To set permissions on a folder and its subfolders in PowerShell, you can use the `icacls` command which allows you to grant specific rights to a user or group, as shown in the code snippet below.
icacls "C:\Path\To\Your\Folder" /grant UserOrGroup:(OI)(CI)F /T
In this command, replace `"C:\Path\To\Your\Folder"` with the path to your folder, and `UserOrGroup` with the username or group name you want to grant permissions to; `(OI)(CI)F` specifies the permissions given to all child objects, and `/T` applies the changes to all files and subfolders.
Understanding Directory Permissions in PowerShell
What Are Directory Permissions?
Directory permissions are crucial in maintaining the security and integrity of data stored within folders on your system. By controlling who can access, modify, or execute files and folders, you ensure that only authorized users have the necessary rights to perform specific actions.
Default Permissions in Windows
When a folder is created in Windows, it is assigned a set of default permissions. Typically, these include:
- Full Control: The ability to read, write, and execute files, as well as change permissions.
- Modify: Permission to read and write files, delete them, and change their content.
- Read & Execute: Users can view and execute files.
- Read: Users can view the contents but cannot modify them.
- Write: Users can add files or data to the folder.
Understanding these default permissions is essential for both system administrators and users who want to utilize PowerShell effectively for setting permissions on folders and subfolders.

PowerShell Cmdlets for Setting Permissions
Introduction to PowerShell Cmdlets
PowerShell commands, known as cmdlets, form the backbone of automation in Windows administrative tasks. For managing permissions specifically, there are two key cmdlets to be aware of: `Get-Acl` and `Set-Acl`.
Key Cmdlets for Setting Permissions
- `Get-Acl`: This cmdlet retrieves the Access Control List (ACL) for a specified file or folder, allowing you to view existing permissions.
- `Set-Acl`: Used to modify the ACL of a file or folder, this cmdlet allows you to set new permissions based on the defined rules.
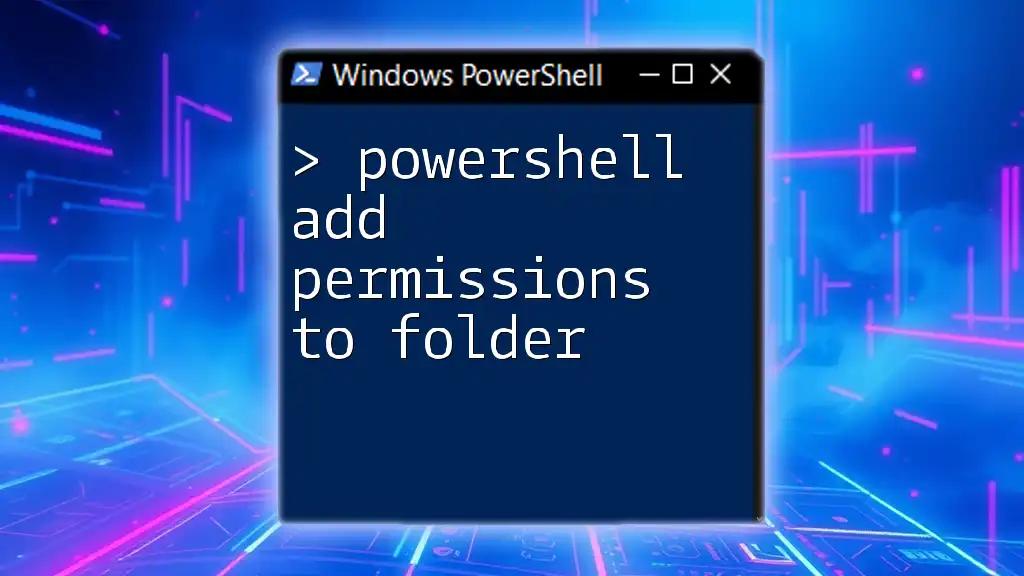
Setting Permissions on a Folder Using PowerShell
Basic Syntax for Setting Directory Permissions
To set permissions, you'll often use the following syntax:
Set-Acl -Path "FolderPath" -AclObject "AclObject"
Example of Setting Permissions on a Single Folder
Suppose you have a folder at C:\ExampleFolder, and you want to grant Full Control permissions to a specific user, you would employ the following code:
$acl = Get-Acl "C:\ExampleFolder"
$permission = "Domain\User","FullControl","Allow"
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule $permission
$acl.SetAccessRule($rule)
Set-Acl "C:\ExampleFolder" $acl
Here is what each line of the script does:
- Get-Acl "C:\ExampleFolder": Retrieves the current permissions set on the folder.
- New-Object System.Security.AccessControl.FileSystemAccessRule: Creates a new rule specifying the permissions to apply.
- SetAccessRule($rule): Applies the created rule to the folder's ACL.
- Set-Acl "C:\ExampleFolder" $acl: Finally, this command writes the updated permissions back to the folder.
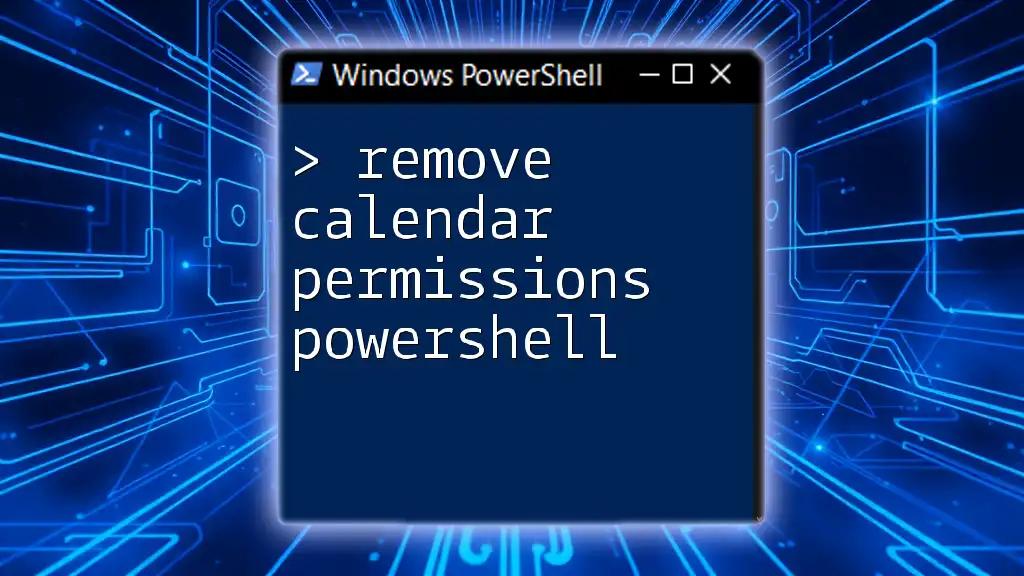
Setting Permissions on Subfolders
How to Apply Permissions to Subfolders
To apply permissions recursively across a folder and all its subfolders, you will often use the `-Recurse` parameter in your commands.
Example of Setting Permissions on Folder and Subfolders
Using the same C:\ExampleFolder, here’s how to apply permissions to both the folder and its subfolders:
$baseFolder = "C:\ExampleFolder"
$acl = Get-Acl $baseFolder
$permission = "Domain\User","FullControl","Allow"
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule $permission
# Set permissions on the base folder
$acl.SetAccessRule($rule)
Set-Acl $baseFolder $acl
# Apply to all subfolders
Get-ChildItem $baseFolder -Recurse | ForEach-Object {
$subAcl = Get-Acl $_.FullName
$subAcl.SetAccessRule($rule)
Set-Acl $_.FullName $subAcl
}
This script does the following:
- Retrieves the ACL of the base folder.
- Creates a new access rule granting Full Control to the specified user.
- Applies this rule to the base folder and then iterates through each subfolder, updating their ACLs accordingly.

Checking Current Permissions
How to View Folder Permissions
To see what permissions are currently set on a folder, you can use the `Get-Acl` cmdlet followed by formatting the output for clarity:
Get-Acl "C:\ExampleFolder" | Format-List
This command will display a detailed listing of all permissions associated with the specified folder, enabling you to verify that the changes have been applied successfully.
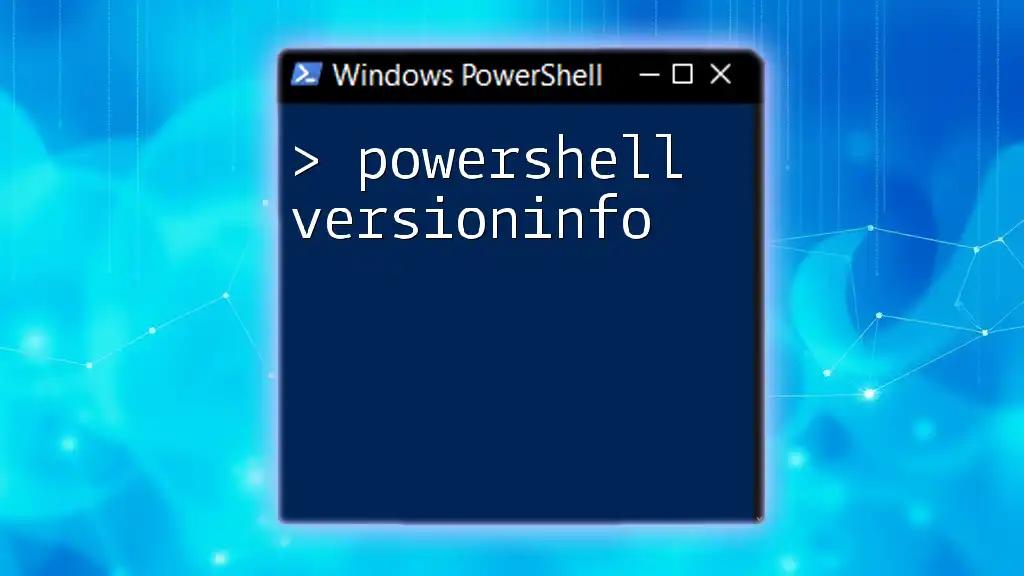
Common Challenges and Troubleshooting
Common Permission Issues
When working with permissions, you may encounter various challenges, such as:
- Insufficient privileges to change permissions, often due to lack of administrative rights.
- Conflicting permissions when multiple users or groups are involved.
- Errors in the script due to incorrect paths or user names.
Troubleshooting Tips
To resolve these common issues:
- Always run PowerShell as an administrator when modifying permissions.
- Use `Get-Acl` to inspect the existing permissions before making changes to prevent conflicts.
- Double-check the paths and user identifiers to ensure they are correct.

Conclusion
In summary, setting permissions on folders and subfolders using PowerShell is a straightforward yet powerful way to manage access and security on your Windows system. By understanding the cmdlets involved and practicing the examples provided, you can ensure that you maintain robust control over your file systems, securing your data from unauthorized access.

Additional Resources
For further reading, consider exploring:
- The official PowerShell documentation.
- Community forums and blogs that cover advanced PowerShell techniques.
- Video tutorials that provide visual demonstrations of permission management.
Engaging with these resources will enhance your understanding and keep you updated with best practices in PowerShell.