Certainly!
In PowerShell, you can easily write JSON data to a file using the `ConvertTo-Json` command combined with `Set-Content` or `Out-File`.
Here’s a concise code snippet to demonstrate this:
$data = @{ Name = 'John Doe'; Age = 30; Occupation = 'Developer' }
$data | ConvertTo-Json | Set-Content -Path 'output.json'
Understanding JSON
What is JSON?
JSON, or JavaScript Object Notation, is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. Its simplicity and human-readable format has made it a popular choice for data exchange between a server and a web application, particularly in web APIs.
One of the main advantages of JSON is its ability to represent complex data structures, including arrays and nested objects, while maintaining a minimalistic syntax. Unlike older formats like XML, which can be verbose and complicated, JSON is compact and straightforward.
Typical Use Cases for JSON
JSON is widely used across various applications:
- Web APIs and Data Exchange: Nearly every modern web service uses JSON as a format for sending data back and forth.
- Configuration Files: Many applications favor JSON for configuration files due to its clarity and ease of modification.
- Data Storage: Lightweight databases often utilize JSON for storing structured data.
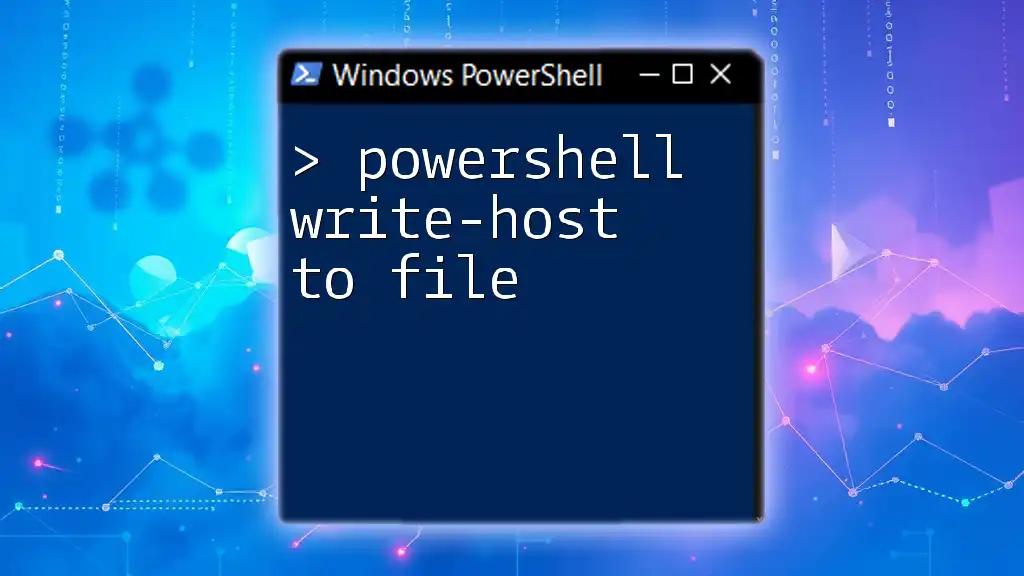
Overview of PowerShell
What is PowerShell?
PowerShell is a task automation and configuration management framework developed by Microsoft. It includes a command-line shell and an associated scripting language that allows users to manage and automate the administration of the operating system and applications.
One of the key advantages of PowerShell is its strong integration with .NET, making it ideal for handling structured data formats like JSON. Additionally, PowerShell can operate across different platforms, including Windows, macOS, and Linux, making it a versatile tool for developers and IT professionals.
Why Use PowerShell for JSON Operations?
Using PowerShell for JSON operations comes with several benefits:
- Integration with .NET Features: PowerShell seamlessly interacts with .NET libraries, providing robust functionality for data manipulation.
- Simplicity and Efficiency of Commands: The syntax is straightforward, making it quick to write and execute.
- Cross-Platform Capabilities: PowerShell Core allows you to manage JSON files across various operating systems without compatibility issues.
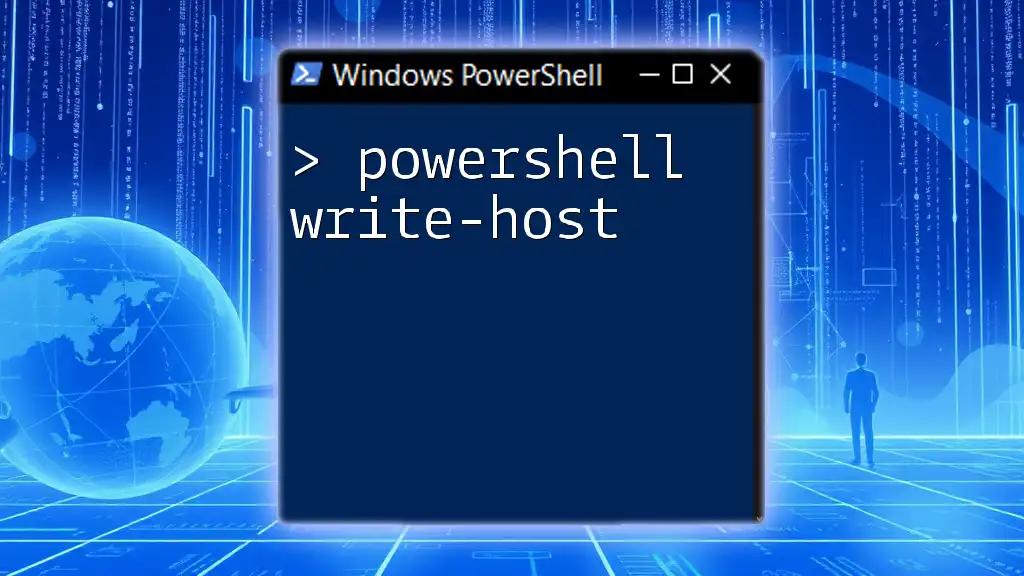
Writing JSON Data to a File in PowerShell
Basic Steps to Write JSON
Writing JSON data to a file in PowerShell involves several key steps:
- Definition of the Data Structure: You need to create an object or an array that contains the data you want to store.
- Conversion to JSON Format: Utilize the `ConvertTo-Json` cmdlet to transform your PowerShell object into a JSON string.
- Writing to a File: The final step is saving this JSON string to a file using `Out-File` or `Set-Content`.
Example 1: Writing a Simple JSON Object to a File
Here's a straightforward example illustrating how to write a simple JSON object to a file:
# Creating a hashtable
$person = @{
Name = "John Doe"
Age = 30
City = "New York"
}
# Converting to JSON
$json = $person | ConvertTo-Json
# Writing to a file
$json | Out-File -FilePath "person.json"
In this example, we create a hashtable representing a person, convert it to JSON, and then write it to `person.json`. The resulting file contains a JSON representation of the hashtable.
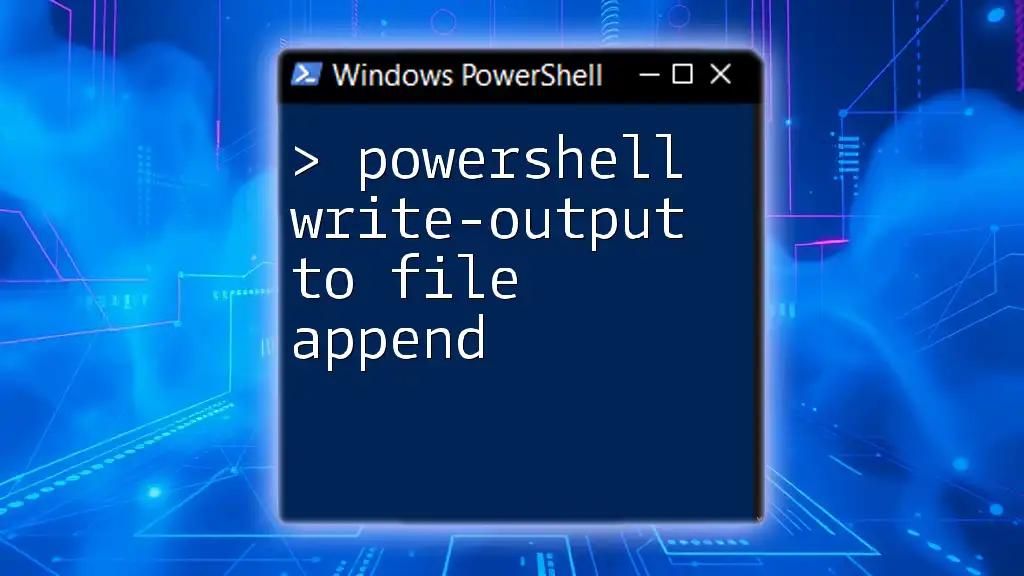
Formatting JSON Output
Customizing Output
When dealing with complex objects, you might want to customize how your JSON output appears. This can be done through two main methods:
- Adjusting Depth: The `-Depth` parameter in `ConvertTo-Json` allows you to specify how deeply nested objects you want to serialize.
- Using Pretty Print: While PowerShell does not provide native support for pretty printing, you can use a combination of JSON formatting libraries or manipulate the output string for enhanced readability.
Example 2: Formatting JSON with a Custom Depth
Consider a more complex object where our JSON needs more detail:
# Creating a complex object
$person = @{
Name = "Jane Doe"
Age = 25
Address = @{
Street = "456 Oak Avenue"
City = "Los Angeles"
}
}
# Converting to JSON with a custom depth
$json = $person | ConvertTo-Json -Depth 3
# Writing to a file
$json | Out-File -FilePath "person_detailed.json"
In this example, we increase the depth to ensure that nested objects are fully captured in the JSON output. The `-Depth 3` parameter guarantees that all properties of the nested `Address` object are included.
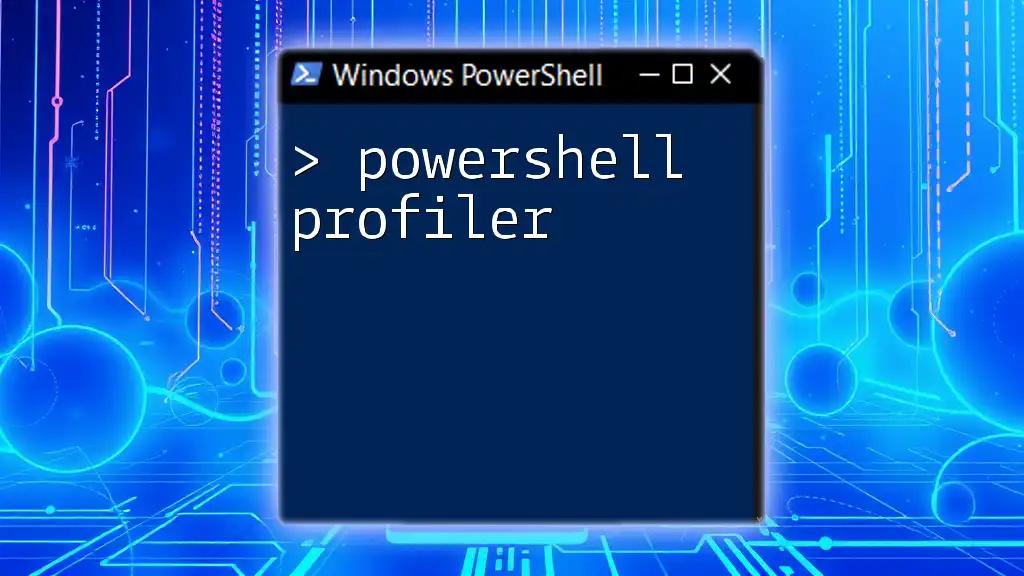
Reading JSON Data from a File
Using PowerShell to Read JSON
Retrieving JSON data is equally simple. PowerShell provides commands to read JSON from a file and convert it back to a usable object format.
Example 3: Reading and Outputting JSON Data
Here's a practical example of reading JSON data from a file and displaying it:
# Reading the JSON file
$jsonContent = Get-Content -Path "person.json" -Raw
# Converting from JSON to PowerShell object
$personObject = $jsonContent | ConvertFrom-Json
# Outputting the data
$personObject
In this case, we read the contents of `person.json` with `Get-Content` and convert it back into a PowerShell object using `ConvertFrom-Json`. The output will display the properties of the original object.
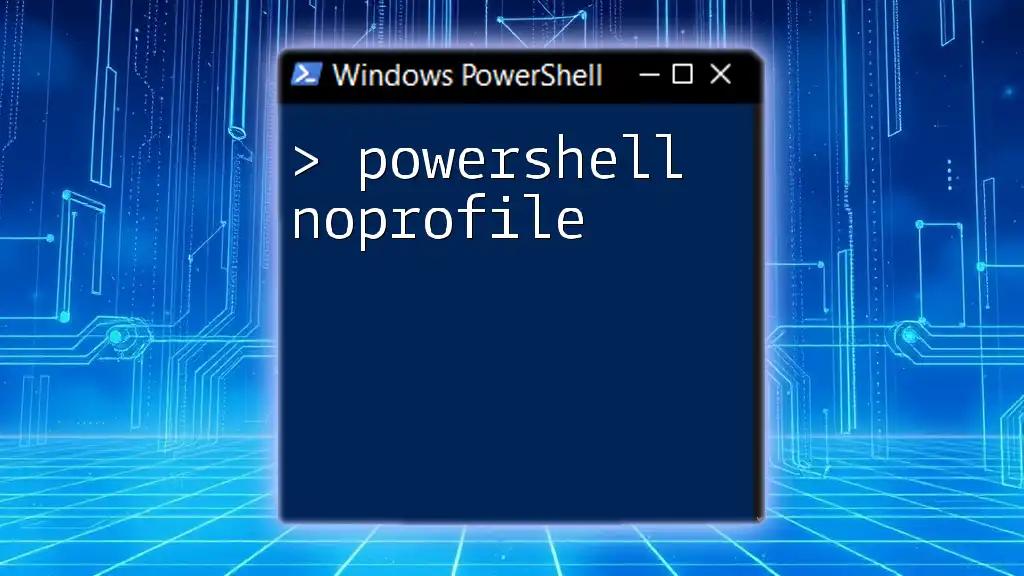
Common Errors and Troubleshooting
Common Issues When Writing JSON Files
While working with JSON, you may face some challenges, such as:
- Formatting Errors: Incorrect syntax in your JSON can lead to failure when attempting to write to a file.
- Permissions Issues: Ensure you have the appropriate permissions to create or modify files in the specified directory.
- Understanding PowerShell's Handling of Special Characters: Be mindful of special characters, which may require escaping.
Troubleshooting Tips
To mitigate issues:
- Verify JSON Syntax: Always check your JSON format using online validators or tools.
- Check File Permissions: Ensure that the script has write access to the target file directory.
- Use Debugging Output: Utilize `Write-Host` to display values during script execution, which can help track down issues.
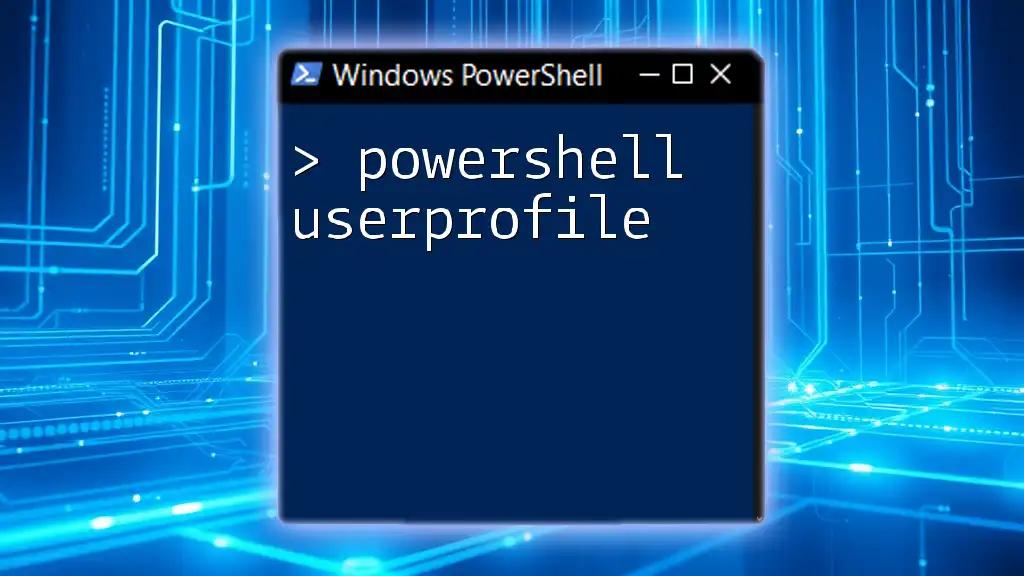
Best Practices
Structuring JSON Data
When crafting your JSON data, consider:
- Recommendations for Organizing Data Structures: Aim for clear, logical structures that reflect the data relationships accurately.
- Importance of Naming Conventions: Use consistent naming conventions to help other users understand your JSON data easily.
Performance Considerations
When writing large JSON files, it's essential to keep performance in mind:
- When to Avoid Large JSON Files: Consider breaking large data sets into smaller chunks if possible.
- Managing Memory and Processing Time: Monitor resource utilization, especially if processing big data sets.
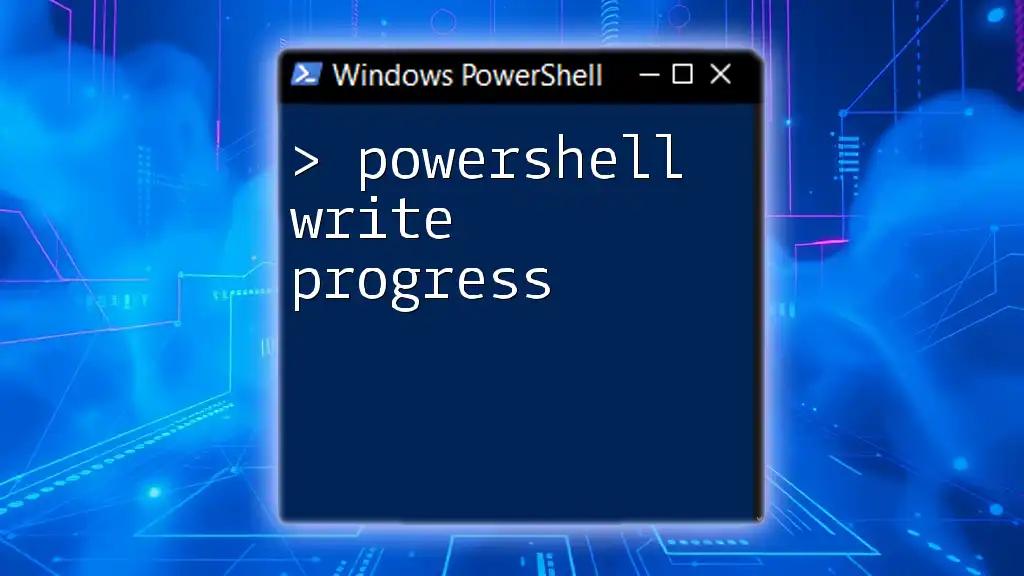
Conclusion
In summary, PowerShell is a powerful tool for writing JSON to files. By understanding the core concepts of JSON, leveraging the capabilities of PowerShell, and following best practices, you can efficiently manage JSON data. Practice writing and reading JSON files using PowerShell commands, and you'll find it becomes an invaluable skill in your development toolkit.
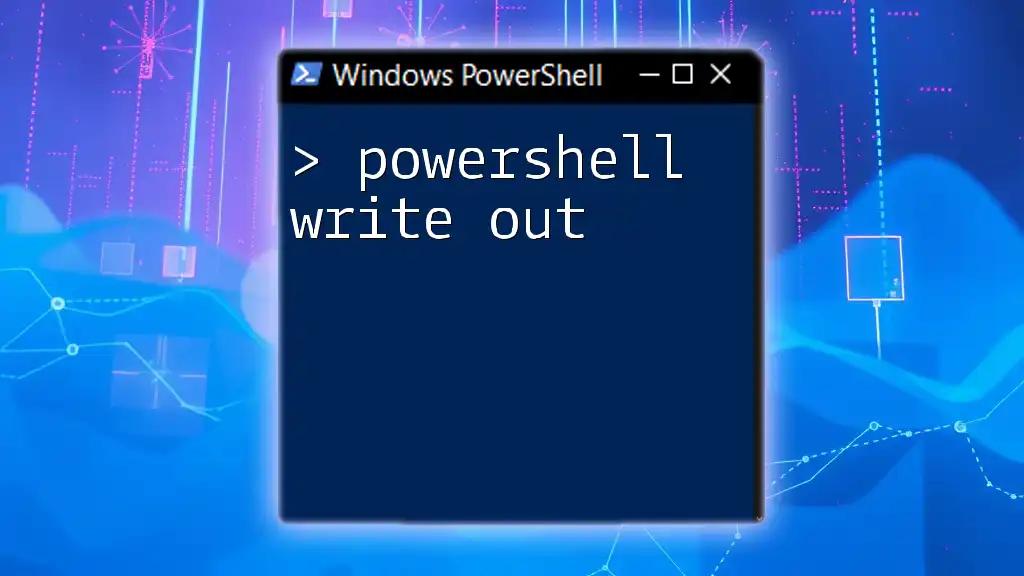
Call to Action
To continue enhancing your PowerShell skills, consider subscribing to our newsletter for more tips and tutorials. Explore related articles or courses we offer to deepen your knowledge about PowerShell commands and scripting.