To add a user to an Active Directory group using PowerShell, you can utilize the `Add-ADGroupMember` cmdlet, as shown in the following code snippet:
Add-ADGroupMember -Identity "GroupName" -Members "Username"
Understanding Active Directory Groups
Active Directory (AD) groups are logical collections of user accounts, computers, and other groups. These groups serve a variety of purposes, primarily regarding security and organization within an environment. Two main types of AD groups exist: security groups and distribution groups.
- Security Groups grant permissions to shared resources, enabling you to easily assign access rights to multiple users at once.
- Distribution Groups are primarily used for email distribution lists and do not have security-related functions.
Managing these groups effectively is crucial for ensuring proper access control, improving efficiency across departments, and facilitating easier administration of users.
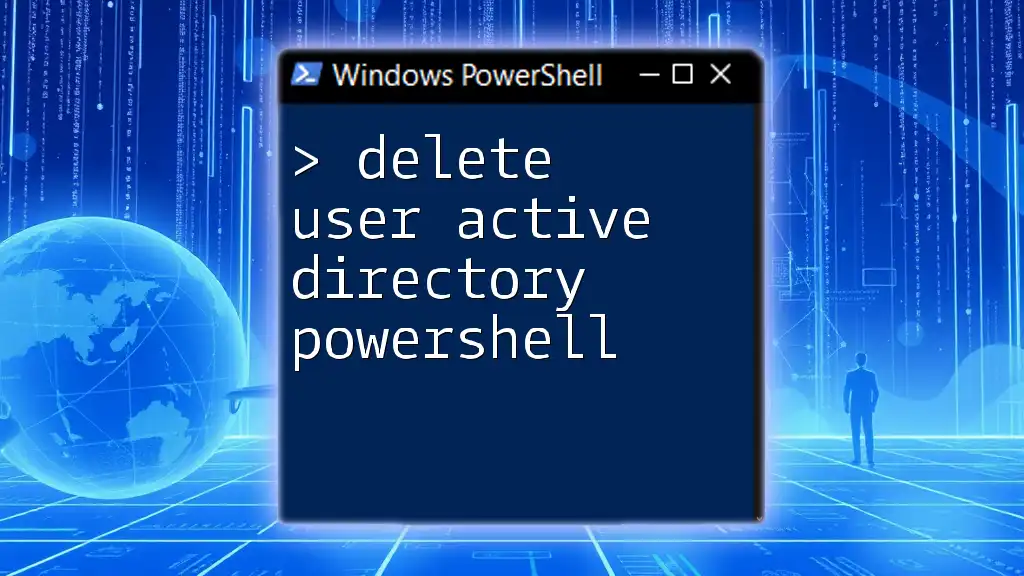
Prerequisites for Using PowerShell with Active Directory
Before diving into how to add a user to an Active Directory group using PowerShell, ensure that:
-
You are using a compatible version of PowerShell, typically Windows PowerShell 5.0 or higher. This ensures access to the necessary cmdlets.
-
The Active Directory module for Windows PowerShell is installed and imported into your session. You can do this by running the command:
Import-Module ActiveDirectory
-
You have the necessary permissions to manage AD groups. Make sure that your account has at least the "Account Operators" role or is part of the "Domain Admins" group to avoid errors.
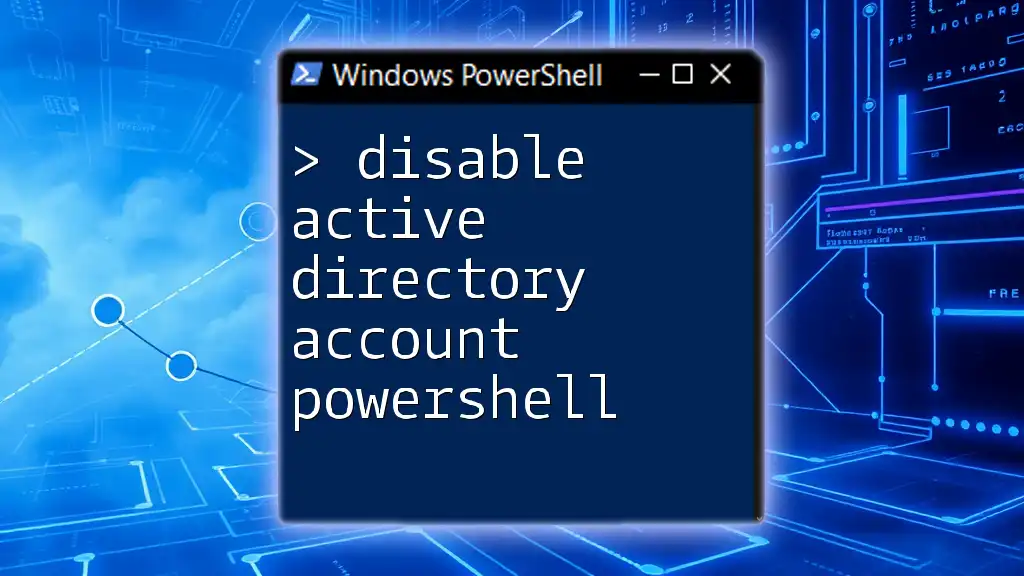
PowerShell Basics for Active Directory Management
Using PowerShell for Active Directory management not only improves efficiency but also allows for automation of repetitive tasks. Familiarizing yourself with some key cmdlets is essential.
- `Get-ADUser`: Retrieves user account details from Active Directory.
- `Get-ADGroup`: Gets information about specific groups.
- `Add-ADGroupMember`: The primary cmdlet used to add users to a group.
This streamlined approach makes it easier to manage users and their permissions, especially in larger organizations.
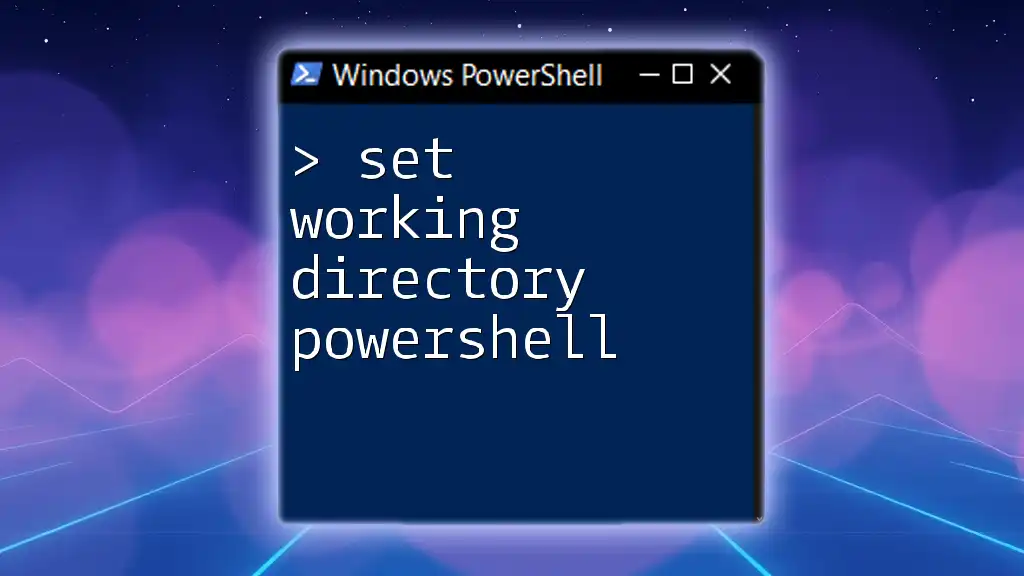
How to Add a User to an Active Directory Group with PowerShell
The primary cmdlet to add a user to an Active Directory group is `Add-ADGroupMember`. The basic syntax is straightforward:
Add-ADGroupMember -Identity "GroupName" -Members "Username"
Example Code Snippet
If you want to add a user named "jdoe" to a group called "SalesTeam", you would run:
Add-ADGroupMember -Identity "SalesTeam" -Members "jdoe"
This command explicitly states which group you are modifying and provides the username of the member to add.
Adding Multiple Users to an AD Group
A powerful feature of PowerShell is the ability to add multiple users in a single command. You can use arrays or lists to efficiently add several members at once.
Example Code Snippet
To add "user1", "user2", and "user3" to the "SalesTeam" group, your code would look like this:
$users = "user1", "user2", "user3"
Add-ADGroupMember -Identity "SalesTeam" -Members $users
This approach significantly reduces the time needed to manage group memberships, especially for larger user bases.
Using a CSV File to Add Users
For bulk additions, using a CSV file is one of the most effective methods. You’ll first need to prepare a CSV file containing user details, typically with a column labeled "Username".
Example Code Snippet
Assuming you have a file named `Users.csv`, the following command will import users and add them to the specified group:
Import-Csv "Users.csv" | ForEach-Object {
Add-ADGroupMember -Identity "SalesTeam" -Members $_.Username
}
This command reads through each line of the CSV and adds the users to the "SalesTeam" group based on the data provided.
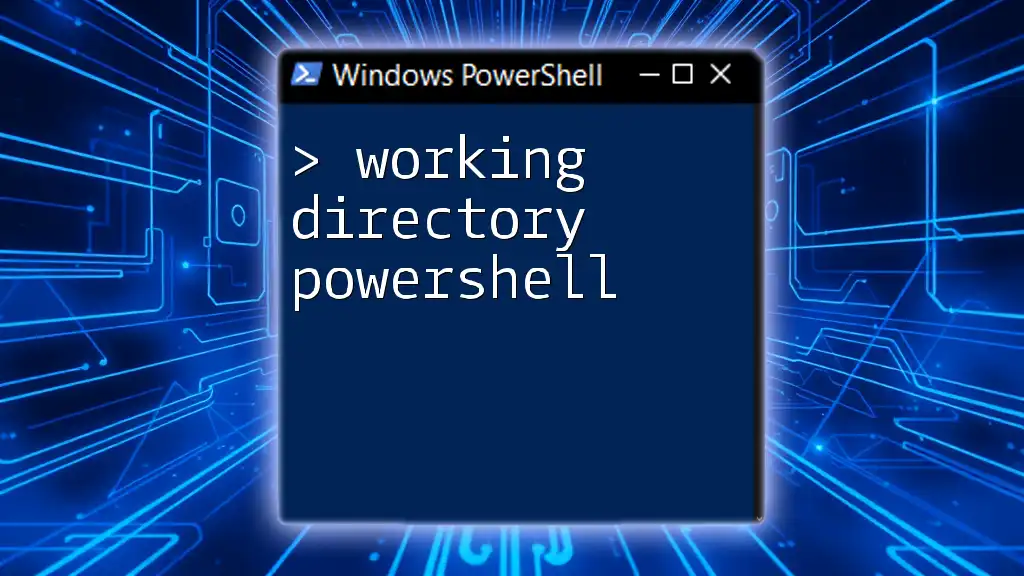
Common Errors and Troubleshooting
When working with PowerShell to add users to AD groups, several common errors may occur:
-
Inadequate Permissions: If you lack the necessary permissions, you'll receive an error message. Always confirm that you have the correct roles assigned.
-
User or Group Not Found: This can occur if the specified username or group name is incorrect. Double-check the values to ensure accuracy.
Troubleshooting Tips
If you encounter issues, use these commands to verify user and group existence before attempting to add users:
To check if a user exists:
Get-ADUser -Identity "jdoe"
To check if a group exists:
Get-ADGroup -Identity "SalesTeam"
These commands are crucial for diagnosing problems related to nonexistent users or groups.
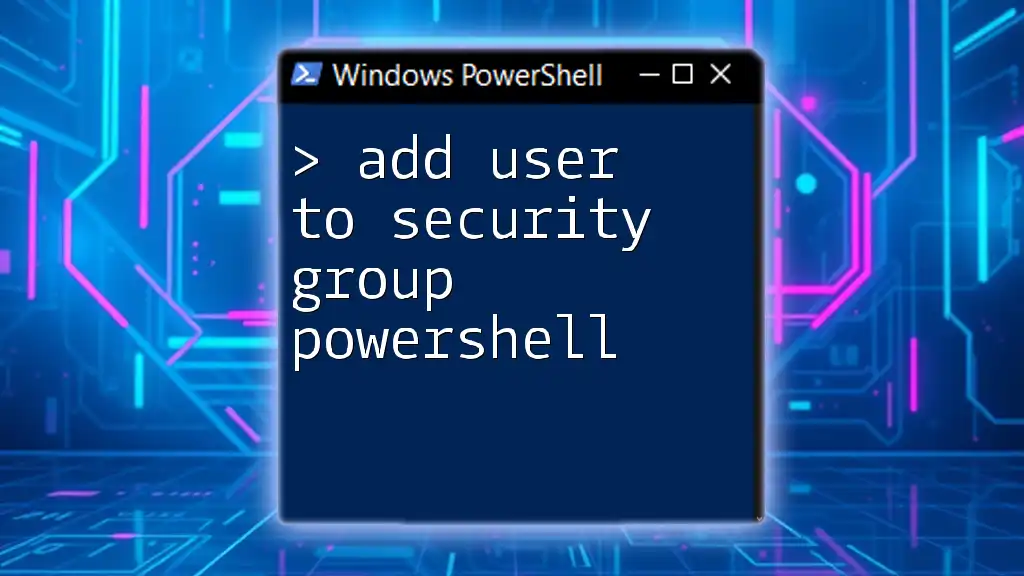
Best Practices for Managing Active Directory Groups with PowerShell
To maintain an efficient and effective management environment, consider these best practices:
-
Documentation and Comments: It's essential to annotate your scripts and commands adequately. This practice not only helps in understanding the code later but also benefits anyone else who may interact with it.
-
Regular Auditing of AD Groups: Periodically check group memberships to ensure compliance and proper management of resources. The following PowerShell command can help you identify existing group members:
Get-ADGroupMember -Identity "SalesTeam"
By routinely auditing AD groups, you can maintain a clean and organized directory structure, reducing the risk of unauthorized access.
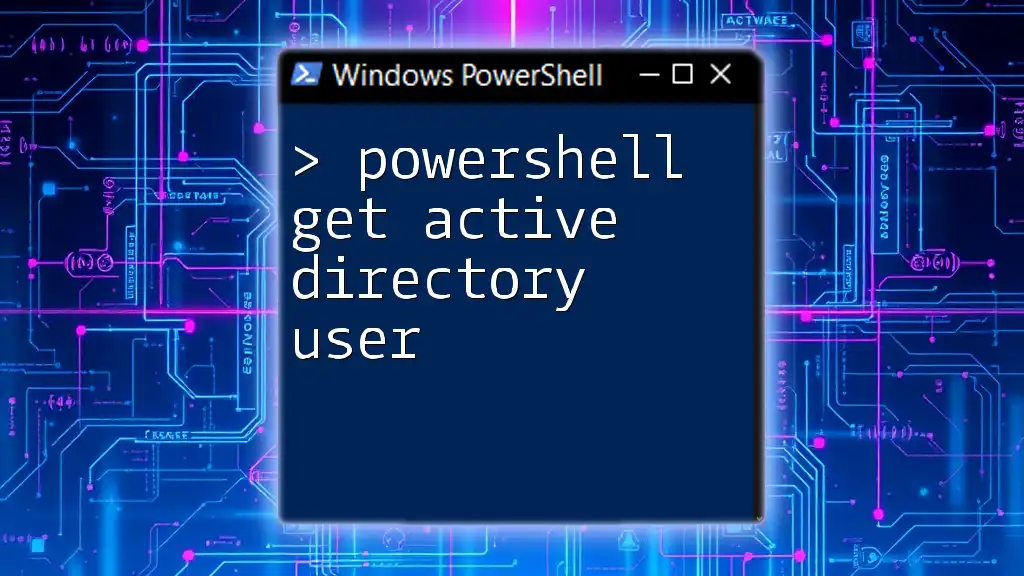
Conclusion
Mastering how to add a user to an Active Directory group using PowerShell not only streamlines user management but also enhances security protocols. By leveraging the provided examples and techniques, you can effectively manage group memberships efficiently.
Practice and experimentation will reinforce your understanding, making you a more adept IT professional. Always consider exploring further resources and communities to expand your PowerShell skills even more, paving the way for a deeper understanding of Active Directory management.