In PowerShell, you can pipe the output of one command to another command, allowing you to pass data seamlessly for further processing, as demonstrated in the following code snippet:
Get-Process | Sort-Object CPU -Descending | Select-Object -First 5
This command retrieves all running processes, sorts them by CPU usage in descending order, and selects the top five processes.
Introduction to PowerShell Piping
What is Piping?
Piping in PowerShell is a feature that allows you to send the output of one command directly into another command for further processing. This chaining of commands enables users to build complex workflows in a simple and efficient manner. The pipe operator, denoted by the vertical bar `|`, connects the output of one command with the input of another.
Why Use Pipes?
Using the PowerShell pipe to direct output into another command significantly enhances productivity and readability. Rather than executing multiple commands individually and storing outputs in variables, you can seamlessly transition from one operation to another, reducing the amount of code you write. For instance, in system administration, it’s common to filter, sort, or select specific data, which can be effortlessly done using pipes. This capability allows you to quickly retrieve useful information without cluttering your script with intermediate variables.

The Basics of Piping in PowerShell
Understanding the Pipe Operator
The pipe operator `|` is the keystone of piping in PowerShell. It takes the output from the command on its left and passes it to the command on its right. This simple operation can drastically simplify your tasks.
The general syntax of using pipes in PowerShell looks like this:
Command1 | Command2
How Data Flows Through Pipes
Understanding how data flows through pipes is crucial. Each command in PowerShell processes objects rather than plain text, making it easier to manipulate. When data is passed through the pipeline, the objects created by the first command are sent to the next.
For example, consider the following command:
Get-Process | Where-Object { $_.CPU -gt 100 }
In this case, the output of `Get-Process`, which retrieves all running processes, is piped into `Where-Object`, which filters those processes to only show ones that utilize more than 100 CPU units.
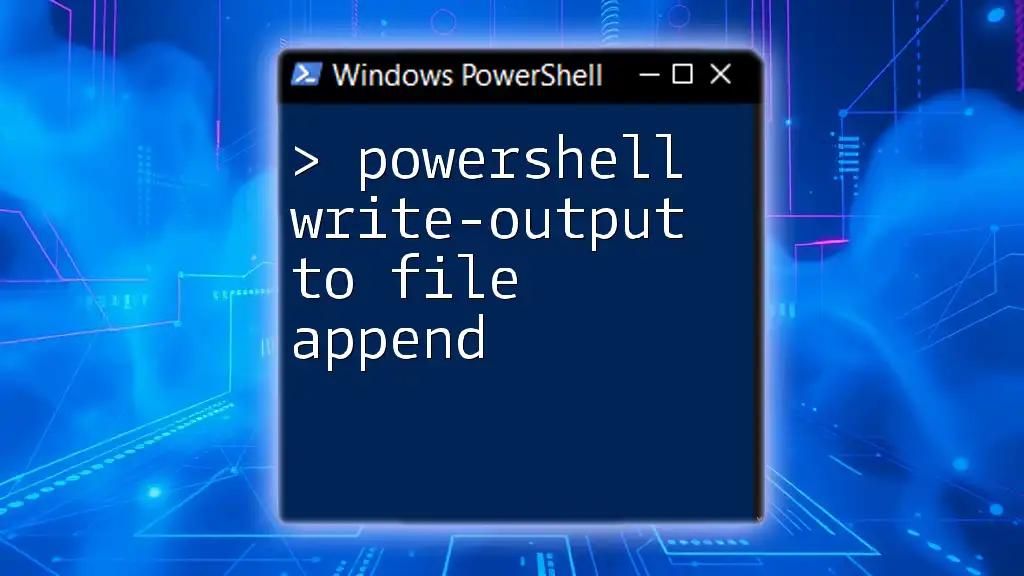
Using Pipeline Output with Specific Commands
Filtering Output with Where-Object
One of the powerful aspects of piping is the ability to filter the output with `Where-Object`. This allows for more focused data manipulation based on specified conditions.
For example, if you want to view only those processes that are consuming more than 2 MB of memory, you would write:
Get-Process | Where-Object { $_.WorkingSet -gt 2MB }
Here, `Where-Object` processes each object outputted by `Get-Process`, checking if the `WorkingSet` property exceeds 2 MB.
Sorting Output with Sort-Object
Sorting the output within the pipeline can be done using `Sort-Object`. This command sorts the results based on specified properties, making it easier to analyze large data sets.
Consider this example where we want to sort processes by their CPU consumption in descending order:
Get-Process | Sort-Object CPU -Descending
With this sequential processing, you can immediately see the processes that are consuming the most CPU at the top of the list.
Selecting Specific Properties with Select-Object
To refine your output even further, you can use `Select-Object` to display only the properties you are interested in. This not only makes the output clearer but also speeds up the processing.
For instance, if you only need the name and status of services, you can execute:
Get-Service | Select-Object Name, Status
This command cuts through unnecessary information, allowing you to focus on just the relevant columns.
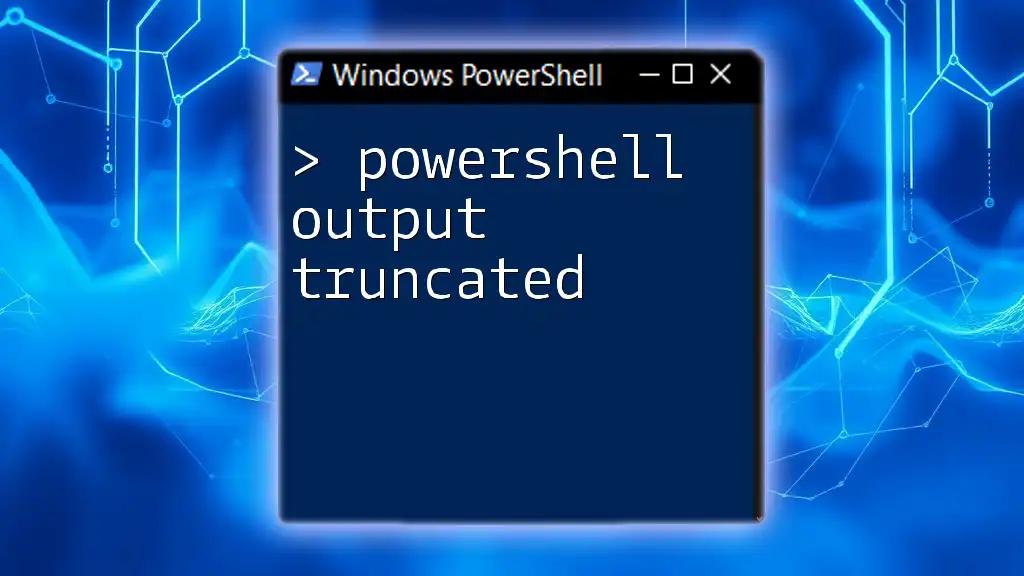
Advanced Piping Techniques
Using Multiple Pipes
For more complex operations, you can chain commands together using multiple pipes. This ability allows for intricate data workflows within a single line of code.
An example of using multiple pipes would be:
Get-EventLog -LogName Application | Where-Object { $_.EntryType -eq 'Error' } | Sort-Object TimeGenerated | Select-Object -First 10
In this command, you first retrieve application logs, filter out just the error entries, sort those entries by when they occurred, and then select the top ten. This illustrates how powerful and concise your scripting can be when utilizing piping effectively.
Error Handling in Piping
Understanding Errors in Pipelines
Errors are an inherent part of scripting, and it is essential to understand how they propagate through a pipeline. If a command fails in the middle of a pipeline, subsequent commands will not execute. To handle errors effectively, you can use `Try-Catch`.
For instance, if you want to read a file but handle errors gracefully should it not exist, you would write:
Try {
Get-Content "C:\NonExistentFile.txt" | Out-Null
} Catch {
Write-Host "An error occurred!"
}
This technique ensures that your script continues to run even when unexpected errors are encountered.
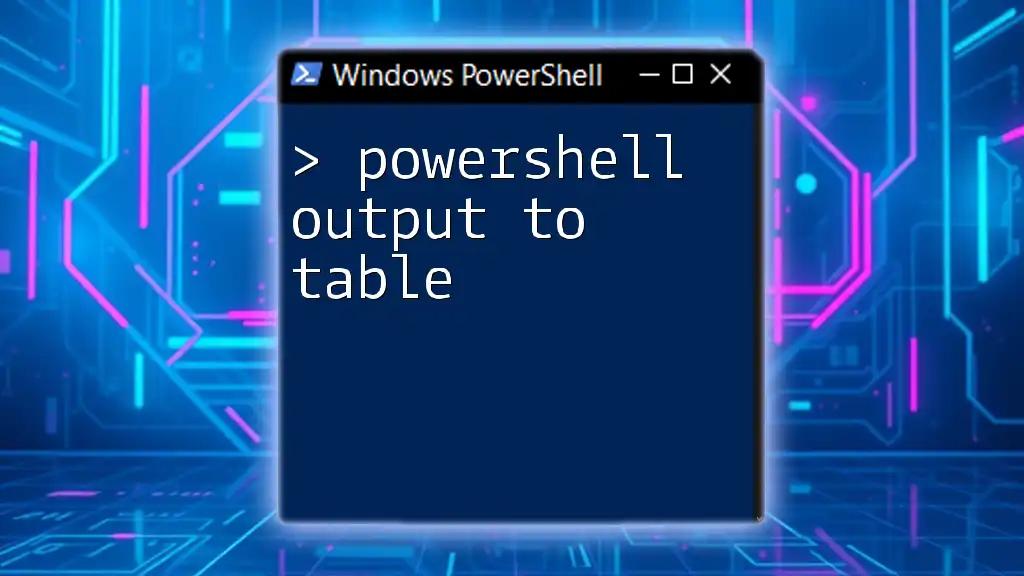
Best Practices for Using Pipes
Keep Commands Simple and Concise
One of the critical rules of using pipes effectively is to keep commands uncomplicated and easy to read. Complex commands can be difficult to manage and debug, leading to potential confusion. For better clarity, it’s often wise to break complicated operations into simpler steps.
Leverage Cmdlet Capabilities
Choosing the right cmdlet for the job is paramount. Leverage the inherent capabilities of cmdlets to minimize the number of commands you need to use. For example, if a cmdlet has a built-in filtering option, use it instead of relying on multiple pipes wherever possible.
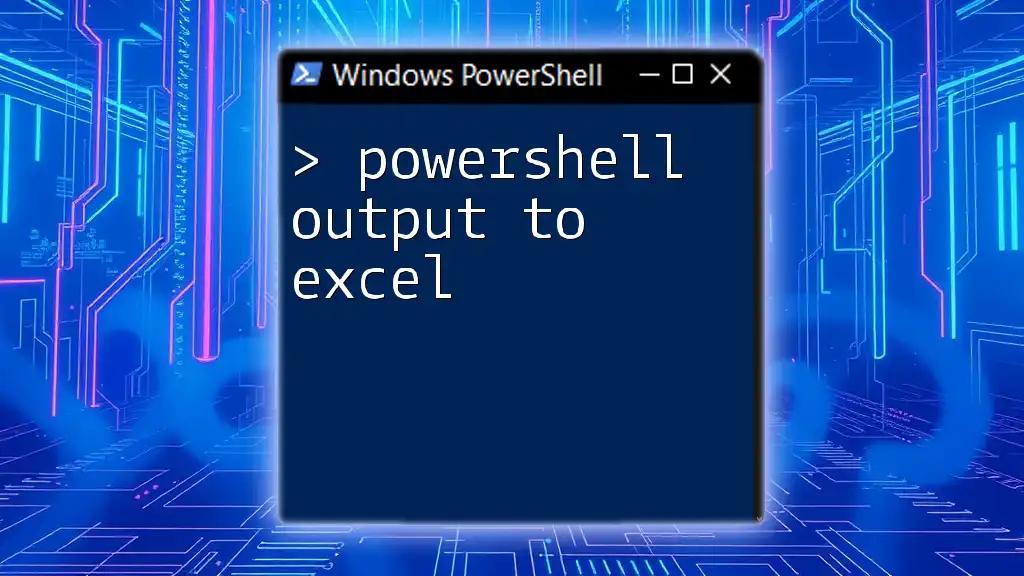
Common Pitfalls to Avoid
Inefficiencies in Commands
Certain pitfalls, such as inefficiencies arising from improper command structuring, can obscure the intended results. Be mindful of how each command processes object types being passed through.
Understanding Object Types in Pipelines
An essential aspect of successful piping involves recognizing the types of objects being utilized. Understanding their properties and methods allows for more precise control and manipulation of data. For instance, if you incorrectly pass a string to a command expecting an object, it could lead to errors or unpredictable results.
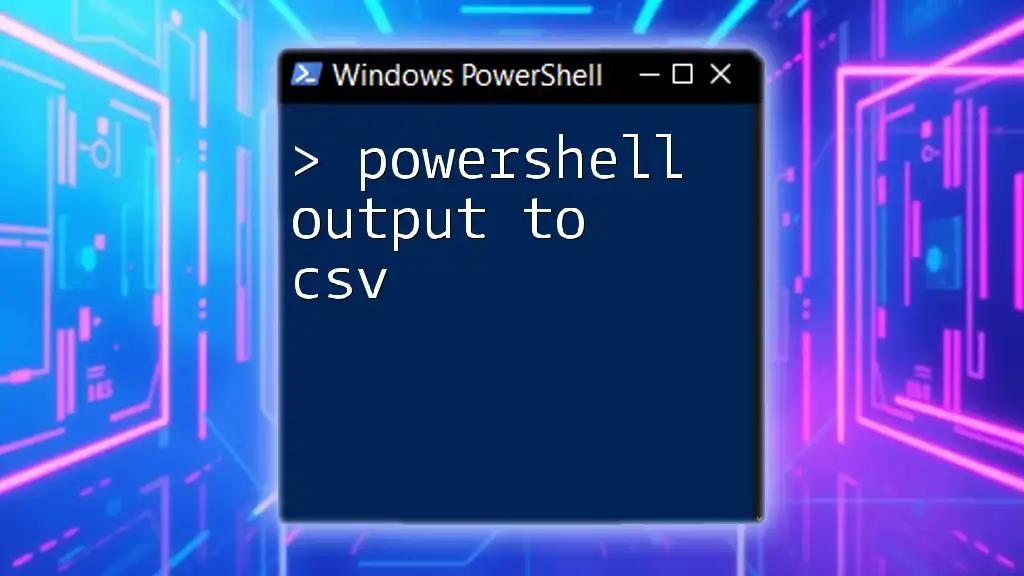
Conclusion
PowerShell piping is an essential skill for any user looking to streamline their operations in a command-line environment. By mastering how to pipe output to another command, you can execute complex tasks with remarkable efficiency. As you begin to experiment with your own PowerShell scripts, remember to keep your commands simple, utilize cmdlet capabilities wisely, and handle errors gracefully.
Engage with this powerful tool, and don't hesitate to push the boundaries of what you can achieve with piping in PowerShell!