In PowerShell, the `Write-Out` command is utilized to display output or messages to the console, allowing users to communicate results or status updates effectively.
Here’s a code snippet demonstrating its usage:
Write-Host 'Hello, World!'
Understanding Write-Output
What is Write-Output?
`Write-Output` is a core cmdlet in PowerShell designed to send output to the pipeline. By using `Write-Output`, users can return data from commands, scripts, and functions, making it an essential tool for managing and manipulating data within scripts. In PowerShell, if you just type an expression or a variable, it implicitly sends that data to the pipeline. However, explicitly using `Write-Output` provides clarity in your code and improves maintainability.
Why Use Write-Output?
There are several scenarios where using `Write-Output` can be beneficial:
-
Readability: By making your intent clear, `Write-Output` helps others (or future you) understand that you are purposely sending information to the next pipeline.
-
Explicit Control: In complex scripts, being explicit about output can help avoid confusion about what data is being passed along.
Although using just the variable will also produce the same output, `Write-Output` makes the intent clear, which is particularly important in scripting environments.
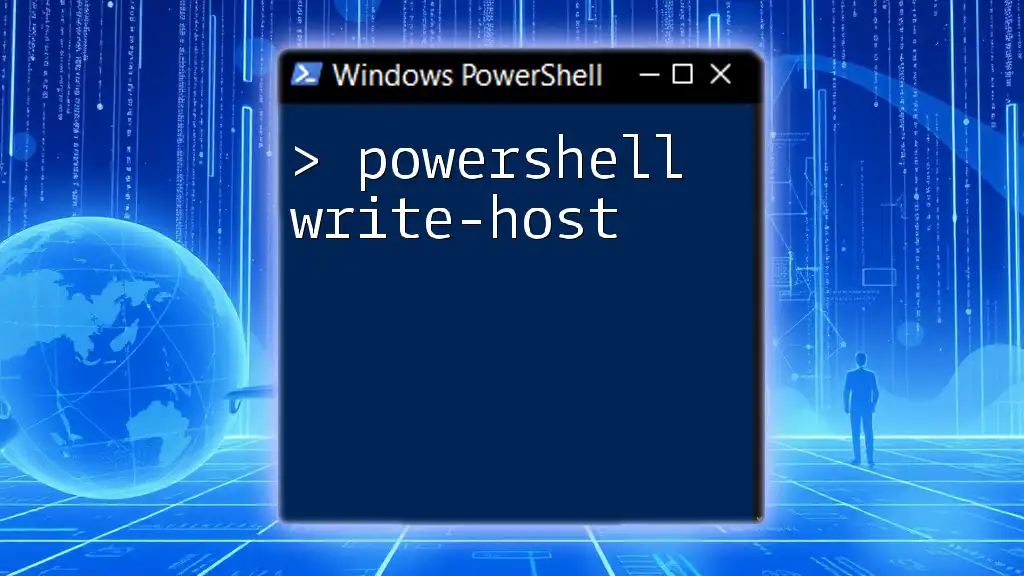
Basic Usage of Write-Output
Syntax
The syntax for `Write-Output` is straightforward:
Write-Output "Your output here"
Simple Examples
Example 1: Basic text output
Using `Write-Output` to print a simple string is often the first example you will see.
Write-Output "Hello, World!"
In this case, when executed, PowerShell would display `Hello, World!` in the console.
Example 2: Outputting a variable
You can also use it to output the value of a variable.
$greeting = "Hello, PowerShell!"
Write-Output $greeting
This code snippet outputs `Hello, PowerShell!`. Notice that the variable itself is passed to `Write-Output`, reinforcing the point that you can send any data type through the pipeline.
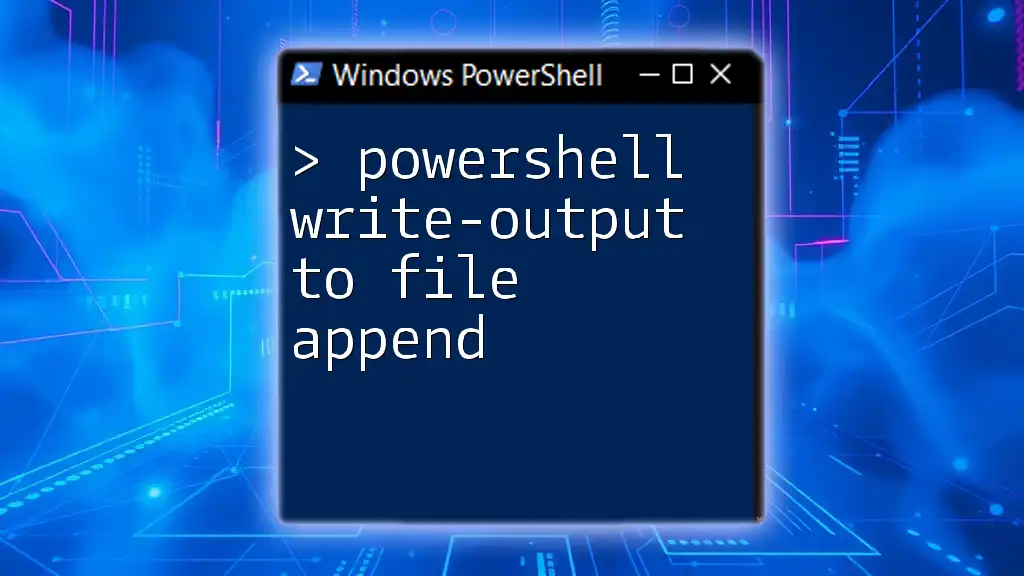
Advanced Use Cases
Sending Output to Files
Using Out-File
One practical application of `Write-Output` is redirecting the output to files. If you want to log data, you can easily direct its output to a file.
Write-Output "Logging some data" | Out-File "log.txt"
In this example, `"Logging some data"` is written to a text file named `log.txt`. The file will be created in the current working directory unless a full path is specified.
Pipelining with Write-Output
How Pipelining Works
PowerShell's pipeline streams output from one command to another. This is where `Write-Output` shines, as it seamlessly integrates into this construct.
Example of Pipelining
Here’s how to combine `Write-Output` with `Where-Object` for filtering data:
1..10 | Where-Object { $_ -gt 5 } | Write-Output
In this snippet, the numbers 1 through 10 are piped into `Where-Object`. It filters the numbers greater than 5, passing them to `Write-Output`, which displays the final output (`6`, `7`, `8`, `9`, `10`). This illustrates how `Write-Output` can fit neatly into the PowerShell pipeline.
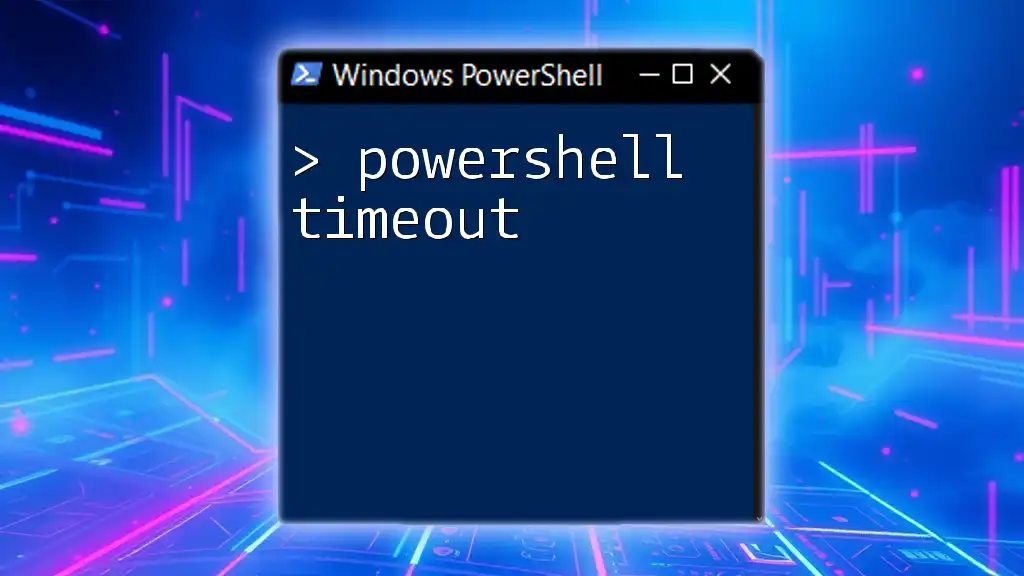
Alternatives to Write-Output
Different Cmdlets for Output
While `Write-Output` is commonly used, there are other cmdlets you might consider:
-
Write-Host: Unlike `Write-Output`, `Write-Host` writes directly to the console and does not send output to the pipeline. This can sometimes lead to confusion as its use is more a matter of presentation.
-
Write-Information: This cmdlet is used for informational messages. Unlike `Write-Host`, `Write-Information` can be controlled for visibility through the `$InformationPreference` variable.
Example Comparisons
Using Write-Host:
Write-Host "This will display directly in the console."
In contrast to `Write-Output`, this outputs data directly to the console without carrying any further down the pipeline, which can be less useful in scripts intended for automation.
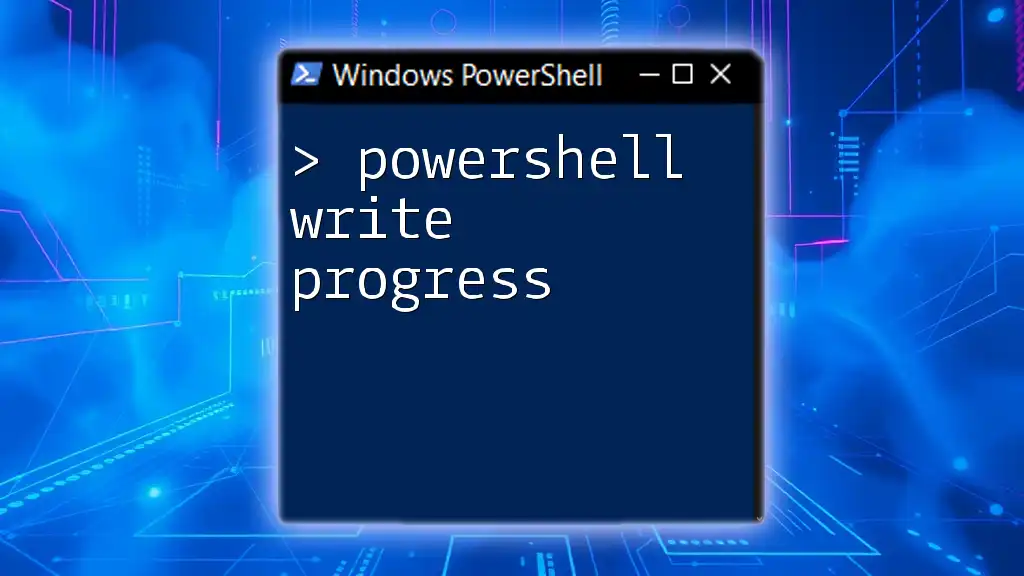
Common Mistakes When Using Write-Output
Forgetting the Pipeline
One common mistake is neglecting the power of the pipeline. Assume you write:
$myVar = Write-Output "This is a test"
Here, while `$myVar` will still store the string `"This is a test"`, it may not be clear to those reading the code that output is being sent to the pipeline.
Using Write-Output Unnecessarily
There are situations where using `Write-Output` can be redundant. For example:
$greeting = "Hello, PowerShell!"
$greeting
In this context, simply referencing `$greeting` automatically outputs the value. Therefore, using `Write-Output` is unnecessary.
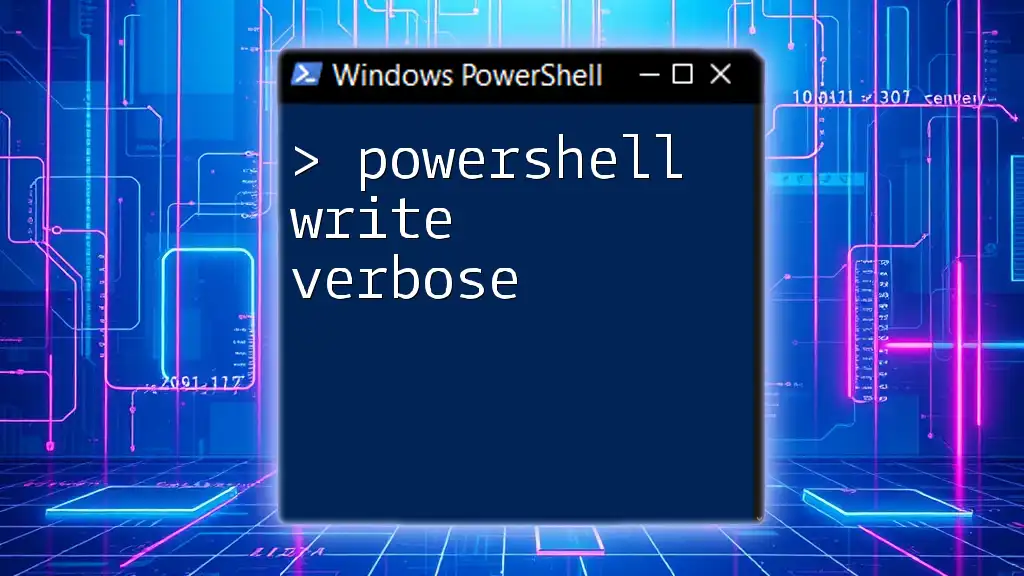
Debugging Output Issues
Troubleshooting Output
If you find that your output isn't appearing as expected, ensure you are considering scope and variable lifetimes. For example, a common issue is accessing a variable outside of its defined scope, which leads to null or unexpected outputs.
Using Verbose Output
For debugging, you can add the `-Verbose` parameter to `Write-Output`:
Write-Output "Debugging Mode" -Verbose
This provides additional context and helps clarify what information is being processed at any point during execution.
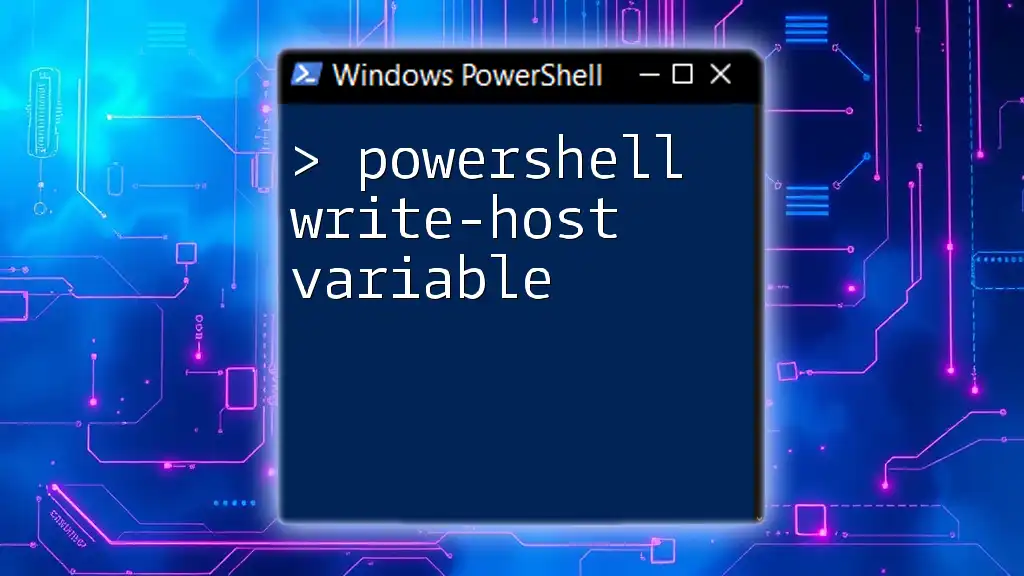
Conclusion
Understanding how to effectively utilize `Write-Output` within PowerShell is crucial for writing clean, maintainable scripts. By using this cmdlet, you craft clearer code that not only serves practical purposes but also enhances readability for anyone reviewing it later. Embrace the power of `Write-Output` and experiment with its various applications to become a more proficient scripter in PowerShell.
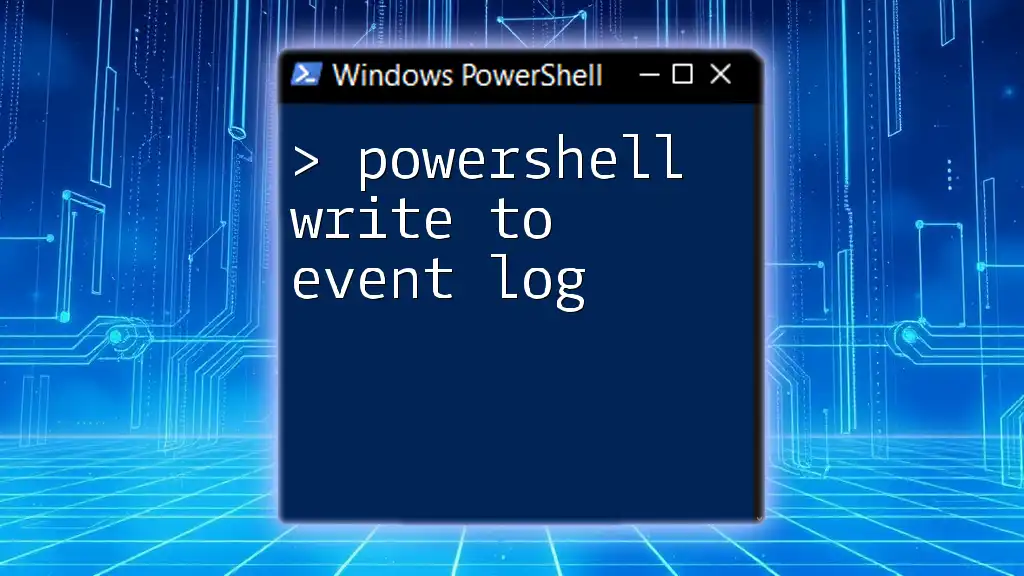
Additional Resources
For further insights, consult the official Microsoft documentation on [Write-Output](https://docs.microsoft.com/en-us/powershell/module/microsoft.powershell.utility/write-output) and explore comprehensive PowerShell resources, including books and online courses for mastering these techniques.
FAQs
What is the difference between Write-Output and Write-Host?
While `Write-Output` sends data to the pipeline, allowing it to be processed by other cmdlets, `Write-Host` outputs directly to the console and isn’t piped further.
Can I use Write-Output in scripts and functions?
Absolutely! `Write-Output` is often used in functions to return results or to send data along the pipeline.
How do I format the output of Write-Output?
You can format output by piping `Write-Output` into other cmdlets, such as `Format-Table` or `Format-List`, which allows for structured data presentation.