To determine the encoding of a file in PowerShell, you can use the `Get-Content` cmdlet with the `-Encoding` parameter specified as `Byte` to read the file and then check its byte order mark (BOM). Here’s a code snippet:
$FilePath = "C:\Path\To\Your\File.txt"
$BOM = (Get-Content -Path $FilePath -Encoding Byte -TotalCount 3) -join ', '
Write-Host "File encoding bytes: $BOM"
Understanding File Encoding
What is File Encoding?
File encoding refers to the method of converting characters into bytes, allowing computers to store and manipulate text efficiently. Different file encodings use various character representations, which is crucial for accurate data interpretation.
Common types of file encodings include:
- UTF-8: A variable-width character encoding capable of encoding all valid character code points in Unicode. It's the most common encoding on the web.
- UTF-16: Used primarily in Windows environments, this encoding can represent every character in Unicode. It often requires more space than UTF-8.
- ASCII: A simpler encoding for representing English characters. It uses one byte per character but is limited to 128 symbols.
Understanding file encoding is vital because it directly affects how text data is read, written, and displayed. Misrepresenting a file's encoding can lead to data corruption, lost information, or errors in scripts.
Why is Encoding Important in PowerShell?
In PowerShell, correctly handling file encoding is essential when reading from or writing to files. If the encoding of a script does not match the encoding of the file being processed, it can result in unexpected behaviors or inaccurate data. This is particularly true in scripts dealing with internationalization or when working with various file formats.
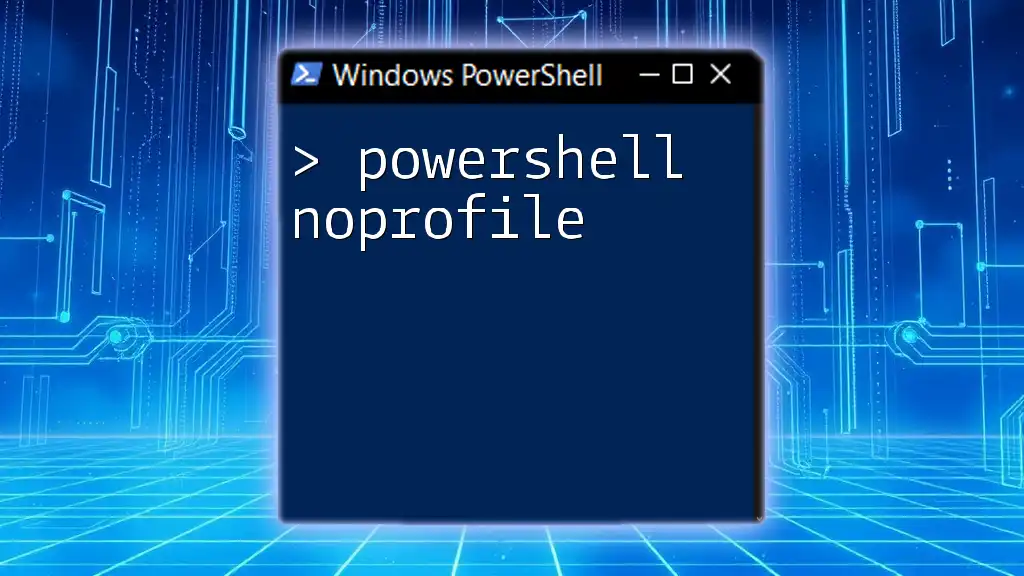
PowerShell Basics for File Encoding
Key Cmdlets Related to File Encoding
PowerShell provides several cmdlets that are useful for managing file content, particularly regarding encoding. Notable cmdlets include:
- Get-Content: Reads the content of a file and can return it with specified encoding.
- Set-Content: Writes content to a file, allowing you to define the file's encoding.
- Out-File: Directs output to a file and allows for determining the encoding type.
Default Encoding in PowerShell
PowerShell's encoding behavior varies among versions. By default, PowerShell 5.1 and later versions use UTF-8 encoding for `Out-File` and `Set-Content` cmdlets, while `Get-Content` reads files using UTF-16 unless specified otherwise.
It's important to understand these defaults to avoid surprises when handling file operations.
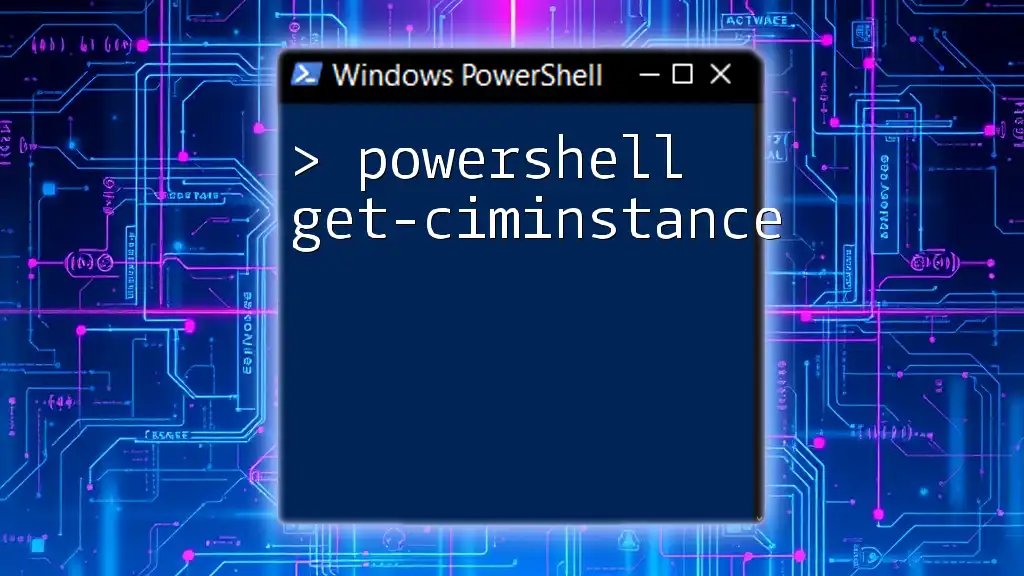
How to Get the Encoding of a File
Using `Get-Content` Cmdlet
To determine the encoding of a file, the `Get-Content` cmdlet can be considered. Reading a file's content as bytes provides insight into its encoding.
Code Snippet:
$content = Get-Content -Path "example.txt" -Encoding Byte
This command reads the file "example.txt" as a byte array, allowing you to analyze the bytes and infer the encoding. You can follow this by inspecting the byte signature, also known as the Magic Number, to identify encodings like UTF-8 or UTF-16.
Reading File Encoding with .NET Classes
Using System.IO.StreamReader
PowerShell is built on .NET, and developers can leverage its robust functionality. The `System.IO.StreamReader` class can be used to read the encoding of a file easily.
Code Snippet:
$reader = [System.IO.StreamReader]::new("example.txt")
$encoding = $reader.CurrentEncoding
This method returns the current encoding in use for the file, providing an easy way to ascertain the file's encoding directly.
Using System.Text.Encoding Class
Another powerful approach is utilizing the `System.Text.Encoding` class to detect file encoding more explicitly.
Code Snippet:
$bytes = [System.IO.File]::ReadAllBytes("example.txt")
$encoding = [System.Text.Encoding]::GetEncoding([System.BitConverter]::ToString($bytes[0..3]))
This example reads the file's bytes into an array and uses the first few bytes to determine the encoding type. It's crucial to note that different file formats may have different byte marker sequences (e.g., BOM) that identify their corresponding encodings.
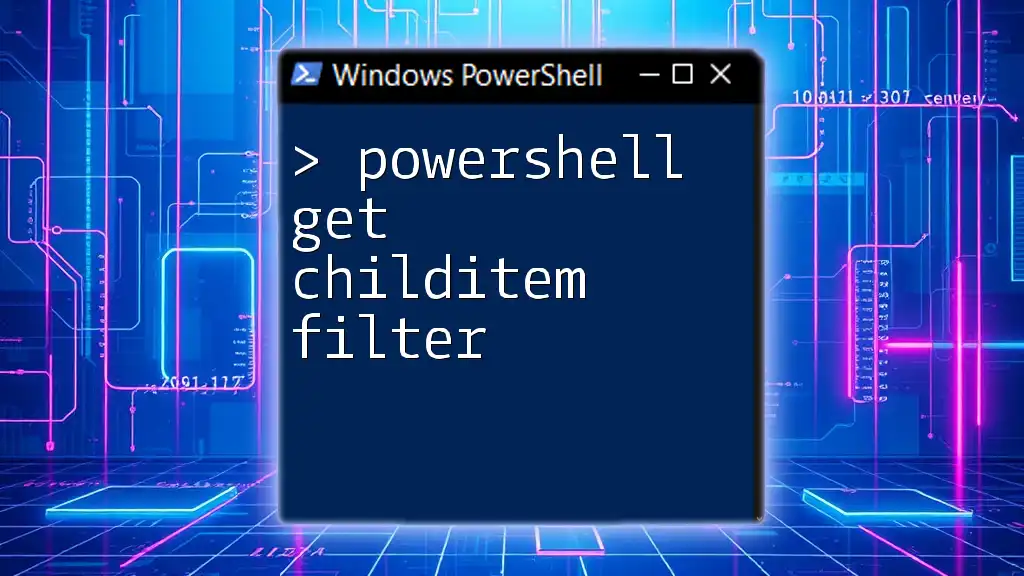
Advantages of Knowing a File's Encoding
Enhancing Script Reliability
Being aware of the file's encoding is essential for script reliability. For instance, mishandling encodings can lead to garbled text or runtime errors, especially when dealing with international characters or special symbols. Knowing the encoding helps ensure that your scripts accurately process data without unexpected interruptions.
Best Practices in File Encoding Management
Here are some best practices for managing file encodings efficiently in PowerShell:
- Specify Encoding: Always specify encoding explicitly when reading from or writing to files to prevent default behaviors from causing issues.
- Test Variability: If working with files from various sources, test and confirm their encoding before processing them in scripts.
- Use consistent encodings: When writing multiple files, choose a consistent encoding to make future data handling easier.
By following these practices, you can minimize errors and enhance your automation processes in PowerShell.
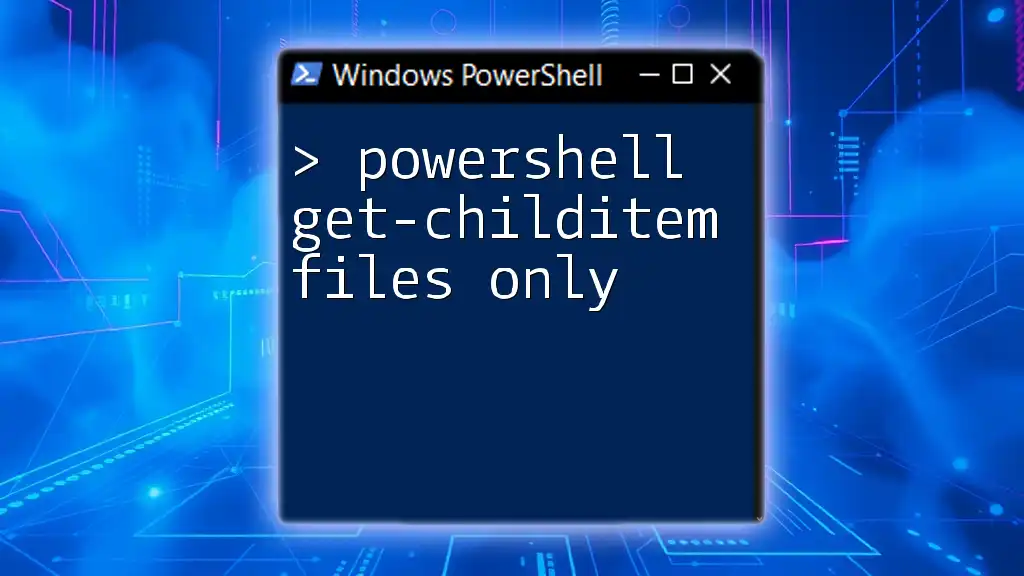
Troubleshooting Common Issues
Error Messages Related to Encoding
Common PowerShell error messages connected to encoding often arise from attempting to read or write files using the wrong encoding type. Typically encountered errors can include:
- “The input is not in the proper format.”
- "Cannot read the file."
To resolve these issues, verify the file's encoding before performing operations. Utilize the methods discussed to determine the correct encoding and adjust your cmdlets accordingly.
Handling Different Encodings in the Same Script
When working with multiple files or sources, it's not uncommon to encounter different encodings. To effectively handle varying encodings in your scripts, consider employing conditional logic or helper functions to detect and manage each file's encoding before processing.
For example, you might create a function to determine a file's encoding upon reading, applying the correct command based on this determination.
function Get-FileEncoding {
param (
[string]$Path
)
$bytes = [System.IO.File]::ReadAllBytes($Path)
return [System.Text.Encoding]::GetEncoding([System.BitConverter]::ToString($bytes[0..3]))
}
With such flexibility, your scripts can adapt as necessary, enhancing their robustness in file processing.
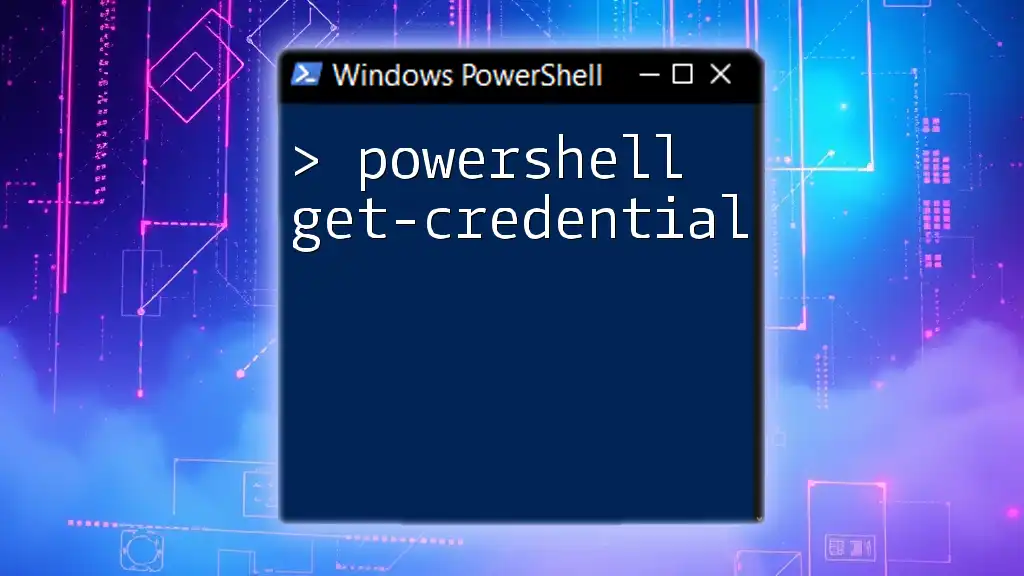
Conclusion
In summary, understanding how to determine the encoding of a file using PowerShell is vital for successful script execution and data manipulation. Mismanaging file encodings can lead to significant issues, but with the techniques reviewed in this article, you can confidently tackle encoding challenges in your automation tasks.
By practicing and applying these methods in your scripts, you'll enhance accuracy and efficiency within your PowerShell workflows.
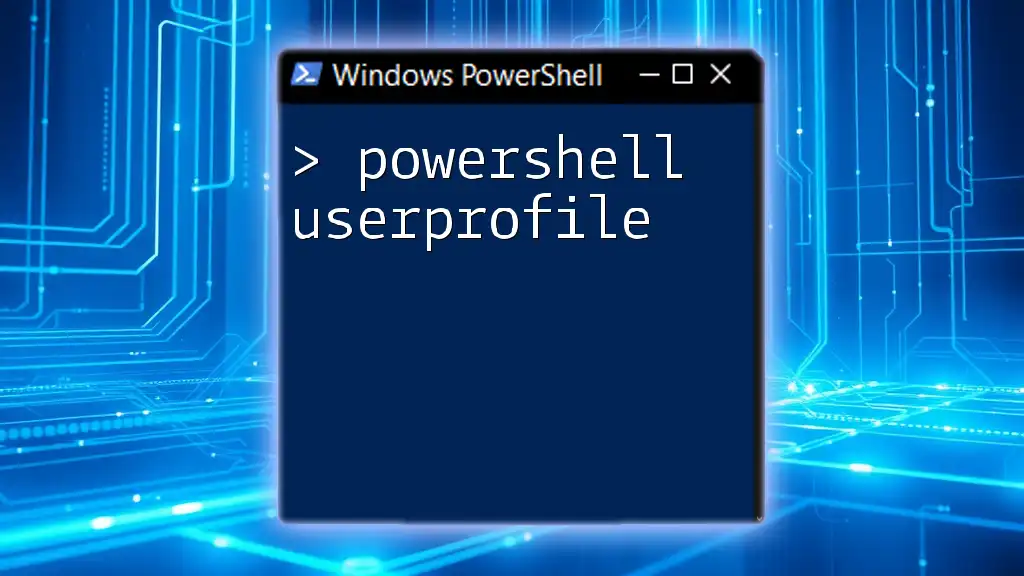
Additional Resources
For further reading, consider checking Microsoft's official documentation on PowerShell encoding or seek out community forums for more in-depth discussions and troubleshooting assistance related to PowerShell and file handling.
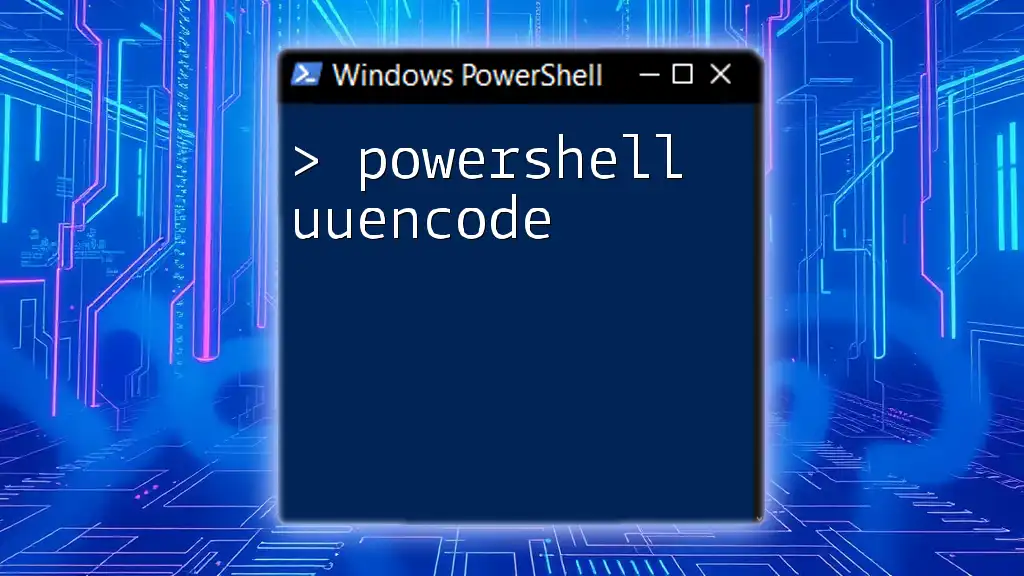
Call to Action
We invite you to engage with the community by sharing your own experiences or asking questions about managing file encodings in PowerShell. Subscribe to stay updated with more tips and tutorials that will enhance your PowerShell skills!