Certainly! Here's a concise explanation along with a code snippet for replacing a line in a file using PowerShell:
To replace a specific line in a file with a new line of text, you can use the following PowerShell command:
(Get-Content "C:\path\to\your\file.txt") | ForEach-Object { $_ -replace 'old line text', 'new line text' } | Set-Content "C:\path\to\your\file.txt"
This command reads the content of the specified file, replaces the old line with the new one, and then writes the updated content back to the file.
Basics of Working with Files in PowerShell
Understanding File Types
In PowerShell, it's crucial to recognize the difference between text and binary files. Text files are structured with readable characters and are usually associated with file extensions like `.txt` or `.csv`. Binary files, on the other hand, consist of data represented in a format that is not human-readable, such as images or application data. Knowing when to use these types of files will help you choose the right approach for manipulation.
Common Commands for File Manipulation
Before diving into replacing a line in a file, let's explore some of the basic commands that PowerShell offers for file manipulation:
-
Get-Content: This cmdlet reads the content of a file and returns it as an array of strings, where each element represents a line in the file.
-
Set-Content: This command writes data to a file, either replacing existing content or creating a new file if one doesn't already exist.
-
Out-File: Useful for redirecting output from any command directly to a file, commonly used in scripting scenarios.
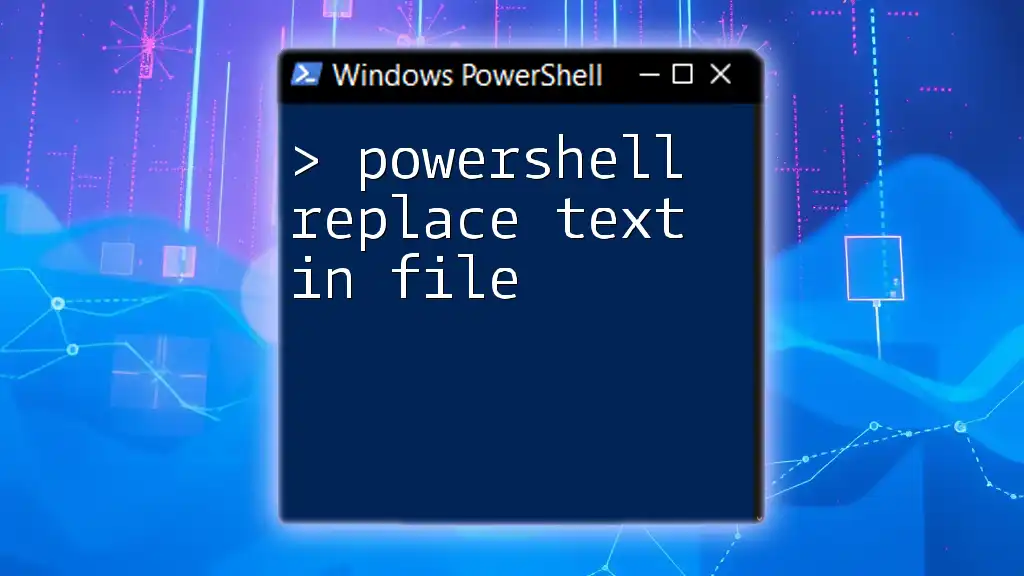
Replacing a Line in a File
Why Replace a Line?
There are numerous scenarios where you might need to replace a line within a file. For example, updating configuration files, modifying log entries, or correcting errors in script files are all tasks that could require line replacement. PowerShell provides powerful commands that can help you perform these actions easily and efficiently.
The Fundamental Approach
The most straightforward way to achieve line replacement involves using both `Get-Content` to read the file and `Set-Content` to write the changes back.
Code Snippet: Basic Line Replacement
Here's a simple code snippet demonstrating how to replace a specific line in a file:
$content = Get-Content -Path "C:\path\to\your\file.txt"
$content[2] = "This is the new line."
Set-Content -Path "C:\path\to\your\file.txt" -Value $content
Explanation of the Code Snippet:
- Get-Content retrieves all lines from `"C:\path\to\your\file.txt"` and stores them in a variable `$content`.
- The line at index `2` (the third line, as indexing starts at zero) is replaced with `"This is the new line."`.
- `Set-Content` then writes the modified content back into the original file, effectively replacing the old line with the new one.
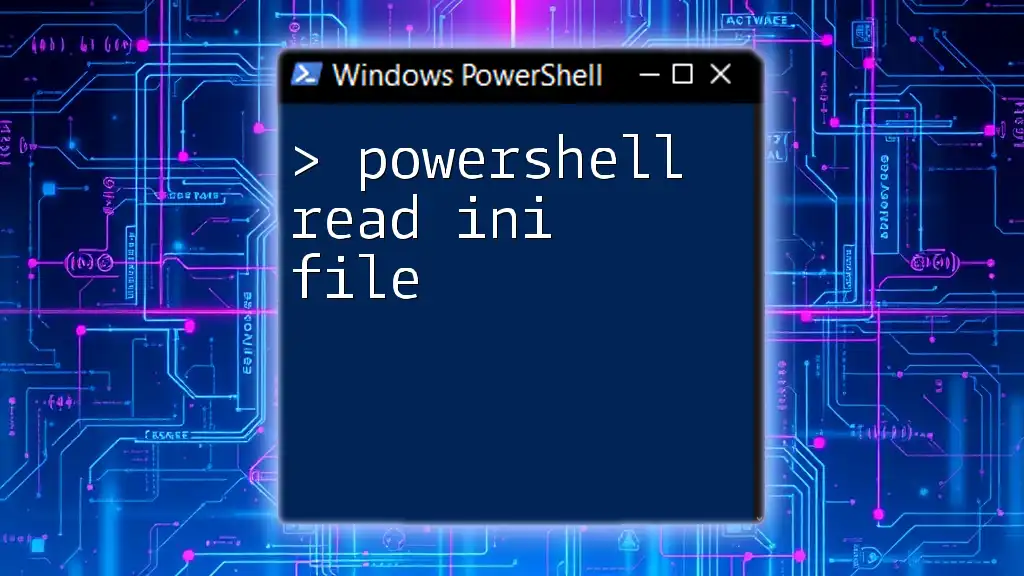
Advanced Techniques for Line Replacement
Using Regular Expressions
Regular expressions (regex) are a powerful tool for pattern matching and can make line replacement more efficient by allowing you to search for complex string patterns.
Example Code Snippet for Regex Replacement
Here’s how you can leverage regex for replacing lines:
(Get-Content "C:\path\to\your\file.txt") -replace 'OldLineContent', 'NewLineContent' | Set-Content "C:\path\to\your\file.txt"
Explanation of the Code Snippet:
In this example, `Get-Content` reads the original file's content, while the `-replace` operator searches for the exact string `'OldLineContent'` and replaces it with `'NewLineContent'`. The modified content is then sent through a pipe to `Set-Content` to overwrite the file.
In-Place Editing with `-Replace`
The `-Replace` operator allows for seamless in-line substitutions which can make scripts cleaner and easier to read.
Example Code Snippet
$filePath = "C:\path\to\your\file.txt"
(Get-Content $filePath) | ForEach-Object { $_ -replace 'Old Text', 'New Text' } | Set-Content $filePath
Step-by-Step Breakdown:
- `Get-Content $filePath` retrieves the content of the specified file.
- The `ForEach-Object` cmdlet iteratively processes each line of content.
- In each iteration, `$_ -replace 'Old Text', 'New Text'` performs the line modification.
- Finally, `Set-Content` saves the updated content back to the original file.
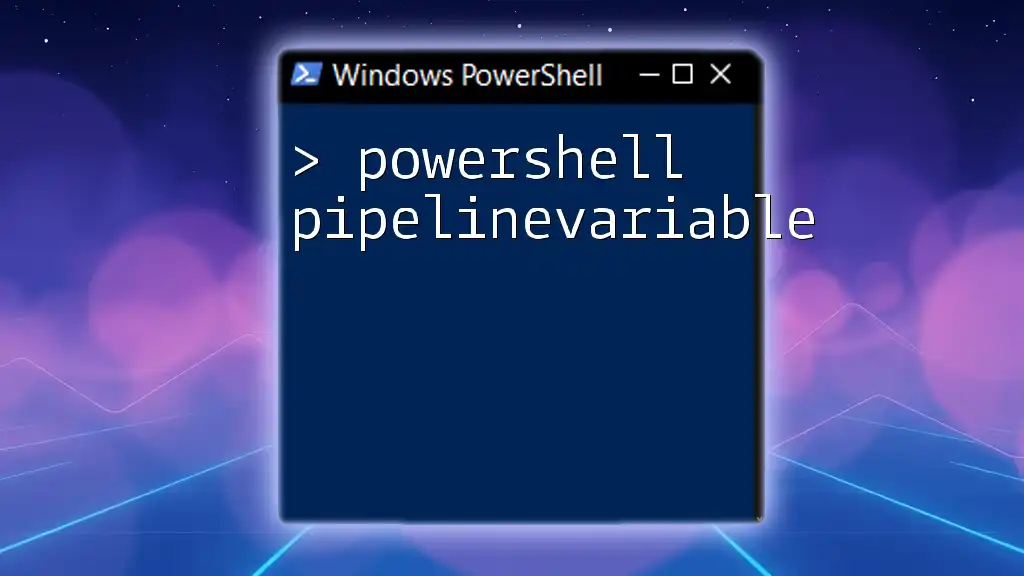
Using PowerShell Functions for Replaces
Creating a Custom Function
Custom functions can enhance reusability and streamline your scripts. Below is a sample function for line replacement.
Code Snippet for Custom Function
function Replace-Line {
param(
[string]$filePath,
[string]$oldText,
[string]$newText
)
(Get-Content $filePath) -replace $oldText, $newText | Set-Content $filePath
}
Explanation of the Function:
- Parameters: The function takes three parameters: `$filePath`, `$oldText`, and `$newText`.
- The function reads the content of the file specified by `$filePath`, replaces occurrences of `$oldText` with `$newText`, and saves the changes back to the same file.
Example Usage of Custom Function
You can call this function as follows:
Replace-Line -filePath "C:\path\to\your\file.txt" -oldText 'Line to Replace' -newText 'New Line Content'
This example demonstrates how to easily replace a line by simply passing the required parameters, making it clear and efficient.
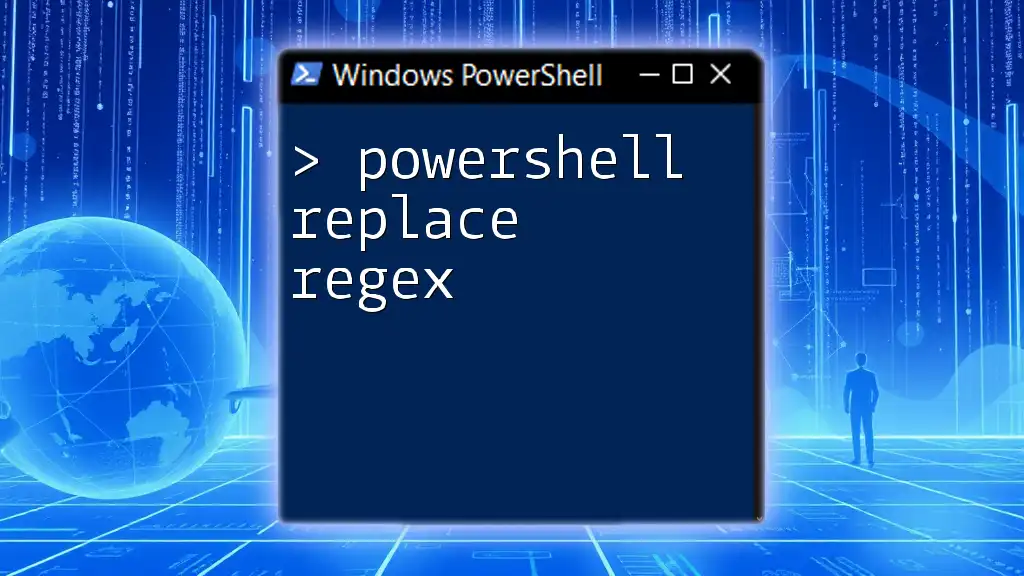
Error Handling and Considerations
Common Errors and Solutions
When working with PowerShell to manipulate files, you might encounter a few common issues:
-
File Not Found: If the file path specified doesn’t exist, PowerShell will throw an error. Ensure that the path is correct or implement checks to confirm the file's existence before running your commands.
-
Access Denied: Issues related to permissions can prevent you from reading or modifying files. Make sure you have the necessary permissions or run PowerShell as an administrator if needed.
Best Practices
-
Always Backup Files: Before executing any line replacement, it’s a good habit to create a backup of existing files. This ensures you can restore the original content if something goes wrong.
-
Test Your Scripts: Run your line-replacing scripts on dummy files to ensure they work as expected. This minimizes the chance of impacting important data.
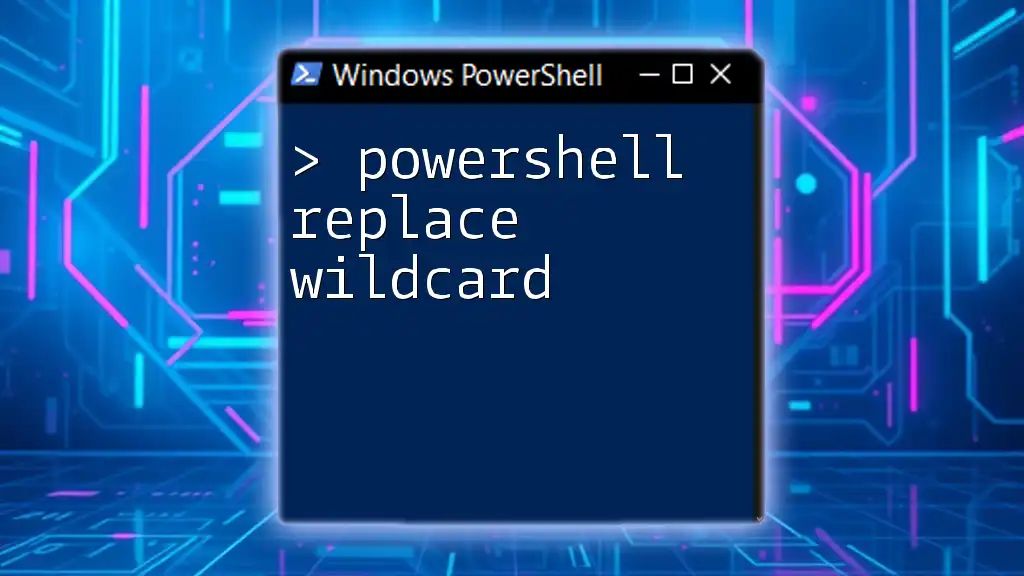
Conclusion
In this article, we covered several methods for using PowerShell to replace lines in a file. By understanding the basic commands and advanced techniques, you can efficiently manipulate file contents to suit your needs. Experiment with these commands and functions to gain deeper insights into PowerShell's capabilities regarding file handling and transformation. The more you practice, the more proficient you'll become in streamlining your file-manipulation tasks.