PowerShell's `Invoke-Expression` allows you to execute a string as a command with parameters by embedding variables directly within the string, enhancing dynamic command execution.
Here’s a code snippet demonstrating its usage:
$param = "World"
Invoke-Expression "Write-Host 'Hello, $param!'"
Understanding Invoke-Expression
What is Invoke-Expression?
Invoke-Expression is a PowerShell cmdlet that allows you to execute a string as a command within the PowerShell environment. This command can be particularly useful for scenarios where you dynamically generate PowerShell commands as strings and need to execute them.
When to Use Invoke-Expression
Invoke-Expression shines in several scenarios, including:
- Dynamic Command Execution: When you need to create commands on-the-fly based on user input or other conditions.
- Scripting Complex Commands: When wrapping complex commands that may include multiple components or parameters.
However, it’s important to be cautious when using Invoke-Expression. Executing arbitrary strings can introduce security vulnerabilities, particularly script injection attacks, if the command strings are not properly sanitized.
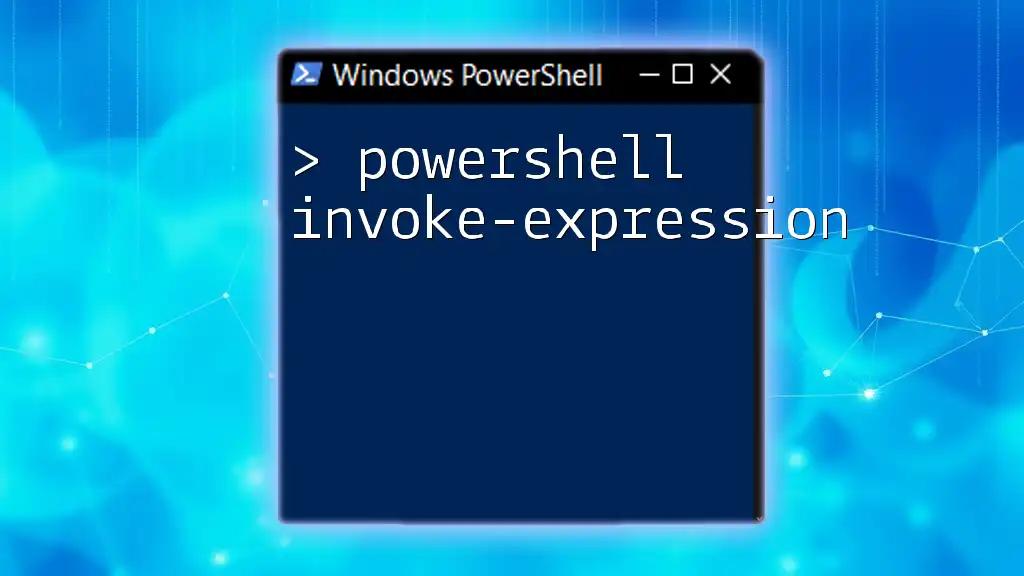
Basic Syntax of Invoke-Expression
Command Syntax
The general syntax for using Invoke-Expression is simple:
Invoke-Expression "<command_string>"
In this syntax, `"<command_string>"` must be a valid PowerShell command encapsulated as a string.
Example of Basic Usage
To illustrate the basic utility of Invoke-Expression, consider the following example:
$command = "Get-Process"
Invoke-Expression $command
In this case, the variable `$command` contains the string `"Get-Process"`, which retrieves a list of running processes. When executed, Invoke-Expression executes the command contained within the string.
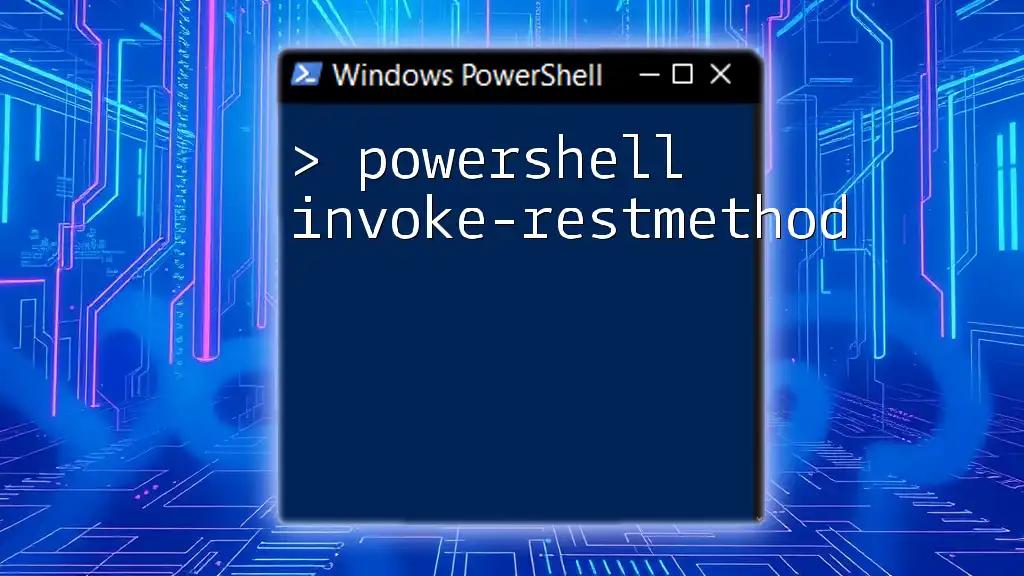
Working with Parameters
Adding Parameters to Commands
Invoke-Expression can take parameters just like any other PowerShell command. Here is how to integrate parameters into your command strings.
For instance, if you want to get information about a specific process, you can do this:
$procName = "notepad"
$command = "Get-Process -Name $procName"
Invoke-Expression $command
Here, `$procName` is used as a variable parameter representing the process name and can be dynamically changed.
Dynamic Parameter Handling
Building on this, if you need to handle parameters dynamically, you might consider an approach like this:
$param = "powershell"
$command = "Get-Process -Name $param -ErrorAction SilentlyContinue"
Invoke-Expression $command
This example not only retrieves information about the process named "powershell" but also incorporates the `-ErrorAction` parameter to handle any potential errors quietly without flooding the console with error messages.

Advanced Usage of Invoke-Expression with Parameters
Combining Multiple Commands
You can also use Invoke-Expression to combine multiple commands. This functionality is essential when working with pipelines and more complex command structures.
Consider the following example:
$cmd1 = "Get-Service"
$cmd2 = "Where-Object { $_.Status -eq 'Running' }"
$fullCommand = "$cmd1 | $cmd2"
Invoke-Expression $fullCommand
In this scenario, Invoke-Expression executes two commands. The first retrieves all services, while the second filters those services to show only those that are currently running.
Error Handling in Invoke-Expression
When working with Invoke-Expression, it’s critical to handle potential errors effectively. Here’s how you can implement error checking:
try {
Invoke-Expression $command
} catch {
Write-Host "An error occurred: $_"
}
This structure encapsulates the Invoke-Expression call within a `try-catch` block, allowing your script to handle errors gracefully rather than crashing unexpectedly.
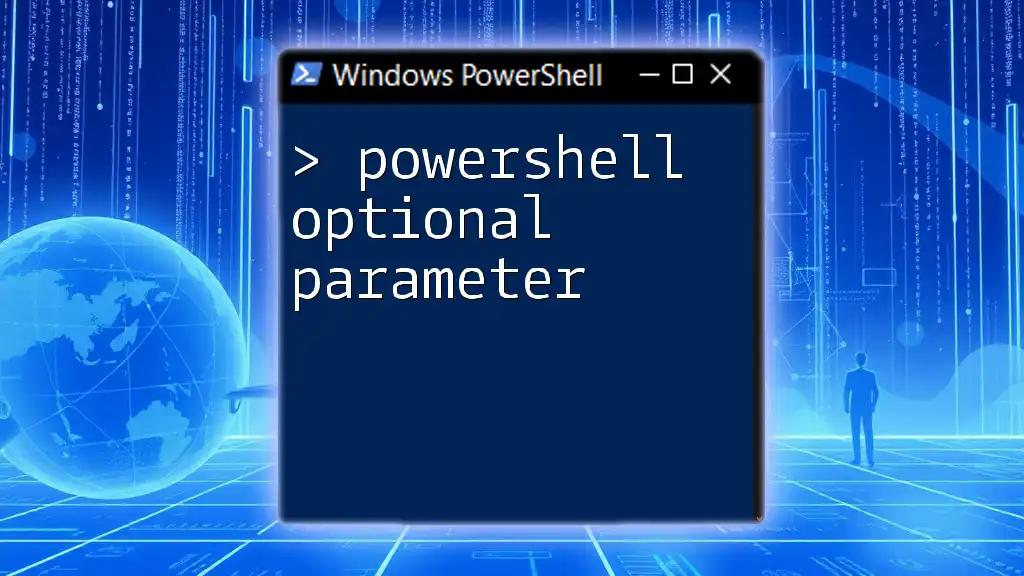
Best Practices for Using Invoke-Expression
Security Considerations
Due to its ability to execute string commands, Invoke-Expression can be a security risk. Be vigilant about where your command strings are generated from. Always sanitize inputs, especially if they stem from user input or external data sources, to protect against injection attacks.
Performance Implications
Excessive reliance on Invoke-Expression can lead to performance issues. It’s often better to use direct command calls or function calls where possible. Using Invoke-Expression might introduce unnecessary overhead compared to native command execution which is faster and safer.
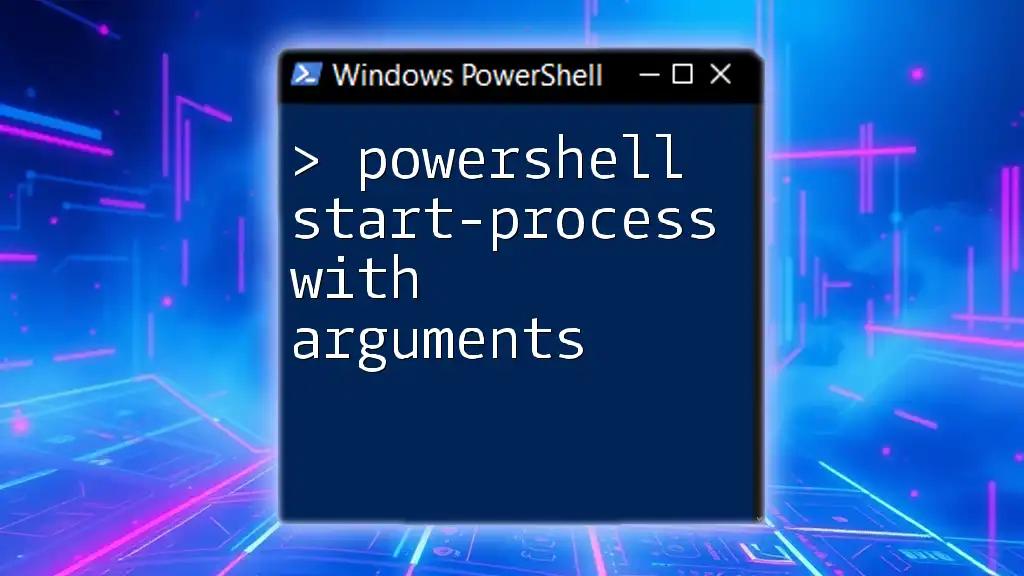
Conclusion
In summary, PowerShell Invoke-Expression with parameters is a powerful tool for executing dynamic commands. Understanding its syntax, appropriate use cases, and best practices will significantly enhance your scripting capabilities in PowerShell. By maintaining a focus on security and performance, you can effectively leverage this cmdlet in your scripts, allowing for greater flexibility and power in your command-line productivity.