In PowerShell, you can append text to a file using the `Write-Output` cmdlet redirected with `>>`, allowing you to add new content without overwriting the existing file.
Write-Output 'Hello, World!' >> C:\path\to\your\file.txt
Understanding Write-Output
What is Write-Output?
`Write-Output` is a fundamental command in PowerShell that sends the output of a command to the pipeline. Unlike `Write-Host`, which outputs directly to the console, `Write-Output` allows data to be streamed to the next command or can be captured for further use, making it a versatile tool for scripting and automation.
Syntax of Write-Output
The basic syntax for `Write-Output` is straightforward. You can simply use:
Write-Output "Your message here"
This command will display "Your message here" in the console, and if piped to another command, it will pass that message for further processing.
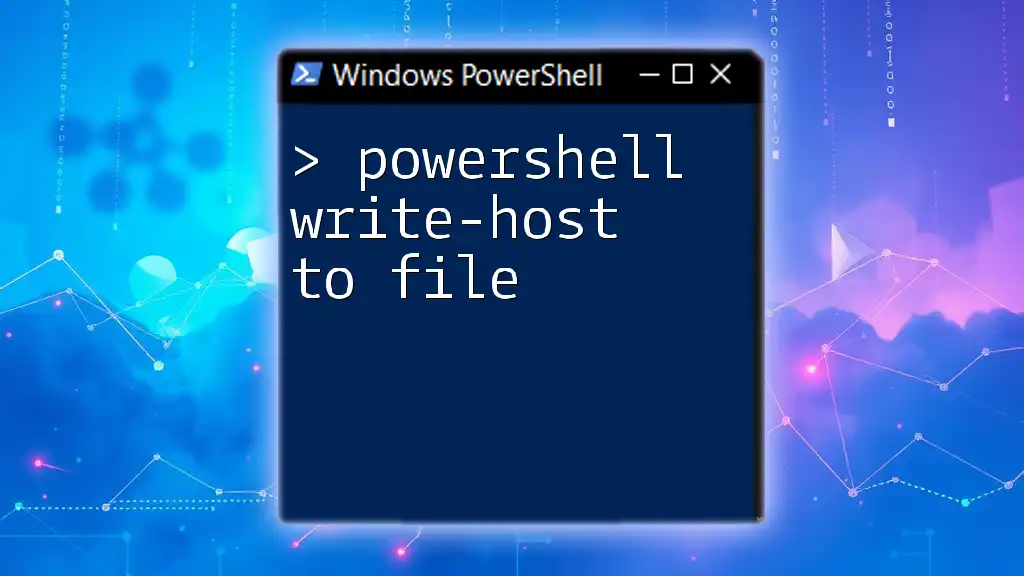
Writing to Files in PowerShell
Introduction to File Handling
PowerShell provides robust methods for handling files that allow users to create, read, write, and manage files efficiently. The most common cmdlets utilized for file writing are:
- `Out-File`
- `Set-Content`
- `Add-Content`
Understanding these commands is essential for effective file manipulation.
The Role of Append Mode
Appending data to a file means adding new content without deleting or replacing existing content. This mode is particularly useful for log files, records, or any scenario where conserving existing information is crucial. In contrast, overwriting a file would discard previous contents entirely.
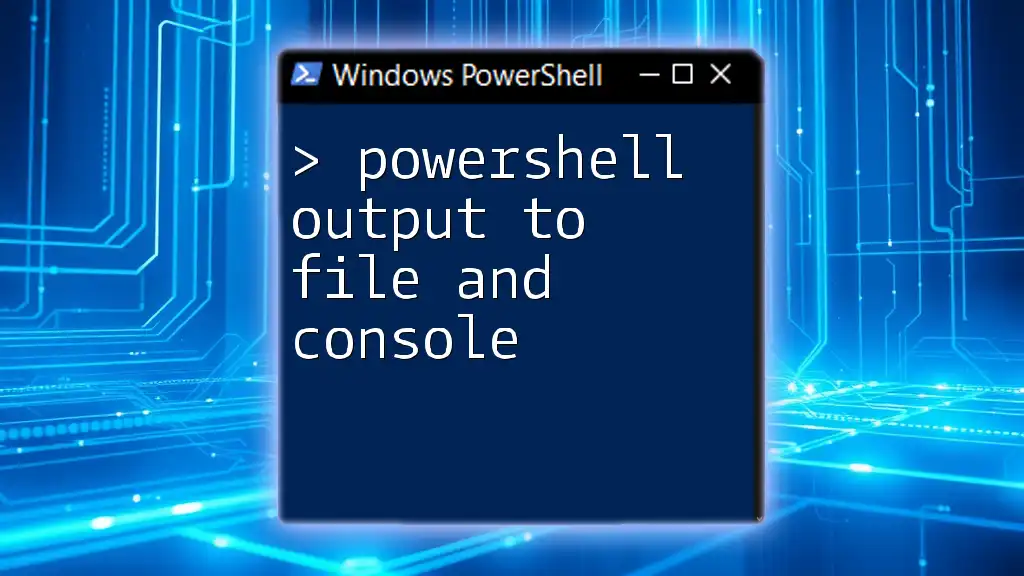
Using Write-Output to Append to Files
Using Write-Output with Out-File
To append data to a file using `Write-Output` in conjunction with `Out-File`, you'll make use of the `-Append` switch. The command structure is as follows:
Write-Output "This is the new line." | Out-File -FilePath "C:\path\to\your\file.txt" -Append
In this example, the text "This is the new line." is added to `file.txt` without removing any existing content.
Using Write-Output with Add-Content
Another method of appending data is through `Add-Content`, which is explicitly designed for this purpose. The command would look like:
Write-Output "This is the new line." | Add-Content -Path "C:\path\to\your\file.txt"
This command achieves the same result, appending the specified text to the existing file content.
Differences Between Out-File and Add-Content
While both `Out-File` and `Add-Content` allow you to append data, they have different applications.
- `Out-File` is more suited for redirecting output from commands and may include additional formatting options.
- `Add-Content` is typically more straightforward for simple text appending tasks.
In scenarios where performance is crucial or extensive use is anticipated, consider using `Add-Content`, as it's optimized for that purpose.
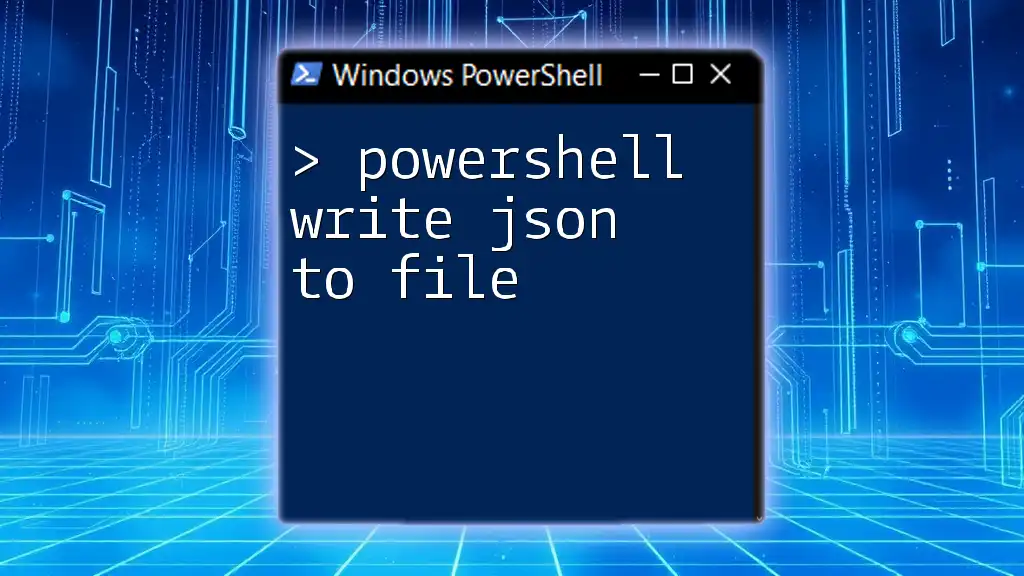
Practical Use Cases
Logging Events
Creating a simple logging mechanism is a common use case for appending data. For instance, you can log event timestamps and messages:
$timestamp = Get-Date -Format "yyyy-MM-dd HH:mm:ss"
Write-Output "[$timestamp] Event started." | Add-Content -Path "C:\path\to\your\logfile.txt"
This approach allows you to maintain a running log of events with timestamps, which can be crucial for debugging and tracking actions within your scripts.
Exporting Data from Cmdlets
You can also use `Write-Output` to export data from various cmdlets. For example, if you want to log all active processes:
Get-Process | Write-Output | Out-File -FilePath "C:\processes.txt" -Append
This command collects the current processes and appends them to `processes.txt`, preserving all previous entries.
Batch File Updates
Appending configurations to files can also be efficiently handled. For instance, if you have a configuration file, you could append a new setting:
Write-Output "NewSetting=Enabled" | Add-Content -Path "C:\path\to\your\config.txt"
This way, the configuration file continuously evolves without loss of previous settings.
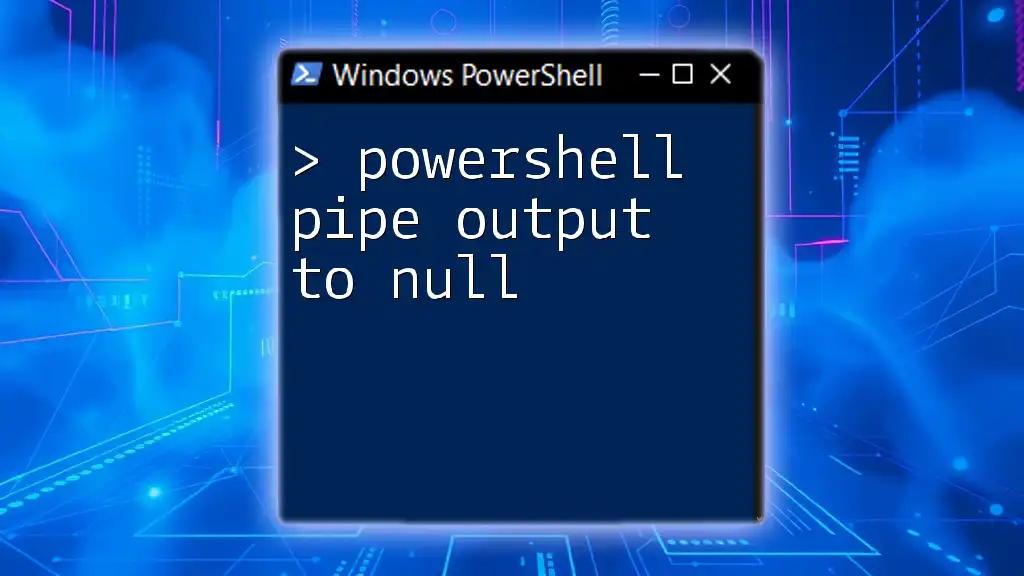
Handling Errors and Exceptions
Common Errors
When attempting to append to files, you may encounter issues, particularly around file permissions or file access. Ensure you have the necessary permissions to modify the file, or you may receive error messages indicating access denied — something crucial to check in enterprise environments.
Using Try-Catch Blocks
To effectively manage errors, implement Try-Catch blocks around your append operations. This can help catch any exceptions and respond accordingly:
try {
Write-Output "This is the new line." | Add-Content -Path "C:\path\to\your\file.txt"
} catch {
Write-Host "An error occurred: $_"
}
By using this structure, you can handle unexpected issues, improving the resilience of your scripts.
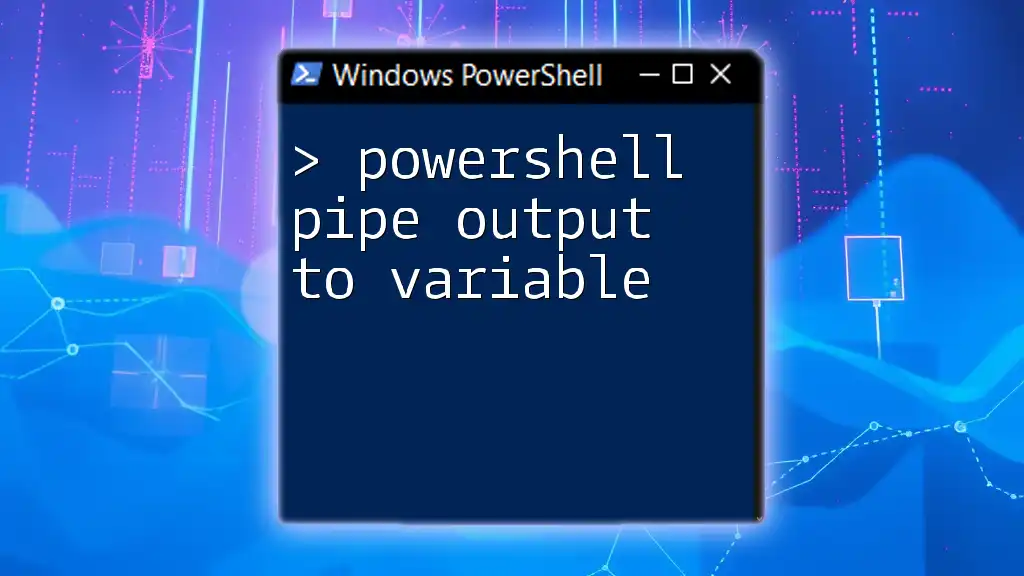
Summary
In summary, using PowerShell write-output to file append allows users to enhance their file management capabilities effectively. By understanding the differences between various commands, the append mode’s importance, and applying practical use cases, you can streamline your data processing and logging tasks in PowerShell. Experimenting with these methods will help you unlock the full potential of PowerShell in your workflow.
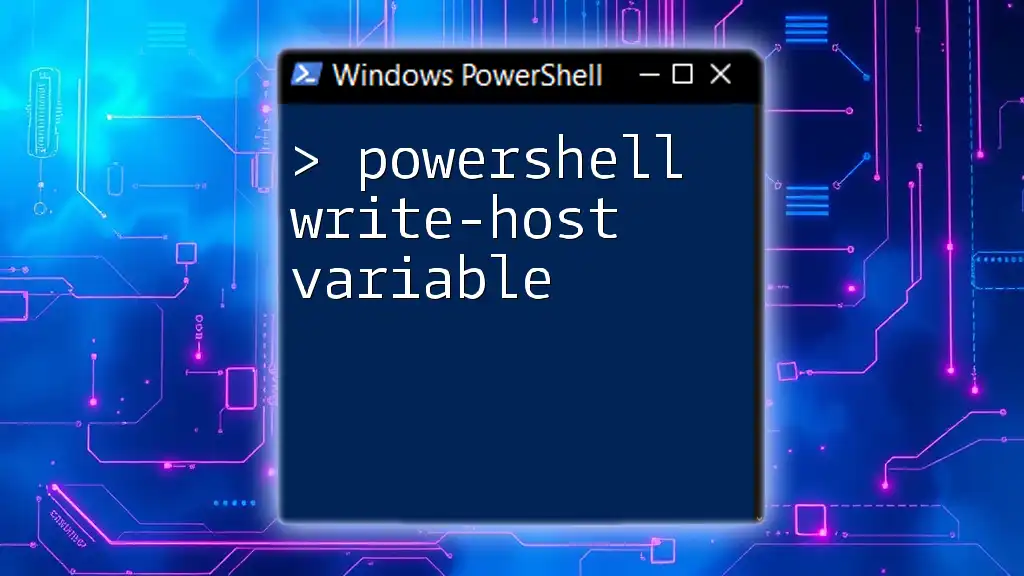
Additional Resources
For further reading and practice, consider exploring the official PowerShell documentation, community forums, or tutorials that focus on mastering file management in PowerShell. Engaging with these resources will deepen your understanding and proficiency in using PowerShell effectively.
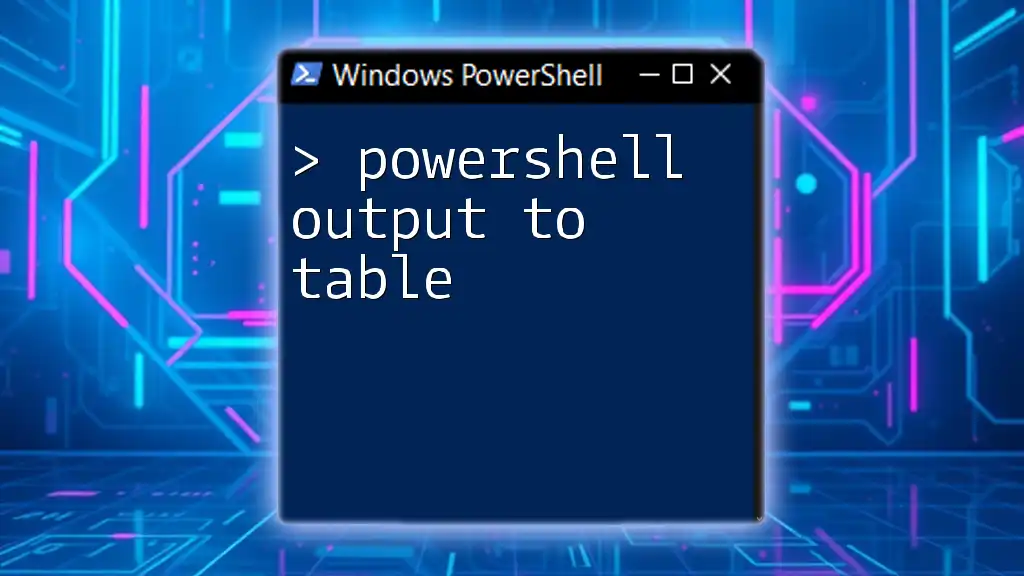
Call to Action
Try appending your own data to files using PowerShell and share your experiences or questions in the comments section or on social media. Your journey into mastering PowerShell starts here!