You can easily export PowerShell command output to a CSV file by using the `Export-Csv` cmdlet, which formats the data into a structured CSV format for easy access and analysis. Here’s a sample code snippet:
Get-Process | Export-Csv -Path "C:\output\processes.csv" -NoTypeInformation
Understanding CSV Files
What is a CSV File?
A CSV (Comma Separated Values) file is a simple, text-based format used to store tabular data, where each line of the file corresponds to a row in the table, and each value within that row is separated by a comma. This straightforward structure allows for easy reading and writing in various applications, particularly spreadsheet software like Microsoft Excel. CSV files are often the go-to choice for exchanging and sharing data due to their simplicity and wide compatibility across different platforms.
Why Use CSV Files with PowerShell?
When working with PowerShell, exporting data to CSV format offers numerous advantages. CSV files are straightforward and human-readable, making it easy to share and analyze data without extensive formatting. Moreover, because CSV is the default format for many data-driven applications, exporting data to this format enables seamless integration into tools that users are already familiar with, such as Excel. In addition, PowerShell provides built-in functionality to handle CSV files efficiently, allowing for quick and automated reporting.
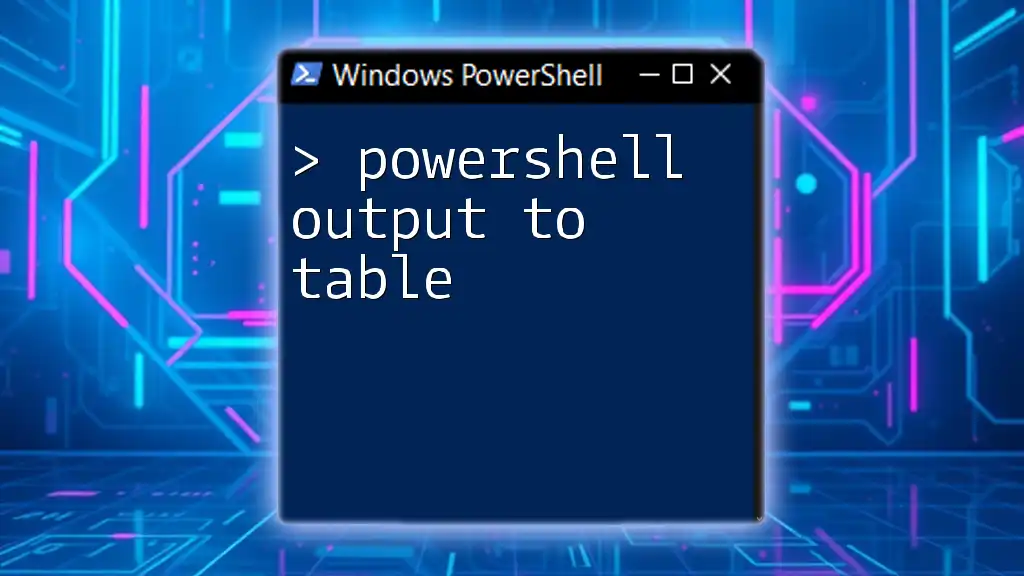
Getting Started with PowerShell
Basic PowerShell Commands
To begin exploring the PowerShell output to CSV, familiarize yourself with essential PowerShell commands. PowerShell can be accessed via the Start menu or by running `powershell` in the Command Prompt.
Understanding Objects in PowerShell
In PowerShell, data is managed in the form of objects. Every command you run typically outputs an object that represents the result of that command. Understanding how PowerShell deals with objects is crucial in effectively using commands like `Export-Csv`. Each object can have multiple properties, which can then be used as fields in your CSV files.
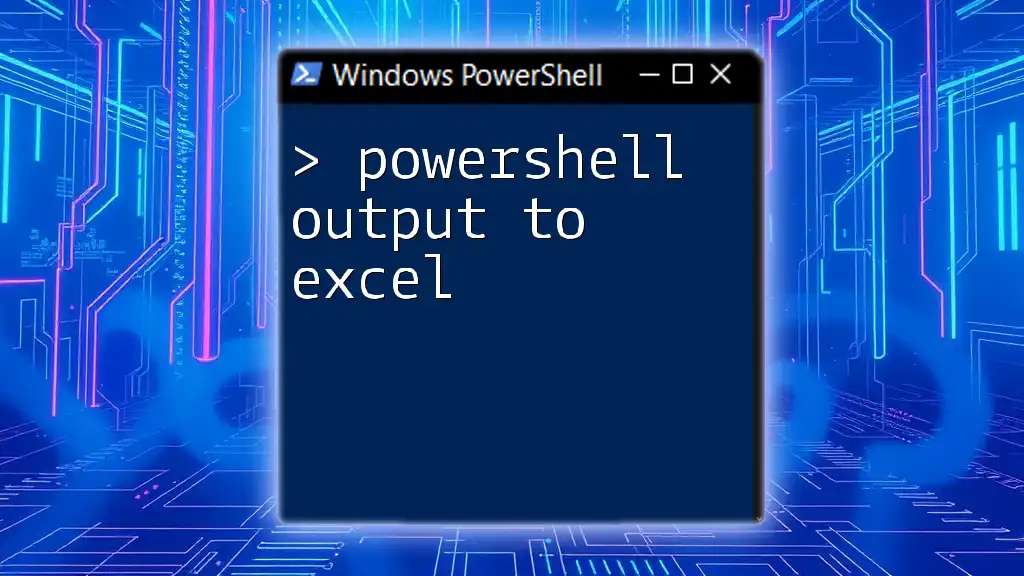
Exporting Data to CSV with PowerShell
The `Export-Csv` Cmdlet
The primary cmdlet for exporting data in CSV format is `Export-Csv`. This cmdlet takes the output of any command and converts it into a CSV file. The syntax of the cmdlet is straightforward:
Export-Csv -Path <String> [-NoTypeInformation] [-Delimiter <Char>] [-Encoding <System.Text.Encoding>]
- Path: Specifies the path where you want to save the CSV file.
- NoTypeInformation: When included, this parameter ensures that type information is not included as the first line in the CSV, keeping the file cleaner.
- Delimiter: Allows you to specify a different delimiter if necessary; the default is a comma.
- Encoding: Lets you set the encoding type.
Here's an example of exporting a list of running processes to a CSV file:
Get-Process | Export-Csv -Path "C:\processes.csv" -NoTypeInformation
In this command:
- `Get-Process` retrieves all current processes.
- `Export-Csv` converts the output into a CSV format and saves it to the specified file path.
Customizing Output with Export-Csv
Specifying the Output Path
When using `Export-Csv`, always carefully define your output path to avoid errors. For instance, if the provided path doesn’t exist or lacks proper permissions, the command will fail. Here’s an example that exports service data:
Get-Service | Export-Csv -Path "C:\services.csv" -NoTypeInformation
Managing Field Headers
Sometimes you might want to customize the headers of your output CSV. The `-Header` parameter lets you specify your own column names. Here’s an example:
Get-Process | Export-Csv -Path "C:\custom.csv" -Header "ProcessName", "ID"
In this case, we replaced the default headers with our own, ensuring the CSV suits our specific reporting requirements.
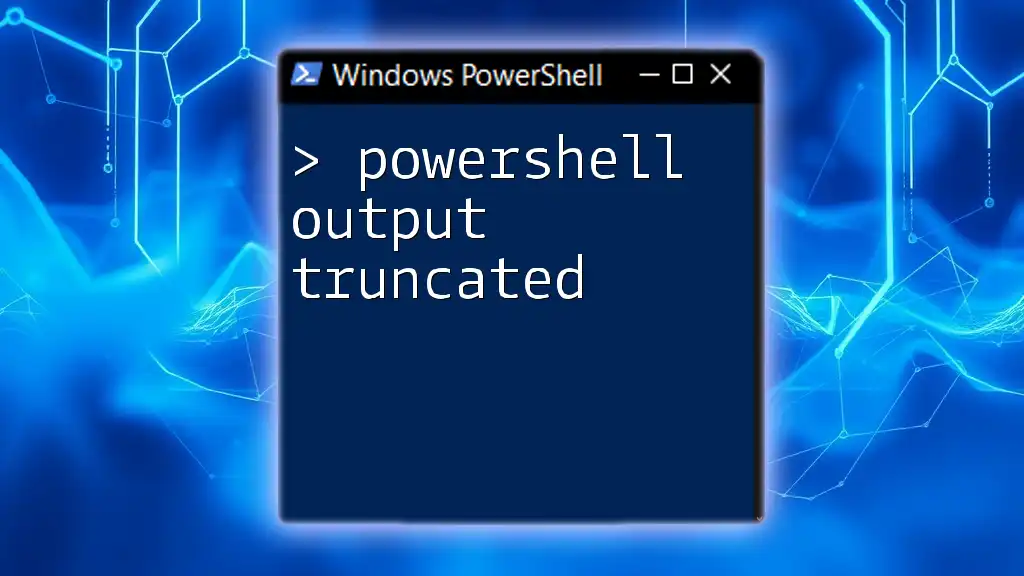
Importing Data Back from CSV
The `Import-Csv` Cmdlet
In addition to exporting data, PowerShell allows for easy importation of CSV files through the `Import-Csv` cmdlet. This enables you to bring in CSV data as PowerShell objects for processing and analysis. Take a look at this example:
$importedData = Import-Csv -Path "C:\processes.csv"
In this snippet, we load the data from the CSV file into the `$importedData` variable, where each row in the CSV becomes an individual object in PowerShell.
Manipulating Imported Data
Once you've imported data from a CSV file, you can manipulate it as needed. For instance, to filter out processes with IDs greater than 1000, you could use the following command:
$importedData | Where-Object { $_.ID -gt 1000 }
This command allows for quick analysis or reporting on specific subsets of data.
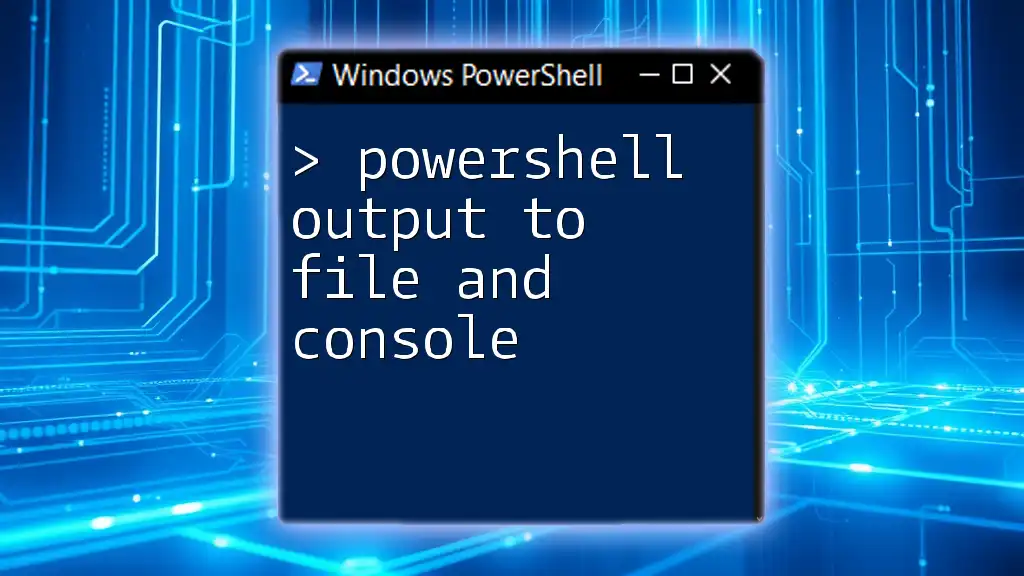
Real-World Use Cases
Managing System Processes
A practical use of exporting PowerShell output to CSV is monitoring active processes. You could create a simple script that outputs details of running processes to a CSV, making it easy to generate reports.
Reporting Active Directory Users
Another useful application is exporting user data from Active Directory. Here is an example command that retrieves all AD users and saves their names and email addresses to a CSV:
Get-ADUser -Filter * | Select-Object Name, EmailAddress | Export-Csv -Path "C:\ADUsers.csv" -NoTypeInformation
This command is particularly valuable for IT administrators who need to maintain comprehensive user records.
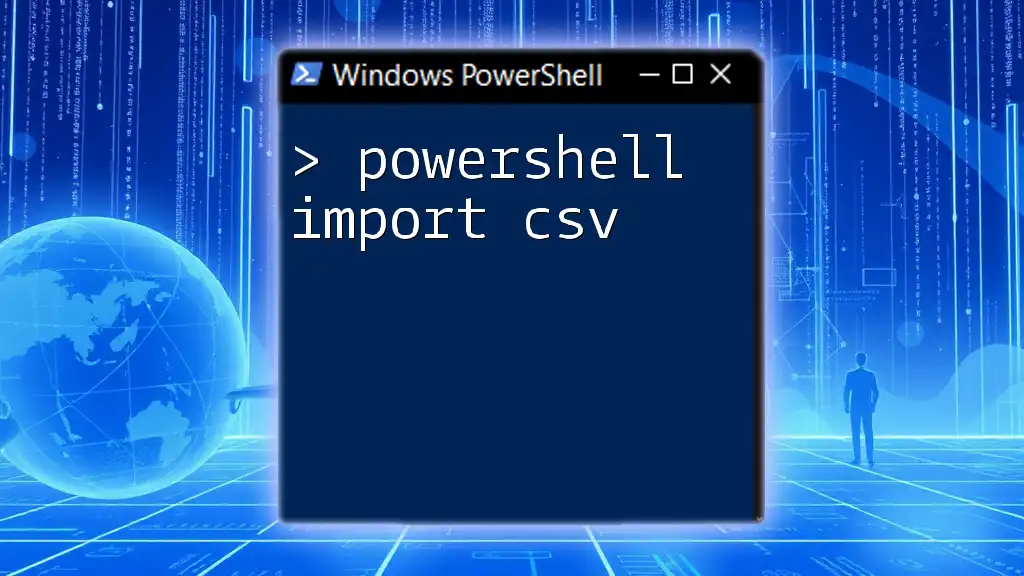
Troubleshooting Common Issues
Permission Issues
One common problem may arise from lack of adequate permissions to write to the specified file path. Ensure that the PowerShell session has the necessary permissions, particularly when running scripts that export data.
Encoding Problems
Encoding problems can occur if you are dealing with special characters in your data. To avoid issues, you can specify the encoding type using the `-Encoding` parameter:
Get-Process | Export-Csv -Path "C:\processes.csv" -Encoding UTF8 -NoTypeInformation
This ensures that the CSV file is saved using UTF-8 encoding, which can properly handle a wide range of characters.
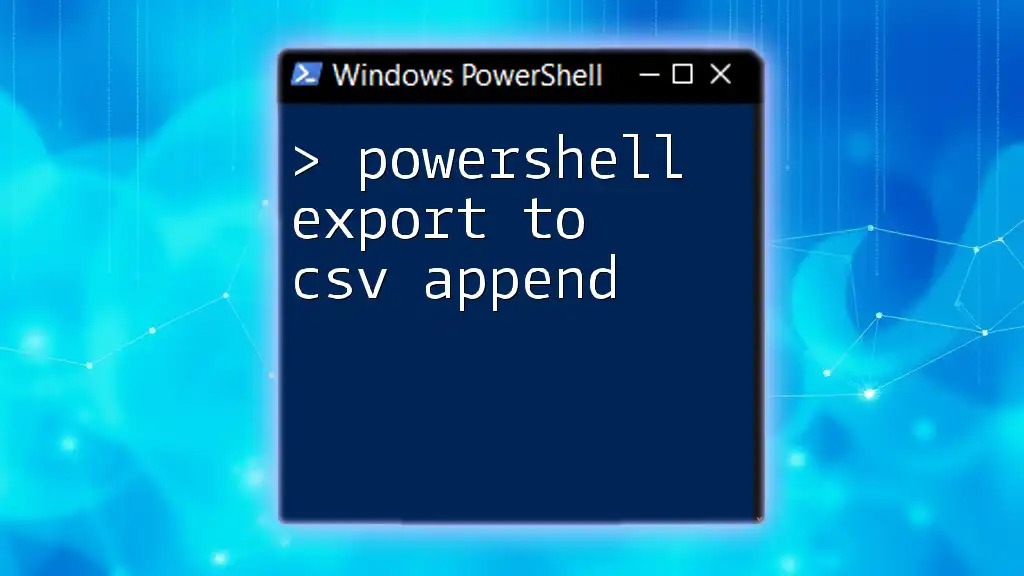
Tips and Best Practices
Choosing the Right Cmdlets
Utilize cmdlets that neatly integrate with `Export-Csv`. Commands like `Get-Process`, `Get-Service`, and `Get-ADUser` are excellent candidates for exporting data efficiently.
Organizing Your Scripts
Always organize your scripts logically and incorporate comments that explain functionality. This practice helps when revisiting projects or sharing your scripts with colleagues.
Automating CSV Exports
Consider scheduling automatic exports using Windows Task Scheduler or PowerShell scripts. This capability can enhance productivity by ensuring data is always up-to-date without manual intervention.
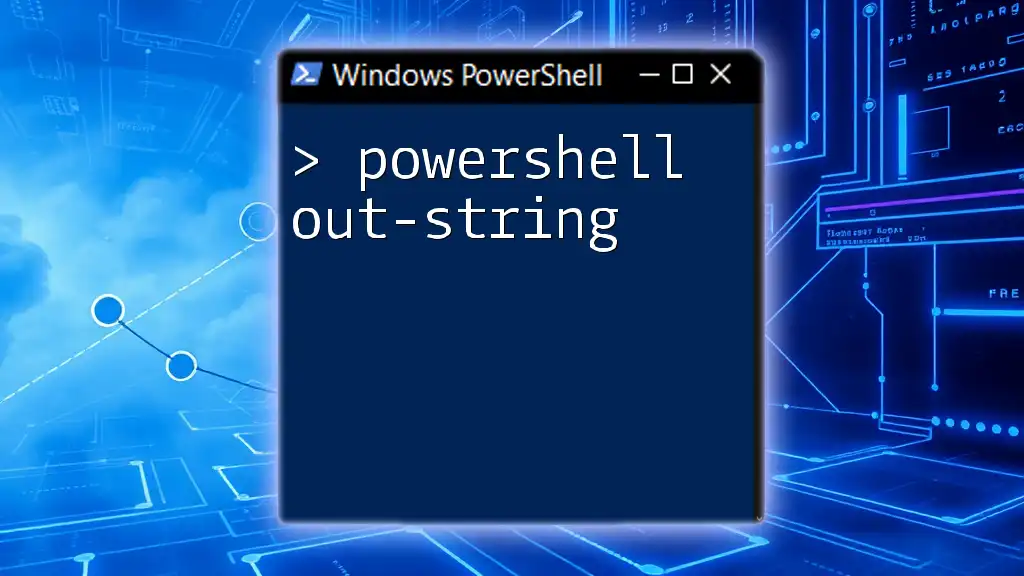
Conclusion
PowerShell is a powerful tool for automating tasks, and its compatibility with CSV files streamlines data management and reporting. By mastering the export and import capabilities of PowerShell, users can harness significant efficiencies in their workflows. Practice makes perfect, so dive into PowerShell and experiment with its commands to see firsthand how easily you can manipulate data and generate reports in CSV format.
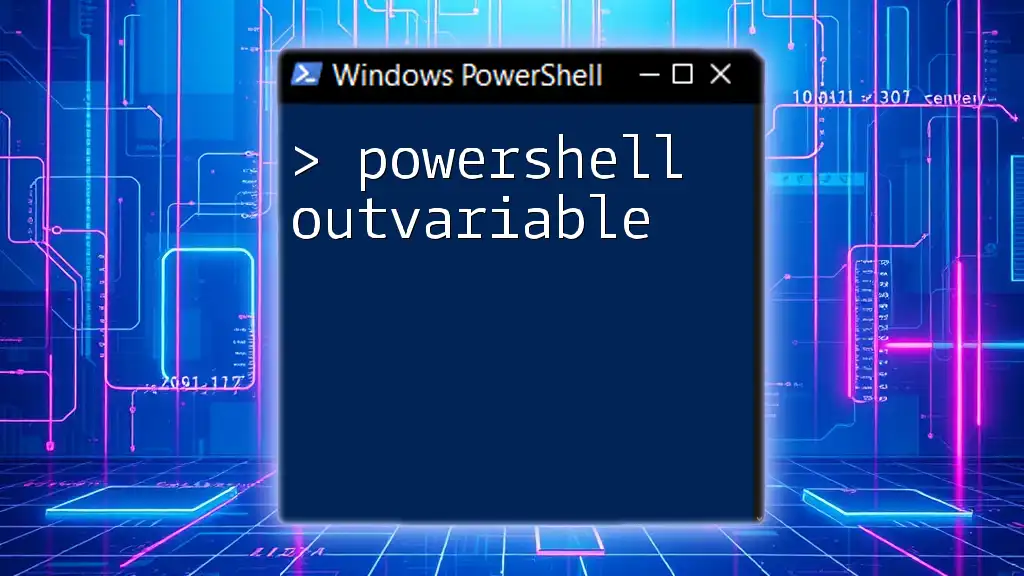
Additional Resources
For further exploration, consider delving into more advanced PowerShell functions or joining communities focused on PowerShell scripting. Microsoft's official documentation is also an excellent resource for in-depth knowledge and updates on cmdlets and features.