In PowerShell, you can set the working directory to a specific path using the `Set-Location` cmdlet (or its alias `cd`), allowing you to navigate your file system efficiently.
Set-Location 'C:\Your\Desired\Path'
Understanding Working Directory
What is a Working Directory?
A working directory in PowerShell refers to the folder that the system is currently focused on when executing commands. Think of it as the default location from which PowerShell will read and write files. When you work with PowerShell scripts or commands, the working directory helps streamline file management by defining where files are located without needing to specify the full path each time.
Why Set a Working Directory?
Setting a working directory is crucial for several reasons:
- Efficiency: It reduces the need to type long file paths for every command. When you've set your working directory, you can simply reference files relative to that directory.
- Error Prevention: Working outside of the intended directory can lead to errors and confusion, especially if files of the same name exist in different folders. By setting the working directory properly, you minimize the risk of these mistakes.
- Improved Organization: When working on specific tasks or projects, it's helpful to confine operations to a designated folder. This ensures that you are always addressing the correct files.
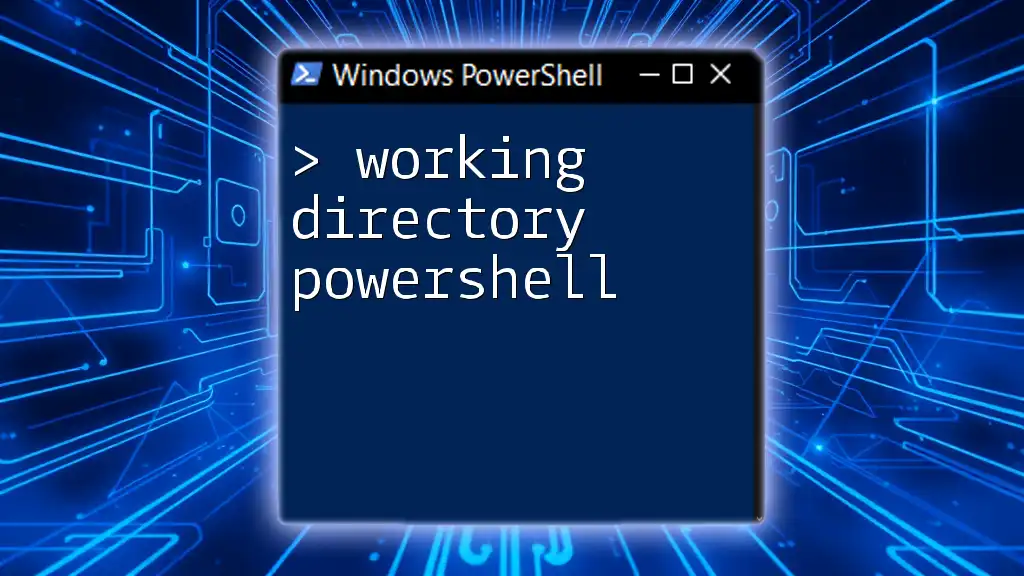
Setting the Working Directory in PowerShell
Using `Set-Location` Cmdlet
The `Set-Location` cmdlet is the primary command for setting your working directory in PowerShell. It allows you to change the current location to another specified location in the file system.
Basic Syntax
The basic syntax for `Set-Location` is as follows:
Set-Location -Path "C:\YourDirectory"
Example Usage
To set your working directory to your Documents folder, you would execute:
Set-Location -Path "C:\Users\YourUsername\Documents"
This command changes the current directory to the path provided. After executing it, any subsequent commands that reference files will be relative to this directory.
Using the Alias `cd`
PowerShell also provides an alias for the `Set-Location` cmdlet, which is `cd`. This shorthand is not only easier to type but feels familiar to users coming from other command-line interfaces.
Basic Syntax
You can use it just like the cmdlet:
cd "C:\YourDirectory"
Example Usage
Setting your working directory with the `cd` command can be done as follows:
cd "C:\Users\YourUsername\Documents"
Both `Set-Location` and `cd` achieve the same outcome; your choice depends on personal preference for command invocation.
Using `Push-Location` and `Pop-Location`
For users who need to navigate between multiple directories, `Push-Location` and `Pop-Location` are invaluable.
What are Push-Location and Pop-Location?
These cmdlets work together to allow you to manipulate your working directory stack. You can temporarily change your working directory and then return to the previous one without losing your place.
Example Usage of Push-Location
To save your current directory while switching to another, use `Push-Location`:
Push-Location "C:\Users\YourUsername\Documents"
This command saves your current location (the previous working directory) on a stack and then shifts to the specified directory.
Example Usage of Pop-Location
To return to the saved directory, you use:
Pop-Location
This command pops the last saved location off the stack and returns the context to it. This is particularly useful in scripts where you may need to navigate in and out of directories multiple times.
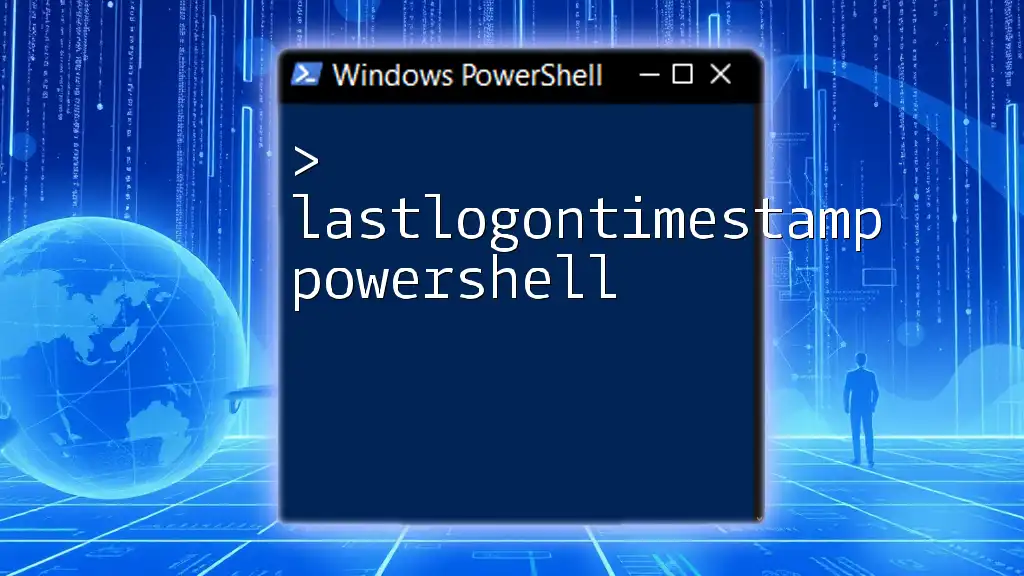
Verifying Your Working Directory
Using `Get-Location`
To check your current working directory, you can use the `Get-Location` cmdlet.
Example Usage
Simply execute:
Get-Location
This command returns the path of your current working directory, confirming where you are in the filesystem.
Outputting Path to Verify
You can also combine `Get-Location` with `Write-Host` to display the current path in a user-friendly manner:
Write-Host "Current Directory: $(Get-Location)"
This code snippet will output a message showing your current location, helping keep track of your working environment.
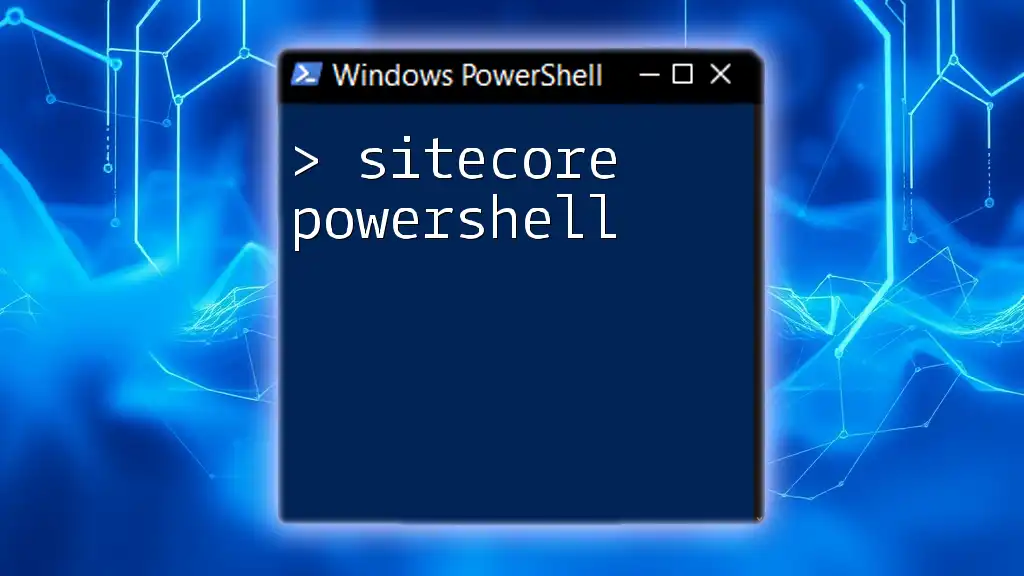
Best Practices for Managing Your Working Directory
Organizing Your Scripts
Maintaining an organized directory structure is essential when working with PowerShell. Creating dedicated folders for specific projects or scripts can significantly improve your workflow. This organization minimizes confusion and ensures that related scripts and files are stored together.
Using Variables for Directory Paths
For enhanced convenience, consider using variables to store directory paths. This method makes scripts cleaner and more maintainable. For example:
$myDirectory = "C:\Users\YourUsername\Documents"
Set-Location -Path $myDirectory
By declaring a variable for your path, you minimize the need to repeatedly type the full path, allowing for quick adjustments if the directory changes.
Error Handling
Working with file paths can lead to errors, especially if a specified directory does not exist. Implementing error handling using `Try-Catch` blocks can make your scripts more robust. For example:
Try {
Set-Location -Path "C:\InvalidPath"
} Catch {
Write-Host "Error: The specified path does not exist."
}
This allows you to catch exceptions gracefully when attempting to change to an invalid directory, preventing crashes and providing helpful feedback.
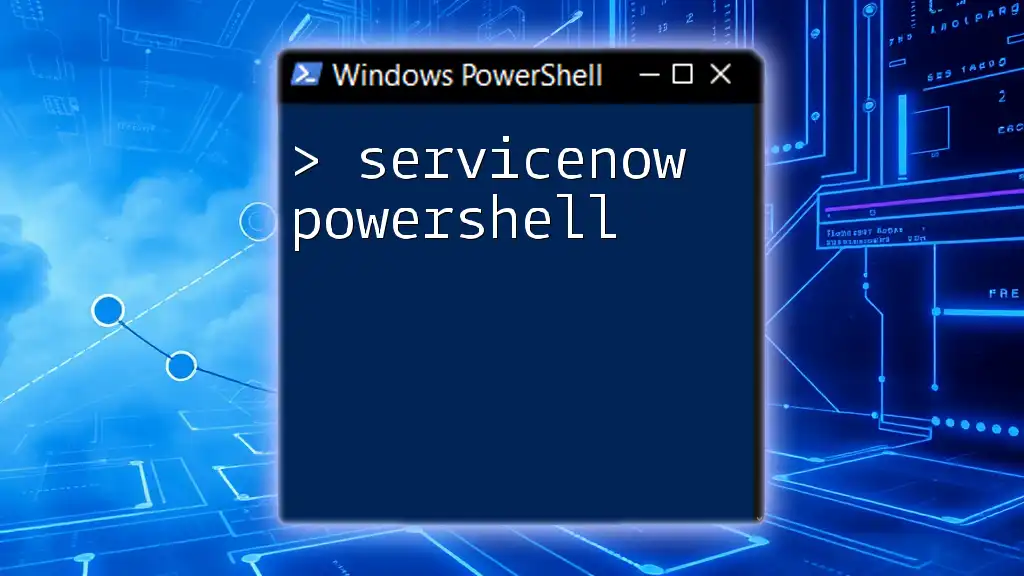
Conclusion
Setting the working directory in PowerShell is an integral part of managing your scripts and files effectively. By mastering the use of `Set-Location`, `cd`, and navigational commands like `Push-Location` and `Pop-Location`, you can facilitate a more efficient workflow while reducing the chance of errors in your commands. Regularly practicing these skills will enhance your productivity and confidence in using PowerShell command lines.
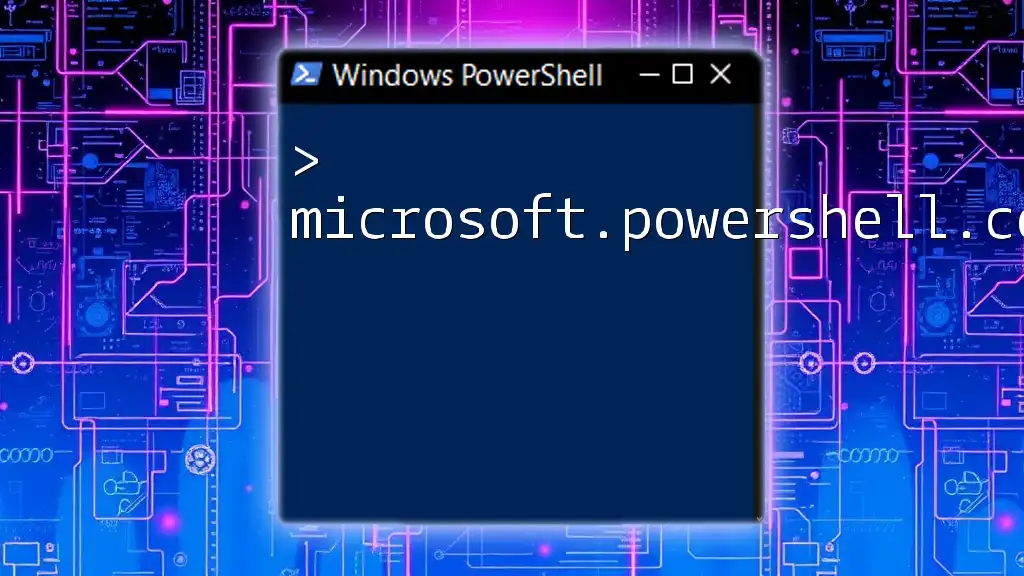
Additional Resources
For further information and advanced techniques, consult the official PowerShell documentation and explore community forums for tips and engaging tutorials that can assist in elevating your PowerShell proficiency.
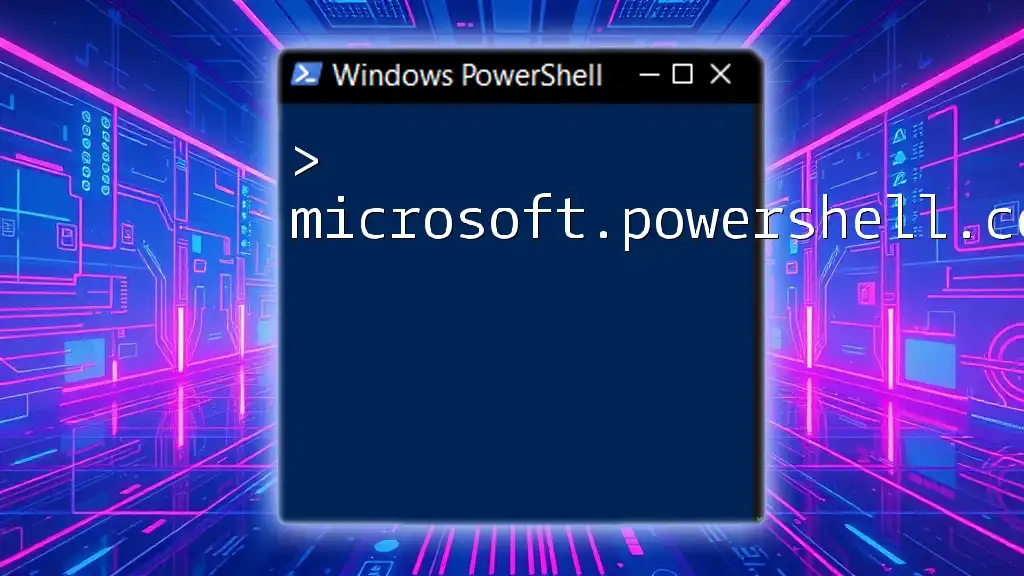
Call to Action
Don’t hesitate to practice setting your working directory using the commands outlined in this guide. Experiment with different directory structures, and feel free to ask questions or share your experiences in the comments section!