To run an executable with arguments in PowerShell, you can use the `Start-Process` cmdlet followed by the executable path and any necessary arguments. Here’s an example:
Start-Process "C:\Path\To\YourProgram.exe" -ArgumentList "arg1", "arg2"
Understanding Executable Files
What is an EXE File?
An EXE file, short for "executable file," is a type of program that can be run on Windows operating systems. These files contain machine code that the system executes when the file is opened. Executables can be used for a wide variety of purposes: from-running applications like web browsers and games to managing system utilities and configuration settings.
What are Arguments and Parameters?
When running an executable, you may often want to pass in specific values that the software can utilize. These are known as arguments or parameters.
- Arguments are the values you tell the executable to use when it runs.
- Parameters are the variables that the executable needs to process those values.
Understanding how to effectively pass arguments and parameters to an EXE is essential for full control over its functionality.
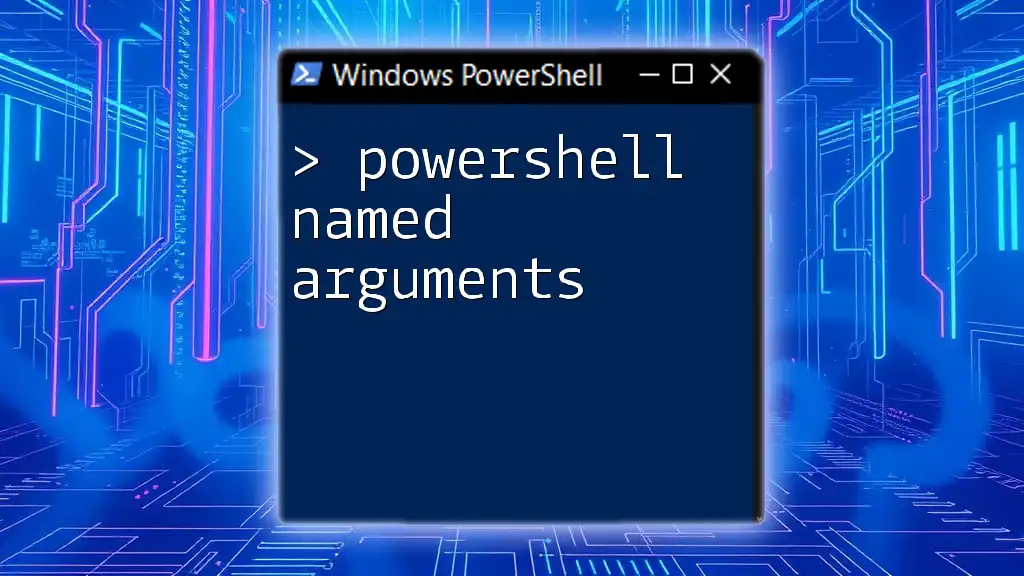
Basics of Running an EXE in PowerShell
PowerShell Command Syntax
PowerShell commands have a specific syntax. In its simplest form, a command to run an executable might look like this:
& "C:\path\to\your\executable.exe"
This structure is quite straightforward: the `&` operator allows you to run the command, and by surrounding the path in quotes, you ensure that PowerShell interprets it correctly.
Running an EXE without Arguments
Sometimes, you may only need to run an EXE without any additional input. In such cases, the command would look like this:
Start-Process "C:\path\to\your\executable.exe"
When executed, this will launch the specified executable without any parameters. You can expect the program to open as if you double-clicked it in File Explorer.
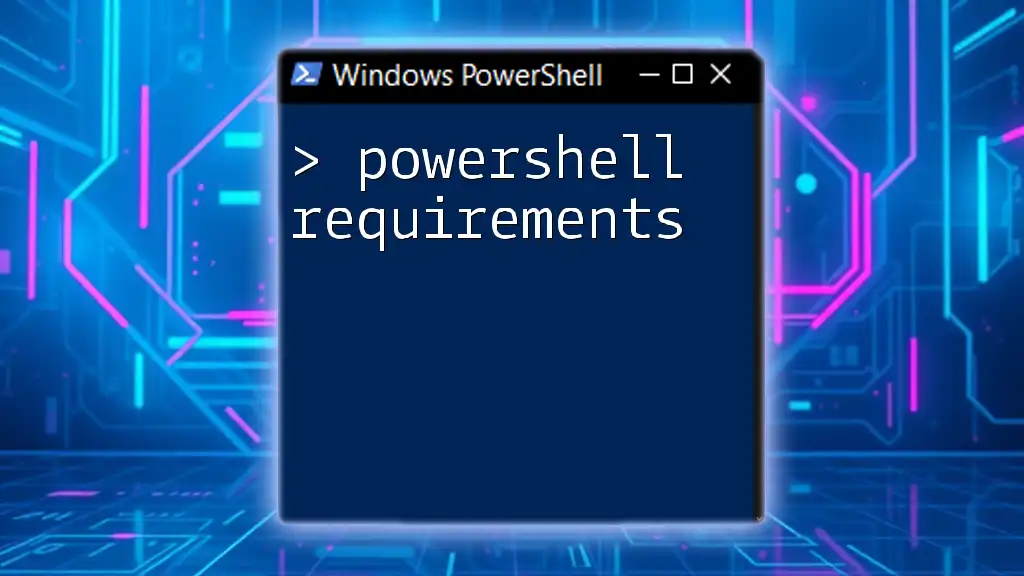
PowerShell Run EXE with Arguments
Using the `Start-Process` Cmdlet
To run an EXE with arguments in PowerShell, one commonly used cmdlet is `Start-Process`. This cmdlet allows you to specify not just the executable's location but also any arguments you want to pass along.
The basic syntax of the `Start-Process` cmdlet is:
Start-Process "C:\path\to\your\executable.exe" -ArgumentList "yourArgument"
Example: Running Notepad with a Specific File
If you want to open a specific text file in Notepad, you would write:
Start-Process "notepad.exe" -ArgumentList "C:\path\to\your\file.txt"
In this example, we are launching Notepad with the specified file. This demonstrates how easy it is to pass file paths as arguments to executable files.
Using `&` (Call Operator)
Another method to run an EXE with arguments is to use the call operator `&`. This operator allows for a slightly different syntax and can be very handy for certain scenarios.
Example: Running a Custom Application
& "C:\path\to\application.exe" "argument1" "argument2"
In this example, we're calling an executable while passing two arguments to it. The advantage of the call operator is in its simplicity when executing commands in scripts or one-liners.
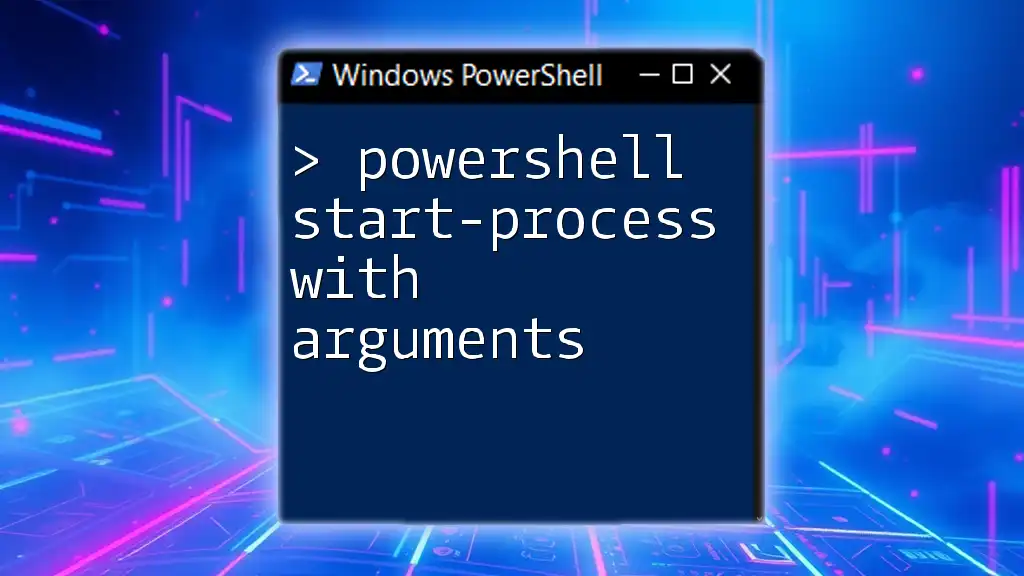
Passing Multiple Arguments
Using Quotes for Arguments with Spaces
If your arguments contain spaces, it's crucial to format them correctly. You need to use quotes to ensure PowerShell interprets the arguments as a single string.
Example: Running an EXE with Complex Arguments
Start-Process "C:\path\to\application.exe" -ArgumentList "arg1", "arg with spaces", "arg3"
Here, we've passed three arguments to the application, incl'ding one with spaces, demonstrating how to ensure clarity in your commands.
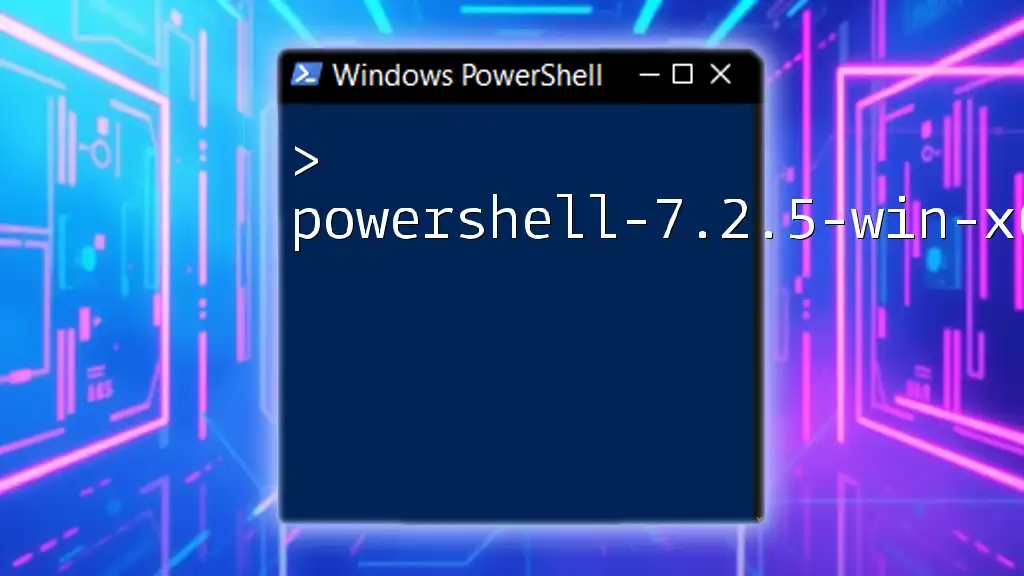
Advanced Techniques
Running Executables with Redirected Output
Capturing the output from an executable can be critical for logging or troubleshooting purposes. In PowerShell, you can redirect the output of an EXE to a file using the `-RedirectStandardOutput` parameter.
Example: Redirecting Output to a File
Start-Process "C:\path\to\application.exe" -ArgumentList "arg1" -RedirectStandardOutput "C:\output.txt"
This command runs the application while saving its output to a file, providing a convenient way to review results later.
Handling Errors in PowerShell
Error handling is an essential aspect of running any script. PowerShell provides a robust way to manage errors through `try` and `catch` blocks.
Example: Try-Catch for Error Management
try {
Start-Process "C:\path\to\application.exe" -ArgumentList "arg1"
} catch {
Write-Host "An error occurred: $_"
}
This example illustrates how to gracefully handle exceptions. If the executable fails to run, you will receive a clear message without crashing your entire script.
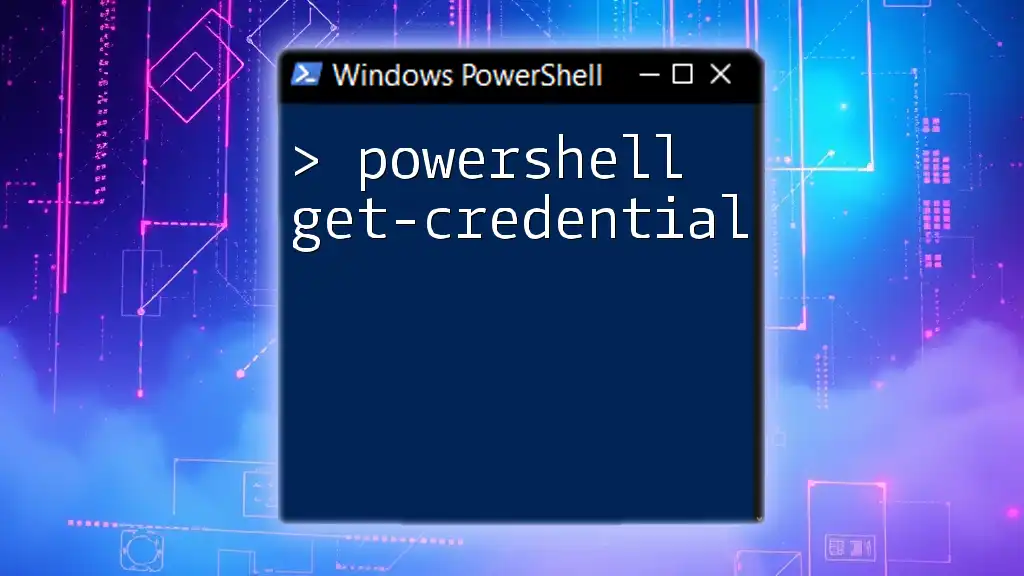
Common Use Cases
Scheduling Tasks with Executables
PowerShell is often used for automation, which can include scheduling tasks that require running executables with arguments at a specific time.
Debugging Executables
If you're working with a custom application or script, you might need to run a debugger alongside it. You can invoke a debugging tool via PowerShell using arguments specific to the debugging process.
In scenarios where passing arguments to an executable can enhance its functionality, mastering the command line and PowerShell tools can be a game changer for your workflow.
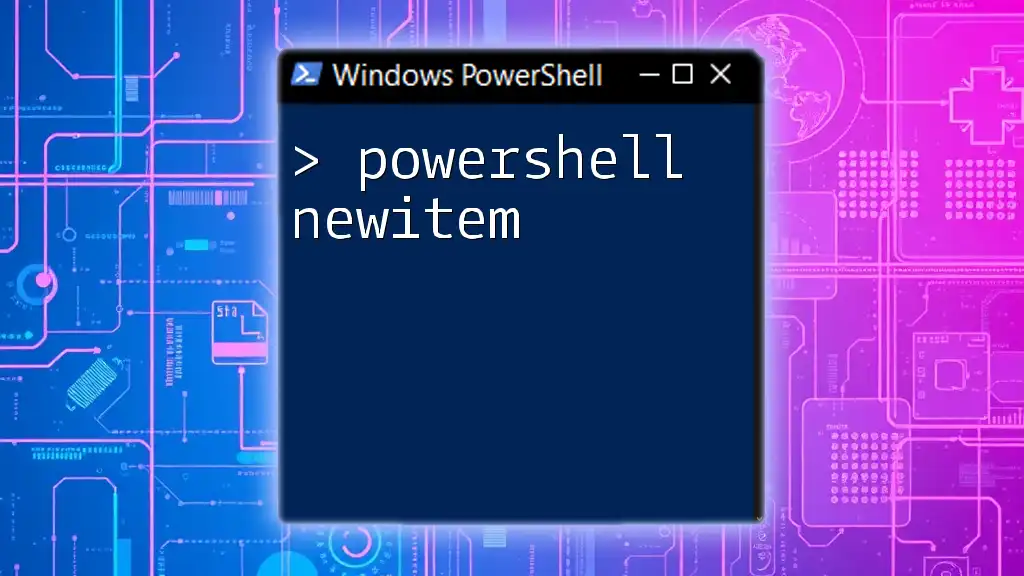
Conclusion
In summary, the ability to use PowerShell to run EXE files with arguments opens up a myriad of possibilities for automation, debugging, and task management. Mastering these techniques will not only simplify your workflow but empower you to utilize PowerShell to its full potential. Keep experimenting with different commands and parameters to discover how they fit into your scripting repertoire, and don't hesitate to dive deeper into the PowerShell community for further learning.
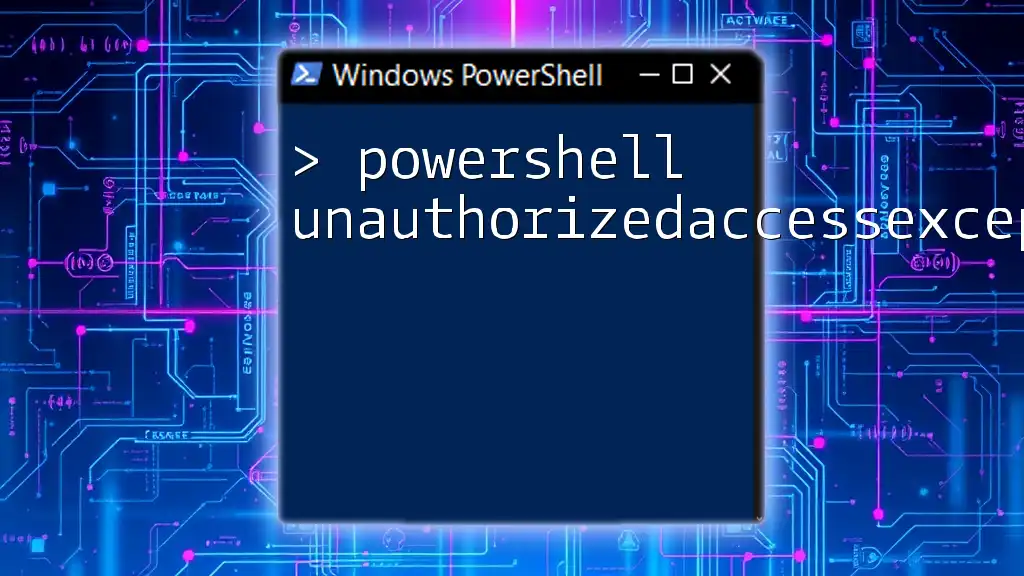
Additional Resources
For those looking to enhance their PowerShell skills, consider referring to official PowerShell documentation or exploring online courses and communities dedicated to PowerShell excellence.