The `Wait-Job` cmdlet in PowerShell is used to pause the execution of a script until one or more background jobs have completed.
Here’s a code snippet demonstrating its usage:
$job = Start-Job { Get-Process }
Wait-Job $job
Receive-Job $job
Understanding PowerShell Jobs
What are PowerShell Jobs?
Jobs in PowerShell are a powerful feature designed for executing tasks asynchronously. This approach allows scripts to run in the background, freeing up the console for other tasks. There are primarily two types of jobs in PowerShell: Background Jobs and Scheduled Jobs, both serving unique purposes.
- Background Jobs are used for executing commands that can take an extended amount of time to complete, allowing you to run multiple jobs concurrently without blocking your session.
- Scheduled Jobs enable you to run tasks at specified intervals, similar to Windows Task Scheduler but within the PowerShell environment.
How Jobs Improve Efficiency
The concept of jobs significantly enhances the efficiency of PowerShell scripts. By enabling asynchronous execution, PowerShell allows multiple commands or scripts to run simultaneously. This is especially beneficial for long-running tasks, such as data backups or system scans, as it can drastically reduce the overall time required for tasks to complete.
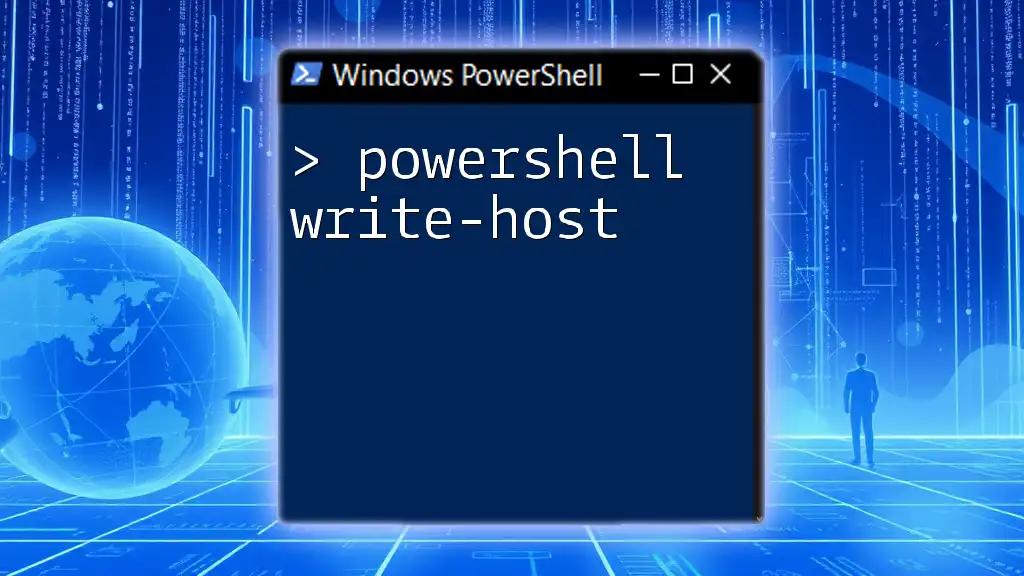
The `Wait-Job` Command
What is `Wait-Job`?
The `Wait-Job` cmdlet is pivotal when dealing with jobs in PowerShell. Its primary purpose is to pause the execution of subsequent commands in a script until the specified job(s) have completed. This capability ensures that the script runs sequentially after the completion of a job, which is crucial when the results of a job are required for further processing.
The syntax you will commonly use is:
Wait-Job -Job <Job>
Common Parameters
Key parameters to be aware of when using `Wait-Job` include:
- `-Job`: This parameter specifies the job or jobs you wish to wait for. You can pass either a job object or an array of job objects.
- `-Timeout`: Defines the maximum amount of time to wait. If the job does not complete within the specified timeout period, the command will continue executing.
- `-Force`: Utilized to stop the job if it exceeds the timeout period. This can be particularly useful in situations where system resources need to be managed tightly.

Using `Wait-Job` in Practice
Basic Usage
The simplest way to incorporate `Wait-Job` is to run a job and ensure the script waits for its completion before proceeding. Here’s a basic example:
Start-Job -ScriptBlock { Get-Process }
Wait-Job -Job 1
In this example, the `Start-Job` command initiates a background job that retrieves the current processes running on the system. The `Wait-Job` command then pauses script execution until the job id `1` completes.
Handling Multiple Jobs
Often, scripts will require waiting for multiple jobs to finish. You can handle this easily with `Wait-Job`:
$job1 = Start-Job -ScriptBlock { Get-Process }
$job2 = Start-Job -ScriptBlock { Get-Service }
Wait-Job -Job $job1, $job2
This code snippet demonstrates starting two jobs—one for getting processes and another for retrieving services. By passing both job objects to `Wait-Job`, you ensure the script awaits the completion of both jobs before moving forward.
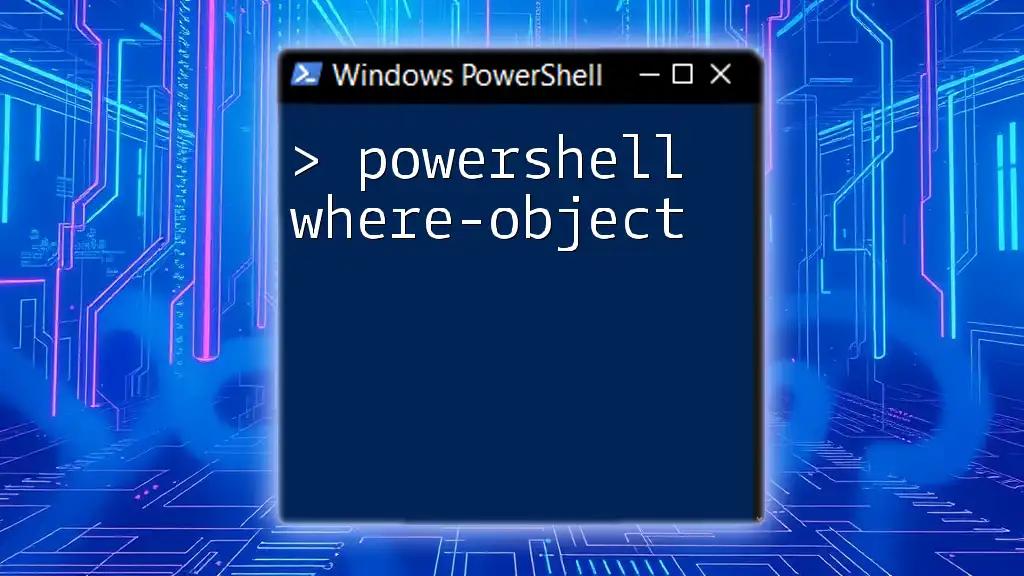
Advanced Techniques with `Wait-Job`
Using the `-Timeout` Parameter
An important aspect of effective job management is understanding how to utilize the `-Timeout` parameter. This allows you to specify how long to wait for a job before proceeding.
Start-Job -ScriptBlock { Start-Sleep -Seconds 10 }
Wait-Job -Job $job1 -Timeout 5
In this scenario, the script starts a job designed to sleep for ten seconds but only waits for five. Consequently, you may encounter a timeout error, which needs to be managed correctly.
Job Monitoring
It's often beneficial to check the status of a job before using `Wait-Job`. The `Get-Job` cmdlet can assist in monitoring job status and give you insights into whether a job is still running.
$myJob = Start-Job -ScriptBlock { Start-Sleep -Seconds 15 }
while ($myJob.State -eq 'Running') {
Write-Host "Job is still running..."
Start-Sleep -Seconds 2
}
Wait-Job -Job $myJob
This code snippet demonstrates a loop that repeatedly checks the state of a job. While the job is still running, a message is outputted to the console every two seconds. Once the job completes, `Wait-Job` is invoked to ensure all resources are handled properly.
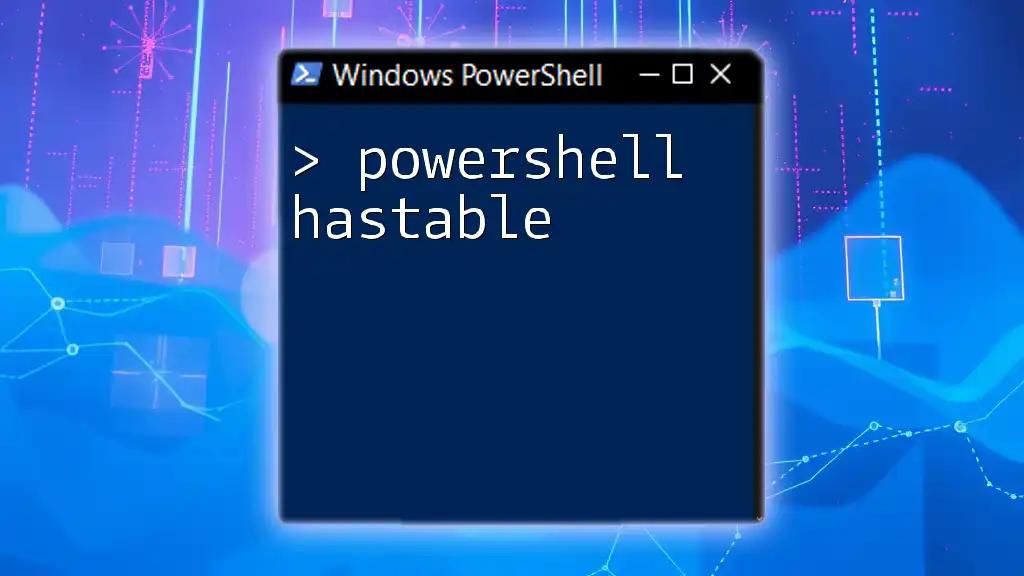
Common Mistakes and Troubleshooting
Common Errors with `Wait-Job`
When using `Wait-Job`, you might encounter several common errors. One typical error is: "Could not wait for job". This usually indicates that the specified job cannot be found or is already completed.
Troubleshooting Tips
When facing issues with `Wait-Job`, consider these troubleshooting steps:
- Ensure that the job ID or job object you're passing is correct and still active.
- Check for any syntax errors in your scripts, as these can prevent jobs from being created or executed correctly.
- Use the `Get-Job` command to list and view the status of all jobs, ensuring you understand their current state before calling `Wait-Job`.
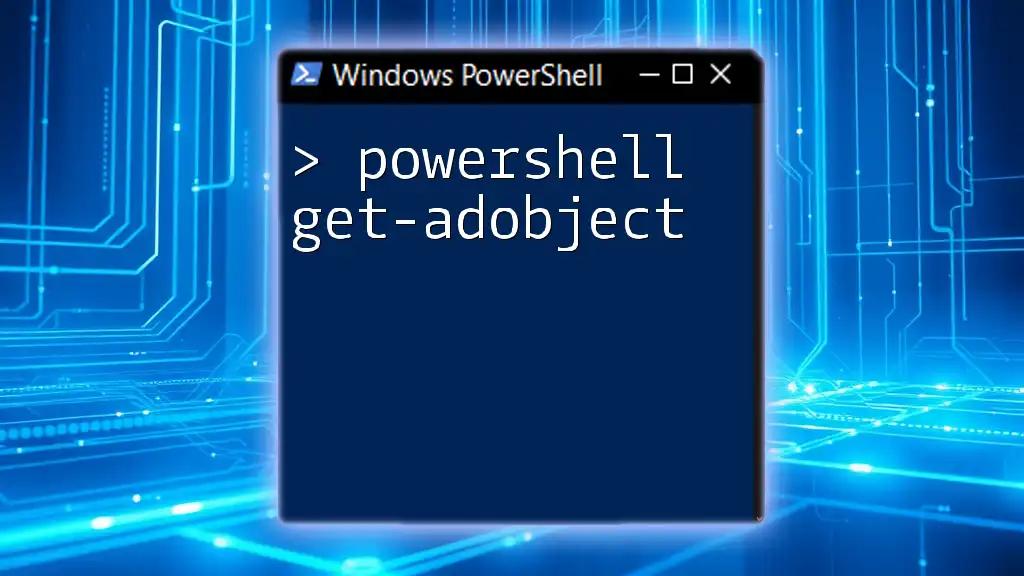
Use Cases in Real-world Scenarios
Automation Scripts
`Wait-Job` can effectively manage complex automation scripts that rely on multiple sequential operations. For instance, if you're running a series of data processing tasks, ensuring that each completes before the next begins can be crucial for maintaining the integrity of your data.
Resource Management
In environments where resources are limited, such as servers running multiple critical applications, employing `Wait-Job` can help prevent over-utilization. By monitoring jobs and waiting for completion, you can ensure that necessary resources are available for follow-up operations.
For example, in a backup script, you might first call a job to backup a database and need to ensure it completes before moving onto additional tasks, such as notifying users or cleaning temporary files.
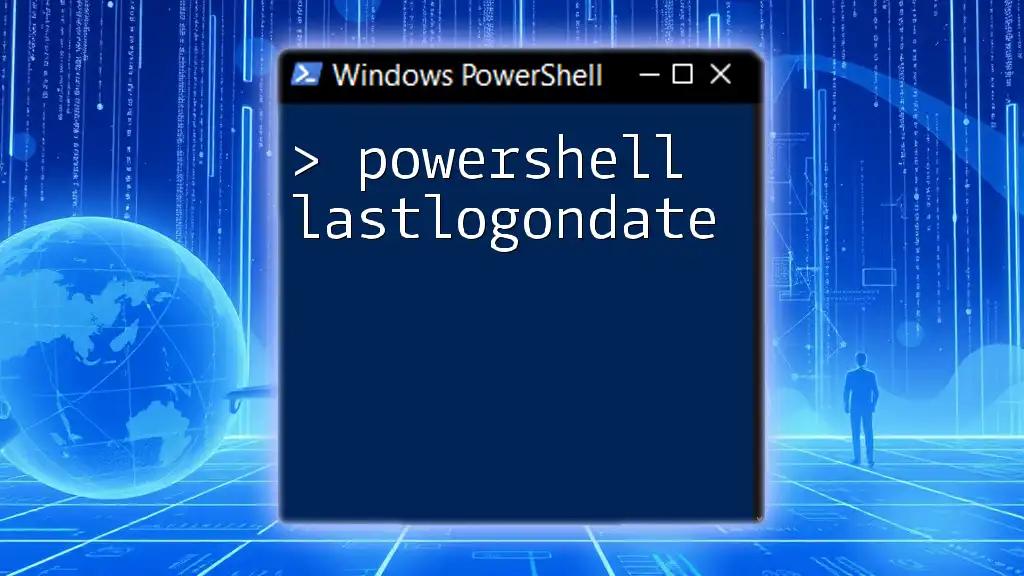
Conclusion
The `Wait-Job` cmdlet is an essential tool for anyone looking to enhance their PowerShell scripting capabilities. By mastering this command and its associated jobs, you can significantly improve the efficiency, reliability, and performance of your scripts. Practice incorporating `Wait-Job` into your daily workflows, and explore its potential to automate and streamline processes effectively.

Call to Action
We encourage you to share your experiences using `Wait-Job` or ask questions if you encounter challenges. Don't forget to sign up for our PowerShell short courses for more in-depth knowledge and hands-on practice!
Additional Resources
For further learning, consider exploring the official PowerShell documentation on jobs and cmdlets, as well as recommended courses and community resources to deepen your understanding of PowerShell's job management functionalities.