PowerShell can export data to Excel using the `Export-Excel` cmdlet, which allows you to easily create and manipulate Excel files directly from your PowerShell scripts.
Here’s a basic code snippet to accomplish this:
# Install the ImportExcel module if you haven't already
Install-Module -Name ImportExcel
# Export data to an Excel file
Get-Process | Export-Excel -Path 'C:\path\to\your\file.xlsx'
Understanding PowerShell and Excel Integration
What You Need to Know About PowerShell Objects
PowerShell operates on objects rather than text. Each command output in PowerShell is an object that contains properties and methods. This means when you are working with data, you are manipulating and exporting structured data formats, which are easily transferable to formats like CSV or Excel. Understanding this concept is vital for effectively using PowerShell for data export.
Prerequisites for Exporting Data to Excel
Before diving into the methods for exporting data, ensure you have the necessary tools:
- Install the required modules, such as ImportExcel, which allows direct exporting to Excel without needing Excel installed on your system.
- Confirm that Excel or a compatible spreadsheet program is installed if you are planning to open Excel files generated from your PowerShell commands.
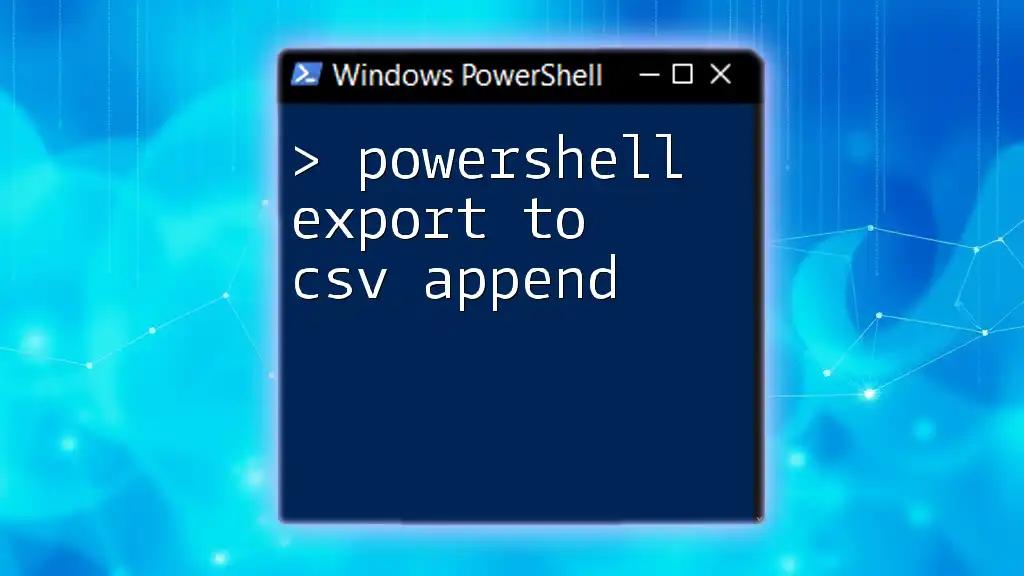
Exporting to Excel Using PowerShell
Exporting Data to CSV as an Intermediate Step
A straightforward way to export data using PowerShell is to first send it to a CSV file. This method is often used for quick exports.
Simple PowerShell Commands to Export to CSV
Using the `Export-Csv` cmdlet, you can easily export data. Here’s an example:
Get-Process | Export-Csv -Path "C:\Processes.csv" -NoTypeInformation
This command retrieves all currently running processes and writes them to a CSV file named `Processes.csv`. The `-NoTypeInformation` parameter prevents type information from being included at the top of the CSV file, which keeps it clean and straightforward.
Importing the CSV in Excel
Once you’ve exported your data to a CSV file, you can easily open it in Excel by navigating to the file location and double-clicking it. Excel will parse the CSV format and display the data in tabular form.
Direct Export to Excel Using the ImportExcel Module
What is the ImportExcel Module?
The ImportExcel module is a powerful tool that enables the ability to work with Excel files directly. It allows for easy reading and writing of Excel files without requiring Excel to be installed on the machine running the scripts.
Steps to Install the ImportExcel Module
To start using ImportExcel, you need to install it if you haven't already done so. You can easily do this with the following command:
Install-Module -Name ImportExcel -Force
Basic Export to Excel
Now that you have the ImportExcel module installed, you can begin exporting data directly to Excel.
Exporting a PowerShell Command Result to Excel
Here's a straightforward example of how to export data to an Excel file:
Get-Service | Export-Excel -Path "C:\Services.xlsx" -AutoSize
This command retrieves the list of services on your computer and exports them to an Excel file named `Services.xlsx`. The `-AutoSize` parameter ensures that the columns in the Excel sheet adjust to fit the data properly.
Advanced Export Options
Formatting the Excel Output
You can enhance the readability of your Excel export by applying various formatting options. For example, you can make the header bold, which can be done using the following command:
Get-Process | Export-Excel -Path "C:\Processes.xlsx" -AutoSize -BoldTopRow
This command exports the data just like before but makes the top row (headers) bold for better visibility.
Adding Multiple Worksheets and Charts
One of the advantages of exporting to Excel is the ability to create complex reports. You can create multiple worksheets or even add charts based on your data.
To export data to a specified worksheet within the same Excel file, you can do:
$data = Get-Process
$data | Export-Excel -Path "C:\ProcessReport.xlsx" -WorksheetName "Processes"
You can then add charts to visualize this data. Here’s how to set up a simple chart:
$excelPkg = Open-ExcelPackage -Path "C:\ProcessReport.xlsx"
$chart = $excelPkg.Workbook.Worksheets["Processes"].Drawing.AddChart("Chart1", "ColumnClustered")
This snippet creates a new column chart called "Chart1" within the "Processes" worksheet, facilitating graphic representation of your data.
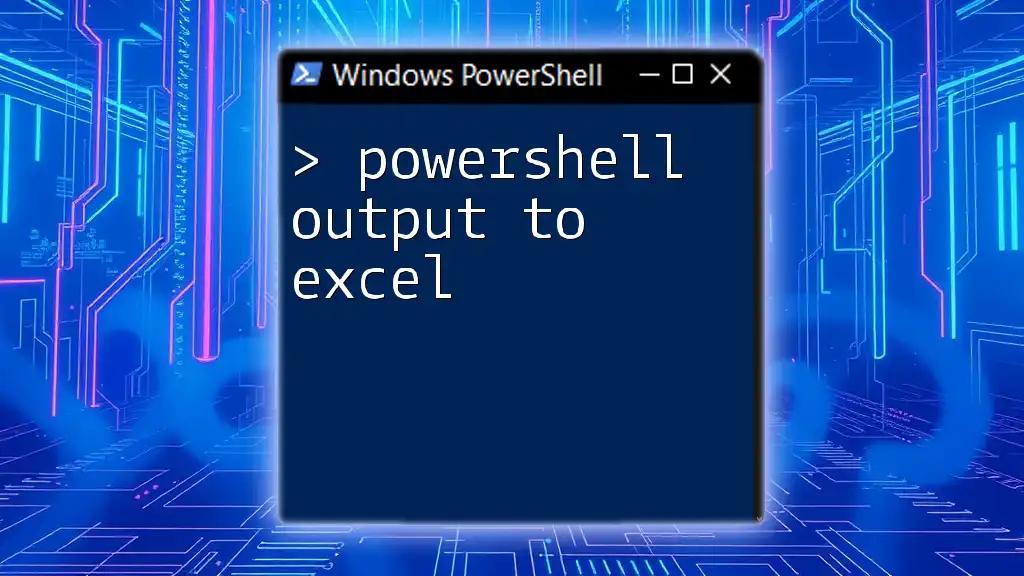
Automating the Export Process
Using Scheduled Tasks for Regular Data Export
PowerShell scripting shines when automating repetitive tasks. For regular exports, use Windows Task Scheduler:
- Create a PowerShell script with your export commands.
- Schedule the script using Task Scheduler to run at designated intervals, ensuring you always have the latest data exported without manual intervention.
Sending Exported Files via Email Automatically
To extend your automation further, you can email your exported Excel files directly. Use the `Send-MailMessage` cmdlet for this:
Send-MailMessage -From "you@example.com" -To "recipient@example.com" -Subject "Weekly Report" -Body "Please find the attached report." -Attachments "C:\ProcessReport.xlsx"
This command sends your Excel report as an email attachment, providing a seamless way to share updates.
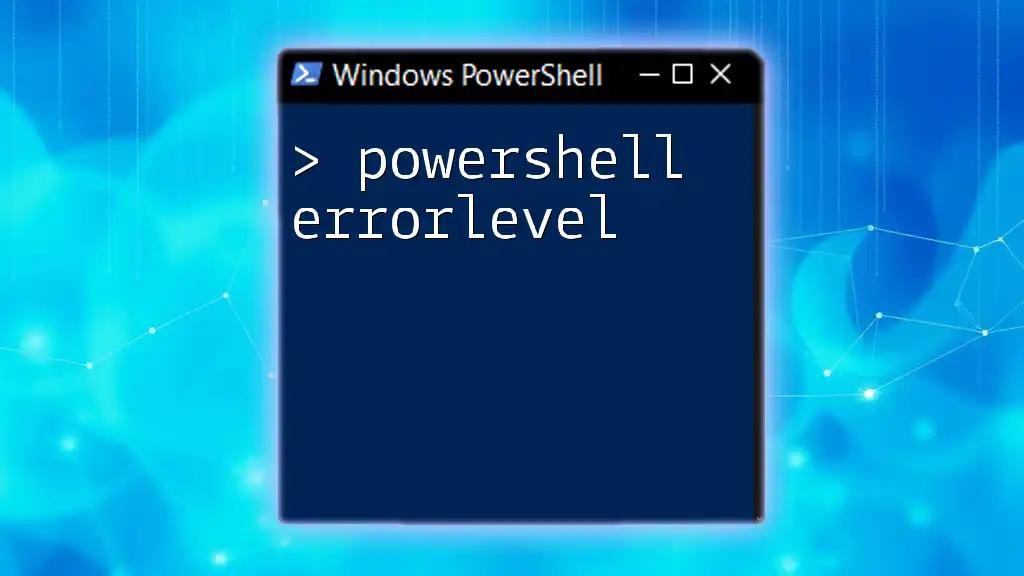
Common Issues and Troubleshooting
Common Errors During Export
When exporting data, you might encounter errors, often related to permissions or file paths. Always ensure you run PowerShell as an administrator and specify valid paths to avoid common pitfalls.
Excel Formatting Issues
After exporting, if the data appears misaligned or improperly formatted in Excel, check your PowerShell commands and the properties being exported. Format strings and data types play a critical role in how data renders in Excel.
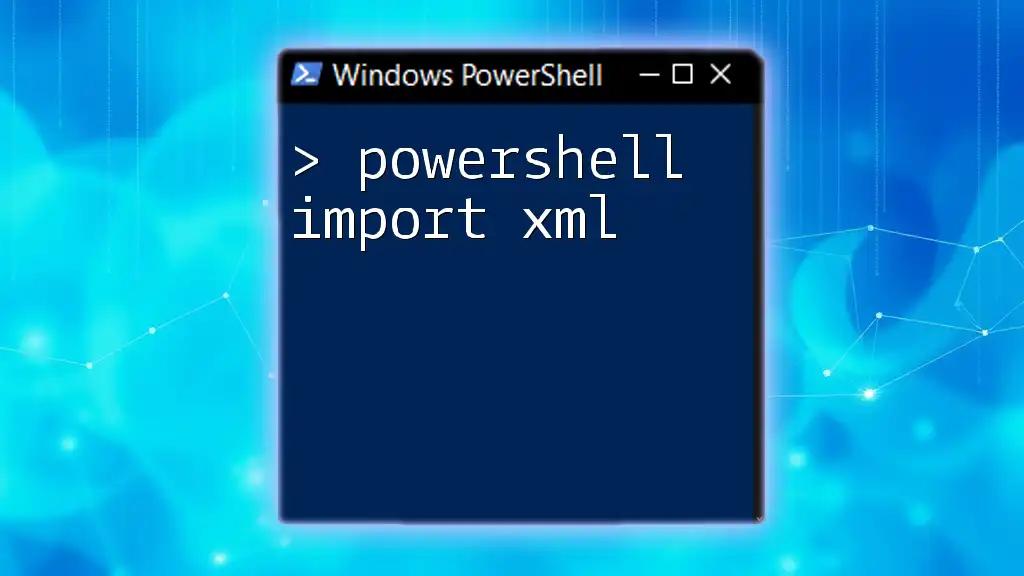
Best Practices for Exporting Data to Excel
Efficient Data Handling Tips
To maintain efficiency, filter your data using PowerShell's capabilities before exporting. This ensures that only relevant data goes into your Excel files, keeping the files manageable and easier to analyze.
Maintaining Data Integrity
When exporting, it's crucial to maintain data integrity. Ensure that data types are correctly interpreted, and use proper formatting to prevent data loss, especially with dates and numbers.
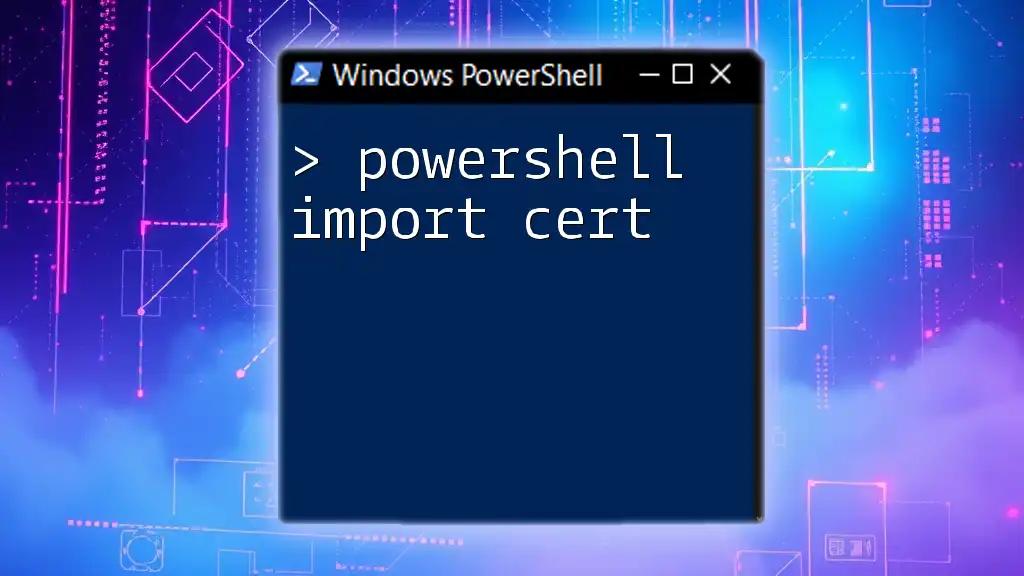
Conclusion
To wrap up, mastering the PowerShell export to Excel process enhances your ability to manipulate and share data effectively. By understanding how to export data to Excel directly or through CSV, utilizing the powerful features of the ImportExcel module, automating tasks, and sending reports via email, you can streamline your reporting process significantly.
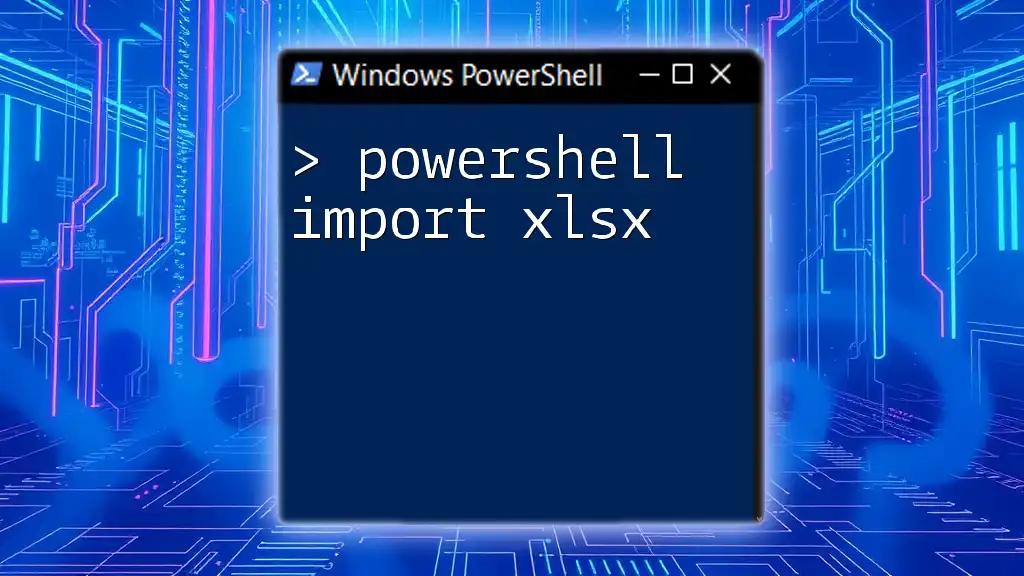
Call to Action
Engage with this material, share your experiences with PowerShell, and feel free to reach out with questions. If you're looking to deepen your PowerShell knowledge further, explore the courses and tutorials we offer to elevate your skills in automation and scripting.