To read the first line of a file in PowerShell, you can use the following command:
Get-Content 'C:\path\to\your\file.txt' -TotalCount 1
Understanding PowerShell File Operations
What is PowerShell?
PowerShell is a cross-platform automation and configuration tool that combines a command-line shell with a scripting language. Initially conceived for system administration, it has evolved into a powerful tool for a wide range of automation tasks, making it an indispensable part of any IT professional's toolkit. With its ability to interact seamlessly with .NET objects and access COM and WMI, PowerShell allows for versatile means of performing complex tasks effortlessly.
Why Read Files in PowerShell?
Reading files is a fundamental operation in many scripting scenarios. You might find yourself needing to quickly inspect configuration files, read log entries, or extract data for further processing. The ability to read files quickly and easily makes PowerShell a preferred choice for many users. Moreover, leveraging PowerShell’s cmdlets allows for efficient data manipulation, which can enhance productivity and automate monotonous tasks.
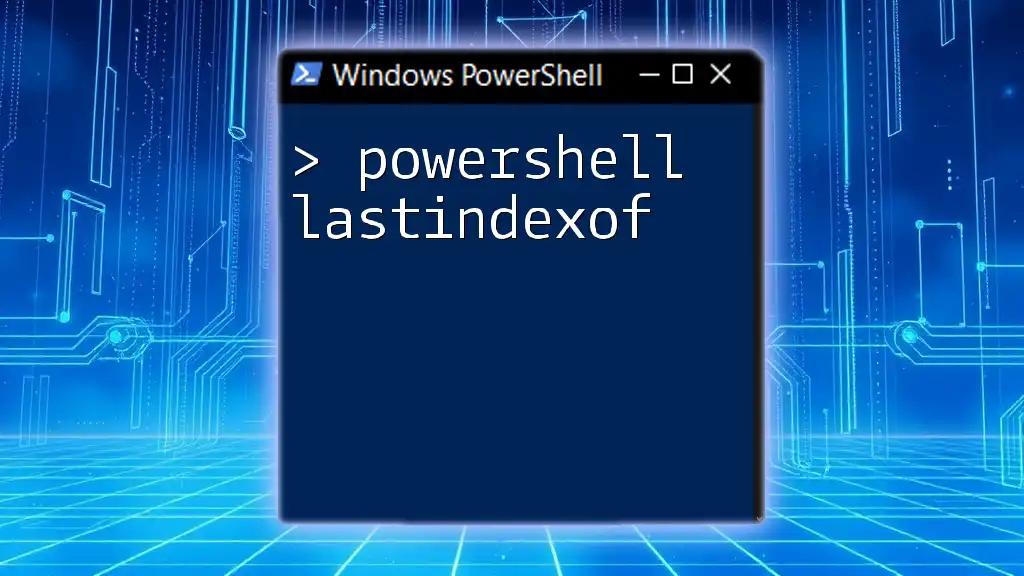
Getting Started with PowerShell
Setting Up Your Environment
Before diving into file operations, ensure your environment is set up correctly. Most Windows operating systems come with PowerShell pre-installed. However, for Linux and macOS, you may need to download and install it from the official PowerShell GitHub repository.
Basic PowerShell Commands
PowerShell uses a command line structure known as cmdlets. A cmdlet is a lightweight command that is used in the PowerShell environment. To read contents from a file, you will mainly use the `Get-Content` cmdlet, which retrieves the content of a text file.
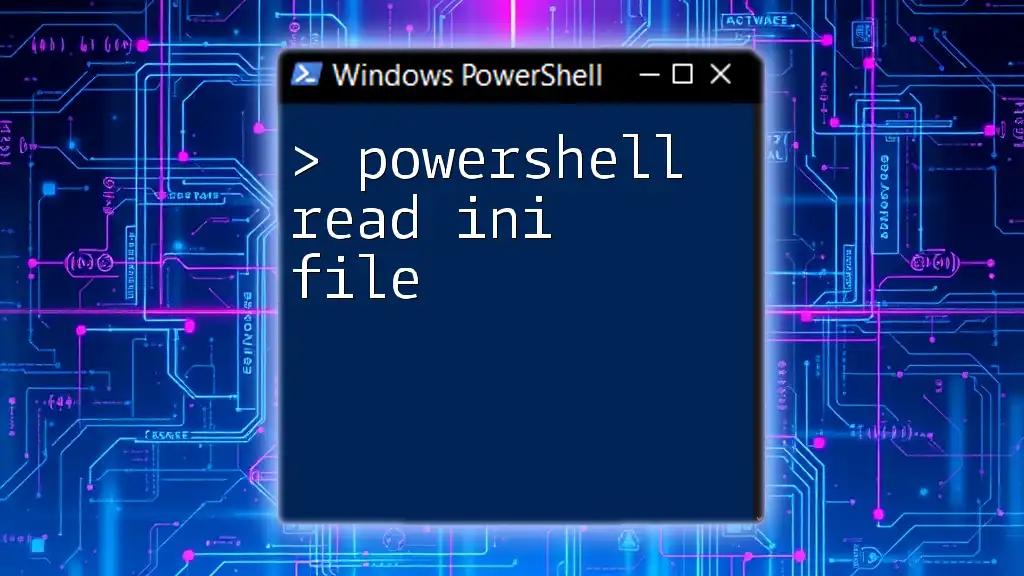
Reading the First Line of a File
Using Get-Content
What is Get-Content?
The `Get-Content` cmdlet is designed to read content from a file. It can read text files and output the contents line-by-line.
Syntax Explanation
The basic syntax of `Get-Content` is straightforward:
Get-Content "C:\path\to\your\file.txt"
This command will read the entire content of the specified file. However, when you're only interested in the first line, you need to employ additional methods.
Reading Only the First Line
Using Select-Object
You can easily read just the first line of a file by combining `Get-Content` with the `Select-Object` cmdlet. Here’s how:
Get-Content "C:\path\to\your\file.txt" | Select-Object -First 1
In this example, only the first line of the file will be returned. This method is efficient because it stops processing as soon as the first line is read, making it resource-friendly.
Using Indexing
You can also access the first line using indexing. In PowerShell, collections and arrays are index-based, meaning you can reference individual elements by their position:
$firstLine = (Get-Content "C:\path\to\your\file.txt")[0]
Write-Output $firstLine
Here, `(Get-Content "C:\path\to\your\file.txt")[0]` retrieves the first line of the file. Note that indexing starts at zero, so the first element is at position 0.

Additional Techniques and Considerations
Reading Files with Different Encoding
Files can have various encodings, which may affect how content is read. It’s crucial to specify the encoding when dealing with files that are not in plain ASCII. Here is how you can do this:
Get-Content "C:\path\to\your\file.txt" -Encoding UTF8 | Select-Object -First 1
This command reads the first line of a file encoded in UTF8.
Handling Multiple Lines Efficiently
If you want to enlarge the scope to read multiple lines but still maintain simplicity, the `-TotalCount` parameter is available:
Get-Content "C:\path\to\your\file.txt" -TotalCount 1
This command will return the first line, ensuring minimal resource usage.
Error Handling
Handling errors gracefully when reading files is essential for robust scripts. Use the `Try/Catch` block to catch and respond to potential issues:
try {
$firstLine = Get-Content "C:\path\to\your\file.txt" | Select-Object -First 1
}
catch {
Write-Output "An error occurred: $_"
}
This structure not only prevents your script from crashing but also allows you to display useful error messages to the user.

Practical Applications
Use Cases in Real-World Scenarios
Configuration Files: Often, scripts require reading configuration settings from files; PowerShell excels in this due to its lightweight file operations.
Log File Analysis: For server-side scripts, reading the first line of log files can provide instant insights, making it easier to troubleshoot.
Scripting Automation Tasks: Automate repetitive tasks by extracting specific information quickly, such as usernames or service statuses from text files.

Conclusion
In summary, mastering how to read the first line of a file with PowerShell is a valuable skill that can streamline your workflow and enhance your automation capabilities. The combination of `Get-Content`, `Select-Object`, and proper error handling equips you with the tools to manage file data effectively.
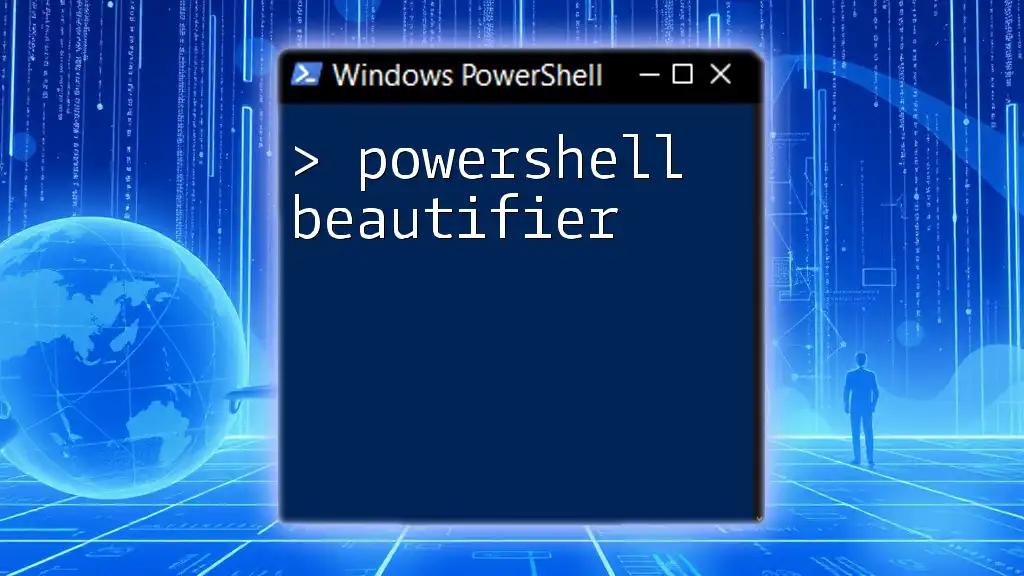
Frequently Asked Questions
How can I read the first line of a file in PowerShell?
To read the first line of a file in PowerShell, you can use:
Get-Content "C:\path\to\your\file.txt" | Select-Object -First 1
Can I read files with different formats (CSV, JSON, etc.)?
Yes, PowerShell can read various file formats, although the methods may differ. For instance, CSV files work well with `Import-Csv`, while JSON can be managed using `Get-Content` coupled with conversion cmdlets.
What are some common pitfalls when using Get-Content?
One common pitfall is not acknowledging file encoding, which can lead to incorrect readings. Also, remember the impact of large file sizes—consider using filtering techniques like `Select-Object` to enhance performance.
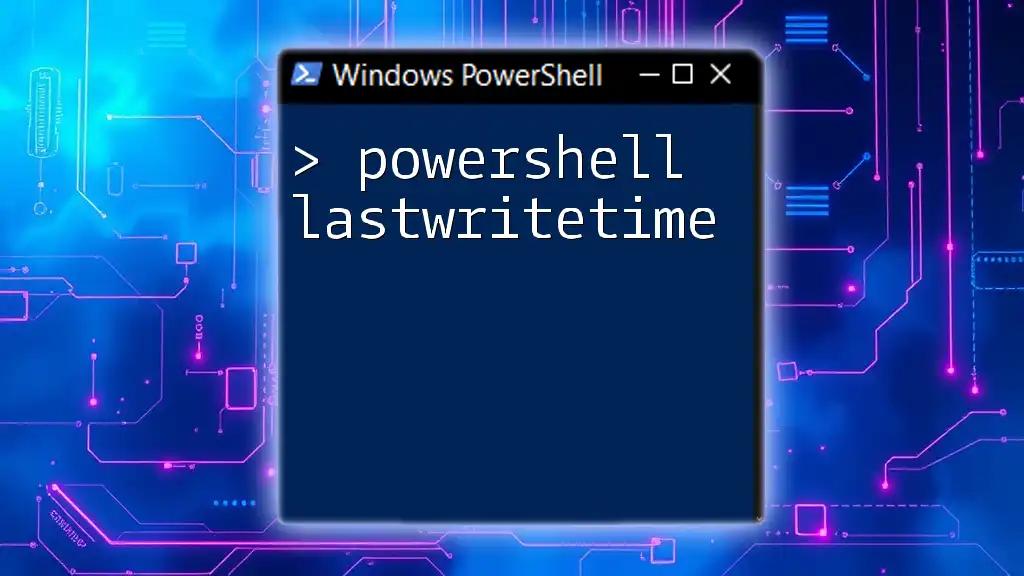
Additional Resources
For further learning, you can visit the official [PowerShell documentation](https://docs.microsoft.com/en-us/powershell/) and explore community forums for practical scripts and solutions.