You can easily export PowerShell output to Excel by using the `Export-Excel` cmdlet from the ImportExcel module, allowing you to create a well-structured spreadsheet from your data.
Here's a code snippet to illustrate this:
Get-Process | Export-Excel -Path 'C:\Path\To\Your\File.xlsx' -AutoSize
Understanding Data Export
Why You Might Want to Export to Excel
Exporting data to Excel can be an invaluable tool, especially in a business context where data analysis, reporting, and visualization play crucial roles. Utilizing Excel allows for easier manipulation, sharing, and presentation of data. It can facilitate decision-making processes by providing a clear representation of information. When using PowerShell output to Excel, you can streamline the way data is gathered and utilized.
Common Use Cases
- IT Reporting: Automating system health checks or user activity logs, allowing for real-time monitoring.
- Performance Metrics: Compiling and analyzing performance data to find trends or areas for improvement.
- Data Audits: Creating detailed records that can be easily reviewed and shared with stakeholders.
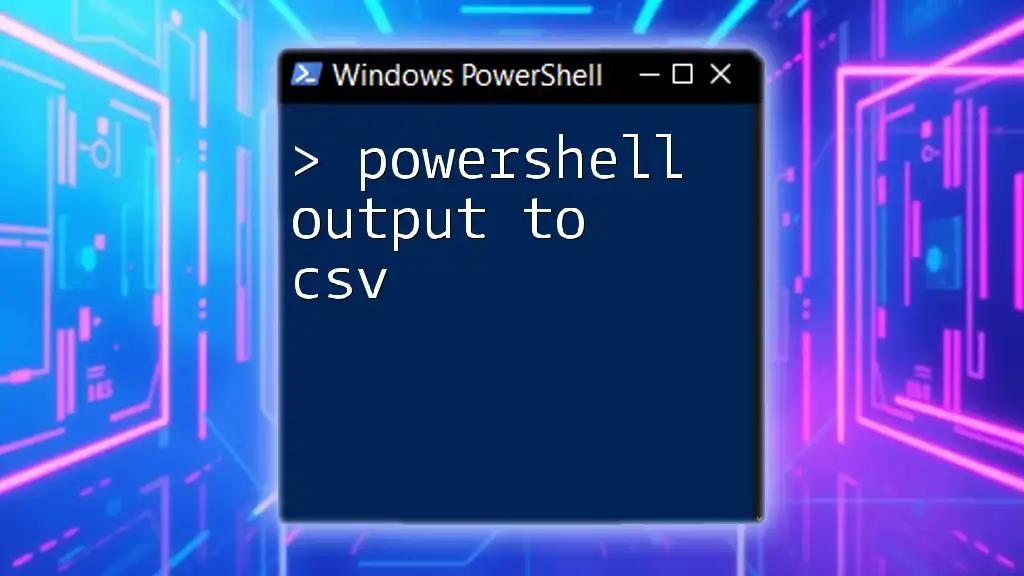
Getting Started with PowerShell
Setting Up Your Environment
To start utilizing PowerShell effectively, ensure you have the appropriate versions installed. The recommended versions of PowerShell include PowerShell 5.1 on Windows or the latest PowerShell Core for cross-platform compatibility. You also need a compatible version of Excel, as this will facilitate the exporting of data.
Basic PowerShell Syntax
Familiarizing yourself with basic PowerShell syntax is essential for operating effectively. The foundational command structure typically follows this format:
Get-Process
This command retrieves a list of all active processes on your machine and serves as a foundational example of how PowerShell outputs data.
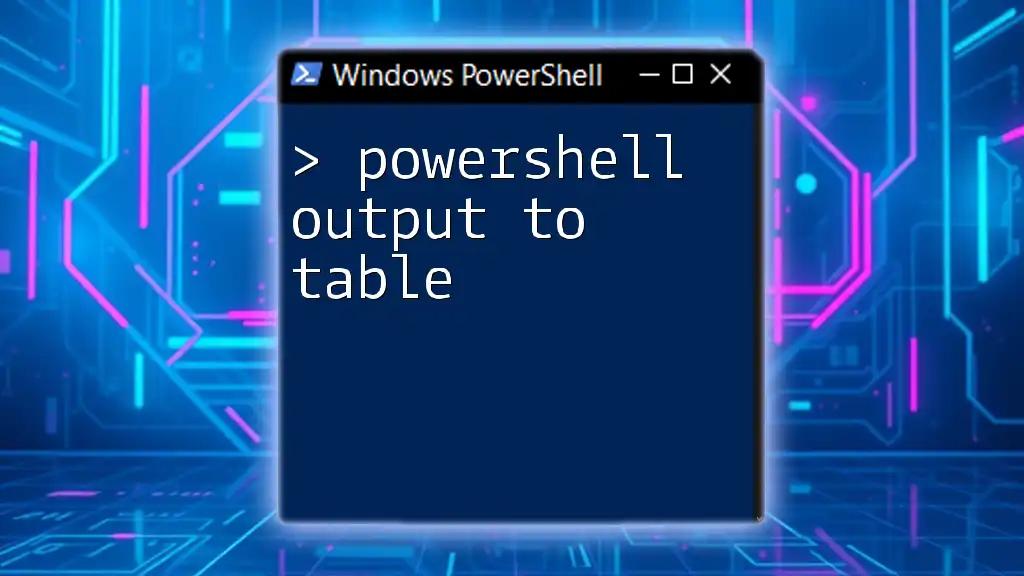
Exporting Data to Excel
Using the `Export-Csv` Cmdlet
The `Export-Csv` cmdlet is a powerful tool in PowerShell that allows you to save data in the CSV format, which Excel can easily read.
Example: Simple CSV export
Get-Process | Export-Csv -Path "processes.csv" -NoTypeInformation
In this example, `Get-Process` retrieves the active processes, and the output is directed into a CSV file named `processes.csv`. The `-NoTypeInformation` flag eliminates the extra header line that specifies the type of data, making your file cleaner and more straightforward.
Opening CSV in Excel
Once you've created a CSV file, opening it in Excel is quite simple. Just double-click the file, and it will launch Excel. You can also import it through the Excel application under the 'Data' tab for additional formatting options. Be mindful of basic formatting, as CSV files will initially display without any style. You may want to adjust column widths and apply filters to enhance readability.
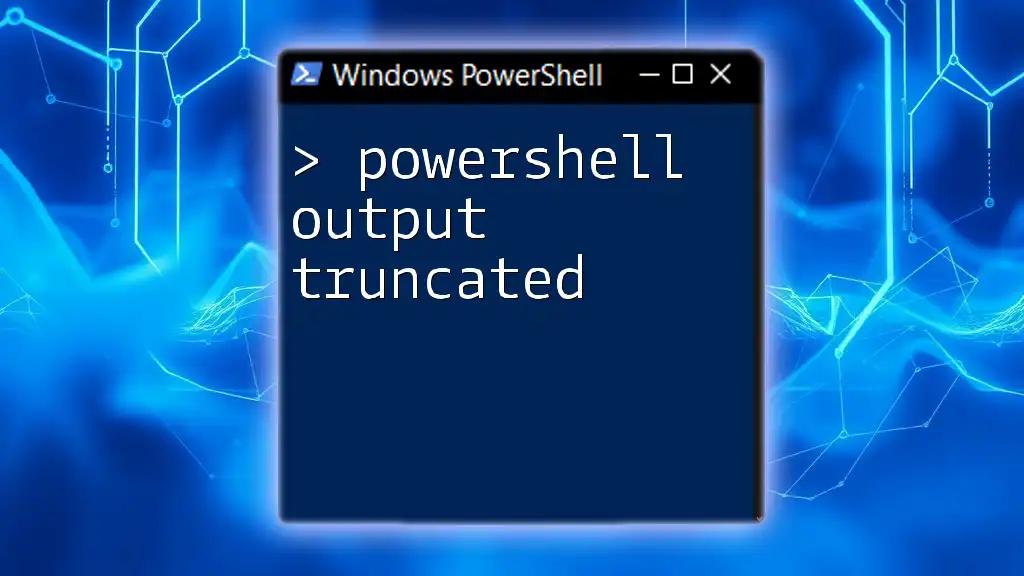
Using Excel COM Objects with PowerShell
What are COM Objects?
COM (Component Object Model) objects allow automation of applications like Excel through scripts. By leveraging COM objects, you can manipulate Excel directly within your PowerShell scripts, adding an advanced level of control to your output.
Creating a New Excel Application
To start using Excel via PowerShell, you need to create a COM object that represents the Excel application.
Example: Launching Excel with PowerShell
$excel = New-Object -ComObject Excel.Application
$excel.Visible = $true
This command initializes a new instance of Excel and makes it visible to the user, allowing you to see the changes made through your script.
Writing Data to Excel
You can programmatically add data to your Excel workbook.
Example: Writing data to a new worksheet
$workbook = $excel.Workbooks.Add()
$worksheet = $workbook.Worksheets.Item(1)
$worksheet.Cells.Item(1, 1).Value = "Process Name"
$worksheet.Cells.Item(1, 2).Value = "Process ID"
$processes = Get-Process
$row = 2
foreach ($process in $processes) {
$worksheet.Cells.Item($row, 1).Value = $process.ProcessName
$worksheet.Cells.Item($row, 2).Value = $process.Id
$row++
}
In this example, a new Excel workbook is created, and the first worksheet is accessed. The headers are set, and a loop is used to populate the worksheet with the names and IDs of all active processes running on the system.
Saving the Workbook
Once you have populated your data, you can save the workbook to your preferred location.
Example: Saving your workbook
$workbook.SaveAs("C:\Processes.xlsx")
$excel.Quit()
This code not only saves the workbook to the specified path, but it also quits the Excel application to free up system resources.
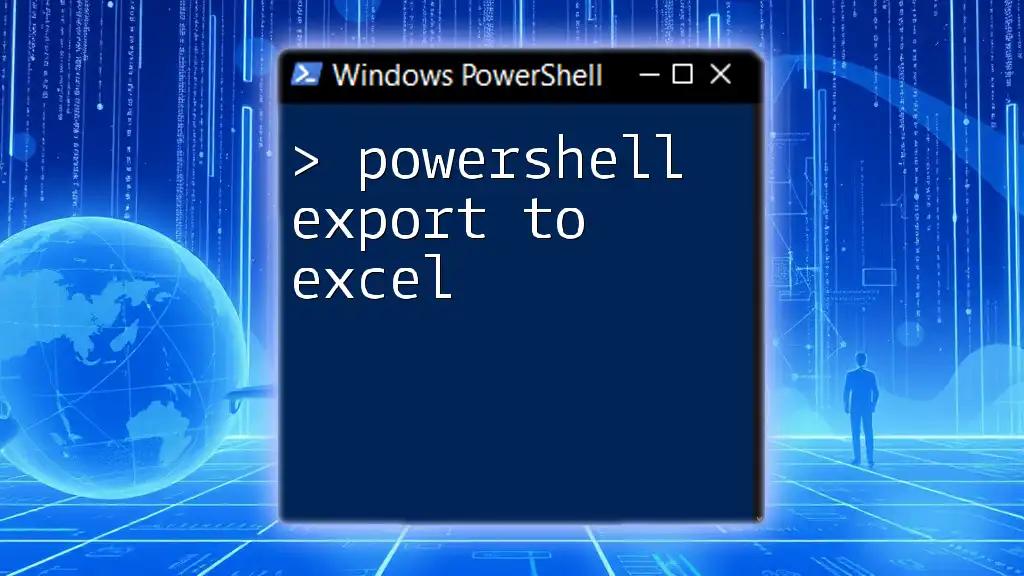
Handling Complex Datasets
Formatting Cells in Excel
To improve the presentation of your Excel output, consider formatting the cells. A well-formatted worksheet enhances readability and clarity.
Example: Setting font style and color
$worksheet.Cells.Item(1,1).Font.Bold = $true
$worksheet.Cells.Item(1,1).Font.Color = [System.Drawing.Color]::Red
This snippet makes the first cell bold and changes its font color to red, helping key information stand out.
Adding Charts and Graphs
In addition to basic data output, you can enhance your reports by adding charts to visualize the data. While this topic will require additional scripting beyond basic output, creating graphs can provide a visual interpretation of the data, making it easier to identify trends.
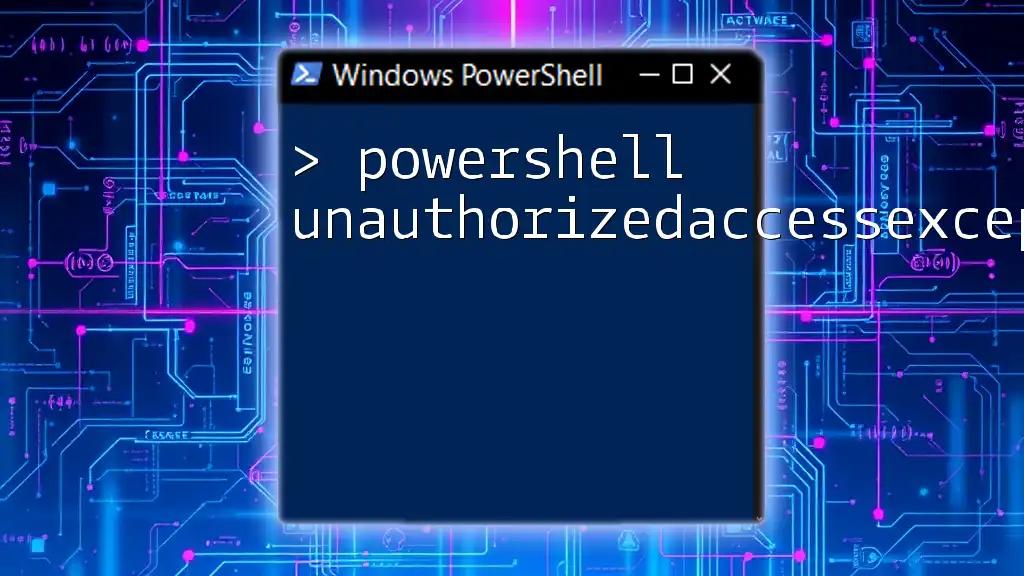
Troubleshooting and Best Practices
Common Issues While Exporting
PowerShell can sometimes yield unexpected results when exporting data. Common issues include:
- Null or Missing Data: Ensure that queries do not return null or empty results.
- Compatibility Issues: Check that your versions of PowerShell and Excel are compatible to avoid unexpected errors.
Best Practices for Data Handling
To ensure the accuracy and reliability of your PowerShell scripts:
- Keep data clean and structured to facilitate easier analysis.
- Implement validation checks to ensure the data being exported meets quality standards.
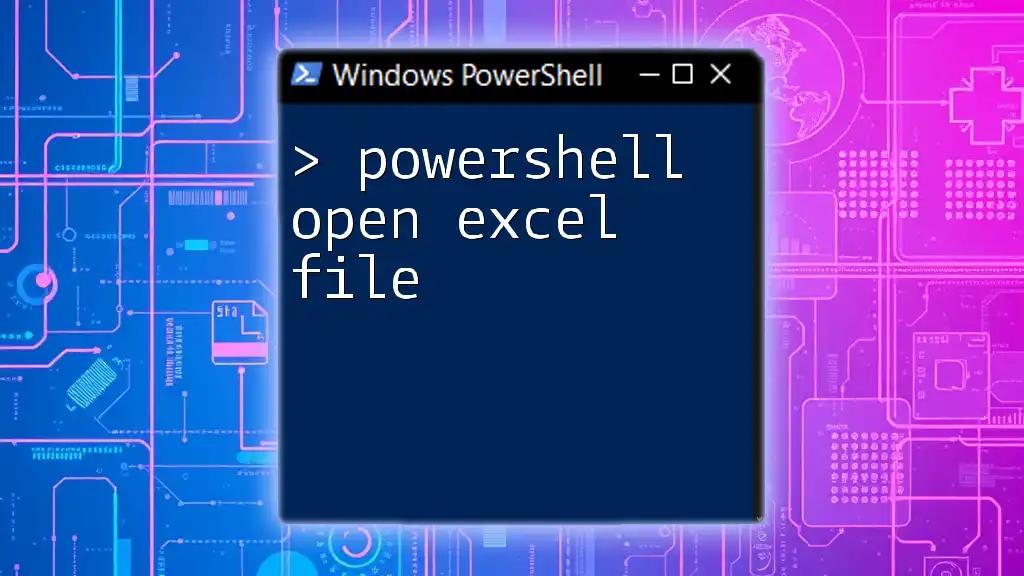
Conclusion
Exporting PowerShell output to Excel is not only a powerful feature but also a vital skill for anyone looking to enhance their data manipulation capabilities. Whether you're compiling reports, analyzing data, or simplifying audits, mastering this process will allow you to leverage PowerShell's full potential. As you continue to practice and explore more PowerShell commands, you'll find countless ways to streamline your workflows and achieve greater efficiency in your tasks.
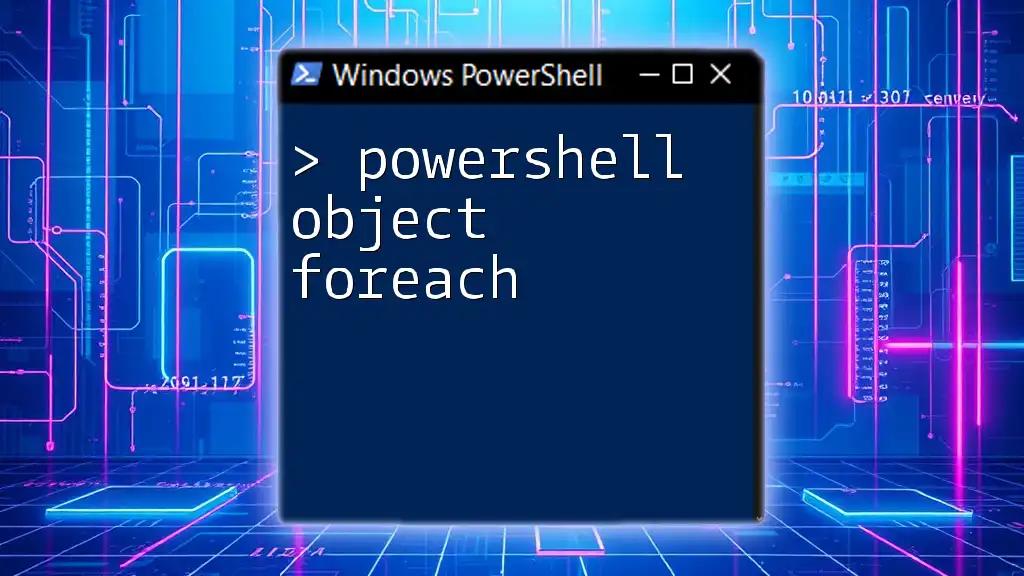
Additional Resources
To further enhance your understanding, consider diving into the following materials:
- Official PowerShell documentation
- Excel object model references
- Online forums or communities focused on PowerShell development
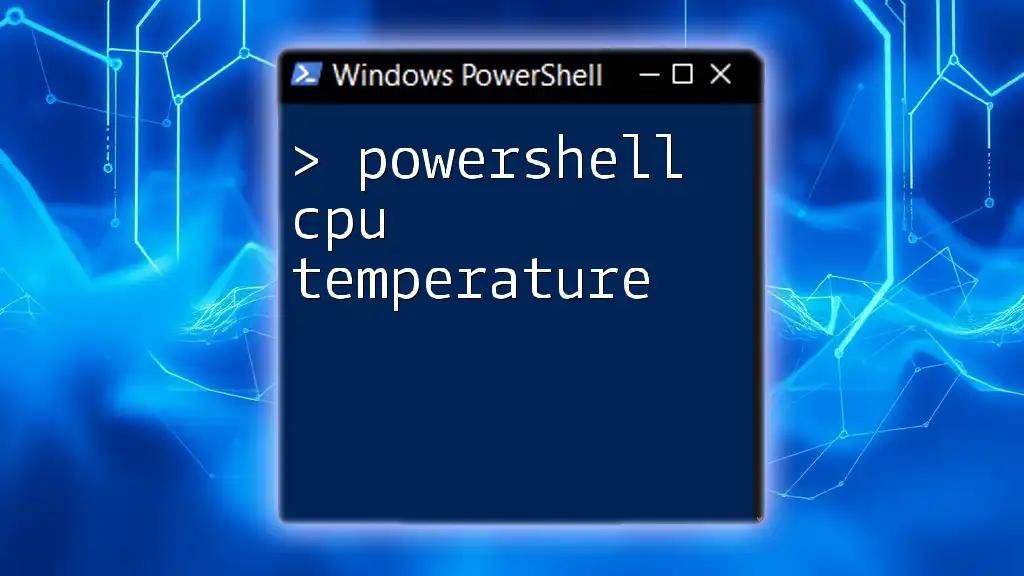
Call to Action
If you found this guide helpful, consider signing up for our workshops or newsletters to continue your journey in mastering PowerShell. Your feedback and questions are always welcome, so feel free to reach out!