In PowerShell, you can pause the execution of your script until the user presses any key by using the `Read-Host` cmdlet.
Here's a code snippet to demonstrate this:
Read-Host -Prompt 'Press Enter to continue...'
Understanding the Concept of Pause in PowerShell
What is a pause? In PowerShell, a pause allows the execution of a script to halt until the user provides input. This can be critically useful in scenarios where human confirmation is necessary before proceeding, such as deployment scripts, user prompts, or debugging sessions.
Common use-cases for pausing scripts include:
- User confirmations: Before executing a potentially destructive operation, such as deleting files or modifying system configurations, it's wise to double-check with the user.
- Script progress checks: In lengthy processes, pausing can give users time to review what's happening, particularly in scripts with multiple stages.
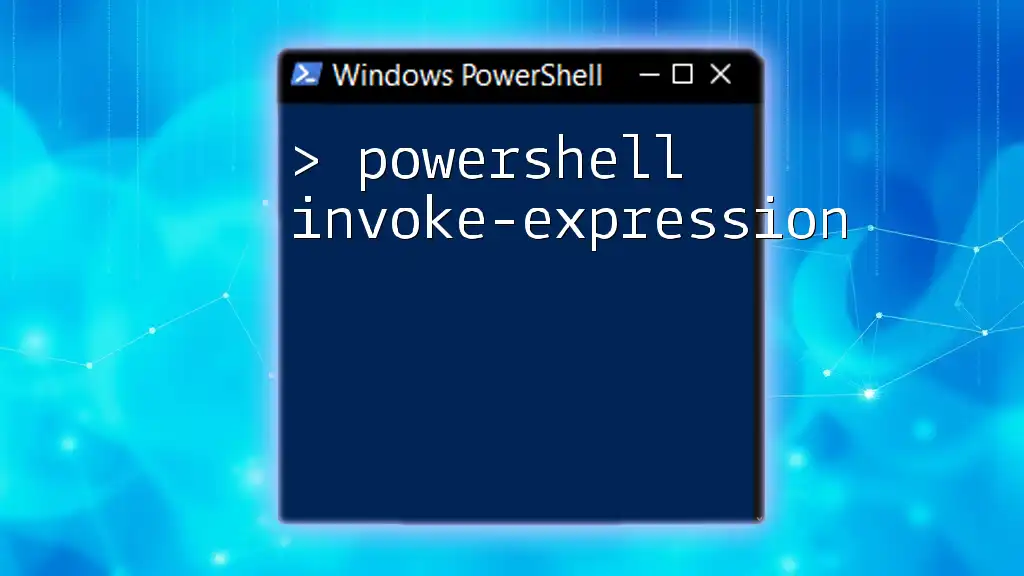
Implementing Keypress Pause in PowerShell
Using `Read-Host` for User Input
Overview of `Read-Host`: This cmdlet prompts the user for input in a straightforward manner, making it a popular choice for creating pauses in scripts.
Basic Example of `Read-Host`: To implement a simple wait for a keypress, you can use the following code:
$userInput = Read-Host -Prompt "Press any key to continue..."
This line of code will display "Press any key to continue..." and pause execution until the user types something and presses Enter.
Using `[System.Console]::ReadKey()`
Introduction to `[System.Console]`: This approach utilizes the .NET Console class, providing more flexibility, including the ability to capture which key was pressed without requiring the user to press Enter.
Basic Implementation Example: Here’s how you can use it to create a pause:
Write-Host "Press any key to continue..."
[System.Console]::ReadKey() > $null
This command displays a message and pauses execution until any key is pressed, with the `$null` redirecting the output to prevent cluttering the console.
Creating Functions for Reusability
Advantages of using functions: By encapsulating the wait logic in a function, you not only make your code cleaner but also allow for reusing this functionality across multiple scripts.
Function Example: A simple function for waiting for keypress might look like this:
function Wait-ForKeyPress {
Write-Host "Press any key to continue..."
[System.Console]::ReadKey() > $null
}
Wait-ForKeyPress
This reusable function can be called whenever a pause is needed, making your scripts more concise and maintainable.
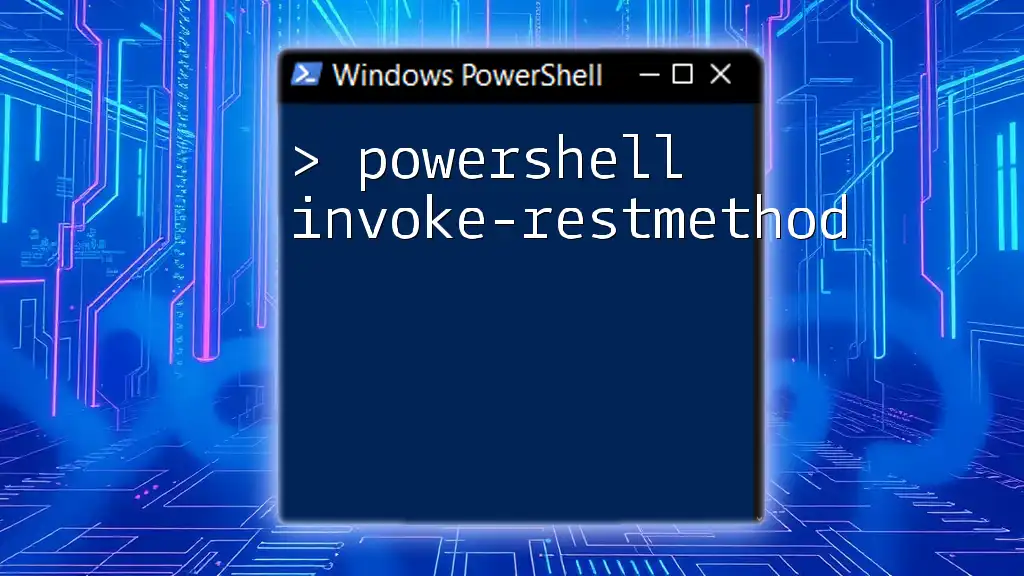
Advanced Techniques
Customizing Keypress Pause
Customizing prompts and messages: You can enhance user experience by allowing custom messages in your wait function.
function Wait-ForKeyPress {
param (
[string]$Message = "Press any key to continue..."
)
Write-Host $Message
[System.Console]::ReadKey() > $null
}
Wait-ForKeyPress -Message "Press ENTER to proceed!"
This allows for a tailored message that can reflect the context of what’s happening in your script.
Adding Timeouts to Keypress
Implementing timeouts with `Start-Sleep`: In some cases, you might want to give users a limited time to respond. Combining this with a sleep command can be effective.
Write-Host "Press any key within 5 seconds..."
$keyPress = [System.Console]::ReadKey($true)
Start-Sleep -Seconds 5
In this example, the script will wait for a keypress and then sleep for 5 seconds, helping manage situations where waiting indefinitely is not ideal.
Creating User-Friendly Messages
Dynamic messaging based on conditions: Crafting messages that adapt to different script states can enhance clarity.
$condition = $true
if ($condition) {
Wait-ForKeyPress -Message "Condition was true! Press to continue."
} else {
Wait-ForKeyPress -Message "Condition was false! Press to exit."
}
This logic adds flexibility to your user prompts, making it clear to users what action they are confirming.
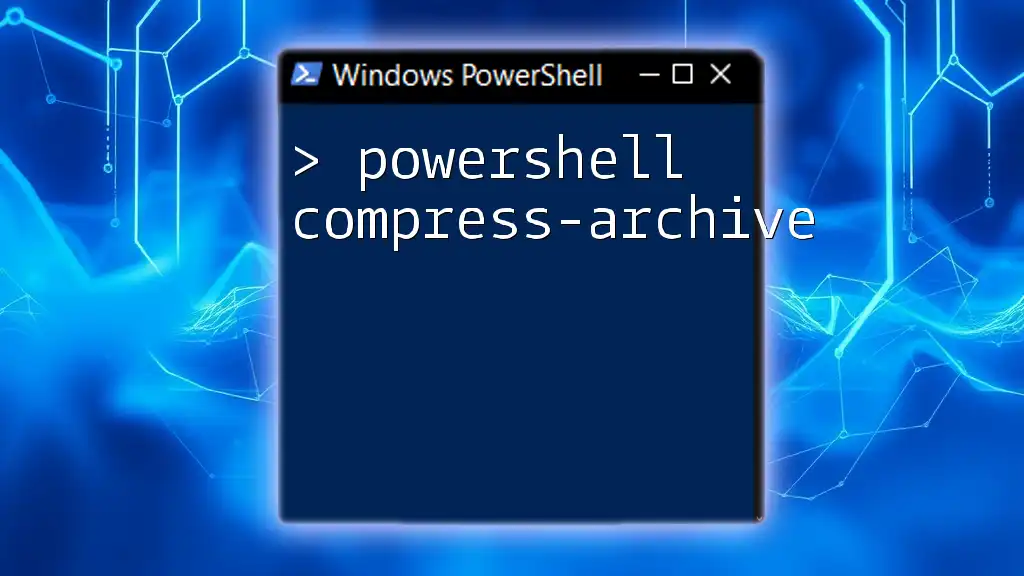
Error Handling and Edge Cases
Handling No Keypress: It's essential to consider situations where a user might not press a key. Implementing error handling in your wait logic can prevent crashes.
Example of Error Handling:
try {
Write-Host "Press any key to continue (you have 10 seconds)..."
if (-not $null -eq [System.Console]::ReadKey(-1).Key) {
Throw "No key was pressed!"
}
}
catch {
Write-Host "An error occurred: $_"
}
This block ensures that if no key is pressed, a meaningful message is displayed to guide the user regarding what went wrong.
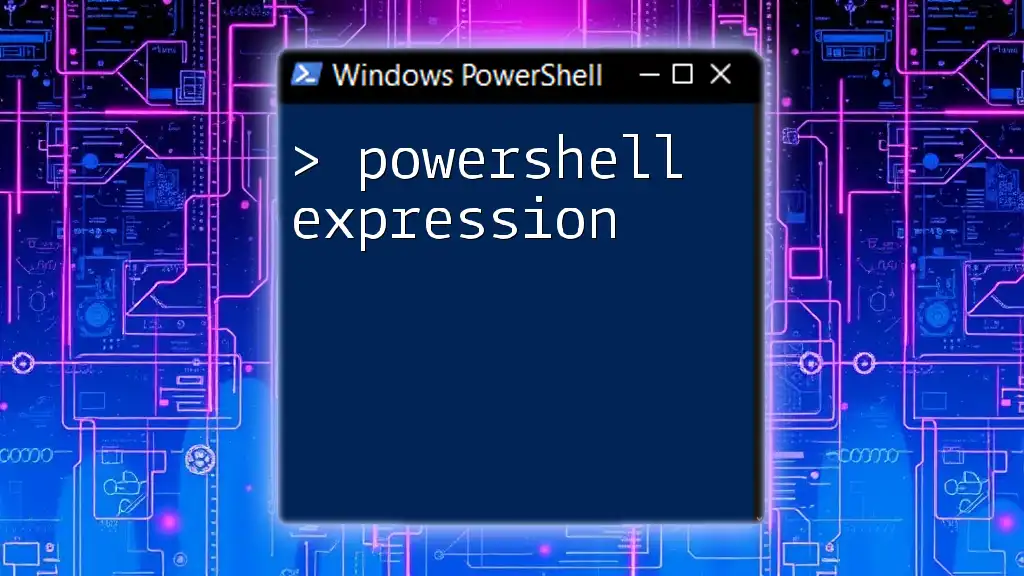
Best Practices for Using Wait for Keypress
When using the PowerShell wait for keypress functionality, it is beneficial to adhere to certain best practices.
- Keep prompts clear and concise: Ambiguous messages can confuse users. Clear and understandable prompts help convey the intent.
- Avoid unnecessary pauses: Too many pauses in a script can diminish user experience. Use waits judiciously to enhance rather than impede workflow.
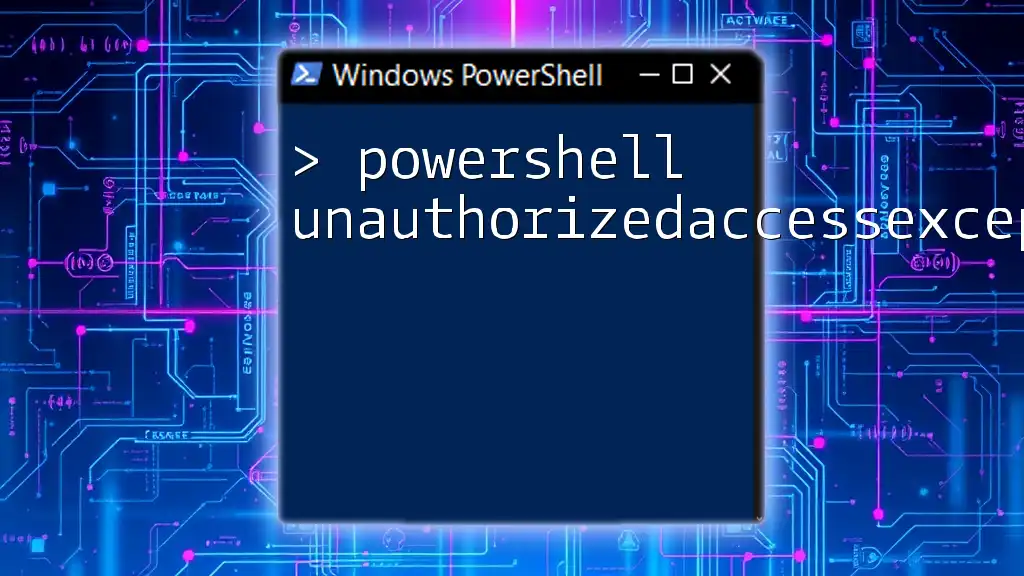
Conclusion
Incorporating the PowerShell wait for keypress functionality can significantly improve user interactions with your scripts. It’s a straightforward yet powerful tool that helps manage flow and provides users with necessary control over script execution. By employing the techniques and examples discussed, you can create more user-friendly, efficient, and robust PowerShell scripts. Remember to explore more PowerShell features to enhance your skills and scripting capabilities.
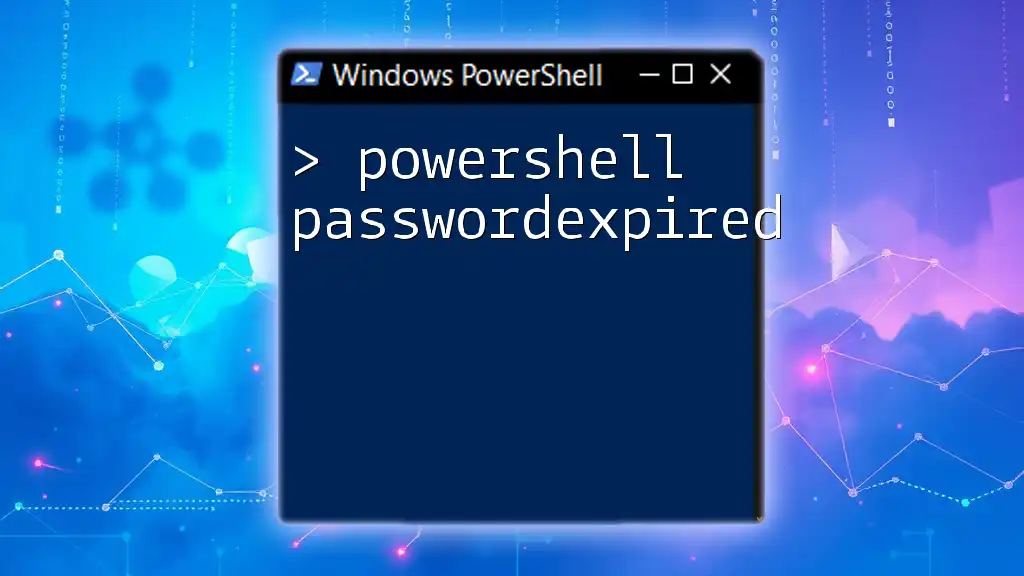
Additional Resources
For further reading, consider visiting PowerShell documentation and forums to engage with the community and deepen your understanding of scripting possibilities. You can also check out books and online courses specifically focused on PowerShell to enhance your learning journey.