You can easily format PowerShell output into a table using the `Format-Table` cmdlet, which allows for clear visualization of data in a structured format.
Here’s a simple example:
Get-Process | Format-Table -Property Name, Id, WS -AutoSize
This command retrieves all running processes and displays their names, IDs, and working set sizes in a neatly formatted table.
Understanding PowerShell Output
What is PowerShell Output?
In PowerShell, output refers to the result generated by executing commands or scripts. PowerShell is fundamentally designed to work with objects rather than just text, allowing you to manipulate and format data effectively. This object-oriented approach provides more flexibility and capability than traditional command-line interfaces.
Importantly, understanding the differences between object output and string output in PowerShell is essential. Object output retains its structured nature, enabling further manipulation and filtering, while string output is limited to plain text. Choosing the right output format is crucial for the clarity and usability of your scripts, particularly when dealing with large amounts of data.
Why Use Tabular Output?
Using tabular formats for displaying data in PowerShell provides several distinct advantages. When working with complex outputs, especially from commands generating extensive lists, tabular output enhances readability, allowing users to quickly scan through data.
Some scenarios where tabular output is particularly beneficial include:
- Viewing system performance metrics
- Analyzing user accounts and permissions
- Generating reports for administrative tasks
In each of these cases, presenting data in a table format makes it much easier to identify trends and anomalies.
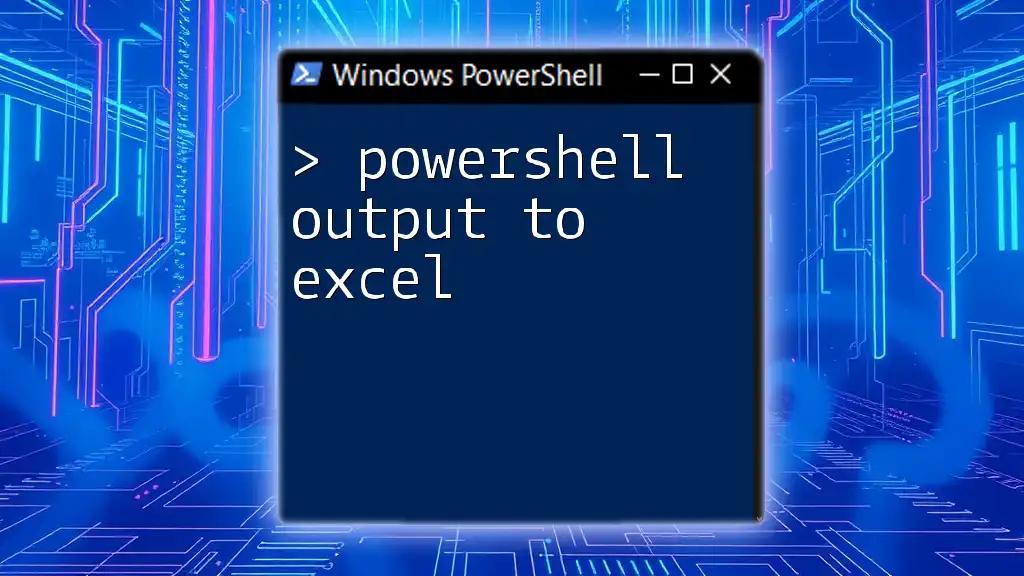
Getting Started with PowerShell Tabular Output
Basic Formatting with `Format-Table`
To begin formatting output in a table structure, PowerShell provides the `Format-Table` cmdlet. This cmdlet allows you to shape your output into a clear and visually structured format.
Overview of `Format-Table` Cmdlet
`Format-Table` changes the output of your command to a table layout, improving the organization of your data.
Syntax and Usage
The basic syntax for using `Format-Table` is as follows:
Format-Table [-Property <String[]>] [-AutoSize] [-Wrap]
Example Command
For instance, if you want to list the currently running processes with their respective CPU usage, you can use the following command:
Get-Process | Format-Table -Property Name, CPU
In this example, `Get-Process` retrieves a list of active processes, and `Format-Table` organizes the output to show only the Name and CPU properties in a neat tabular format.
Specifying Properties and Calculating Fields
One of the powerful features of `Format-Table` is that you can specify which properties to display. This allows you to tailor your output to focus on the most relevant data.
Selecting Specific Properties
To choose which properties to display, you can directly mention them in the `-Property` parameter.
Example Command with Calculated Field
You can also use calculated properties to add more context. For example:
Get-Service | Format-Table -Property Name, Status, @{Name='StartType'; Expression={$_ | Get-Service | Select-Object -ExpandProperty StartType}}
In this example, we are not just listing services but also including their start type as a calculated field.
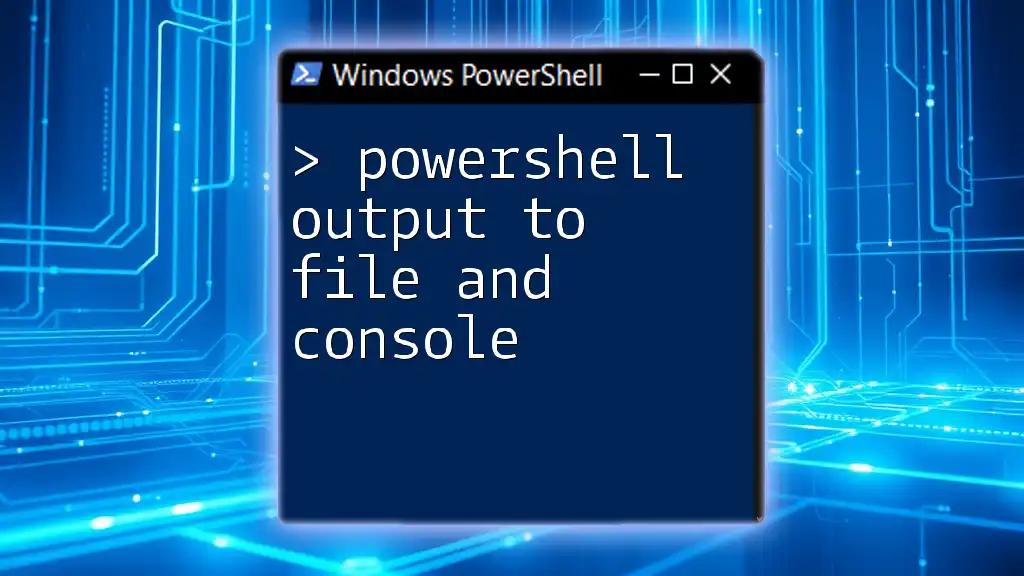
Enhancing Your Table Output
Adding Additional Features with `Format-Table`
Width and Alignment Options
The `Format-Table` cmdlet also allows you to control the display elements such as width and alignment. To ensure that your output looks clean and professional, you might want to set the width of your columns explicitly:
Get-Process | Format-Table Name, CPU -AutoSize
The `-AutoSize` parameter automatically resizes columns for optimal viewing.
Colorizing Output
You can enhance the readability of your tables by applying color. Although PowerShell doesn’t support direct color formatting in traditional table outputs, you can still use other methods to catch the user's eye, such as alert messages or conditions.
Sorting and Paging Output
Sorting with `Sort-Object`
Sorting can significantly improve your ability to analyze data quickly. By combining `Sort-Object` with `Format-Table`, you can prioritize what is most important to you.
Get-Service | Sort-Object Status | Format-Table -AutoSize
This command will organize the services by their status, making it easy to see which ones are running, stopped, or in another state.
Using `Out-Host` for Paging
When your output is too long to fit on a single screen, you can use `Out-Host` to paginate the display. This is useful for reviewing extensive datasets without losing context.
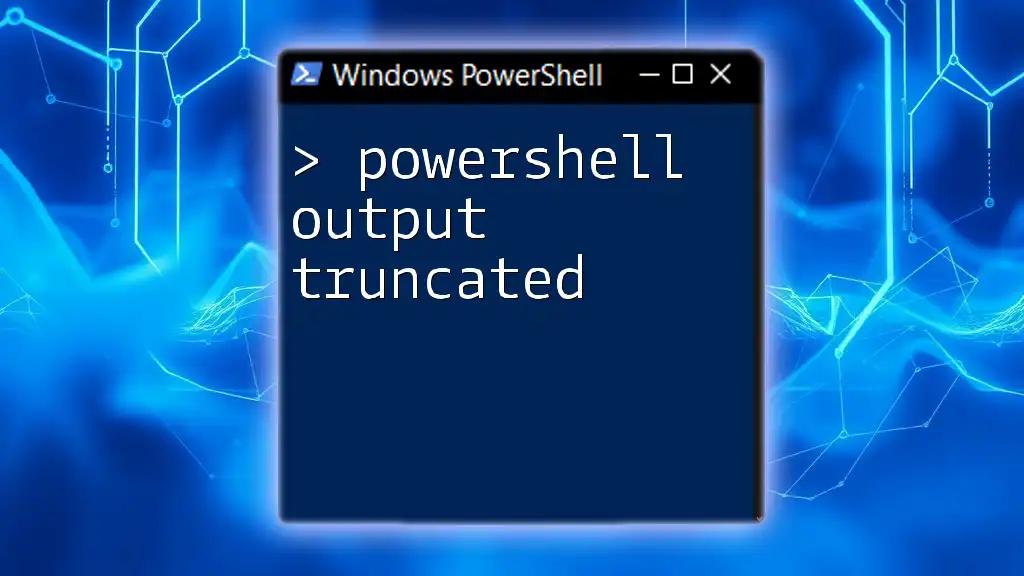
Advanced Techniques
Converting Output to a Table Format
Using `ConvertTo-HTML` for Web Outputs
Sometimes it’s beneficial to present data in web format. You can easily convert your tabular output to HTML, which can be viewed in web browsers:
Get-Process | ConvertTo-Html | Out-File "processes.html"
This command generates an HTML file containing your process data in a table format, making it accessible for reporting or sharing.
Creating CSV Files for External Use
Exporting table output to a CSV file is another handy feature of PowerShell. This allows you to manipulate your data in spreadsheet applications. Here’s an example:
Get-Process | Export-Csv "processes.csv" -NoTypeInformation
This command saves a structured CSV file of your process list, which can be opened and analyzed in various programs.
Using Custom Objects
Creating Custom Objects for Enhanced Tables
To enhance your table display even further, you can create custom PowerShell objects. This option allows for more complex data structures and representations.
Example of Custom Object Creation
Here’s how to create and format a custom object to display in a table:
$customObj = New-Object PSObject -Property @{
Name = "Example Process"
CPU = 1.2
Memory = "512MB"
}
$customObj | Format-Table
This example demonstrates how a custom object can represent unique data while maintaining the ability to format it neatly in a table.
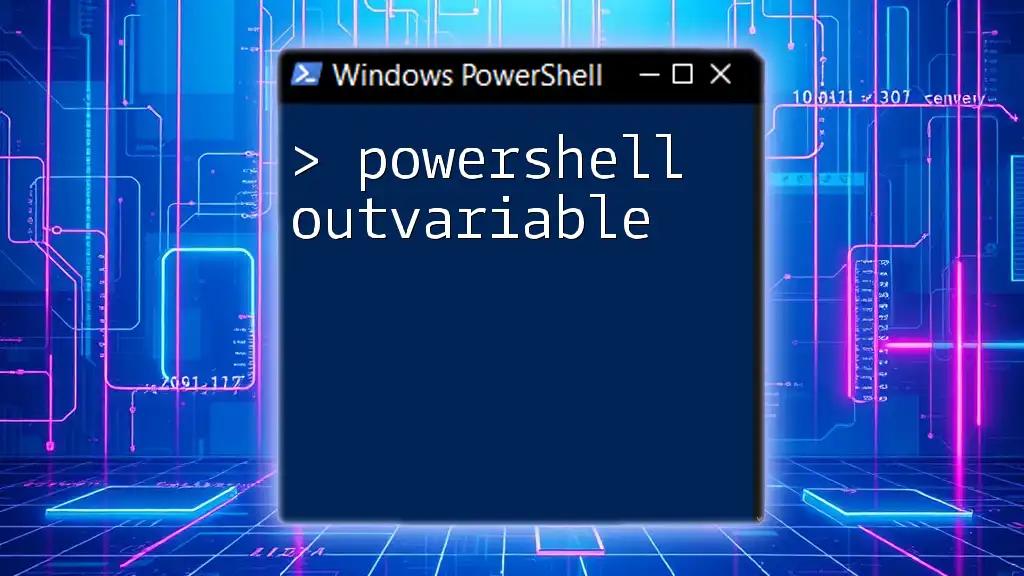
Common Pitfalls & Troubleshooting
Common Formatting Issues
When using `Format-Table`, you might encounter some frequent formatting problems like truncated data or misaligned columns. These can often be remedied by utilizing the `-AutoSize` parameter or by manually adjusting the widths of columns.
Performance Considerations
While it’s great to have extensive table formatting, doing so extensively can impact performance. When scripting for larger datasets, opt for streamlined outputs and minimize the use of complex formatting to maintain script efficiency.
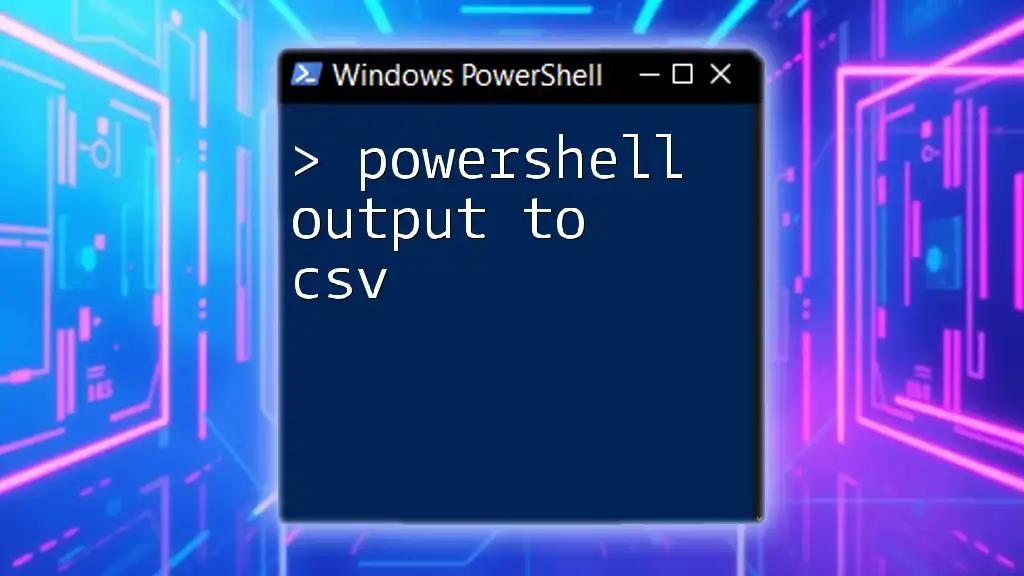
Conclusion
Mastering PowerShell output to table is a vital skill for any PowerShell user. By understanding the concepts and techniques covered in this guide—from basic `Format-Table` usage to advanced custom object creation—you'll be better equipped to present your data clearly and effectively. Regular practice with these commands will help you become proficient, making your PowerShell scripts more user-friendly and informative.