The `ConvertTo-Json` cmdlet in PowerShell is used to convert PowerShell objects into JSON format, making it easy to serialize data for web applications or APIs.
Here’s a code snippet demonstrating its use:
$object = @{ Name = "John"; Age = 30; City = "New York" }
$json = $object | ConvertTo-Json
Write-Host $json
Understanding PowerShell ConvertTo-JSON
ConvertTo-JSON is a cmdlet in PowerShell that transforms PowerShell objects into JSON format. JSON, or JavaScript Object Notation, is a widely used format for serializing structured data, often utilized in web services and RESTful APIs. Understanding how to efficiently use ConvertTo-JSON can significantly enhance your scripting and automation capabilities in PowerShell.
Basic Syntax of ConvertTo-JSON
The basic syntax for the ConvertTo-JSON command is as follows:
ConvertTo-Json [-InputObject] <PSObject> [[-Depth] <Int32>] [-AsArray] [-Compress] [-NoTypeInformation]
It allows various parameters which cater to specific needs, leading to flexible and powerful usage.
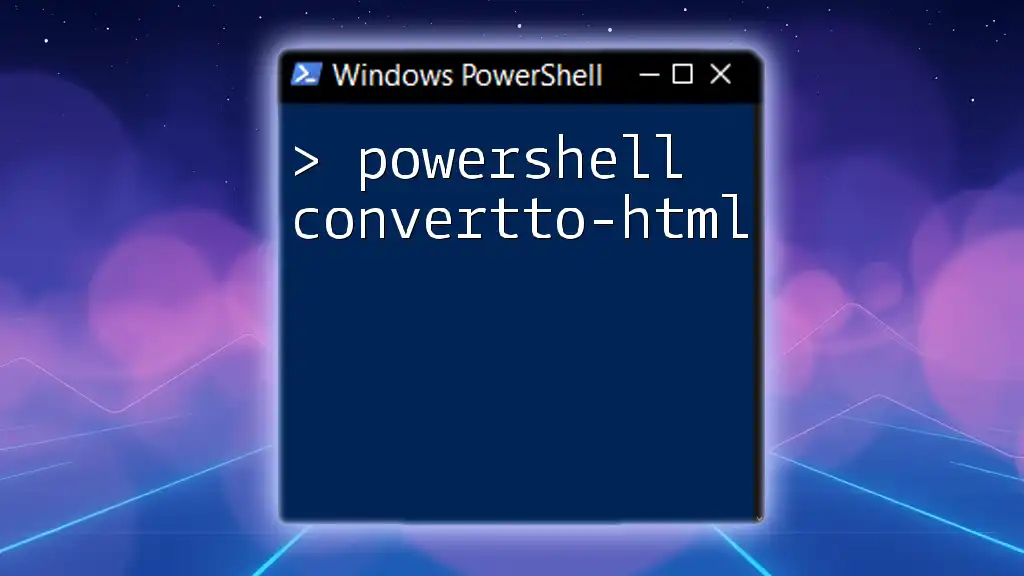
Basic Usage of ConvertTo-JSON
To get started with ConvertTo-JSON, you can begin with a simple PowerShell object. Below is an example demonstrating how to convert a PowerShell hashtable to JSON format.
Let's say we want to represent a person’s details:
$person = @{
Name = "John Doe"
Age = 30
City = "New York"
}
$json = $person | ConvertTo-Json
Write-Output $json
The output will be a JSON string representing the `person` object, similar to this:
{
"Name": "John Doe",
"Age": 30,
"City": "New York"
}
This straightforward conversion showcases how ConvertTo-JSON effortlessly handles basic data structures.
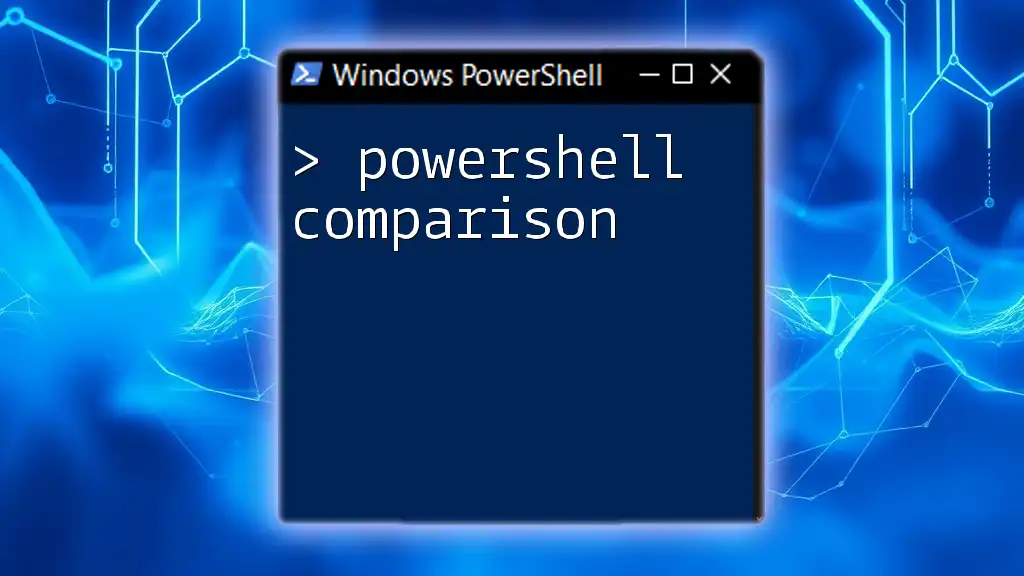
Advanced Options and Parameters
Using the Depth Parameter
One of the most useful parameters of ConvertTo-JSON is the -Depth parameter. This becomes crucial when working with nested objects, allowing you to specify how many levels deep the conversion should go.
Consider the following example with a nested hashtable:
$data = @{
Name = "Jane Smith"
Address = @{
Street = "123 Main St"
City = "Los Angeles"
State = "CA"
}
}
$json = $data | ConvertTo-Json -Depth 3
Write-Output $json
With the -Depth 3 set, the JSON will correctly represent all properties of the `Address` object. Without adequately setting the depth, certain nested properties may be omitted, resulting in incomplete data.
Using the AsArray Parameter
The -AsArray parameter is particularly useful when converting collections or arrays of objects. If you apply this parameter, PowerShell will treat the input as an array.
Here's an example using an array of people:
$people = @(
@{ Name = "Alice"; Age = 25 },
@{ Name = "Bob"; Age = 28 }
)
$json = $people | ConvertTo-Json -AsArray
Write-Output $json
The resulting JSON will take the form of an array, making it suitable for APIs that expect JSON arrays:
[
{
"Name": "Alice",
"Age": 25
},
{
"Name": "Bob",
"Age": 28
}
]
Using the Compress Option
The -Compress option minimizes whitespace in the output, resulting in a more compact JSON representation. This can be beneficial when you want to reduce the size of the output for transmission or storage.
For example:
$options = @{
Name = "Compressed Data"
Description = "This is a test"
}
$json = $options | ConvertTo-Json -Compress
Write-Output $json
The output is a neatly compressed JSON string:
{"Name":"Compressed Data","Description":"This is a test"}
Using NoTypeInformation
The -NoTypeInformation parameter prevents type information from being included in the output, a common requirement when generating JSON for APIs, making it cleaner.
An example implementation would be:
$product = @{
Name = "Gadget"
Price = 19.99
}
$json = $product | ConvertTo-Json -NoTypeInformation
Write-Output $json
This will yield JSON without including any type data:
{"Name":"Gadget","Price":19.99}
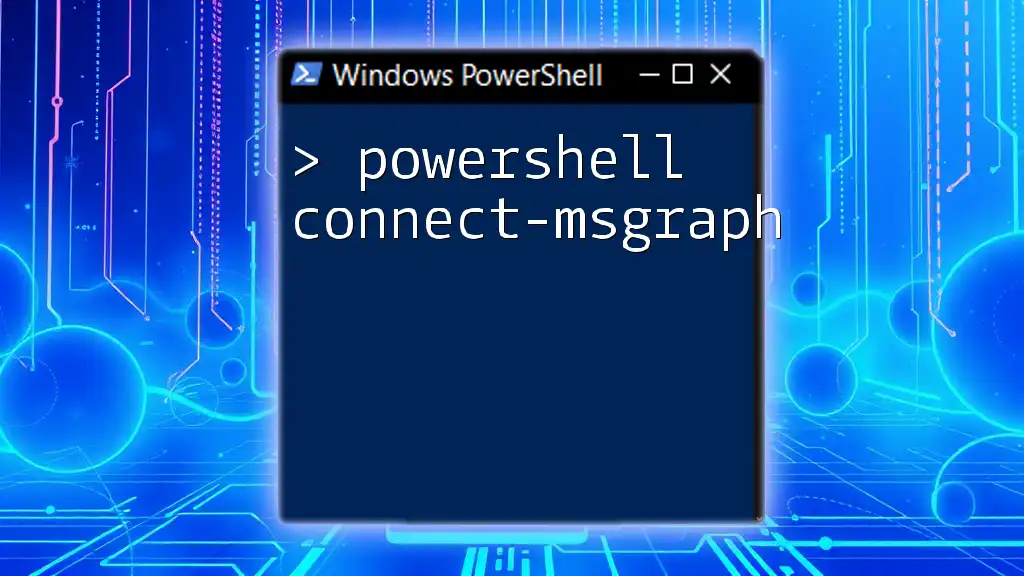
Common Use Cases for ConvertTo-JSON
Exporting Data for APIs
One common scenario for using ConvertTo-JSON is exporting data to be sent to APIs. Many web services accept data in JSON format, making this cmdlet invaluable for web automation tasks or when interfacing with RESTful services.
Serialization for Configuration Files
JSON is also a popular format for configuration files. Using ConvertTo-JSON, you can serialize your application settings into a JSON string for easier storage and readability.
Interfacing with JavaScript and Web Development
Web developers often need to exchange data between back-end services (like those built with PowerShell) and front-end applications (like those written in JavaScript). Knowing how to use ConvertTo-JSON can greatly facilitate this data interchange.
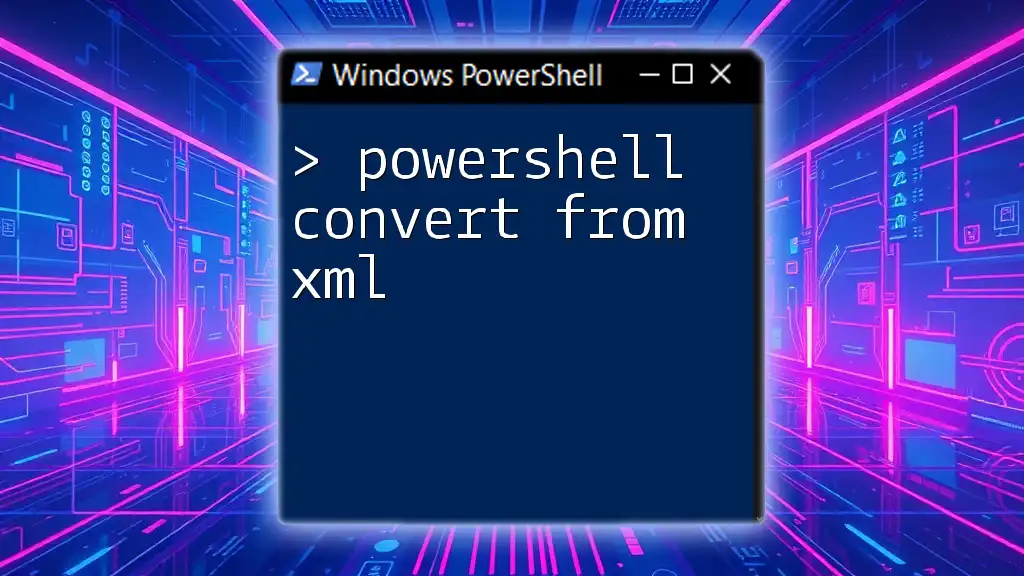
Troubleshooting Common Issues
Common Errors and Fixes
While using ConvertTo-JSON, you may encounter errors, especially with complex objects. It's pivotal to ensure that your input object is compatible with conversion to JSON format. Common pitfalls include circular references and unsupported data types.
Performance Considerations
When handling large datasets, keep in mind that ConvertTo-JSON may lead to performance issues. For optimal performance, consider the structure of your data and utilize the -Depth and -Compress parameters judiciously.
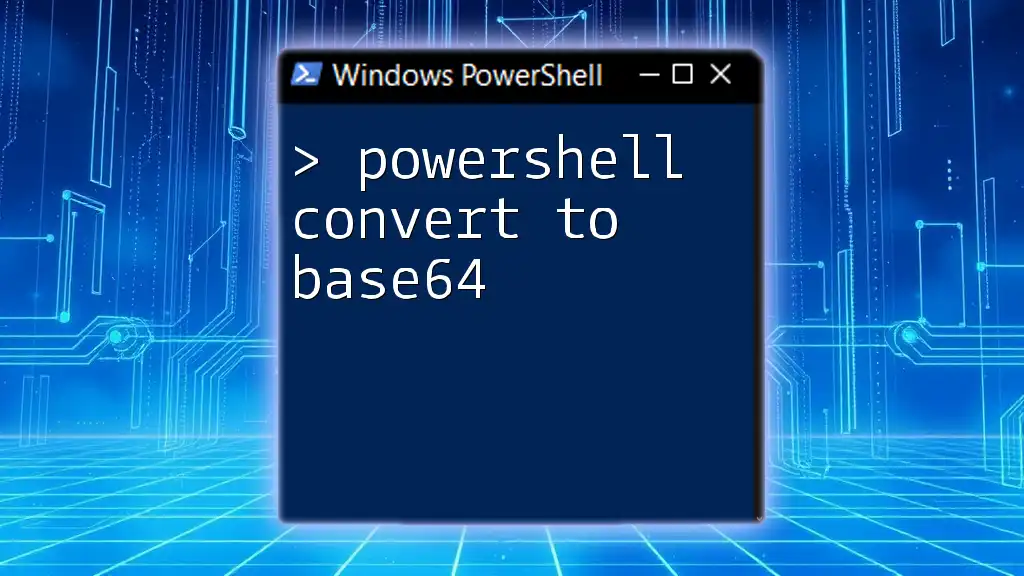
Conclusion
Mastering the PowerShell ConvertTo-JSON cmdlet can significantly enhance your scripting ability. From converting simple objects to handling deeper, complex hierarchies, this cmdlet is versatile and powerful.
Feel encouraged to experiment with your own scripts, and as you delve deeper into PowerShell, particularly the ConvertTo-JSON command, you'll find that it opens up new avenues for handling and manipulating data efficiently.
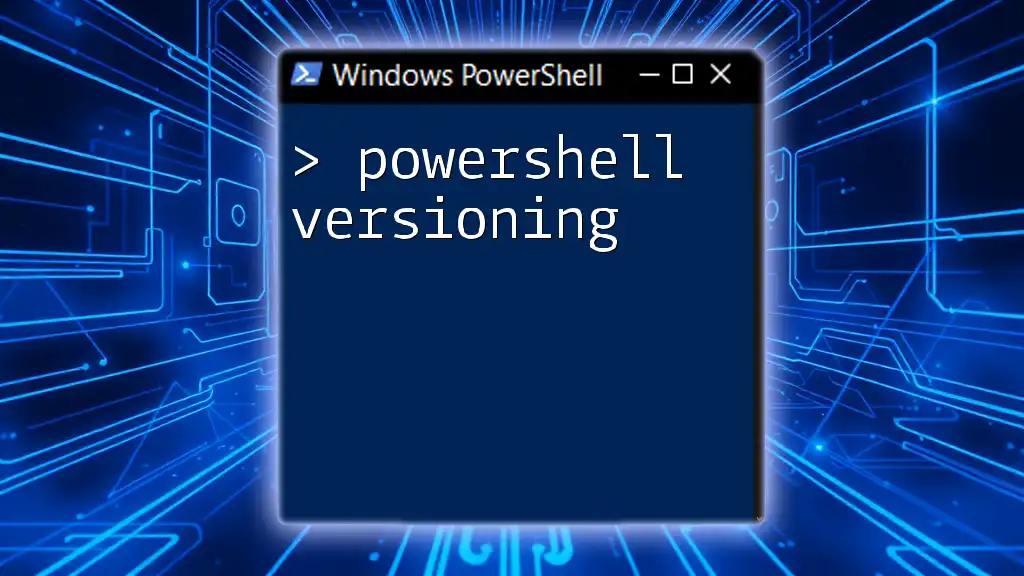
Additional Resources
For further reading and detailed command explanations, consider checking the official Microsoft documentation for ConvertTo-JSON. Additionally, exploring books and online courses can provide deeper insights into harnessing the full potential of PowerShell.
Bonus: Community Input
We invite you to engage with us by sharing your experiences with using ConvertTo-JSON in your projects or by asking any questions that might arise as you work with this cmdlet. Your insights could not only help you but also assist fellow learners in the PowerShell community!