You can easily append data to a CSV file in PowerShell by using the `Export-Csv` cmdlet with the `-Append` parameter, which allows you to add new entries while preserving existing data.
Here's a code snippet to illustrate this:
$NewData = @(
[PSCustomObject]@{Name='John Doe'; Age=30; Occupation='Developer'},
[PSCustomObject]@{Name='Jane Smith'; Age=28; Occupation='Designer'}
)
$NewData | Export-Csv -Path 'C:\Path\To\Your\File.csv' -NoTypeInformation -Append
What is CSV?
Understanding CSV Files
CSV stands for Comma-Separated Values, a simple file format used to store tabular data, making it a staple for data management. This format allows data to be exported from database management systems and spreadsheet applications, facilitating easy exchange between different programs.
CSV files are widely appreciated for their simplicity and readability. The structure—plain text separated by commas—means they can be easily viewed and edited with basic text editors or opened in applications like Microsoft Excel. As a result, CSVs are often employed in data analysis, reporting, and importing/exporting data.
PowerShell and CSV Integration
PowerShell is a powerful command-line tool and scripting language developed by Microsoft. Its robust capabilities enable users to automate tasks, manage configurations, and manipulate data efficiently.
Integrating PowerShell with CSV files provides numerous benefits. It streamlines batch processing and data management tasks, enhances automation capabilities, and allows for dynamic data manipulation without the need for extensive coding knowledge.

Appending Data to a CSV File
Basics of Appending in PowerShell
Appending data in the context of file management refers to the process of adding new data to an existing file without overwriting its current contents. In PowerShell, this can be done seamlessly with the right cmdlets, ensuring that data integrity is maintained while continually updating the file.
PowerShell Append to CSV Command
To effectively append data to a CSV file, PowerShell uses the `Export-Csv` cmdlet coupled with the `-Append` parameter. This combination allows users to add entries without deleting pre-existing information.
Here's an example command:
Import-Csv -Path "source.csv" | Export-Csv -Path "destination.csv" -Append
In this command, `Import-Csv` reads the source file, while `Export-Csv -Append` ensures that the resulting data is added to `destination.csv` without removing any prior data. Understanding the parameters and their roles is crucial for efficient data management.

Using `Export-Csv` to Append Data
Understanding the `Export-Csv` Cmdlet
The `Export-Csv` cmdlet is a fundamental PowerShell tool designed to convert data objects into a CSV format. When used with the `-Append` parameter, it allows users to add new data to existing CSV files easily.
The `-NoTypeInformation` parameter is also essential, as it prevents PowerShell from adding additional metadata that can complicate the structure of the resulting CSV. This keeps the format simple and clean, ideally suited for subsequent data processing.
Example of Appending Data
Appending to an Existing CSV
When it comes to appending data, creating a consistent data structure is essential. Consider the following example:
$data = @"
Name, Age, Occupation
John Doe, 30, Developer
"@
$data | ConvertFrom-Csv | Export-Csv -Path "people.csv" -NoTypeInformation -Append
In this code snippet:
- A string is defined as `data`, representing new entries in a CSV-friendly format.
- `ConvertFrom-Csv` transforms that string into PowerShell objects, ready for exporting.
- Finally, `Export-Csv -Path "people.csv" -Append` writes the new data to an existing file without overwriting it.

Step-by-Step Guide to Append Data to CSV
Starting with an Existing CSV File
Before appending data, it’s crucial to ensure that the CSV file already exists. This can be easily accomplished with the `Test-Path` cmdlet:
Test-Path "mydata.csv"
This command checks if the file `mydata.csv` exists, preventing unnecessary errors when attempting to append data to a non-existent file.
Creating a New Data Entry
Creating a new data entry or set of entries for appending can be accomplished through PowerShell objects. The following example illustrates this method:
$newEntry = New-Object PSObject -Property @{
Name = "Jane Smith"
Age = 28
Occupation = "Designer"
}
$newEntry | Export-Csv -Path "people.csv" -NoTypeInformation -Append
In this snippet:
- A new object is created with specific properties using `New-Object`.
- This object represents the new data to be appended.
- The object is piped directly into `Export-Csv`, allowing for a seamless addition to our existing CSV file.
Appending Multiple Entries
PowerShell also supports appending multiple entries at once. Here’s how to do it:
$entries = @(
New-Object PSObject -Property @{ Name = "Chris Evans"; Age = 35; Occupation = "Actor" },
New-Object PSObject -Property @{ Name = "Natasha Romanoff"; Age = 33; Occupation = "Spy" }
)
$entries | Export-Csv -Path "people.csv" -NoTypeInformation -Append
This example consolidates several new entries into an array:
- Each entry represents a unique block of data.
- They are simultaneously appended to the CSV through a single `Export-Csv` call, demonstrating how PowerShell can handle multiple data inputs efficiently.

Common Issues and Troubleshooting
Handling Data Overwrites
One of the most common pitfalls when working with CSV files in PowerShell is not using the `-Append` switch, which can result in overwriting existing data. Always double-check your commands to prevent unintentional loss of critical data.
CSV Format Issues
CSV format requires consistency in structure. If new entries do not align with the existing column names or formatting, the result may lead to parsing errors. It's crucial to validate your data before appending to ensure accuracy.
PowerShell Permissions
Permissions can restrict file access. If you're unable to append data due to permission errors, use the following command to check the file's attributes and modify them if necessary:
Get-Acl "people.csv" | Format-List
This command reveals the current file permissions, allowing you to identify any issues.
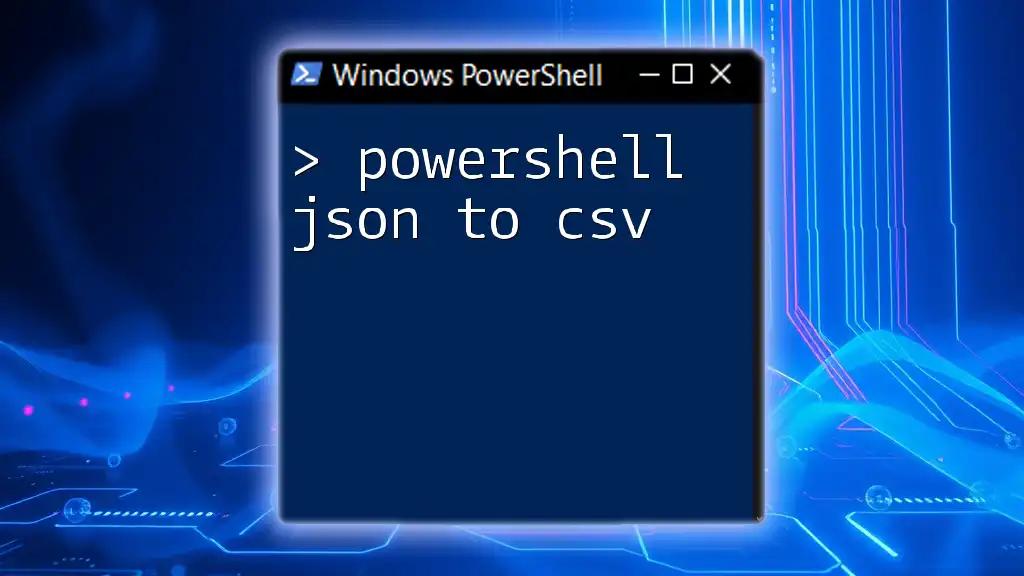
Best Practices for Appending Data to CSV
Data Validation Before Appending
Don’t underestimate the importance of validating your data to ensure the correctness of entries. Before proceeding with an append operation, consider implementing checks to verify that the data conforms to expected formats and types.
Consistent CSV Formatting
Maintaining consistent column names and data types is critical when appending. Differences in naming conventions or formatting can lead to complications when processing data later.
Backup Existing CSV Files
Before making changes to significant data files, it’s a good practice to create a backup. By duplicating your CSV, you safeguard against data corruption or loss during the appending process.
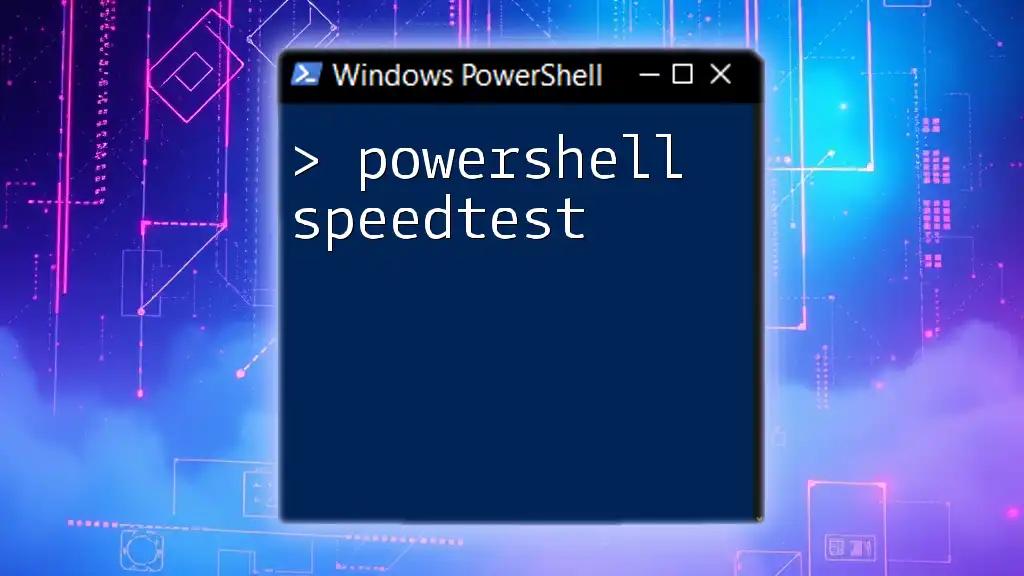
Conclusion
Now that you are familiar with how to effectively use PowerShell commands to append data to CSV files, you possess a valuable skill for managing and automating data operations seamlessly. With practice, these techniques will enhance your proficiency in handling data tasks and enable you to leverage the full power of PowerShell in your projects.
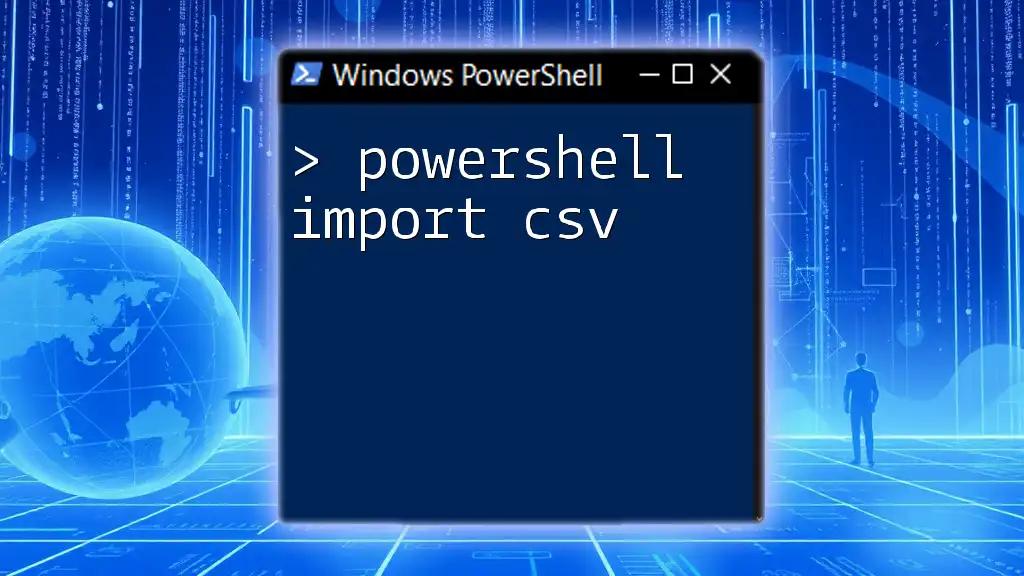
Additional Resources
For further exploration, consider reviewing official Microsoft documentation on PowerShell, as well as resources on CSV file handling and data management practices. Staying informed will ensure you are equipped to tackle any challenges you may encounter in your data endeavors.