To add permissions to a folder using PowerShell, you can use the `Add-NTFSAccess` command from the NTFSSecurity module, which allows you to specify the user and the permissions you want to grant. Here’s a code snippet to demonstrate this:
Add-NTFSAccess -Path "C:\YourFolder" -Account "DOMAIN\User" -AccessRights Modify
Understanding File System Permissions
What Are Permissions?
Permissions are the rules that determine what users or groups can do with files and folders in a file system. These permissions define the level of access, including the ability to read, write, modify, or have full control over the contents of a folder. It's crucial to understand these permissions to ensure that your data remains secure and accessible only to the appropriate users.
Why Permissions Matter
Properly managing folder permissions is essential for maintaining a secure environment. Incorrectly set permissions can lead to unauthorized data access or data loss. Without adequate controls, sensitive information may be exposed or inadvertently modified by unauthorized users. By effectively managing permissions, you mitigate security risks and bolster your system’s integrity.

PowerShell Basics
What is PowerShell?
PowerShell is Microsoft's task automation framework that combines a command-line shell with an associated scripting language. It allows users to automate tasks and manage configurations across both local and remote systems. With PowerShell, you can manipulate and manage permissions seamlessly, making it an invaluable tool for system administrators.
Key Concepts to Know
Familiarity with key PowerShell concepts will greatly enhance your ability to use it effectively. PowerShell utilizes cmdlets for performing specific operations, and each cmdlet follows a standardized naming convention of Verb-Noun (e.g., `Get-ACL`). Understanding the pipeline is also essential, as it allows you to pass data from one cmdlet to another for further processing.

Adding Permissions to a Folder in PowerShell
Overview of the Process
Adding permissions to a folder involves modifying the folder’s Access Control List (ACL), which defines who can access the folder and what actions they can perform. This process can involve several scenarios, such as granting access to individual users or entire groups.
Understanding the ACL (Access Control List)
What is an ACL?
An ACL is a set of rules that grant or deny permissions to users or groups regarding a specific resource, such as a folder. Each entry in an ACL specifies a security identifier (SID) along with the allowed or denied permissions.
Navigating the ACL with PowerShell
To view the current permissions on a folder, you can use the `Get-Acl` cmdlet. For example:
Get-Acl "C:\Path\To\Your\Folder"
This command retrieves and displays the ACL for the specified folder, allowing you to understand existing permissions before making any changes.
The Set-Acl Cmdlet
Syntax and Usage
The `Set-Acl` cmdlet is used to modify the ACLs of specified files and folders. The basic syntax is:
Set-Acl -Path "PathToFolder" -AclObject "AccessControlObject"
This command allows you to define the modified ACL that will be applied to the folder.
Adding Permissions with PowerShell
Using `Add-LocalGroupMember`
To grant permissions to a local user or group, the `Add-LocalGroupMember` cmdlet can be employed. This is particularly useful when adding a user to a specific group that already has the required permissions. For example:
Add-LocalGroupMember -Group "Administrators" -Member "UserName"
This command grants the specified user administrative privileges on the local machine.
Modifying ACLs Directly
You can also modify ACLs directly for more granular control. Here’s how to do that:
-
First, retrieve the current ACL for the folder:
$acl = Get-Acl "C:\Path\To\Your\Folder"
-
Next, define the permission you want to add:
$permission = "Domain\User","FullControl","Allow"
-
Create a new access rule and add it to the ACL:
$accessRule = New-Object System.Security.AccessControl.FileSystemAccessRule $permission $acl.AddAccessRule($accessRule)
-
Finally, apply the modified ACL back to the folder:
Set-Acl "C:\Path\To\Your\Folder" $acl
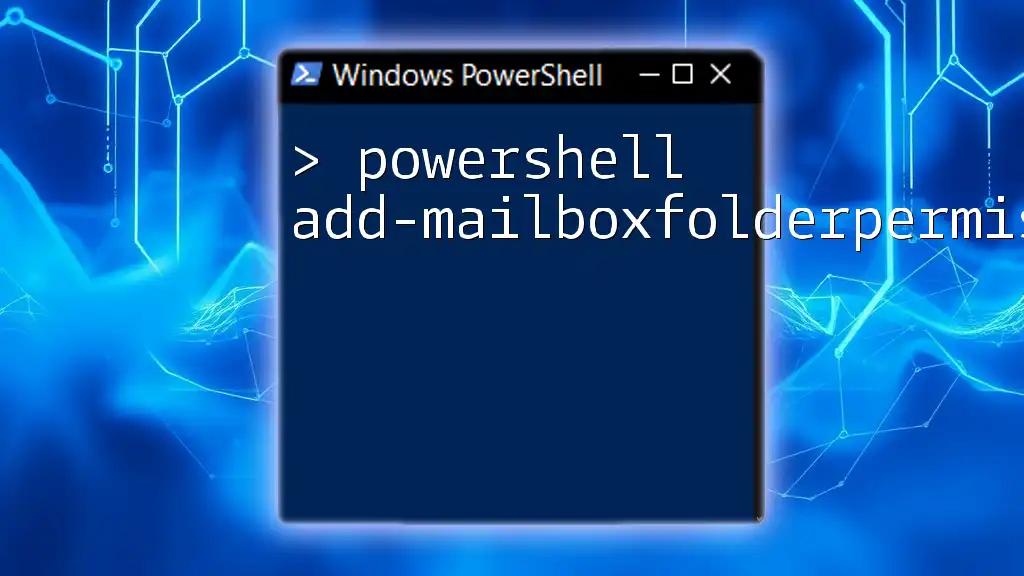
Common Scenarios
Granting Access to Individual Users
To grant specific permissions to an individual user, you can do the following:
$folderPath = "C:\SampleFolder"
$user = "UserName"
$acl = Get-Acl $folderPath
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule($user, "Modify", "Allow")
$acl.SetAccessRule($rule)
Set-Acl $folderPath $acl
In this example, the user is granted the ability to modify the contents of `SampleFolder`.
Granting Access to a Group
You can also easily grant permissions to an entire group using a similar approach:
$group = "Domain\GroupName"
$acl = Get-Acl $folderPath
$rule = New-Object System.Security.AccessControl.FileSystemAccessRule($group, "ReadAndExecute", "Allow")
$acl.SetAccessRule($rule)
Set-Acl $folderPath $acl
This grants read and execute permissions to all members of the specified group.
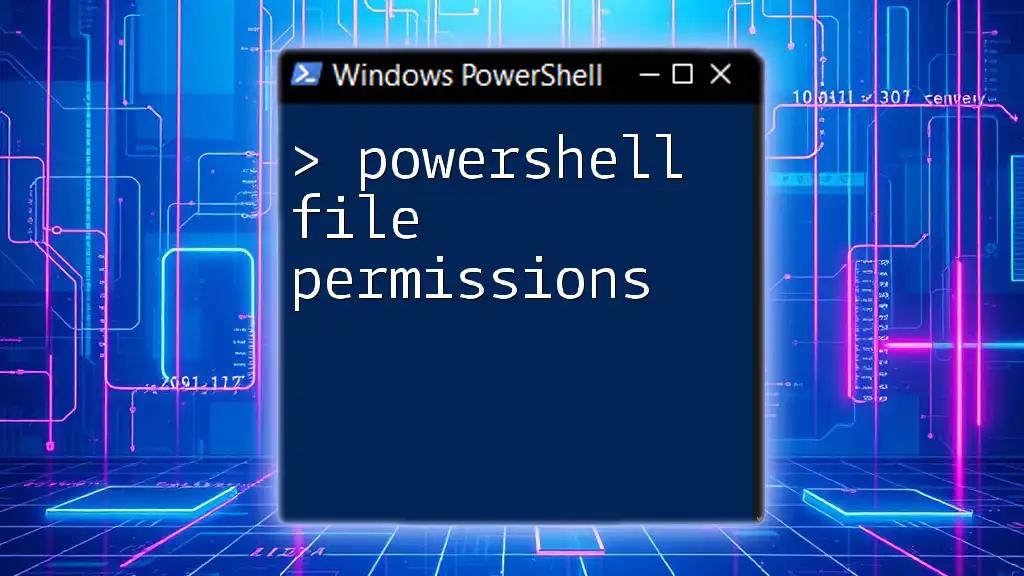
Best Practices for Managing Permissions
Regular Audits
It's essential to regularly audit folder permissions to ensure that they align with your organization's security policies. You can use PowerShell to generate reports, helping identify any unauthorized changes or access levels that need adjustment.
Documenting Your Changes
Keeping a log of permissions changes is critical. Record who was granted access and when modifications were made. This practice not only aids in tracking but also helps in troubleshooting any permission-related issues in the future.
Understanding Inheritance
What is Inheritance?
Folder permissions can often propagate from parent folders, meaning changes made to a parent directory's permissions can affect all subfolders and files. Understanding how this inheritance works is crucial for efficient permission management.
Managing Inheritance with PowerShell
If you need to stop a folder from inheriting permissions from its parent, you can do so with the following commands:
$acl = Get-Acl "C:\FolderWithInheritance"
$acl.SetAccessRuleProtection($true, $false)
Set-Acl "C:\FolderWithInheritance" $acl
This command effectively breaks the inheritance chain, allowing you to set unique permissions for the specified folder.
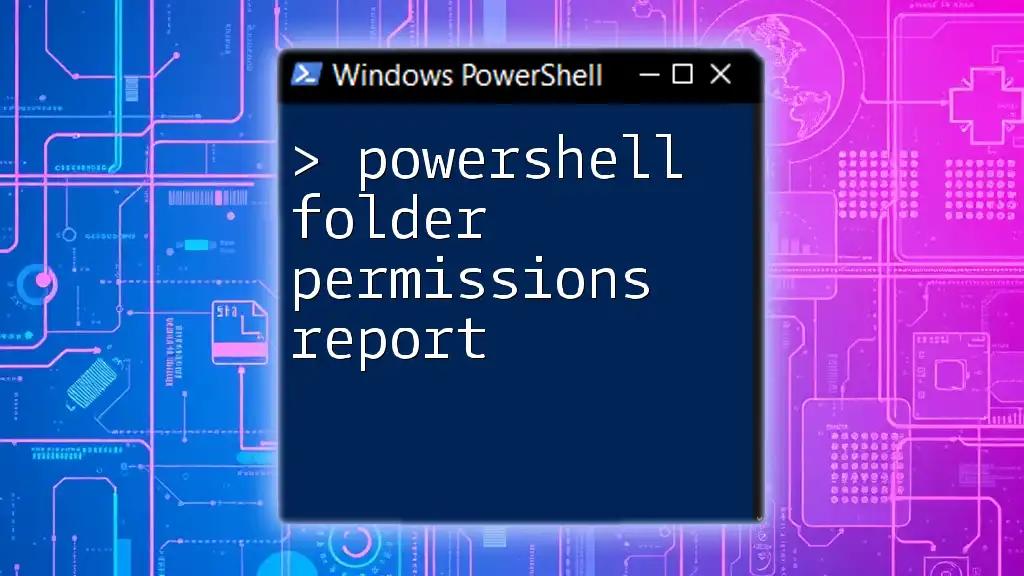
Troubleshooting Common Permissions Issues
Permission Denied Errors
If you encounter "permission denied" errors while trying to access a folder, it usually indicates insufficient permissions on the folder for your user account. You can check the current permissions with:
Get-Acl "C:\YourFolder" | Format-List
This output will help you diagnose the access issues.
Verifying Changes
After making changes to folder permissions, it’s essential to verify that these changes were applied correctly. The following command can confirm the current ACL:
Get-Acl "C:\YourFolder" | Format-List
This command displays all current access rules, ensuring your modifications have been successful.
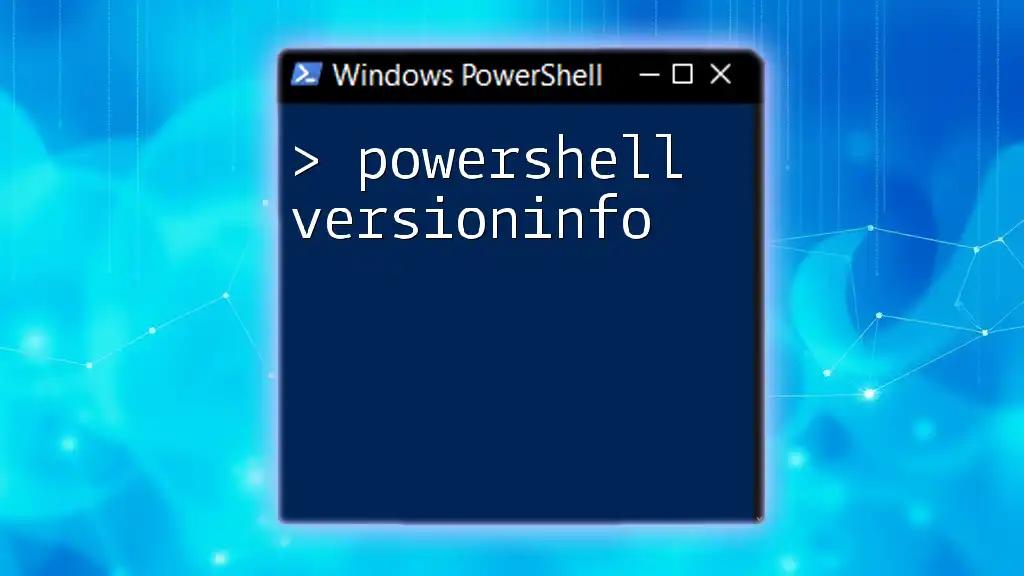
Conclusion
In conclusion, understanding how to effectively add permissions to a folder using PowerShell is vital for any system administrator. Proper folder permissions not only protect sensitive data but also help maintain a secure computing environment. By practicing the techniques outlined in this guide, you'll be well-equipped to manage permissions efficiently and safeguard your organizations' information.