In PowerShell, you can simultaneously output text to both a file and the console by using the `Tee-Object` cmdlet.
Here's a code snippet demonstrating this:
"Hello, World!" | Tee-Object -FilePath 'output.txt' | Write-Host
The Basics of Output in PowerShell
Understanding Output Types
In PowerShell, commands generate various types of output, including simple text and structured objects. Understanding these types is essential for managing and utilizing output effectively. When you execute a command, you might receive direct text responses, such as listing processes or services, as well as complex objects containing multiple properties and methods.
Example: Displaying Simple Text and Structured Objects
Write-Host "This is a simple text output."
Get-Process
In this example, the `Write-Host` command directly outputs text to the console, while the `Get-Process` command returns a collection of process objects displaying critical information such as `ProcessName` and `Id`.
The Default Output System
PowerShell follows a default output system where the results of commands are displayed directly in the console. When a command is executed without any redirection, PowerShell sends the output to the standard output stream, which is typically the console window.
Example: Running `Get-Process` and Observing Console Output
Executing the following:
Get-Process
By default, this command will list all running processes in the console, showcasing the process names and their respective IDs.
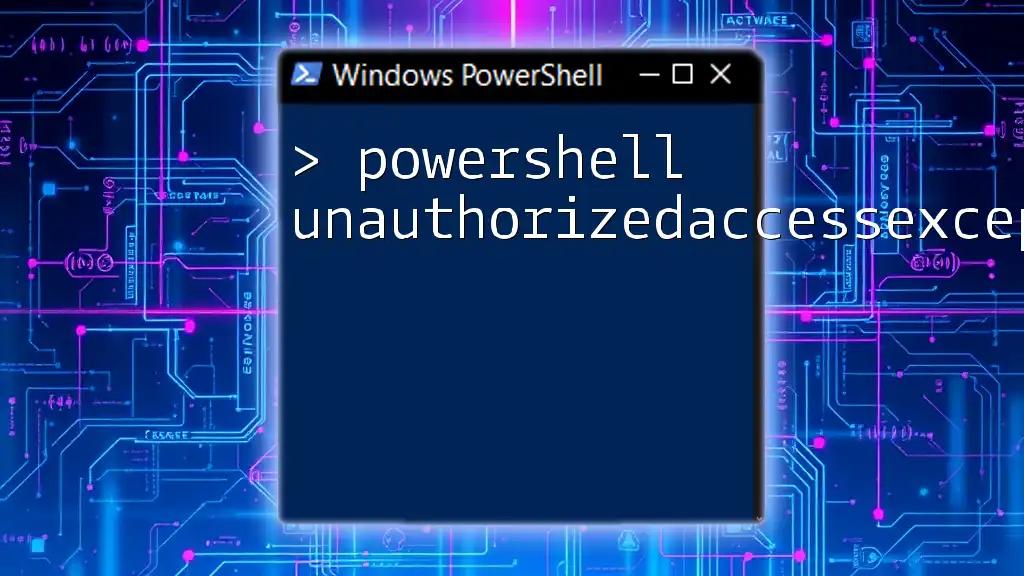
Output to Console
Standard Output Streams
PowerShell uses different output streams, allowing you to manage standard output and error outputs separately. The default output stream is used for typical success messages, but understanding the error output stream can help you catch and manage errors efficiently.
Example: Demonstrating Standard Output with a Simple Command
Get-Service
This command displays all the services running on your system, illustrating PowerShell's ability to generate visible output.
In addition, differentiating between `Write-Host` and `Write-Output` is crucial:
- `Write-Host`: Outputs directly to the console, often used for informational messages.
- `Write-Output`: Sends output to the pipeline, allowing further commands or redirection.
Manipulating Console Output
Manipulating the output for improved readability is often necessary. PowerShell provides formatting cmdlets like `Format-Table` and `Format-List`, which help structure the output visually.
Example: Formatting Output with `Format-Table` and `Format-List`
Get-Process | Format-Table -Property Name, Id, CPU
Using `Format-Table`, you can display only specific properties, such as the process name, ID, and CPU usage, in a neat table format.
Additionally, using `Write-Host` allows you to customize your console's appearance with colors and styles.
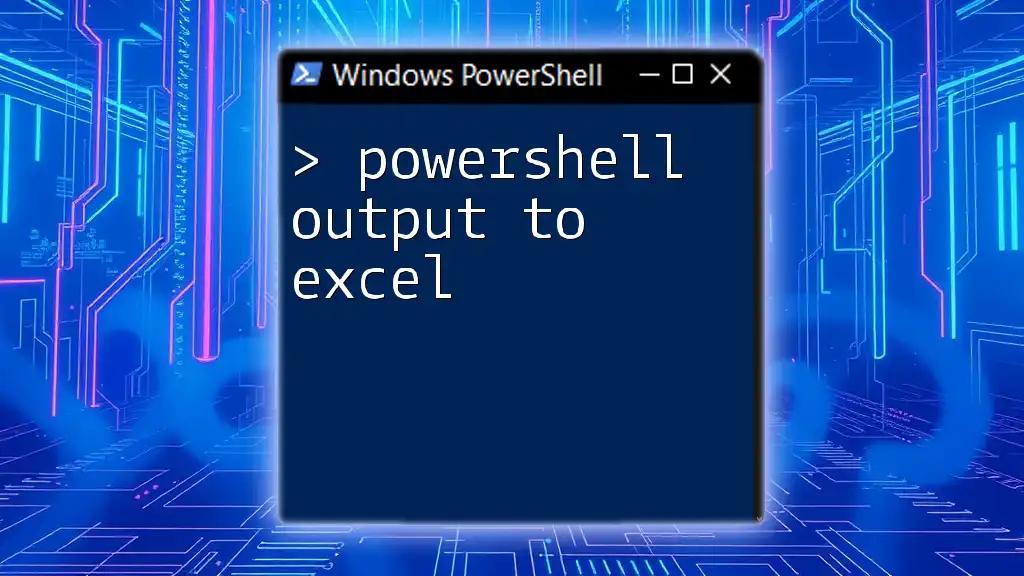
Output to File
Redirecting Output to a File
PowerShell makes it easy to store outputs in files using redirection operators. The `>` operator creates a new file or overwrites an existing file with the output. Conversely, `>>` appends the output to the end of the existing file.
Example: Saving Command Output to a Text File
Get-Process > processes.txt
This command retrieves the list of processes and saves it directly to a file named `processes.txt`, ensuring you have a persistent record of the current system's processes.
Outputting Different Data Formats
When outputting data to files, the format matters significantly. PowerShell allows saving data in various formats, including plain text and CSV files.
Example: Using `Export-Csv` for Structured Data
Get-Service | Export-Csv -Path services.csv -NoTypeInformation
In this example, the command gathers information about services and saves it to a CSV file, which can be easily opened in applications like Microsoft Excel for further analysis.
Appending to Files
Utilizing the `>>` operator is an effective way to preserve previous outputs while adding new data. This method is beneficial when logging ongoing processes or events.
Example: Using the `>>` Operator to Add Additional Entries
Get-EventLog -LogName Application >> application_log.txt
This will append new entries from the Application event log directly into `application_log.txt`, maintaining a comprehensive record.
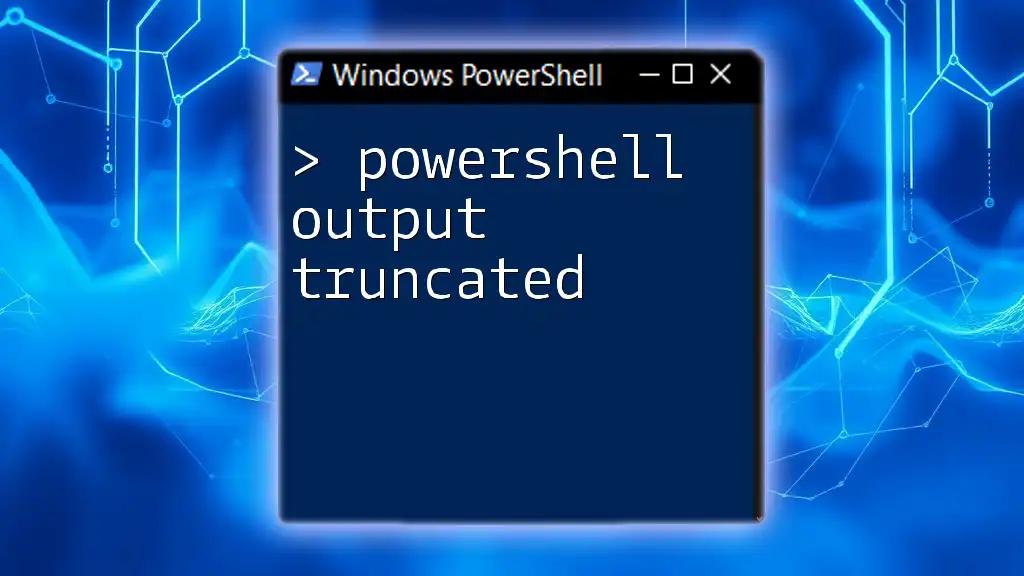
Combining Output to File and Console
Using Tee-Object
A powerful feature in PowerShell is `Tee-Object`, which enables you to send output to both a file and the console simultaneously. This functionality is particularly useful for monitoring tasks while retaining logs.
Example: Outputting to Both a File and the Console Simultaneously
Get-Process | Tee-Object -FilePath processes.txt
In this case, the command retrieves the process list and displays it on the console while also saving it to `processes.txt` for future reference.
Real-World Scenario
Imagine a situation where you aim to monitor how a script performs over time. By using `Tee-Object`, you can track the activities on your console while logging everything for detailed analysis later.
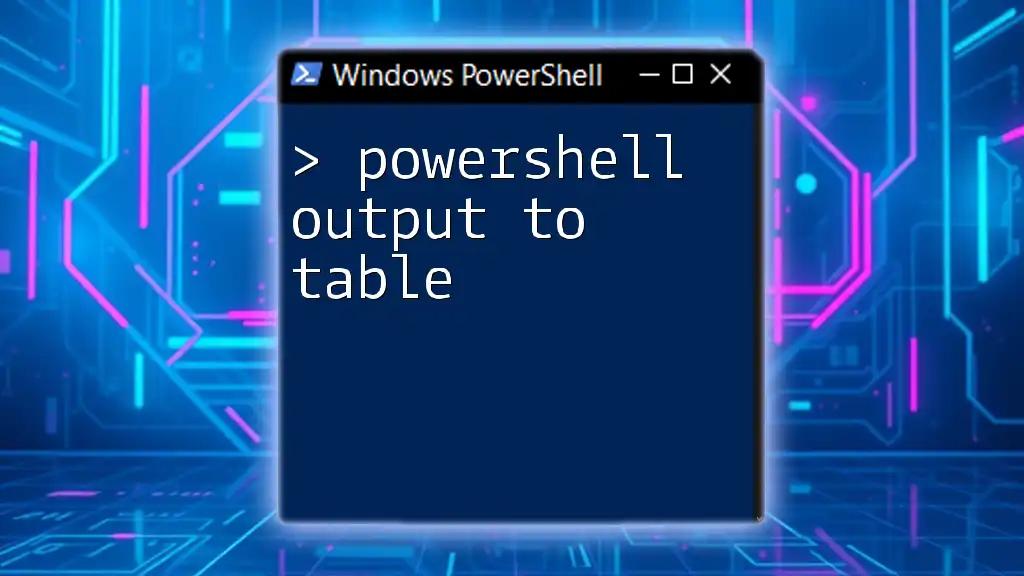
Handling Errors in Output
Redirecting Errors
Effectively managing errors is crucial for robust scripting. PowerShell allows you to redirect error outputs to a file using `2>`. This means that if a command fails, you'll have a record of the error without cluttering the console.
Example: Redirecting Error Output to a File
Get-Process -Name NonExistentProcess 2> errors.txt
This executes a command that attempts to retrieve a non-existent process and sends any error messages to `errors.txt`, maintaining a clean console output.
Viewing Errors in Console
Sometimes, you'd want to see error messages while still logging them. You can achieve this by combining error redirection with normal output redirection to separate files for better organization.
Example: Combining Standard Output and Error Output in One Command
Get-Process -Name NonExistentProcess *> output.txt
This command forwards both standard and error outputs to the same file but is best tailored for advanced users who require a complete view of their command's performance.
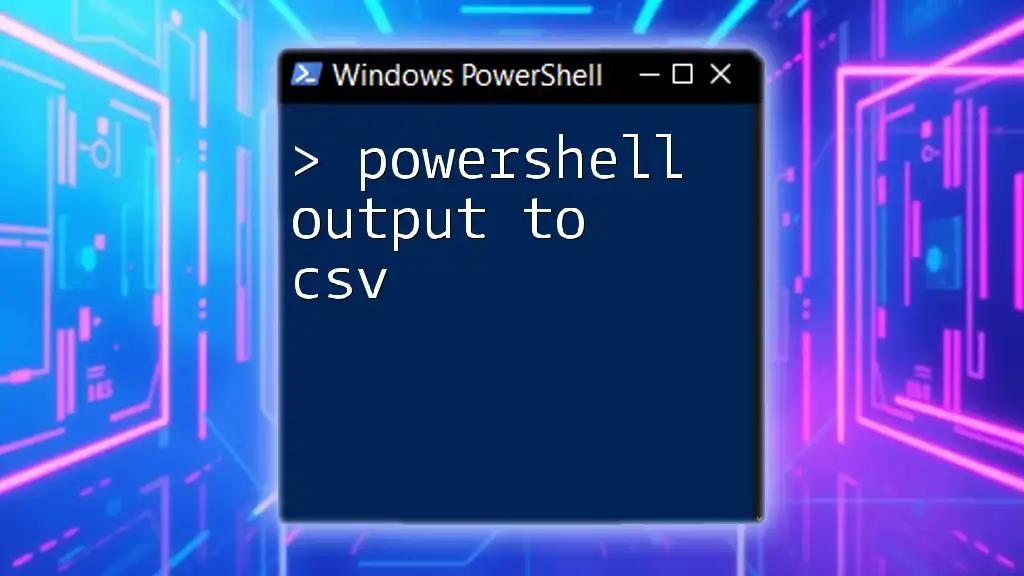
Best Practices for Managing Output
Choosing the Right Output Method
Identifying the appropriate method for output is critical in scripting. Consider if the information needs temporary review on the console or long-term storage in a file. Sensitive data should also dictate your choice; avoid unnecessary console display if the information could be exposed.
Documenting Outputs
Proper documentation enhances clarity when reviewing scripts later. Use comments liberally to explain what each command does, especially regarding the output handling part.
Example: Writing Comments in Scripts Regarding Output Handling
# This command retrieves the list of current processes and logs it
Get-Process > processes.txt
This practice fosters better collaboration among team members and helps you remember your thought process when returning to code after a break.
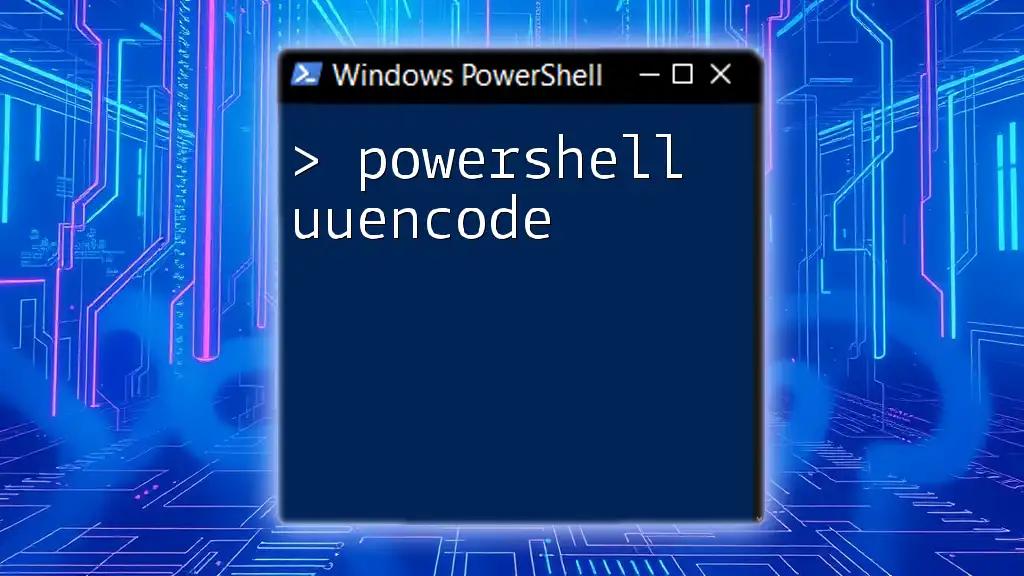
Conclusion
Effective management of PowerShell output is a cornerstone of successful scripting and automation. Understanding how to direct output to both console and files will empower you to build more powerful scripts. Embrace the capabilities of PowerShell and improve your skills by experimenting with these techniques.

Additional Resources
For those eager to dive deeper into PowerShell, explore various online courses and communities that offer advanced insights. The official Microsoft PowerShell documentation is also an excellent resource for updated information and commands.
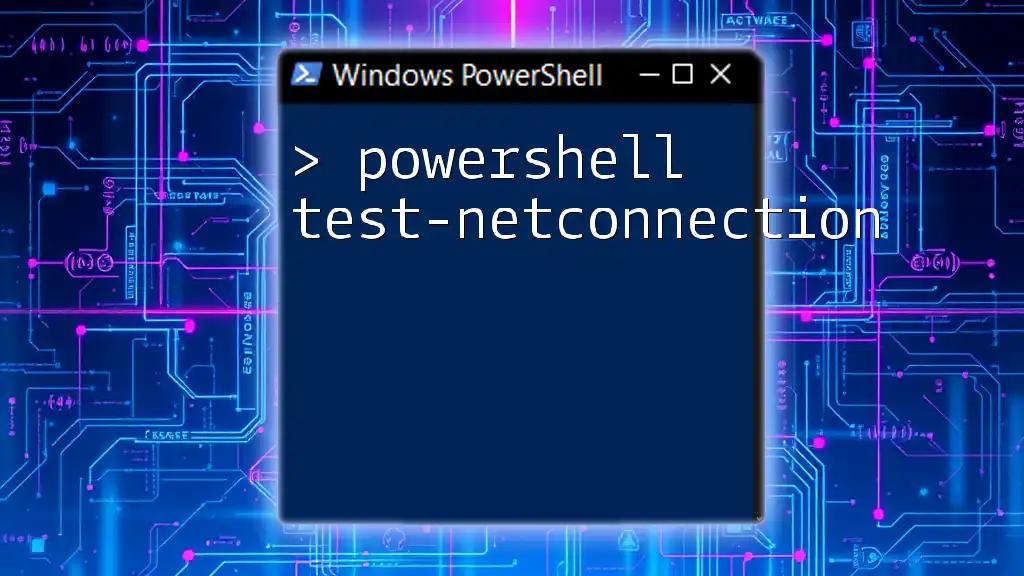
Call to Action
Challenge yourself to implement what you've learned here. Start by practicing the commands described, and feel free to share your experiences or any nuances you discovered along the way. Don't forget to subscribe for more PowerShell tutorials and tips to further enhance your scripting journey!