To list all files in a directory and its subdirectories using PowerShell, you can utilize the `Get-ChildItem` cmdlet with the `-Recurse` parameter.
Here’s the code snippet:
Get-ChildItem -Path "C:\Your\Directory\Path" -Recurse
Make sure to replace `"C:\Your\Directory\Path"` with the actual directory path you want to search.
Understanding the Basics of PowerShell
What is PowerShell?
PowerShell is a powerful scripting language and command-line shell designed specifically for system administration. With its intuitive syntax and rich set of built-in commands, PowerShell allows users to perform a wide range of tasks, from file manipulation to system configuration. Unlike the Command Prompt, which has a limited set of commands, PowerShell operates on a more extensive range of cmdlets that interact with .NET objects, making it much more versatile.
Why List Files in a Directory?
Listing files in a directory and its subdirectories is an integral part of file management. It helps in various scenarios, such as auditing file systems, organizing documents, locating specific files, or preparing for a backup. Understanding how to effectively list files using PowerShell can enhance your productivity and streamline your workflow.
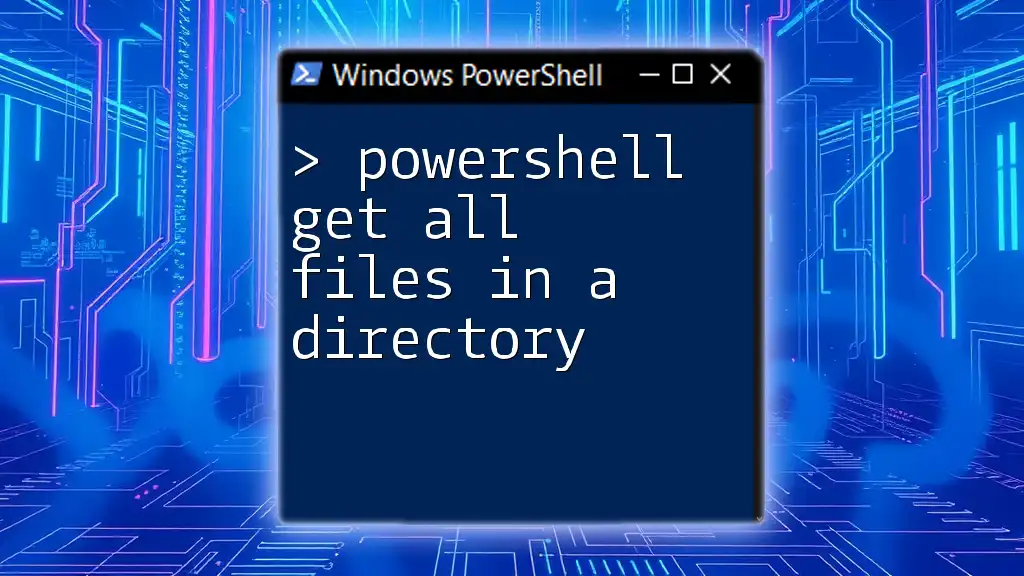
Getting Started with File Listing Commands
Navigating the File System with PowerShell
The foundation of listing files is the `Get-ChildItem` cmdlet. This command retrieves the items (files and directories) in a specified location. It can be thought of as the PowerShell equivalent of traditional directory listing commands.
Basic Syntax of `Get-ChildItem`
The basic syntax of `Get-ChildItem` follows this structure:
Get-ChildItem -Path "<Directory Path>"
You can use parameters such as `-Path`, `-Recurse`, and `-File` for more refined searches. The `-Path` parameter specifies the directory to search, while `-Recurse` allows you to include all subdirectories. The `-File` parameter filters out directories from the results.
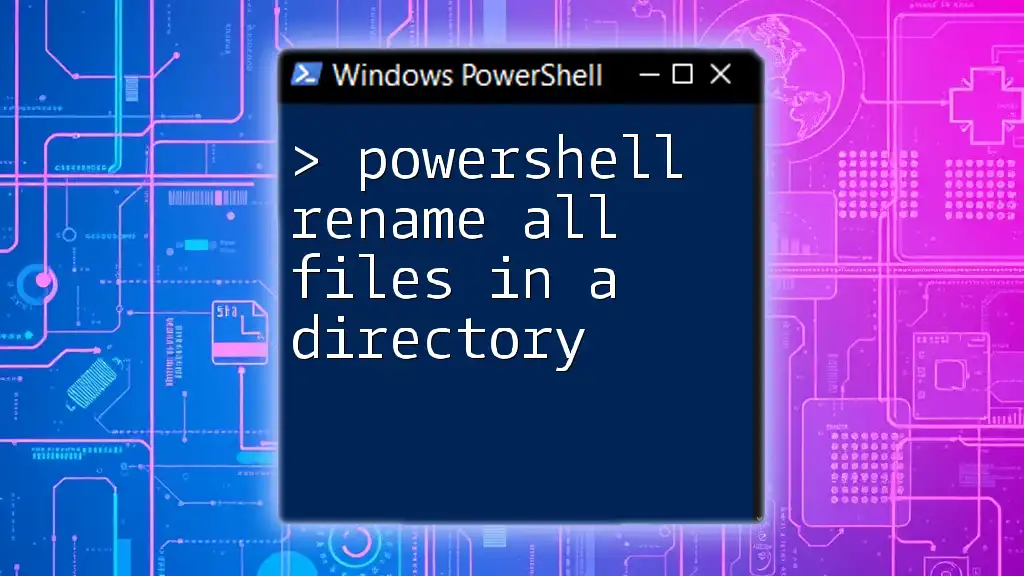
Listing Files in a Single Directory
Simple Examples
To list all files in a specific directory, you can issue the following command:
Get-ChildItem -Path "C:\ExampleDirectory"
This command will display all files and folders located within "C:\ExampleDirectory". The output includes details such as the name, last modified date, and size of each file.
Filtering File Types
Using Wildcards
If you need to list only specific types of files, such as `.txt` files, you can use wildcards:
Get-ChildItem -Path "C:\ExampleDirectory\*.txt"
Wildcards allow for flexible searching by helping you filter file types based on their extensions.
Using `-Filter` Parameter
Another method for filtering is by using the `-Filter` parameter. This provides improved performance, especially when dealing with a significant number of files:
Get-ChildItem -Path "C:\ExampleDirectory" -Filter "*.txt"
This command accomplishes the same as the previous example but may execute faster and be more resource-efficient.
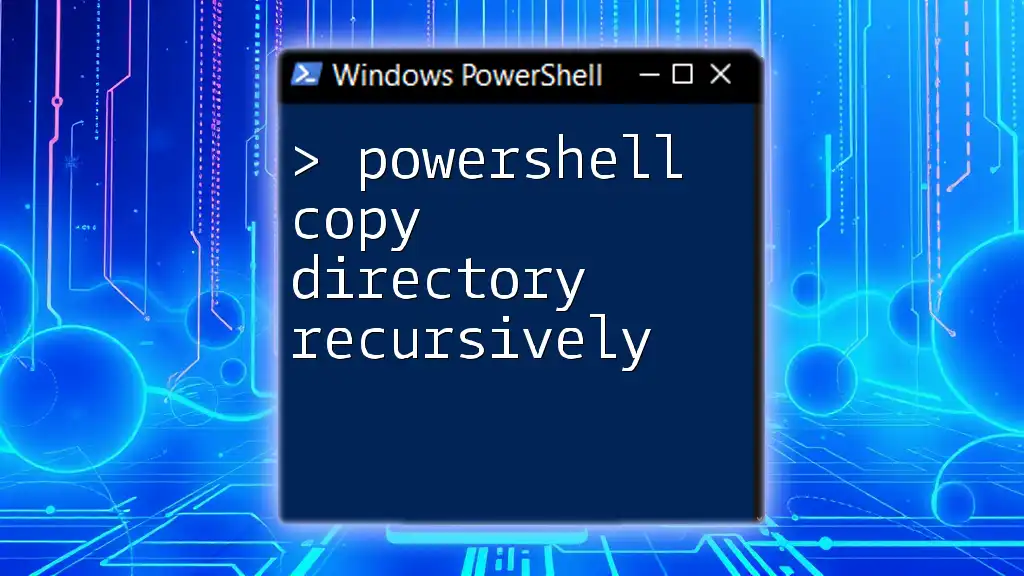
Listing Files in Subdirectories
Enabling Recursive Search
To list files not only in a specified directory but also in all of its subdirectories, you utilize the `-Recurse` parameter:
Get-ChildItem -Path "C:\ExampleDirectory" -Recurse
This command traverses through all subdirectories under "C:\ExampleDirectory", providing a comprehensive view of all contained files.
Advanced Use Cases
Filtering by File Attributes
Sometimes, it’s advantageous to list only files while excluding directories. To accomplish this, you can add the `-File` parameter:
Get-ChildItem -Path "C:\ExampleDirectory" -Recurse -File
This command filters the output to display only file objects, making it easier to focus on relevant items.
Using `Where-Object` for Complex Filtering
For scenarios requiring more complex filtering—such as listing files larger than a specific size—you can use the `Where-Object` cmdlet alongside `Get-ChildItem`:
Get-ChildItem -Path "C:\ExampleDirectory" -Recurse | Where-Object { $_.Length -gt 1MB }
In this example, we retrieve all files in "C:\ExampleDirectory" and its subdirectories, then filter them down to only include those that exceed 1 MB in size.
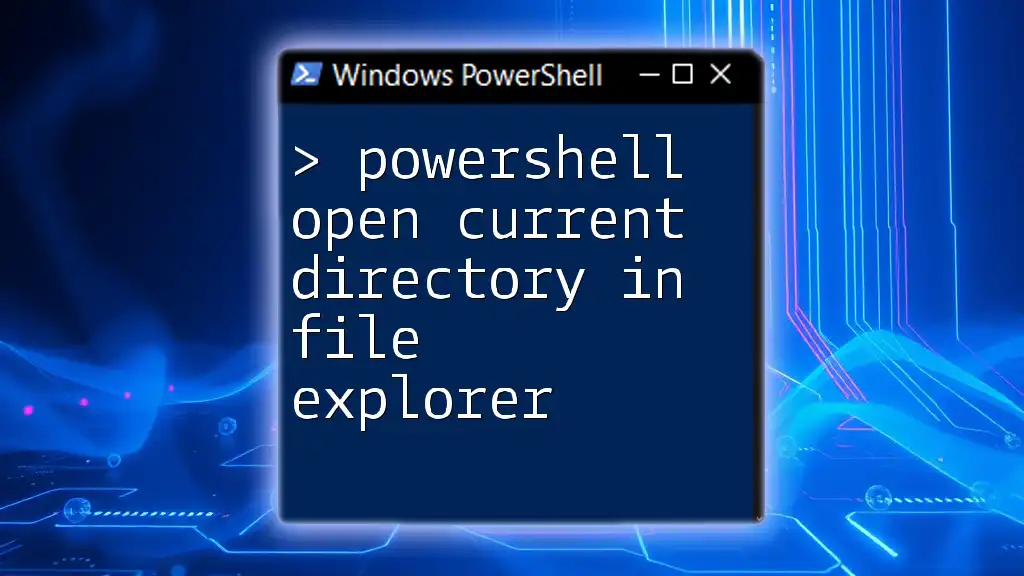
Formatting the Output
Changing the Display Format
You can customize and format the output of your file listings for easier readability by using the `Select-Object` cmdlet. This allows you to select specific properties to display:
Get-ChildItem -Path "C:\ExampleDirectory" -Recurse | Select-Object Name, Length, LastWriteTime
This command presents a clear, concise view containing just the file name, size, and last modified date.
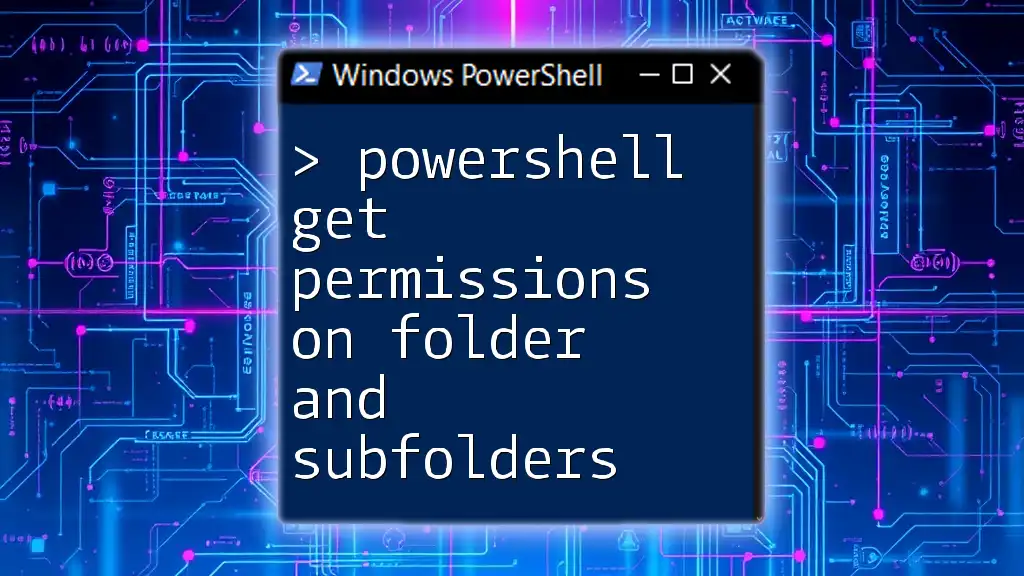
Saving or Exporting the List
Exporting to CSV
If you need to save the list of files for later use or for reporting purposes, exporting the data to a CSV file is simple:
Get-ChildItem -Path "C:\ExampleDirectory" -Recurse | Export-Csv -Path "C:\output.csv" -NoTypeInformation
This will create a CSV file named "output.csv" in the root of your C: drive, containing an organized list of all files.
Generating Plain Text Listings
For straightforward text output, PowerShell allows you to save the file list to a text file effortlessly:
Get-ChildItem -Path "C:\ExampleDirectory" -Recurse | Out-File -FilePath "C:\output.txt"
This command generates "output.txt" in the specified path, containing a plain text listing of files.
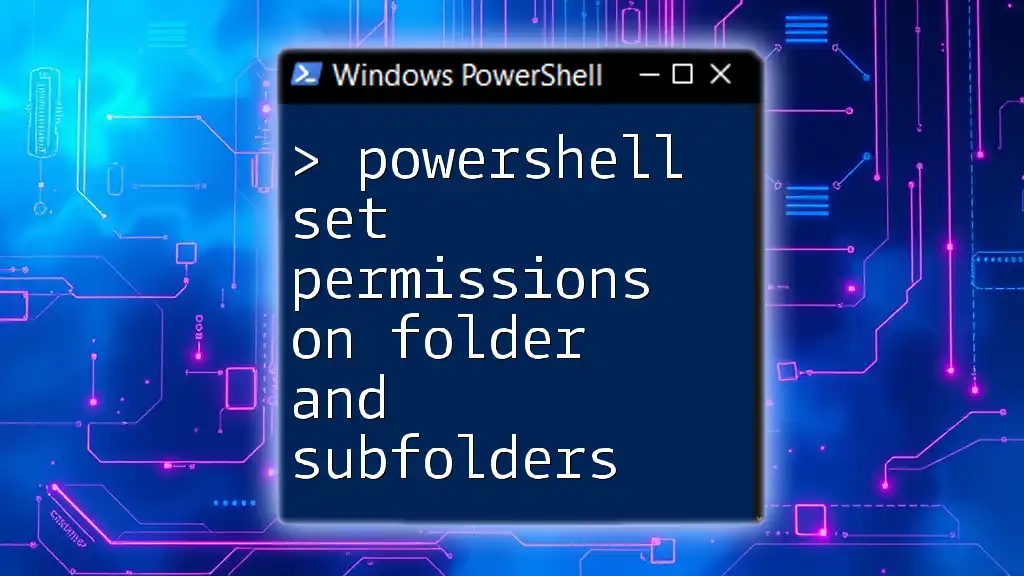
Common Troubleshooting Tips
Handling Permission Issues
When working with PowerShell, you may encounter permission-related errors, especially when querying certain directories. Ensure that you are running PowerShell with elevated privileges by right-clicking the icon and selecting "Run as Administrator." If access is still denied, check the directory's permissions or consult with your system administrator.
Performance Considerations
If you are handling large directories, the performance of your command may slow down. To improve execution time, it can be beneficial to limit the search scope using the `-Path` to target specific directories or filter outputs as needed.
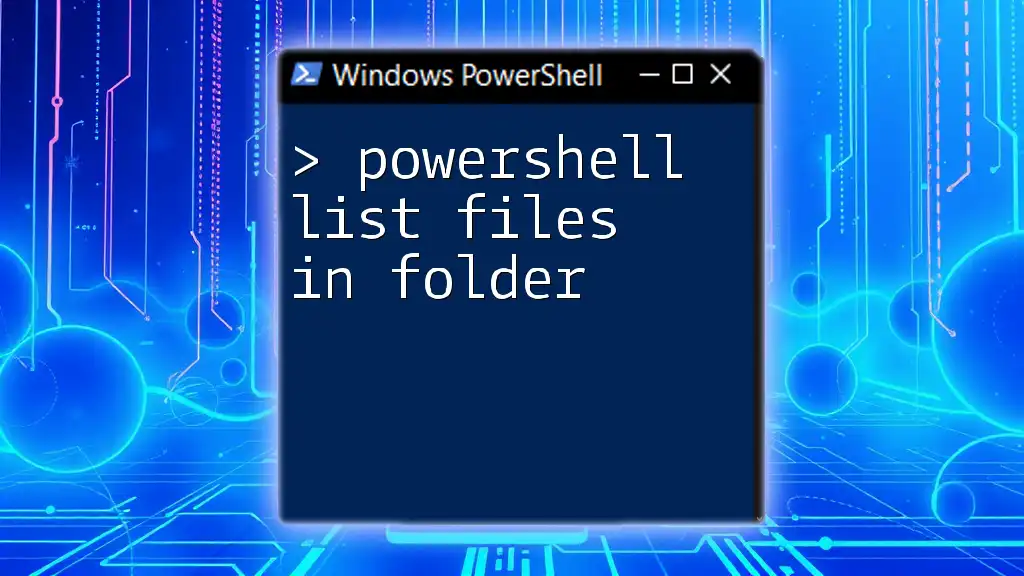
Conclusion
By mastering the PowerShell commands to list all files in a directory and its subdirectories, you empower yourself with essential skills for more efficient file management. Practice these commands regularly, experiment with the parameters, and explore the rich functionality of PowerShell to better suit your administrative needs.
This knowledge will undoubtedly enhance your productivity and give you a competitive edge in managing your file systems. Take the time to test these commands in various scenarios, and you will find that PowerShell can significantly simplify your tasks.
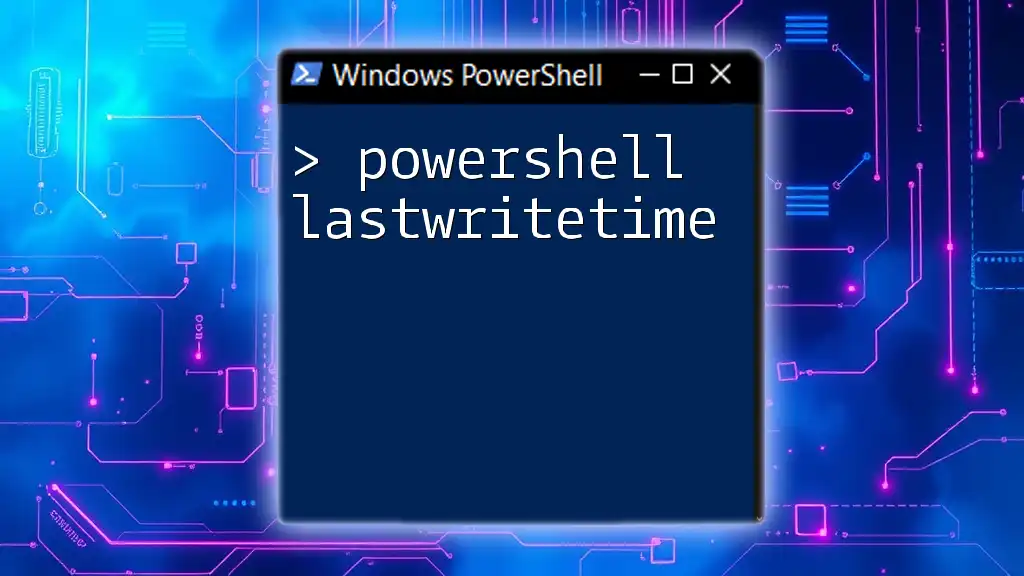
Call to Action
If you enjoyed this guide and seek to delve deeper into the world of PowerShell, consider subscribing to our newsletter for more tutorials and tips, and share your experiences or questions in the comments section below!
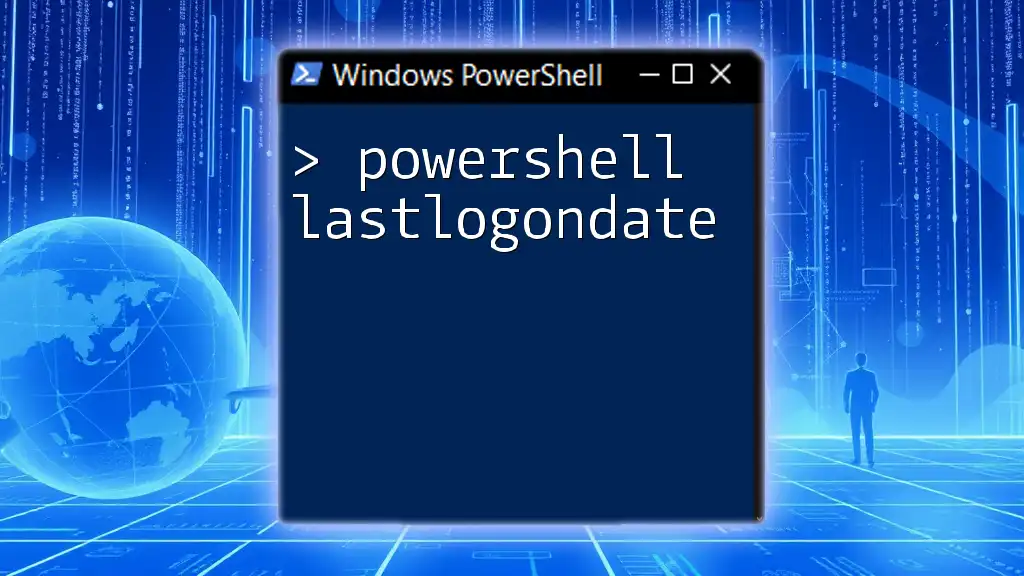
Additional Resources
To further your learning, check out these resources:
- PowerShell documentation on Microsoft’s website
- Books on PowerShell scripting and best practices
- Online forums and communities dedicated to PowerShell enthusiasts