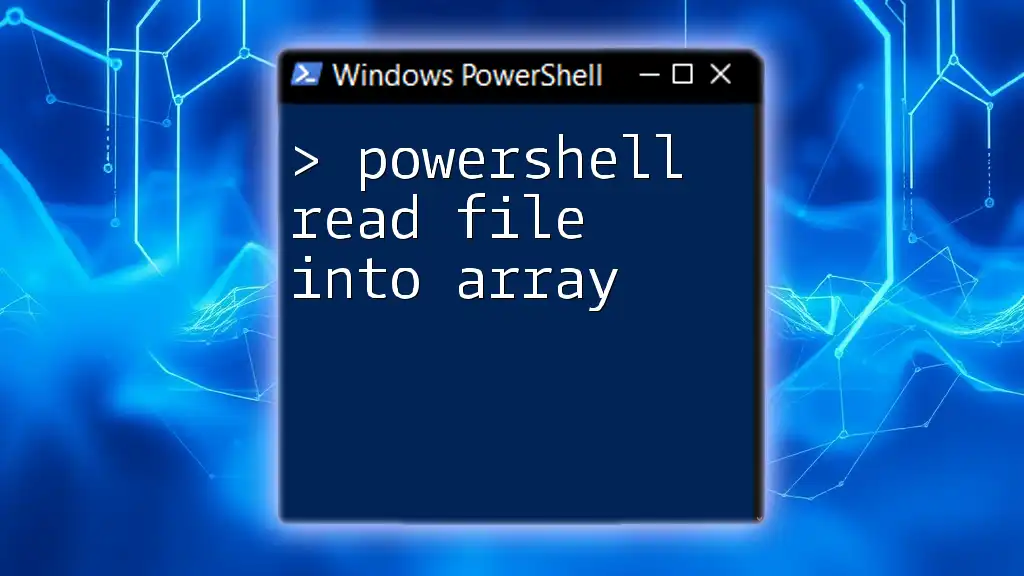
Advanced Techniques
Selectively Reading Lines
In certain scenarios, you might not need the entire contents of a text file. You can selectively access lines using the `Select-Object` cmdlet. For instance, to read the first ten lines of a file, use:
Get-Content -Path "C:\path\to\your\file.txt" | Select-Object -First 10
This technique allows you to grab small samples of data for quick checks or analysis.
Filtering Lines
Using `Where-Object`, you can filter specific content directly during the reading process. For example, to read and filter lines containing a keyword:
Get-Content -Path "C:\path\to\your\file.txt" | Where-Object { $_ -like "*keyword*" }
This command effectively narrows down the lines you retrieve, making it easier to focus on relevant information.
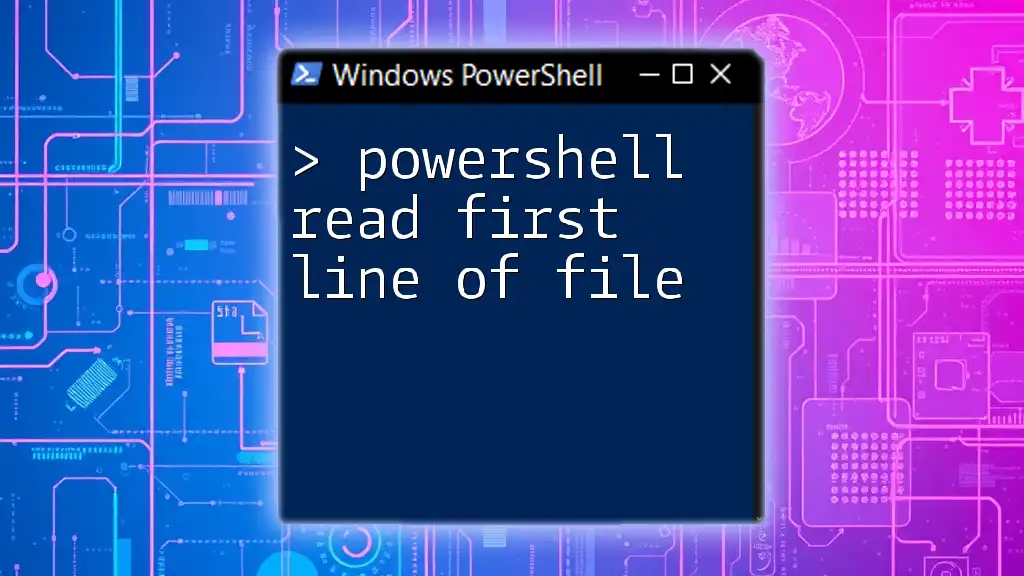
Performance Considerations
When working with large files, performance can become an issue. PowerShell provides several techniques to handle large datasets efficiently.
Processing Large Files
Using `-ReadCount` with `Get-Content` can significantly improve performance by controlling how many lines are read at a time. For instance:
Get-Content -Path "C:\path\to\your\file.txt" -ReadCount 0
This command reads the entire file in one go, reducing the overhead of multiple `Get-Content` calls, which can be beneficial for large files.
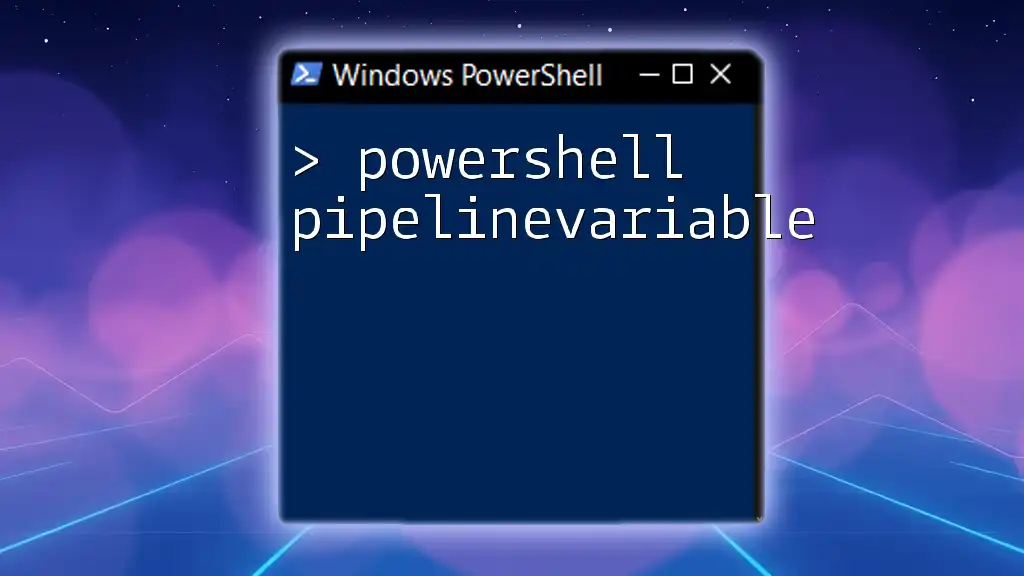
Error Handling
Working with files sometimes leads to unexpected issues, such as missing files or permission errors. Implementing error handling enhances the robustness of your scripts.
Common Errors
Some typical issues include:
- File not found errors.
- Unauthorized access errors.
- Empty file errors.
Using Try-Catch Blocks
A simple way to handle potential errors is with `try-catch` blocks. Here's a basic example:
try {
Get-Content -Path "C:\path\to\your\file.txt"
} catch {
Write-Error "An error occurred: $_"
}
Using this structure captures any errors thrown by `Get-Content` and displays a user-friendly message, ensuring that your script can fail gracefully.
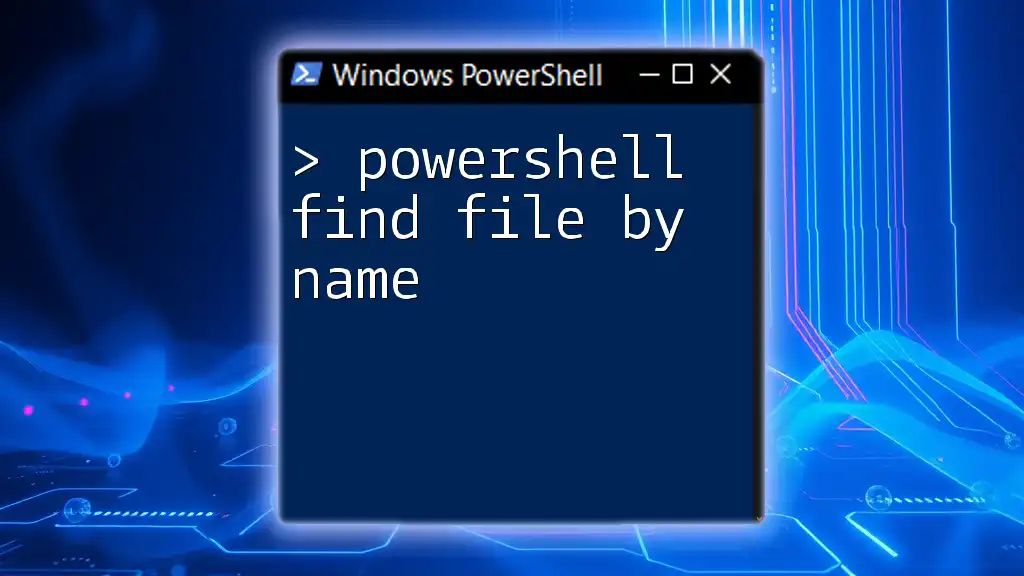
Conclusion
Throughout this article, we've explored the essential aspects of Powershell reading file line by line. Understanding how to use the `Get-Content`, `ForEach-Object`, and `Where-Object` cmdlets equips you with powerful tools for managing files effectively. As you practice these techniques, you'll gain confidence in your automation tasks, enabling you to tackle more complex workflows.
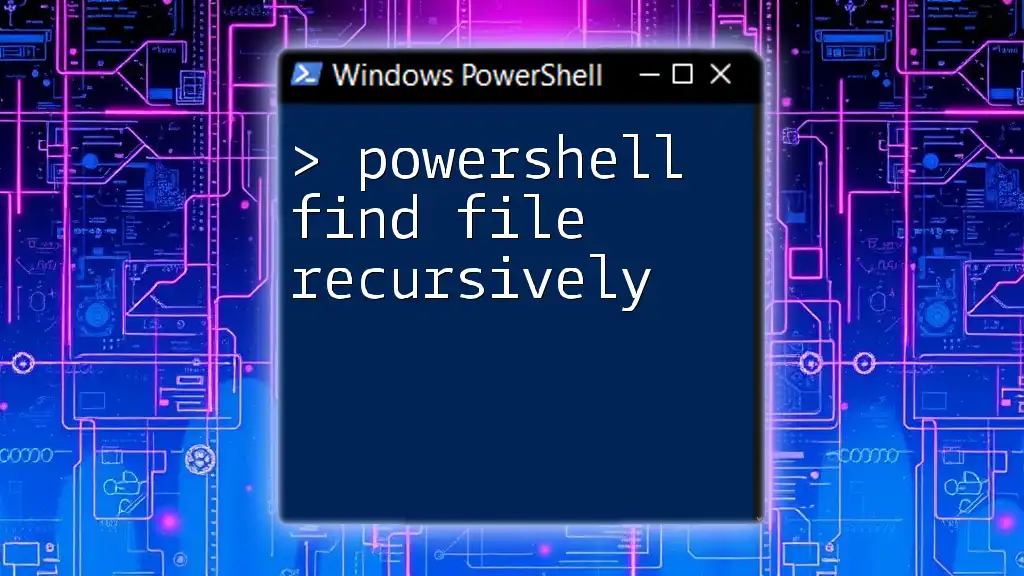
Call to Action
Jump into your next PowerShell project armed with these insights! Share your experiences, challenges, and successes with the PowerShell community. By collaborating, we can all learn and grow together in mastering this powerful scripting language. Don't forget to subscribe for more tips and tricks on PowerShell!
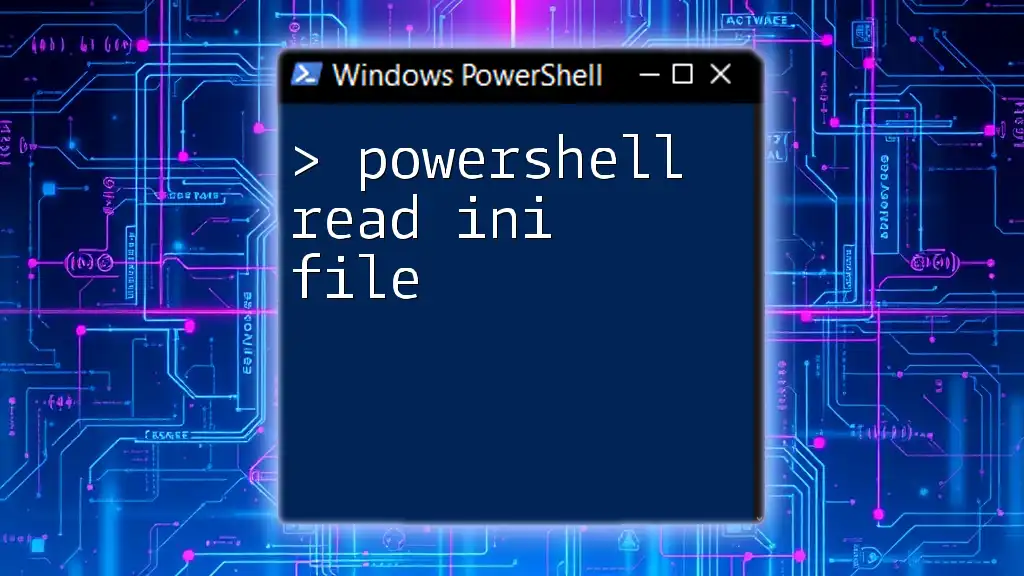
Additional Resources
For further exploration, here are some resources to enhance your PowerShell skills:
- Official [Microsoft PowerShell Documentation](https://docs.microsoft.com/powershell/)
- PowerShell community blogs and tutorials for real-world use cases.