In PowerShell, you can append text to a file using the `Add-Content` cmdlet, which adds specified content to the end of a file without overwriting its existing data. Here's a code snippet demonstrating this:
Add-Content -Path "C:\path\to\your\file.txt" -Value "Hello, World!"
What Does Append Mean in PowerShell?
In the context of file operations, appending refers to the action of adding new content to the end of an existing file without altering or deleting the existing data. This is in contrast to overwriting, where the entire file is replaced with new content. Understanding this distinction is crucial for effective file management and data integrity in PowerShell.

Why Use PowerShell to Append to a File?
PowerShell is a powerful tool designed for automation and task management, particularly when it comes to file handling. Here are some advantages of using PowerShell for appending to files:
- Automation: PowerShell can automate repetitive tasks, enabling you to run scripts that append data consistently without manual intervention.
- Efficiency: With simple and concise commands, you can quickly append data to files without complex programming logic.
- Versatility: PowerShell can handle different file types, ranging from text files to CSVs, making it a flexible choice for various scenarios.

Overview of File Writing in PowerShell
Before diving into how to append to a file in PowerShell, it's important to understand file writing operations. PowerShell primarily utilizes several cmdlets for file handling:
- Out-File: Overwrites or creates a new file.
- Set-Content: Replaces content in a file.
- Add-Content: Appends data to the end of a file.
For appending text, we will focus on the `Add-Content` cmdlet.

Using PowerShell to Append to a File
The Basic Command Structure
The cmdlet that allows you to append text to a file is `Add-Content`. This is the primary command you'll use when you want to add data without affecting existing content in your file.
Syntax of the Command
The syntax of the `Add-Content` cmdlet can be broken down as follows:
Add-Content -Path "Path\To\Your\File.txt" -Value "Text to append"
- `-Path`: Specifies the file to which you want to append data.
- `-Value`: The content that you wish to add.
Practical Example: Appending Text
Let’s look at a straightforward example of appending text to a file:
Add-Content -Path "C:\temp\myfile.txt" -Value "This is a new line of text."
After executing this command, the text "This is a new line of text." will be added to the end of myfile.txt without deleting any existing lines.
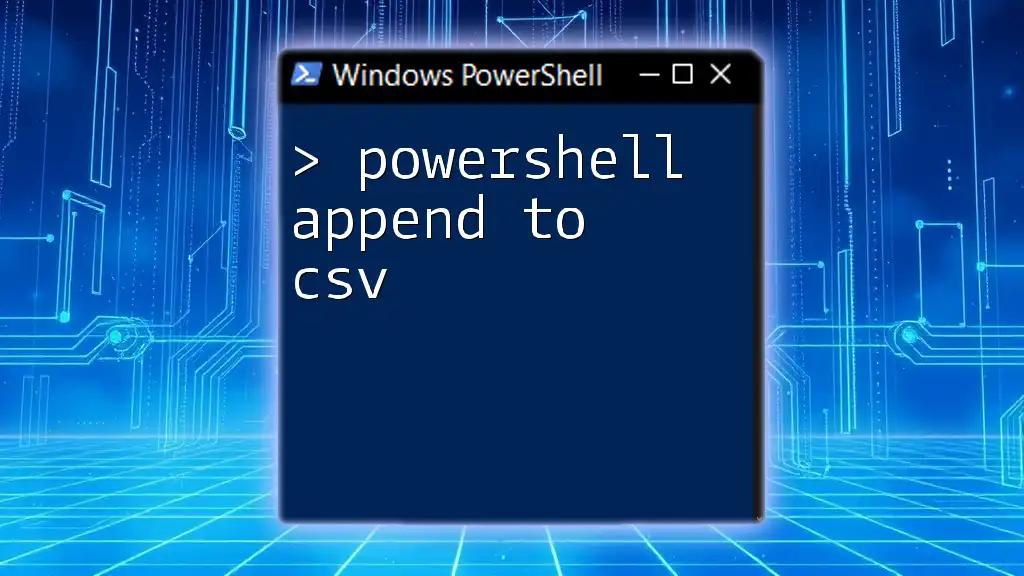
Appending Multiple Lines of Text
Using an Array to Append
To append multiple lines to a file in one go, you can utilize an array. Here’s how it works:
$lines = "First line", "Second line", "Third line"
Add-Content -Path "C:\temp\myfile.txt" -Value $lines
This command will append all three lines consecutively to the end of myfile.txt. It’s a concise method to add multiple entries without repetitive commands.
Reading from One File and Appending to Another
PowerShell also allows you to read content from one file and append it to another. Here’s a practical example:
Get-Content -Path "C:\temp\source.txt" | Add-Content -Path "C:\temp\destination.txt"
This command will take the content of source.txt and append it to destination.txt, efficiently consolidating file data.
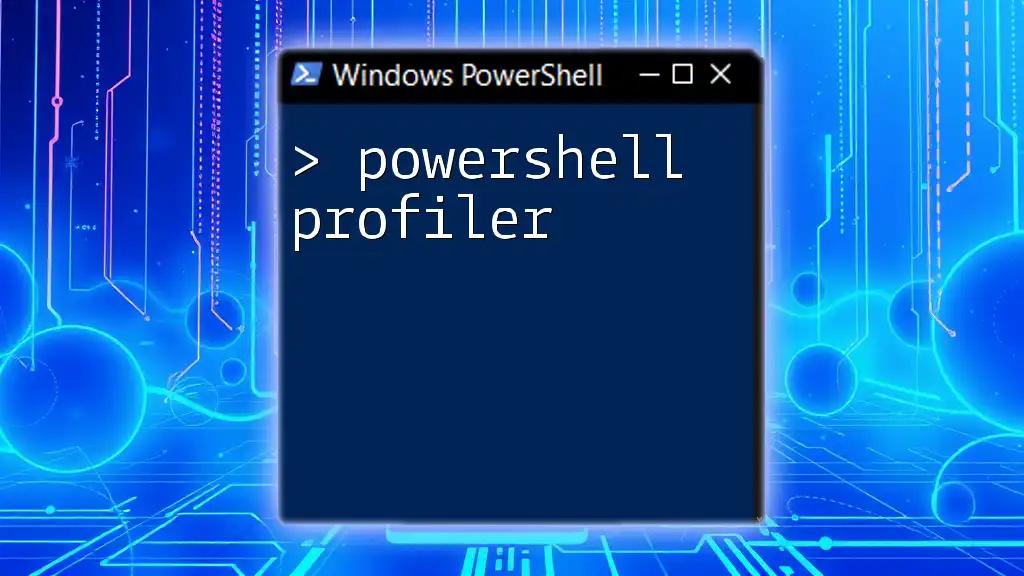
Advanced Appending Techniques
Conditional Appending using PowerShell
Sometimes, it may be necessary to append text only if certain conditions are met. For instance, you may want to append new data only if specific text isn't already present in the file. Here’s how to implement this:
if (-not (Get-Content -Path "C:\temp\myfile.txt" | Select-String -Pattern "Specific Text")) {
Add-Content -Path "C:\temp\myfile.txt" -Value "Appended text only if not present."
}
In this example, the command checks the content of myfile.txt for "Specific Text". If this text isn’t found, it appends a specified string. This can be particularly helpful when avoiding duplicates.
Error Handling During Append Operations
Error handling is a critical aspect of file operations. You can ensure your appending process is robust by incorporating `Try-Catch` blocks. Here’s an example:
Try {
Add-Content -Path "C:\temp\invalidPath\myfile.txt" -Value "Trying to append"
} Catch {
Write-Host "An error occurred: $_"
}
In this example, if the specified path is invalid, PowerShell will catch the error and display a descriptive message, preventing the script from crashing and providing feedback.
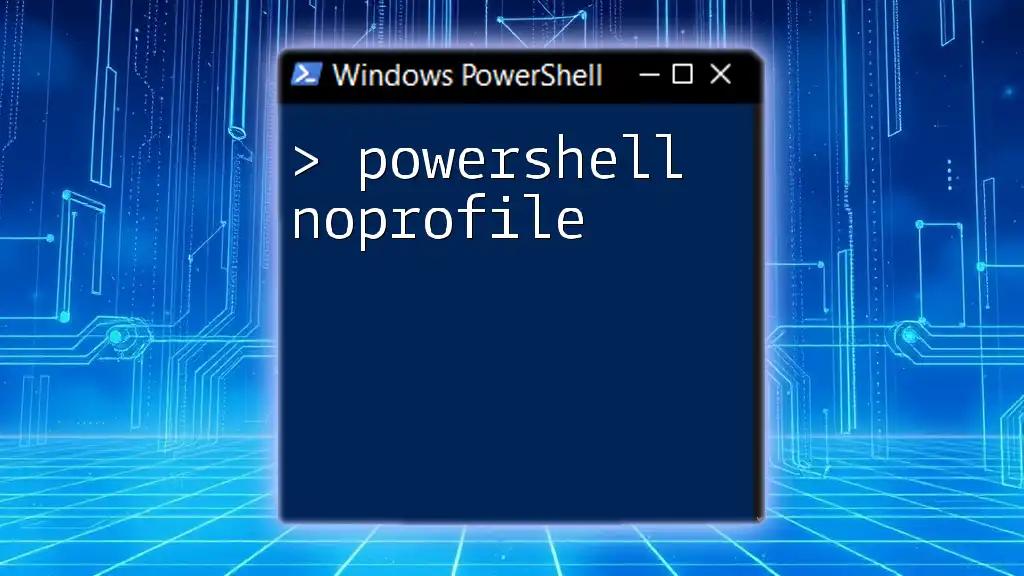
Best Practices for Appending to Files in PowerShell
To ensure effective file operations, consider the following best practices:
- Ensure Existence: Always verify that the target file path exists before attempting to append data.
- Use Absolute Paths: Utilizing full file paths mitigates confusion and ensures your commands target the correct files.
- Documentation: It’s essential to document your PowerShell scripts to allow for easier understanding and maintenance by others.
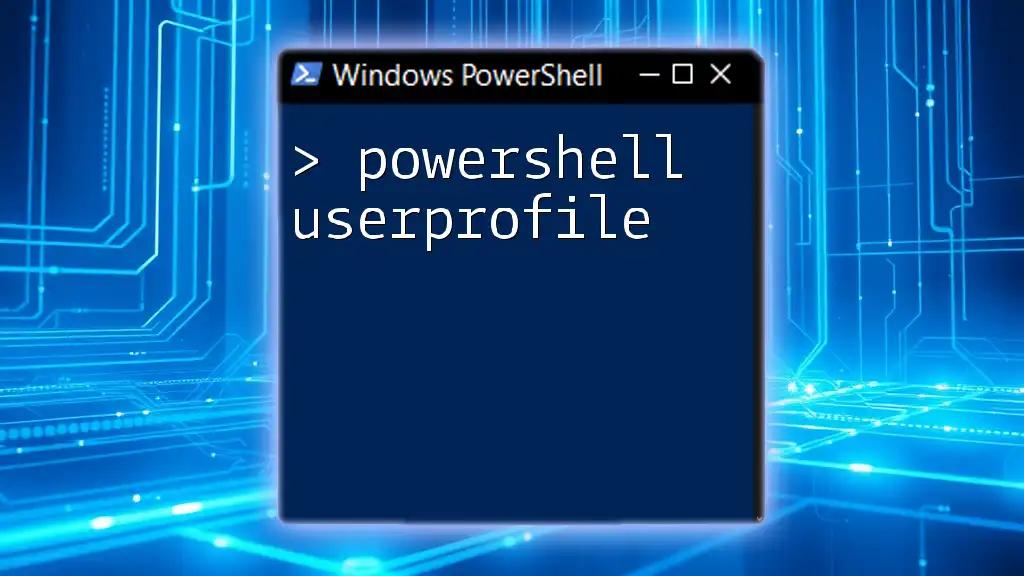
Conclusion
Mastering the ability to append to a file in PowerShell is a valuable skill that enhances your file management capabilities. With straightforward commands like `Add-Content`, combined with techniques for error handling and conditional appending, you can manipulate files effectively and efficiently.
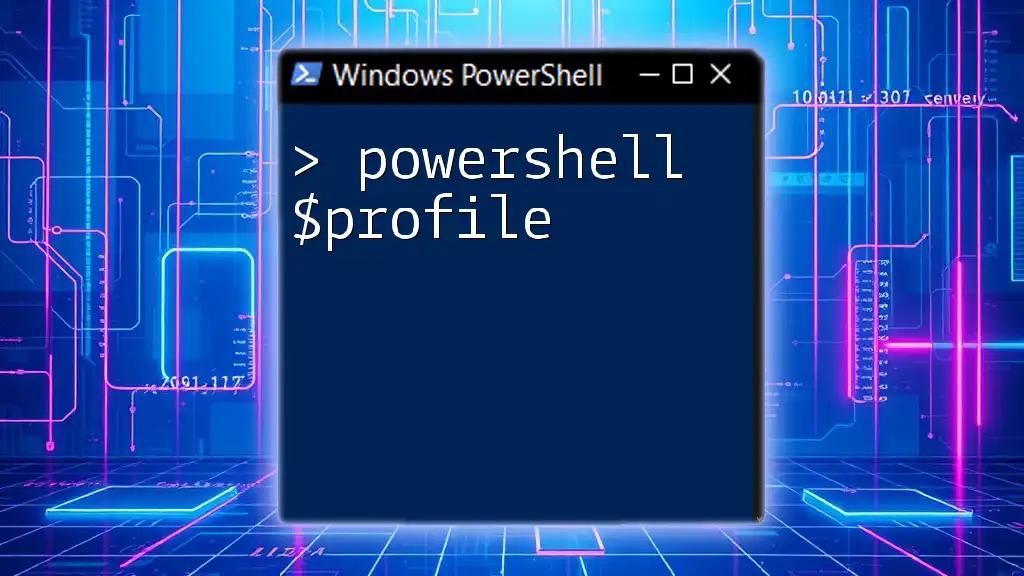
Call to Action
Feel free to share your thoughts or any questions you might have regarding appending to files in PowerShell. Don’t forget to subscribe for more tips, tricks, and tutorials on PowerShell functionalities that can aid your scripting journey!
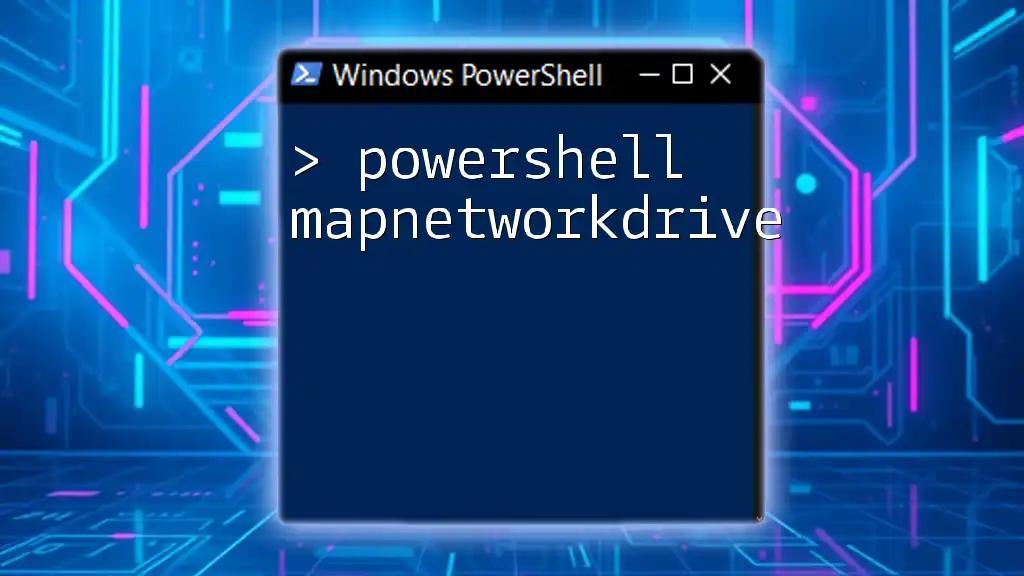
References
For further information and resources, consider exploring the official Microsoft documentation on PowerShell cmdlets related to file handling.