PowerShell logging to a file allows you to capture and save your script output or commands executed in your PowerShell session by redirecting it to a specified file location.
Here's a code snippet to log output to a text file:
Get-Process | Out-File -FilePath "C:\Logs\processlog.txt"
This command retrieves the list of currently running processes and saves it to a file named `processlog.txt` in the `C:\Logs` directory.
Understanding PowerShell Logging
What is Logging?
Logging refers to the process of recording events, errors, warnings, and informational messages generated by an application or script. In the context of PowerShell, logging allows script authors and administrators to review output from their scripts, enabling them to understand execution flow, troubleshoot issues, and maintain an audit trail. Effective logging practices enhance the reliability and maintainability of scripts.
Benefits of Logging to File
Tracking Script Performance: By logging various stages of a script's execution, you can pinpoint where the script is spending its time. This is crucial for optimization.
Error Diagnosis: When a script encounters an error, having logs can be instrumental in diagnosing what went wrong, allowing for quicker resolutions.
Auditing Actions: Logs provide a historical record of operations performed by the script, which is essential for compliance and review purposes.
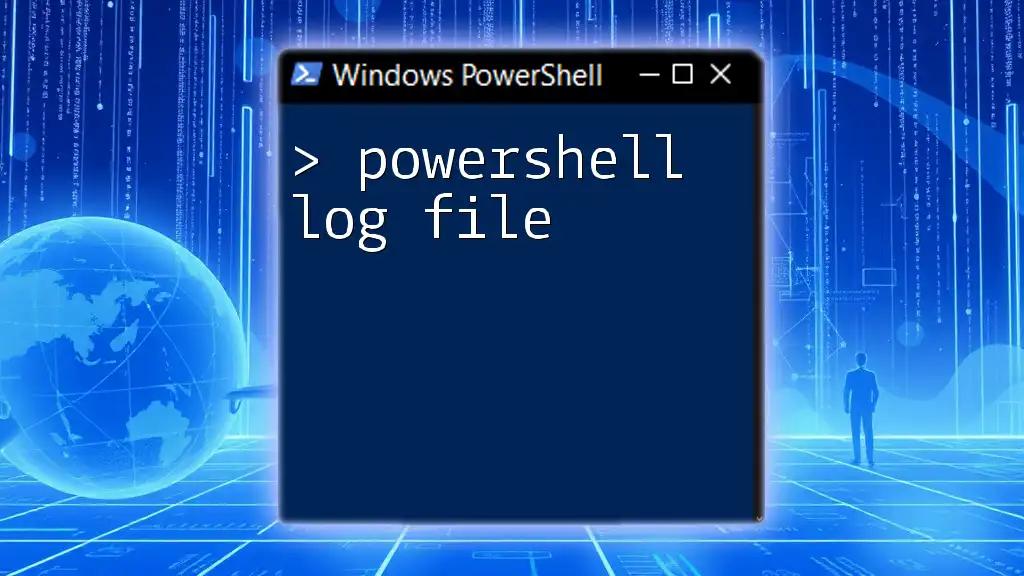
Getting Started with PowerShell Logging
Preparing the Environment
Before you start logging to a file, ensure that you have the necessary permissions to create and write to log files in your desired directory. It's critical to understand the file system so you can choose an appropriate location for storing logs.
Basic Logging Concepts
What is a Log File?
A log file is a text file that stores events recorded by a script, typically containing timestamped entries that describe what happened during script execution. Considerations for log file size and retention are paramount. As logs can grow indefinitely, managing their size and implementing a cleanup process is essential.
PowerShell Write Log File
Using Out-File Cmdlet
One of the most straightforward methods to log information in PowerShell is by using the `Out-File` cmdlet. This cmdlet can take output from other commands and direct it into a log file.
Example: Basic logging
"Script started at $(Get-Date)" | Out-File -FilePath "C:\Logs\scriptlog.txt" -Append
In this example, we are creating a log entry that captures the start time of the script. The `-FilePath` parameter specifies where to save the log, and the `-Append` option ensures that new entries are added to the end of the file without overwriting previous content.
Leveraging the Start-Transcript Cmdlet
For capturing an entire session’s output, the `Start-Transcript` cmdlet is invaluable. It records all outputting text until you stop the transcript.
Example: Capturing session output
Start-Transcript -Path "C:\Logs\transcriptlog.txt"
# Your code goes here
Stop-Transcript
Using `Start-Transcript` allows you to maintain a complete log of all commands run and their outputs, streamlining auditing and debugging efforts.
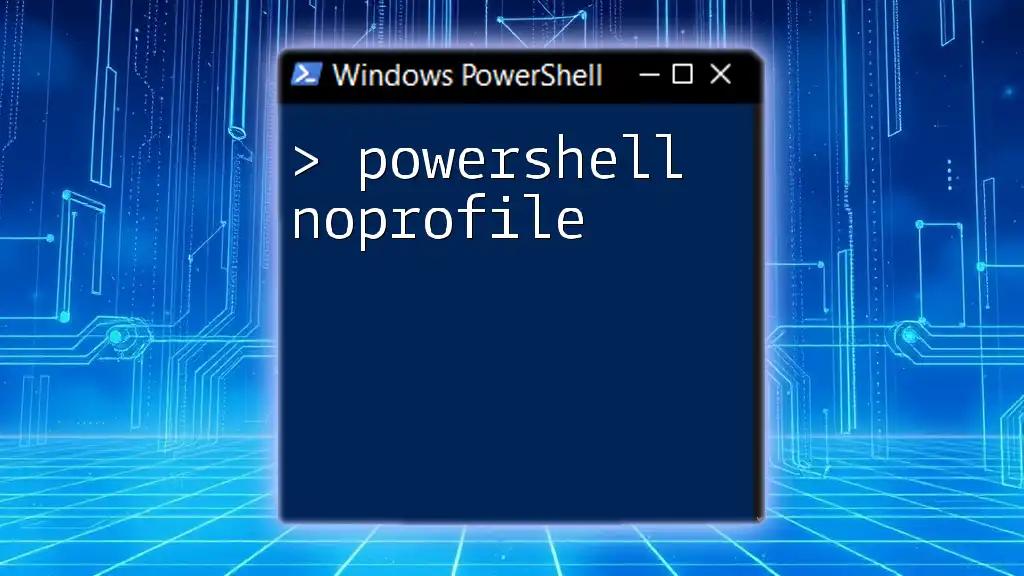
Advanced Logging Techniques
Writing Custom Log Functions
To take logging a step further, you can write your own logging functions that enhance flexibility and control over how log messages are formatted and logged.
Creating a Simple Logging Function
Here is an example of a basic custom logging function that includes a timestamp with each log message:
function Write-Log {
param (
[string]$Message,
[string]$LogFilePath = "C:\Logs\customlog.txt"
)
$Timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
"$Timestamp - $Message" | Out-File -FilePath $LogFilePath -Append
}
In this example, the `Write-Log` function takes a message and an optional file path for the log file. Each time the function is called, it logs a message with a timestamp, making it easier to track when events occur.
Implementing Log Levels
To categorize logs meaningfully, consider implementing log levels such as Info, Warning, and Error. This structure allows for finer control over log output depending on the significance of the message.
Example: Logging with levels
function Write-Log {
param (
[string]$Message,
[string]$Level = "INFO",
[string]$LogFilePath = "C:\Logs\customlog.txt"
)
$Timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
"$Timestamp - [$Level] - $Message" | Out-File -FilePath $LogFilePath -Append
}
With this implementation, users can log different severity levels directly, increasing the utility of the logging output.
Log Rotation and Management
Best Practices for Log Maintenance: It’s essential to manage the size of log files to prevent excessive storage usage and maintain performance. Regularly rotate and archive logs, especially for scripts that run frequently.
Automating Log Cleanup
To automate log management, you can implement scripts that periodically remove or archive old logs.
Example: PowerShell script for cleanup
$LogPath = "C:\Logs\"
Get-ChildItem -Path $LogPath -Filter *.txt | Where-Object { $_.LastWriteTime -lt (Get-Date).AddDays(-30) } | Remove-Item
This example identifies log files older than 30 days and removes them, helping to keep your logging environment manageable.

Troubleshooting Common Logging Issues
Permission Errors
A common hurdle when logging to a file is encountering permission errors. These often occur when the script lacks the necessary permissions to write to the specified directory. Ensure that the script has adequate rights or adjust execution contexts to resolve these issues.
Log File Size and Performance Considerations
As log files grow large, they can impact performance. Keep logging to an appropriate minimum and consider excluding verbose output in production environments to maintain efficiency.
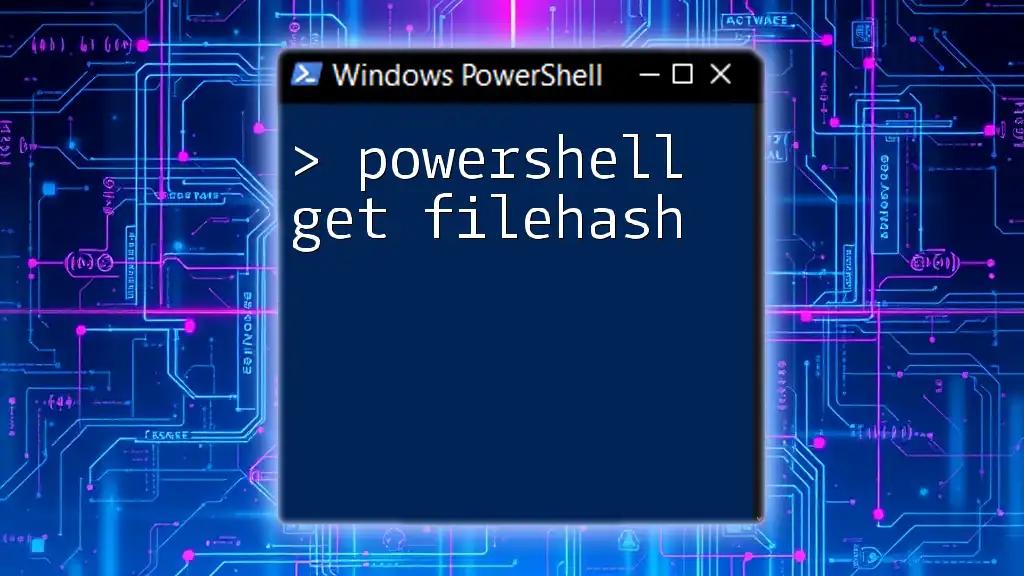
Conclusion
PowerShell logging to file is a powerful feature that enhances script reliability and provides valuable insights into script execution and performance. By leveraging the techniques outlined in this guide, you can effectively implement logging that meets your needs, enabling you to track performance, diagnose issues, and maintain comprehensive records of script executions. Start logging today to ensure your PowerShell scripts are both manageable and actionable.
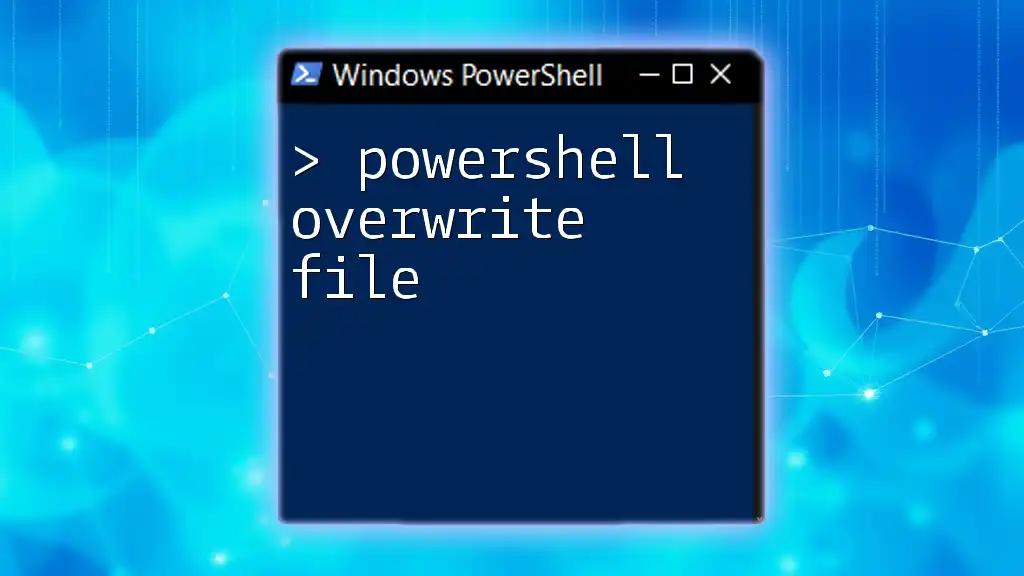
Frequently Asked Questions (FAQs)
Common questions arise regarding logging practices in PowerShell, including how to adapt logging techniques to various environments and specific use cases. Engaging with the PowerShell community or perusing resources from Microsoft can provide you with additional guidance and methods to refine your logging process.