To unset an environment variable in PowerShell, you can use the `Remove-Item` cmdlet to delete it from the `Env:` drive. Here's how you can do it:
Remove-Item Env:YOUR_VARIABLE_NAME
Replace `YOUR_VARIABLE_NAME` with the name of the environment variable you wish to unset.
Understanding Environment Variables in PowerShell
Definition of Environment Variables
Environment variables are dynamic values that affect the processes and behavior of applications on your system. Think of them as configuration settings that provide a way for the system to manage data used by the operating system and applications. For instance, they can store information like file paths, user preferences, and system configurations.
Common Uses of Environment Variables
Environment variables serve multiple purposes, such as:
- System configuration: They allow the operating system to access certain settings without hard-coding paths and values.
- Storing user-specific settings: Different users on the same system can have distinct configurations based on their environment variables.
- Application data paths: Applications can dynamically adjust their behavior using these variables, allowing better compatibility across different environments.
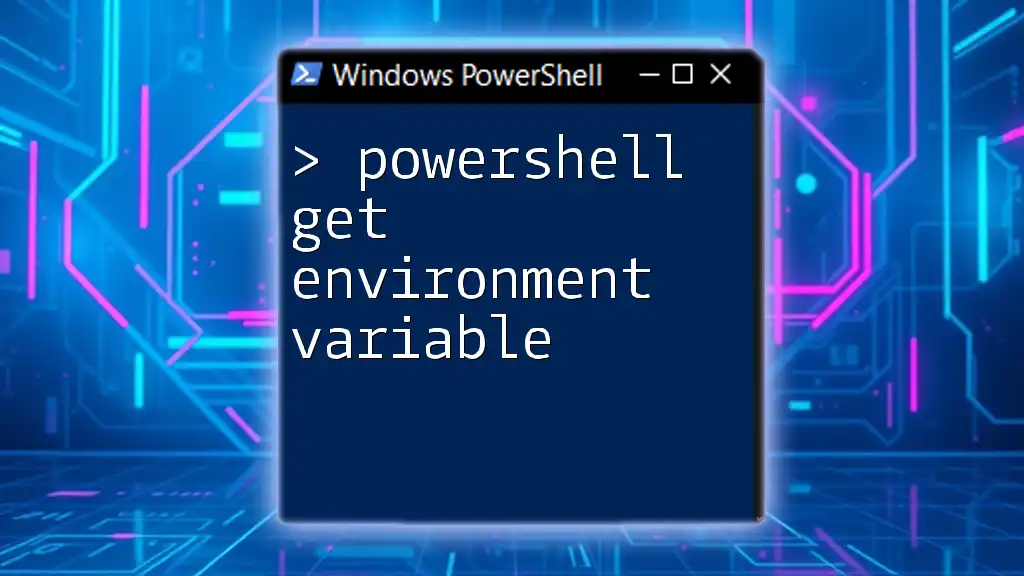
How to View Current Environment Variables
Using PowerShell to List Environment Variables
To get a view of the current environment variables in your PowerShell session, you can use the `Get-ChildItem` command on the `Env:` drive. This command retrieves a list of all environment variables available in your session, allowing you to see what values are currently set.
Here’s how to do it:
Get-ChildItem Env:
Running this snippet will result in a list of environment variables along with their current values.
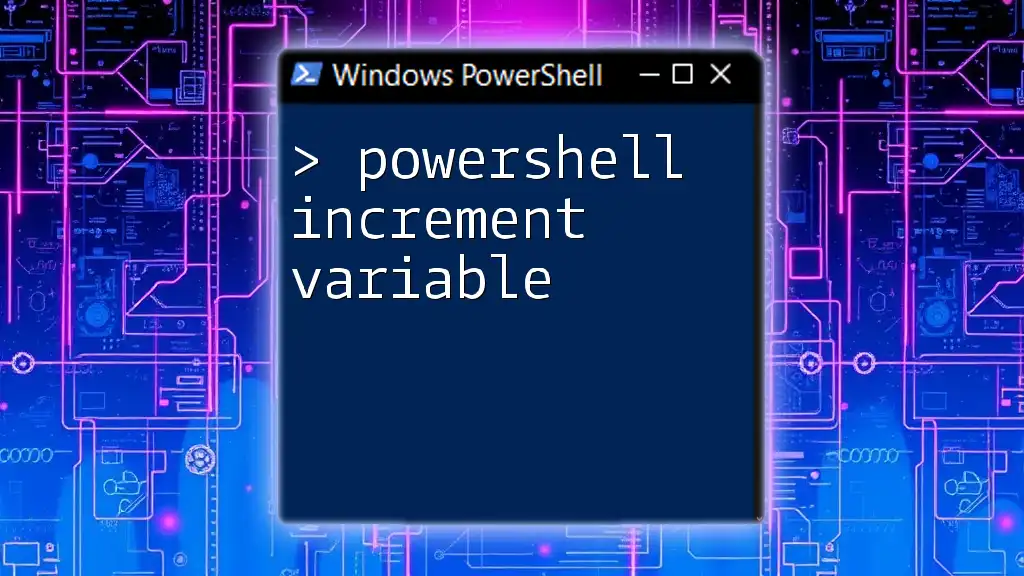
Unsetting Environment Variables in PowerShell
What Does "Unset" Mean?
In the context of PowerShell, unsetting an environment variable refers to the process of removing the variable from the current session or environment. This operation effectively makes that variable unavailable for future use, contrasting with merely altering its value. Understanding this distinction is crucial for managing your environment properly.
PowerShell Remove Environment Variable Command
To unset an environment variable in PowerShell, you primarily use the `Remove-Item` cmdlet. This command allows you to delete a specified environment variable and free up system resources associated with it.
The syntax for this command is straightforward:
Remove-Item Env:VARIABLE_NAME
For example, if you have an environment variable named `MY_VARIABLE` that you wish to unset, you would execute:
Remove-Item Env:MY_VARIABLE
After running this command, you have successfully removed the `MY_VARIABLE` from the environment, making it unavailable in the current session.
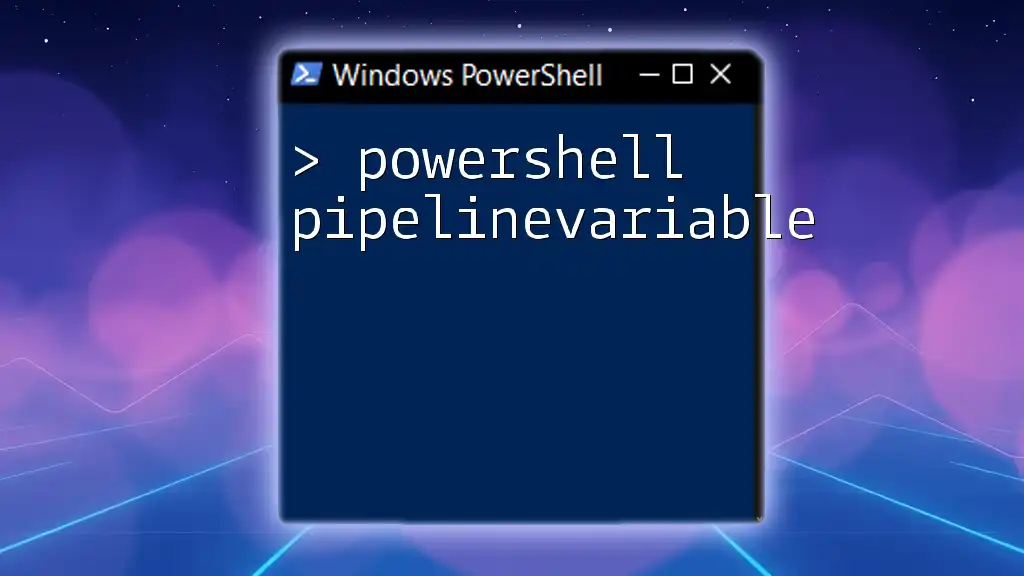
How to Set Environment Variables Back
Setting an Environment Variable in PowerShell
Once you've unset an environment variable, you may want to set it again. This can be done easily with the `setx` command, which sets a variable in both the user and system environment levels.
The syntax for setting an environment variable is:
setx MY_VARIABLE "new_value"
For instance, if you'd like to reintroduce `MY_VARIABLE` with a new value, execute:
setx MY_VARIABLE "new_value"
It’s important to note that changes made using `setx` persist beyond the current session, unlike variable assignments done directly in PowerShell which only last until the session ends.
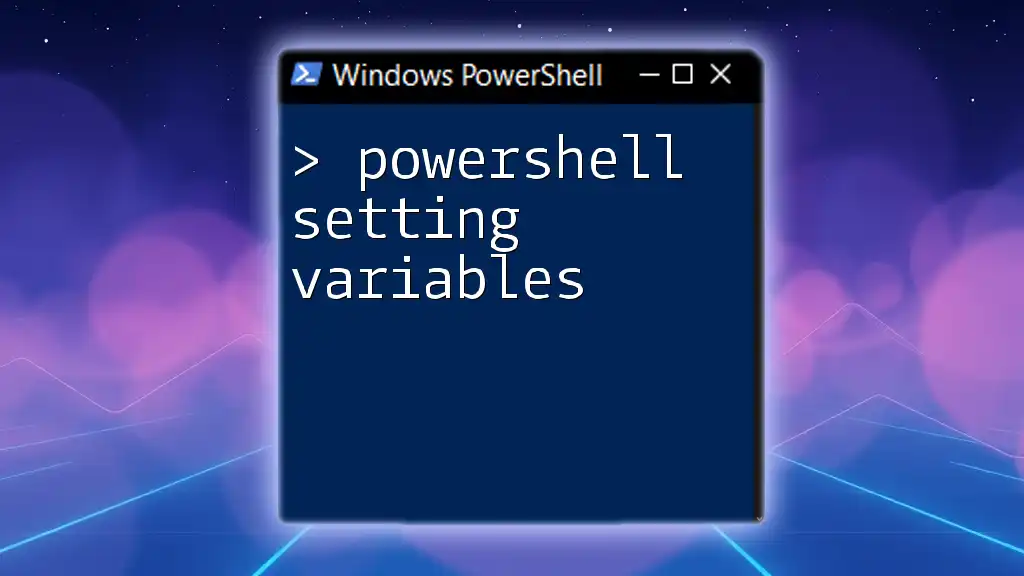
Verifying the Removal of Environment Variables
Confirming Unset Environment Variables
After you've executed the unset command, it's good practice to ensure that the variable has been removed. You can verify this by attempting to retrieve the variable using the `Get-ChildItem` command.
For example, to check if `MY_VARIABLE` still exists:
Get-ChildItem Env:MY_VARIABLE
If the variable was successfully unset, the command will return nothing, confirming that it no longer exists in the environment.
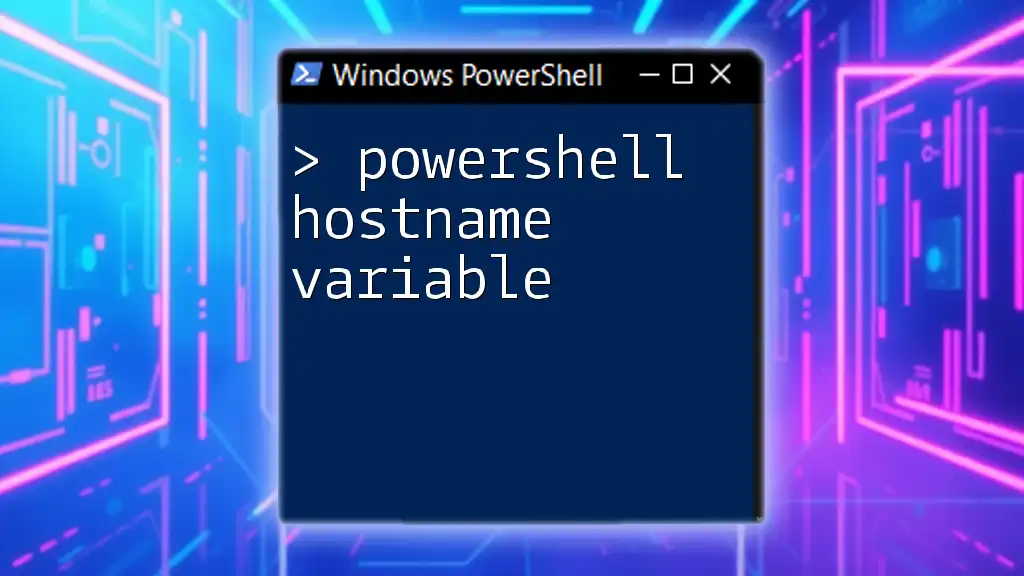
Handling Common Errors When Unsetting Environment Variables
Common Issues
You may encounter some complications when unsetting environment variables. Here are some common scenarios you might face:
- Trying to unset a non-existent variable: If the variable is not present when you attempt to remove it, PowerShell will return an error message.
- Permissions issues: If you do not have sufficient privileges, you might be unable to unset certain environment variables, especially those that are system-level.
Troubleshooting Tips
To troubleshoot these issues, consider the following tips:
- Verify permissions: Ensure you have the necessary administrative rights for large-scale changes, particularly for system variables.
- Check syntax: Always double-check your command syntax to ensure no typographical errors have been made.
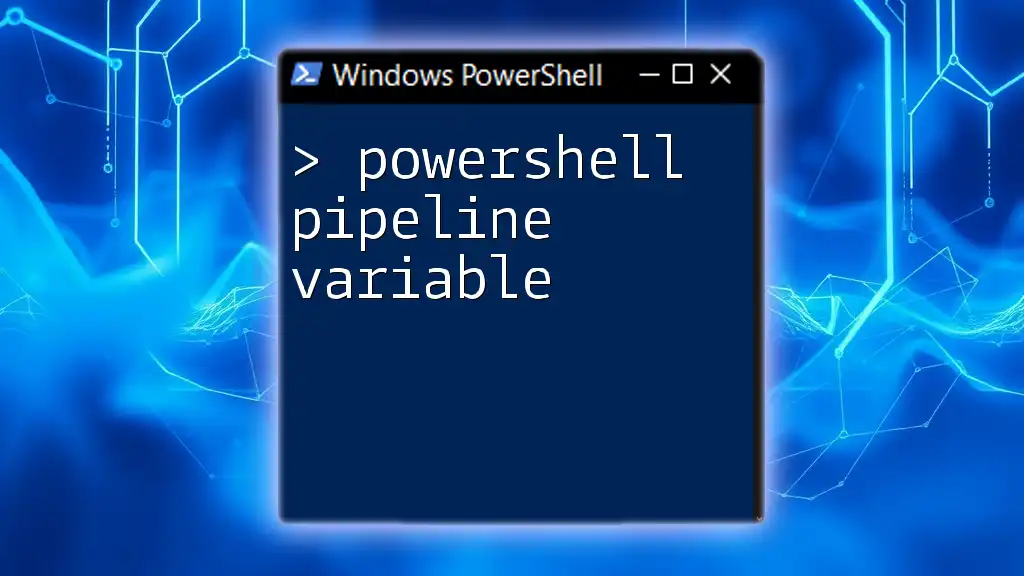
Best Practices for Managing Environment Variables in PowerShell
Regular Maintenance
Regular audits of your environment variables can aid in keeping your system organized and efficient. Remove any redundant or obsolete variables to ensure you're not cluttering your environment with unnecessary data.
Documentation
Documenting your environment variables is an excellent habit. Keeping a record of what each variable does can save you time when managing them in the future. Consider creating a standard operating procedure that outlines how to set, unset, and modify variables effectively.
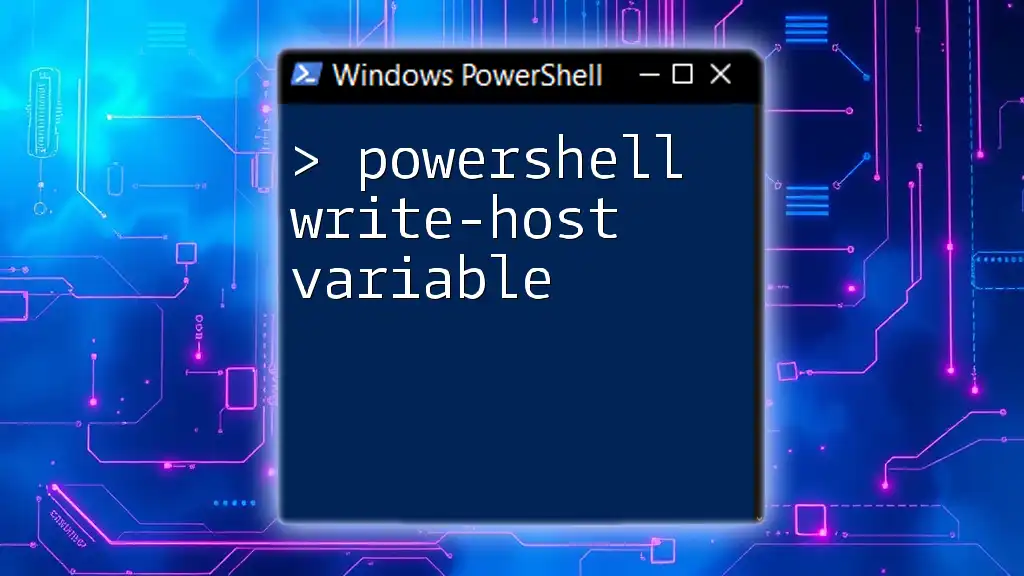
Conclusion
In summary, "PowerShell unset environment variable" operations play a crucial role in managing your work environment. Understanding how to properly use commands like `Remove-Item` and `setx` can empower you to effectively manage the variables that govern your system's behavior. Embrace these practices and continue to explore PowerShell commands to enhance your automation capabilities. Happy scripting!
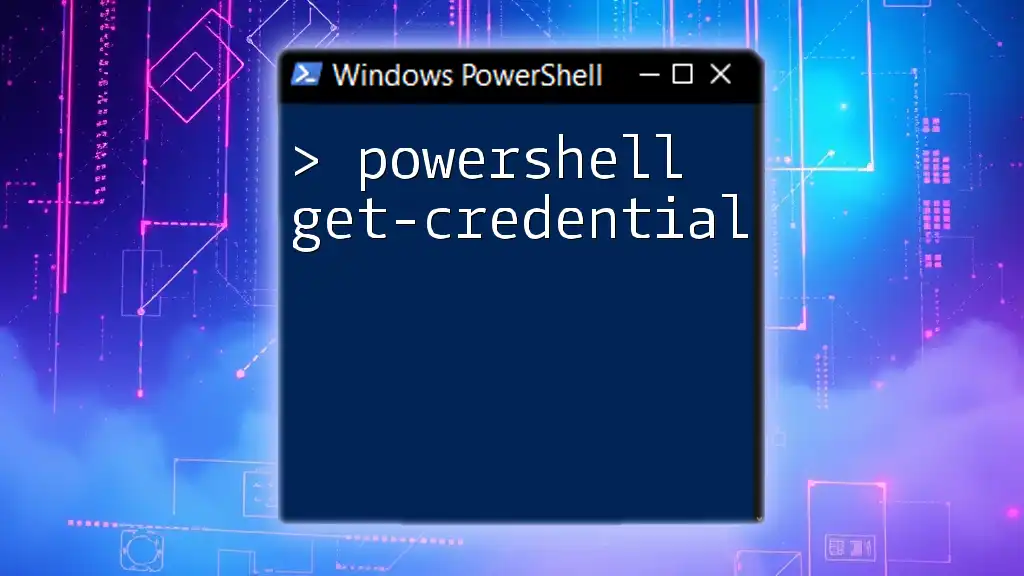
Additional Resources
For those who want to dive deeper into the world of PowerShell, consider checking the official PowerShell documentation, as well as recommended tutorials to broaden your understanding and skills.