The `Write-Progress` cmdlet in PowerShell provides a visual indication of the progress of a script or operation by displaying a progress bar to the user, enhancing the user experience during longer-running tasks.
Here’s a simple example of how to use `Write-Progress`:
for ($i = 1; $i -le 10; $i++) {
Write-Progress -PercentComplete ($i * 10) -Status "Processing item $i" -CurrentOperation "Working..."
Start-Sleep -Seconds 1 # Simulate work
}
Understanding Write-Progress
What is Write-Progress?
`Write-Progress` is a cmdlet designed to provide real-time feedback to users when executing long-running PowerShell scripts or commands. By visually indicating the progress of an ongoing task, `Write-Progress` enhances user interaction and awareness, making it particularly useful in scenarios where scripts may take a significant amount of time to complete.
Key Benefits of Using Write-Progress
Incorporating `Write-Progress` into your scripts brings several advantages:
- Improved User Feedback: By displaying a progress bar, users can see how far along a task is, which reduces uncertainty.
- Enhanced User Experience: Users appreciate knowing that something is happening, especially during extensive operations.
- Anxiety Reduction: The visual indicators alleviate concerns regarding whether a script is still running, thereby improving the overall user experience.
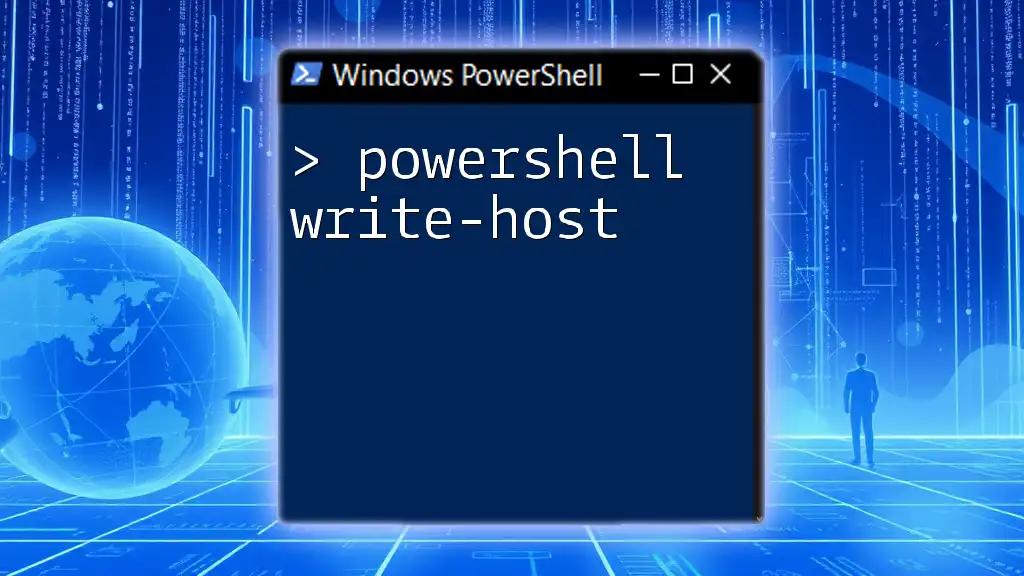
Basic Syntax of Write-Progress
Syntax Structure
The general syntax for the `Write-Progress` cmdlet is as follows:
Write-Progress -Activity <string> -Status <string> -PercentComplete <int>
Parameters Explained
- -Activity: This parameter describes the overall task being performed (e.g., "Processing Files").
- -Status: This provides additional detail about the current operation or status (e.g., "50% Complete").
- -PercentComplete: This value should range from 0 to 100, indicating how much of the task is complete.
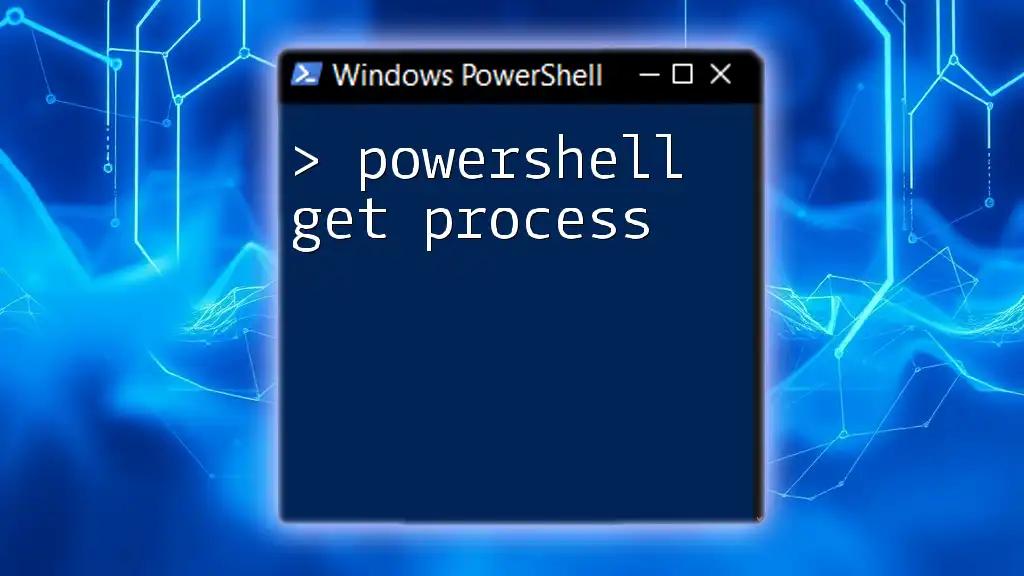
Practical Examples of Write-Progress
Example 1: Simple Progress Display
In the simplest form, you can use `Write-Progress` within a loop to indicate the progress of a task:
for ($i = 0; $i -le 100; $i++) {
Write-Progress -Activity "Processing Files" -Status "$i% Complete" -PercentComplete $i
Start-Sleep -Milliseconds 50
}
In this example, for every iteration, the progress bar updates to show the percentage of completion. The `Start-Sleep` cmdlet simulates a delay, emulating a long-running process. This approach not only communicates the progress to the user but also keeps them engaged during execution.
Example 2: Integrating with Long-Running Tasks
Using Write-Progress with File Operations
Let’s consider a practical scenario where you're copying files. Here’s how you would track the progress:
$files = Get-ChildItem "C:\Files"
$totalFiles = $files.Count
for ($i = 0; $i -lt $totalFiles; $i++) {
Write-Progress -Activity "Copying Files" -Status "Copying: $($files[$i].Name)" -PercentComplete (($i / $totalFiles) * 100)
Copy-Item $files[$i].FullName "D:\Backup"
}
In this example, the script retrieves all files from a specified directory and copies them to another location. The use of `Write-Progress` here gives users constant updates on which file is currently being copied and how much of the copying task is complete.
Example 3: Complex Scripts with Multiple Progress Bars
For scripts that process multiple tasks, `Write-Progress` can display multiple progress indicators sequentially:
$tasks = @("Task1", "Task2", "Task3")
$totalTasks = $tasks.Count
foreach ($task in $tasks) {
for ($i = 0; $i -le 100; $i++) {
Write-Progress -Activity $task -Status "Processing..." -PercentComplete $i
Start-Sleep -Milliseconds 20
}
}
Here, each task in the `$tasks` array gets a progress display as it processes. This allows users to visibly track the progress of different tasks in a linear sequence, providing a clearer picture of overall script performance.
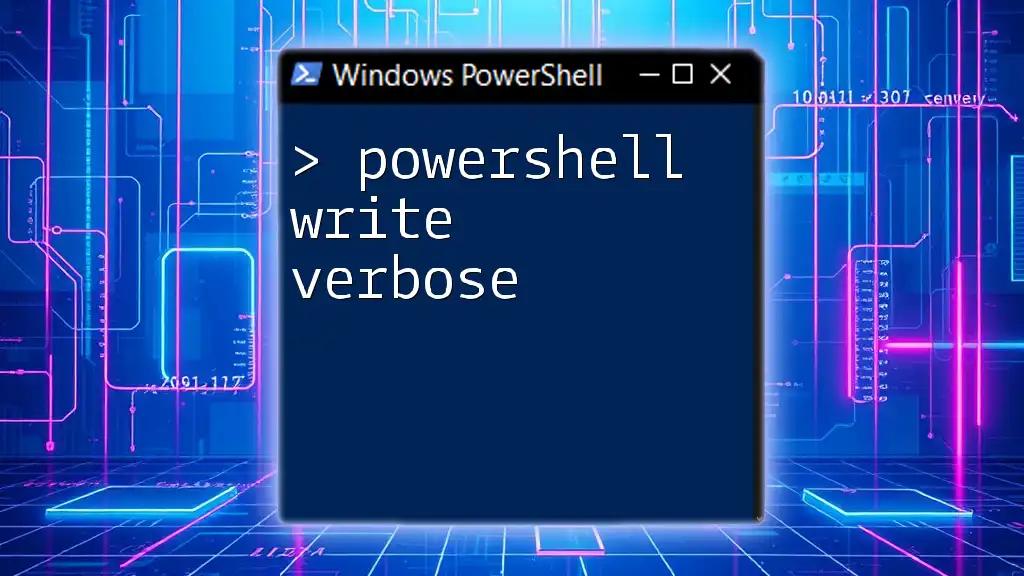
Advanced Use Cases for Write-Progress
Customizing Progress Bars
You can further enhance the utility of `Write-Progress` by customizing and styling the progress bars. This could involve using additional parameters like `-CurrentOperation` and `-Id` to create distinct progress indicators for various scripts running simultaneously.
Handling Errors with Write-Progress
It’s essential to manage errors while also informing users about ongoing processes. Consider the following example:
try {
Write-Progress -Activity "Data Import" -Status "Beginning import..." -PercentComplete 0
# Simulate data processing here
Throw "Simulated Error"
} catch {
Write-Progress -Activity "Data Import" -Status "Error: $_" -PercentComplete 0
}
In this snippet, a simulated error is triggered during a data import operation. Instead of leaving users in the dark, the `Write-Progress` cmdlet maintains communication, letting them know that an error occurred.
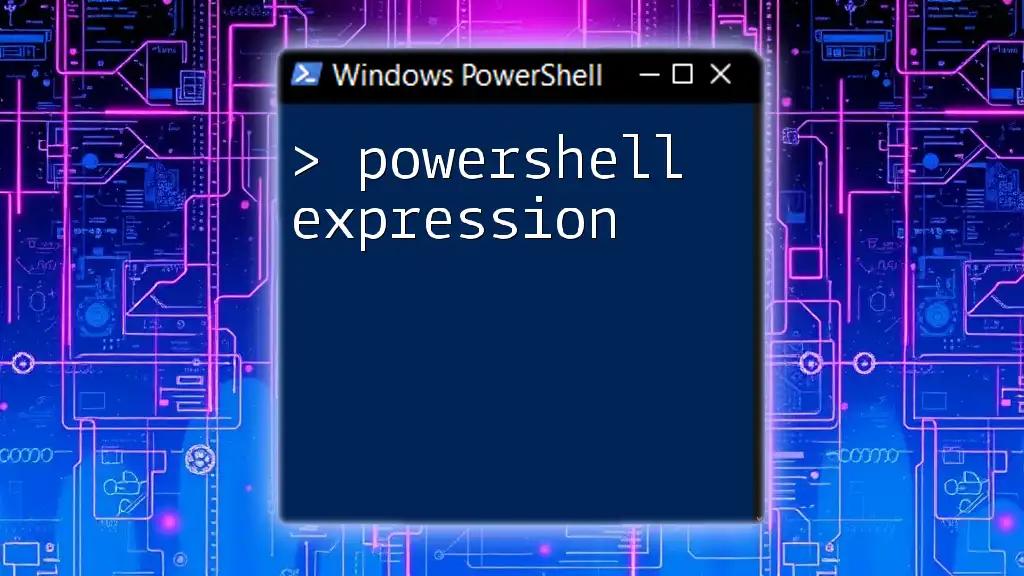
Best Practices for Using Write-Progress
When to Use Write-Progress
Apply `Write-Progress` in scenarios involving lengthy or complex operations. Examples include file transfers, data imports, or any time-consuming tasks where user feedback is beneficial.
Common Pitfalls to Avoid
Ensure that you don't overuse progress indicators. If everything in your script provides a progress update, it may overwhelm users instead of helping. Also, remember to reset progress at the end of operations to avoid confusing users.
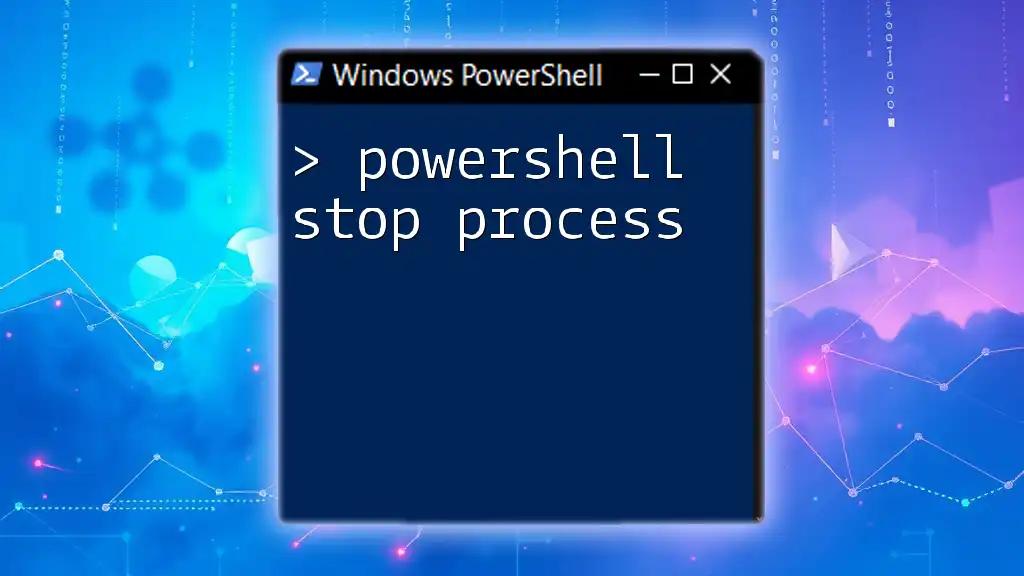
Conclusion
`PowerShell Write-Progress` serves as a powerful tool for enhancing user experience during script execution. By providing visible feedback on task completion, it keeps users informed and engaged. Whether you're working on simple scripts or complex operations, implementing `Write-Progress` will undoubtedly add value to your PowerShell applications.
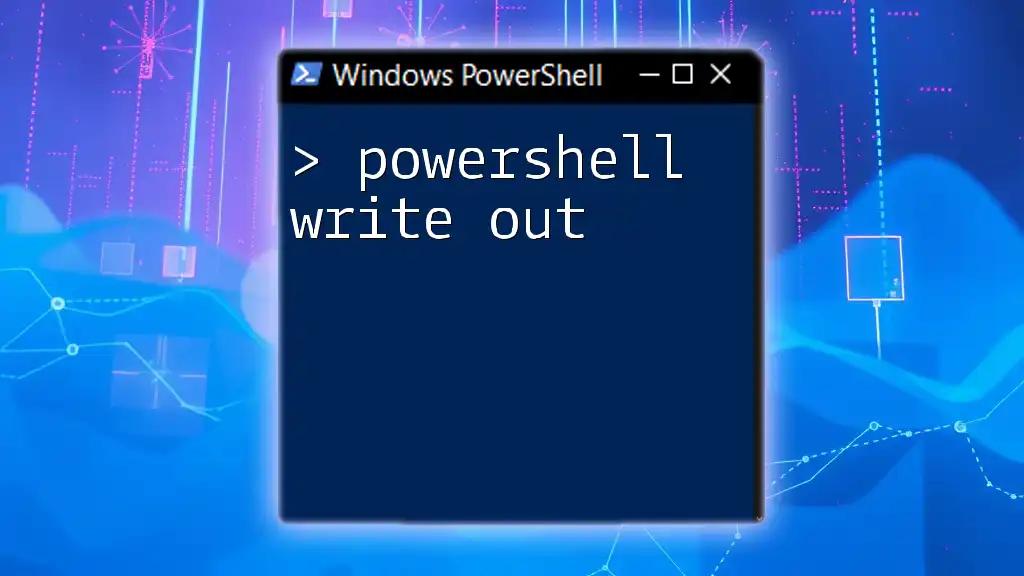
Additional Resources
For further information, you can consult the official PowerShell documentation, explore tutorials, and seek advanced readings that delve deeper into mastering PowerShell capabilities.