PowerShell's `uuencode` is a method used to convert binary data into a text format for easier transmission, especially in scenarios involving email or text-only systems.
Here’s a code snippet to demonstrate how to use `uuencode` in PowerShell:
$inputFile = "path\to\your\file.bin"
$outputFile = "path\to\your\file.uue"
[System.Convert]::ToBase64String([System.IO.File]::ReadAllBytes($inputFile)) | Set-Content $outputFile
Understanding UUEncoding
What is UUEncoding?
UUEncoding, short for Unix-to-Unix encoding, is a method for converting binary data into a text representation. This encoding scheme is particularly useful for scenarios where binary data needs to be transferred in environments that only support text. Its primary purpose is to allow safe transmission of binary files over media that may corrupt or limit binary data, such as email systems.
History of UUEncoding
Initially developed in the early days of Unix, UUEncoding was designed to facilitate the sharing of files between different systems. Over the years, despite the emergence of more modern encoding techniques like Base64, UUEncoding remains relevant for specific applications, especially in legacy systems and email protocols.
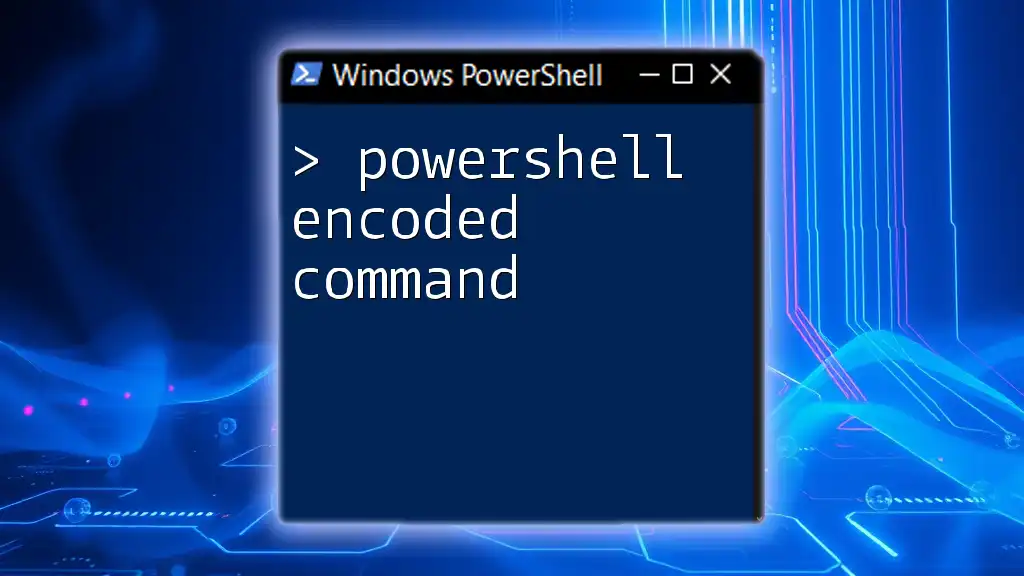
PowerShell and UUEncode
Overview of PowerShell
PowerShell is a powerful task automation and configuration management framework, consisting of a command-line shell and an associated scripting language. It allows users to perform administrative tasks on both local and remote Windows systems. Its flexibility and capabilities make it an ideal environment for executing various types of data manipulation, including file encoding.
Why Use UUEncode in PowerShell?
Using PowerShell for UUEncoding offers several advantages:
- Simplicity: PowerShell commands are easy to understand and execute.
- Integration: Organizations can integrate the encoding process into larger automation scripts.
- Versatility: PowerShell can handle various file types, making it a versatile tool for multiple applications, from sending emails to storing data in databases.
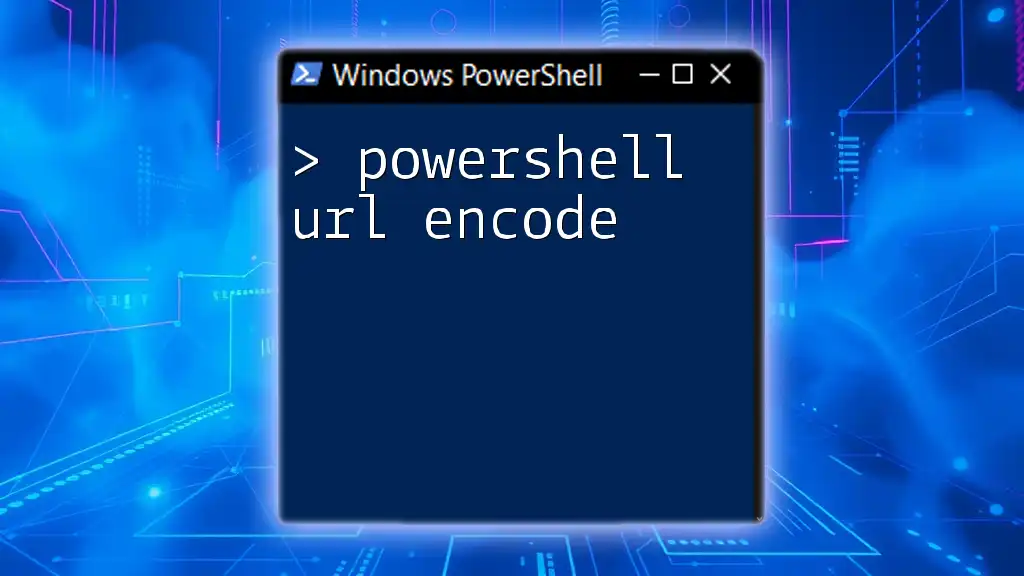
How to UUEncode in PowerShell
Basic UUEncode Syntax
PowerShell provides several cmdlets that can assist with the encoding process. While PowerShell does not have a built-in UUEncode cmdlet, you can achieve similar functionality through Base64 encoding, which is widely compatible.
Step-by-Step Guide to UUEncoding Files
Creating a Sample File
Before encoding, let’s create a simple text file that we will encode:
Set-Content -Path "path\to\your\file.txt" -Value "This is a sample text."
Encoding the File
Once the file is created, you can encode it using the following code:
$filePath = "path\to\your\file.txt"
$encodedString = [Convert]::ToBase64String([System.IO.File]::ReadAllBytes($filePath))
Write-Output $encodedString
In this snippet:
- `[System.IO.File]::ReadAllBytes($filePath)` reads the contents of the file as a byte array.
- `[Convert]::ToBase64String(...)` converts the byte array into a Base64 string, effectively performing the encoding.
- `Write-Output` then displays the encoded string in your PowerShell console.
UUDecoding in PowerShell
Decoding a UUEncoded string back into binary data can be achieved similarly. Here’s how you do it:
$decodedBytes = [Convert]::FromBase64String($encodedString)
[System.IO.File]::WriteAllBytes("path\to\new\file.txt", $decodedBytes)
In this example:
- `[Convert]::FromBase64String($encodedString)` converts the Base64 string back into a byte array.
- `[System.IO.File]::WriteAllBytes(...)` saves the byte array back into a new file on disk.
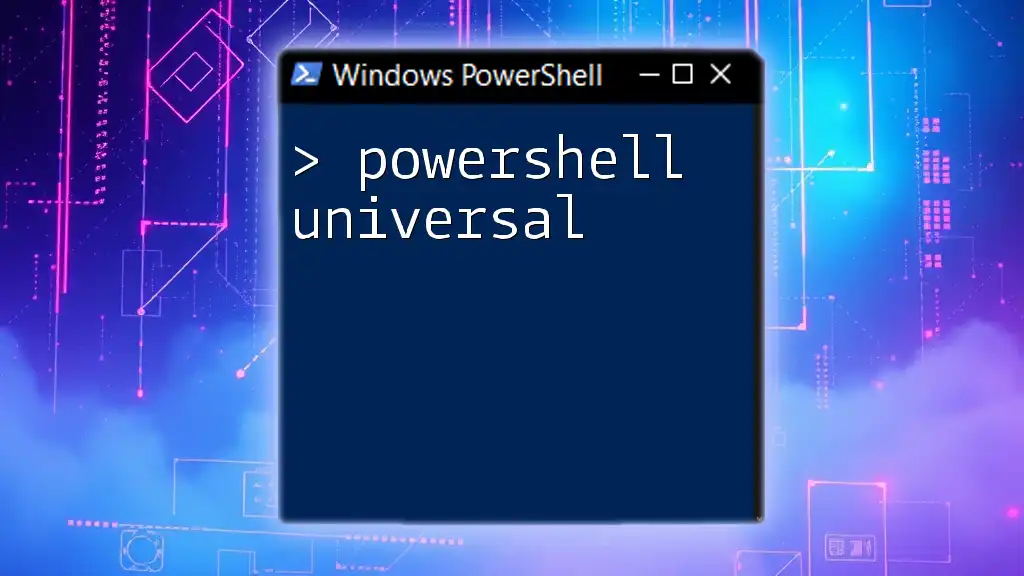
Practical Examples of Using UUEncode
Example 1: Emailing UUEncoded Attachments
One of the common uses for UUEncoding is sending files as attachments in emails. You can achieve this by leveraging PowerShell's email capabilities and integrating UUEncoding.
$attachment = "path\to\your\file.txt"
$encodedAttachment = [Convert]::ToBase64String([System.IO.File]::ReadAllBytes($attachment))
# Send email with the encoded attachment here using Send-MailMessage or similar cmdlet
In this code:
- The process of reading and encoding the attachment is the same as before.
- After creating the encoded attachment string, you would typically use a cmdlet like `Send-MailMessage` to send it as part of an email body or as an actual attachment, ensuring the encoded string is correctly handled.
Example 2: Storing UUEncoded Data in a Database
UUEncoding can also be used to store binary data, such as images or documents, within a database. Here's a concise example:
$imagePath = "path\to\your\image.png"
$encodedImage = [Convert]::ToBase64String([System.IO.File]::ReadAllBytes($imagePath))
# Insert into database logic here, using $encodedImage to store
Here:
- The encoding process is identical to previous examples.
- After encoding, you can execute SQL commands or use PowerShell’s database cmdlets to insert this string into a table designed to hold binary data.
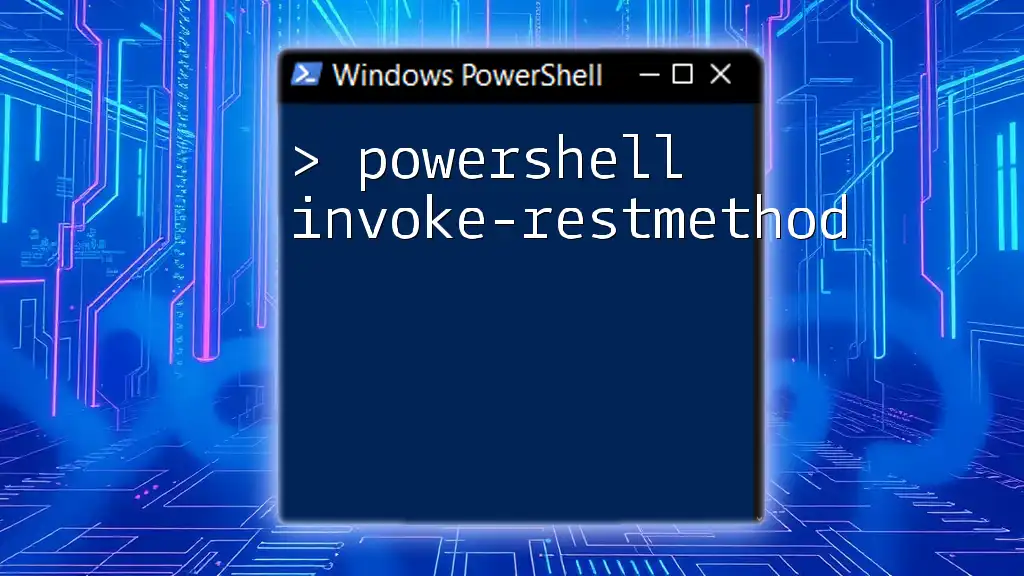
Best Practices and Considerations
Security Implications
While UUEncoding allows for the safe transmission of binary data, you should be aware of potential security risks. Always validate and sanitize any data, particularly if it will be stored or transmitted, to avoid injection attacks or data corruption. Additionally, treat UUEncoded data just like any other binary data; ensure secured channels for transmission and encrypted storage if necessary.
Performance Considerations
When deciding to use UUEncoding (or its equivalent, Base64 encoding), consider the size of the files being handled. Encoding increases the size of the data by approximately 33%, which may impact network performance and storage practices. For large files, consider alternate methods of transfer, such as direct file transfer protocols (FTP) that handle binary data more efficiently.
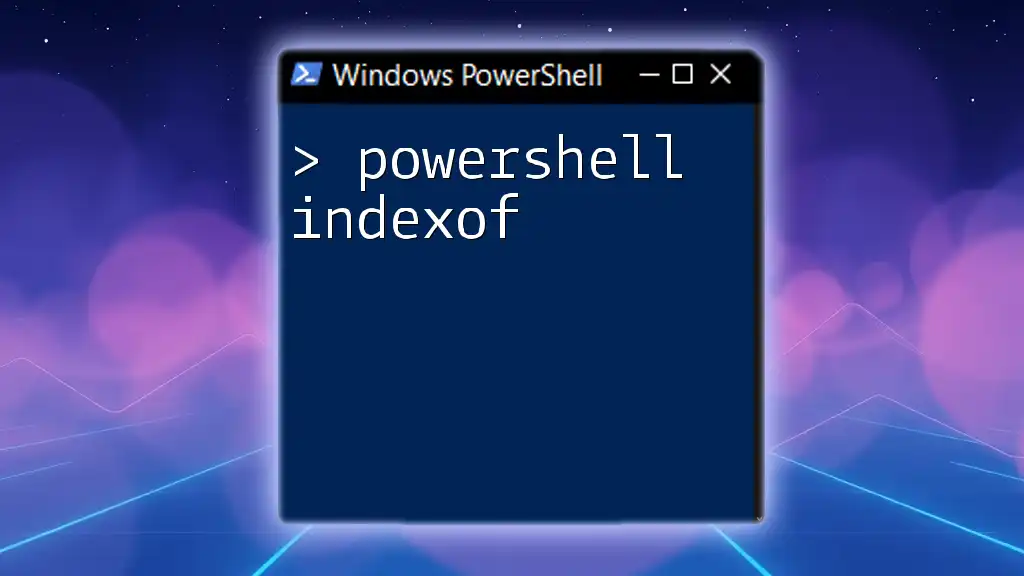
Conclusion
In summary, PowerShell UUEncode provides a flexible framework for encoding and decoding files. Whether you need to send binary data via email or store it securely in a database, understanding how to utilize PowerShell's built-in functions for this purpose can greatly enhance your data management capabilities. By practicing these skills, you can automate tasks and work effectively with various data types in your PowerShell scripts.
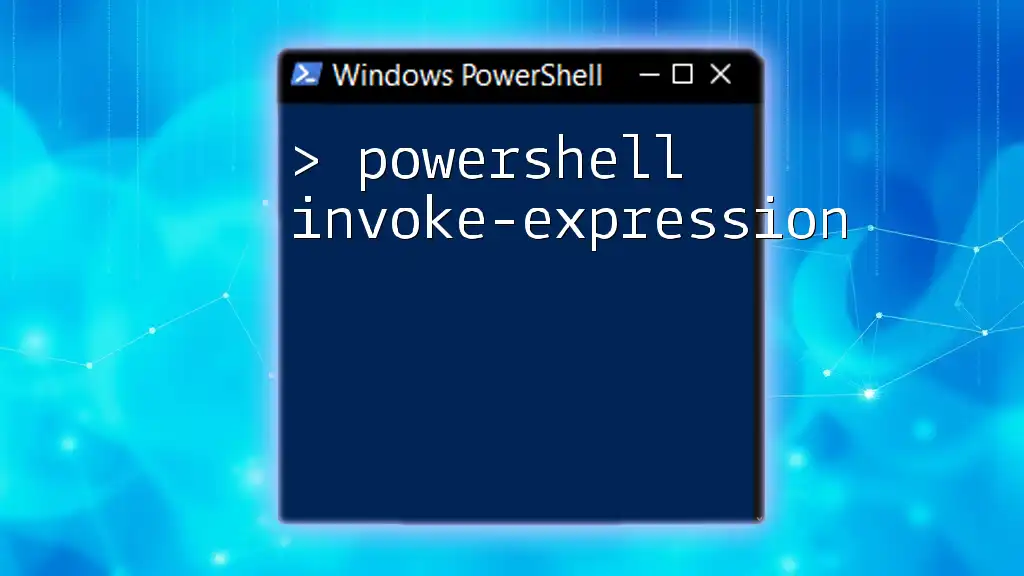
Additional Resources
To further hone your PowerShell skills, consider exploring:
- Online tutorials and courses focused on PowerShell scripting.
- Community forums like Stack Overflow or Microsoft’s Tech Community.
- Documentation and guides available on the official Microsoft PowerShell website.
Embrace the PowerShell community and continue experimenting with different commands and use cases to expand your knowledge and proficiency!