In PowerShell, a return code (also known as exit code) indicates whether a command was executed successfully or encountered an error, with a return value of `0` typically signifying success.
# Check the return code of a command
$process = Start-Process "notepad.exe" -PassThru
$process.WaitForExit()
if ($process.ExitCode -eq 0) {
Write-Host "The process completed successfully."
} else {
Write-Host "The process failed with exit code: $($process.ExitCode)"
}
What is a Return Code in PowerShell?
A return code, also known as an exit code, is a numerical value returned by a command-line application or script in PowerShell to signal whether its execution was successful or if an error occurred. Understanding return codes is crucial for effective scripting, as they provide insights into the success or failure of commands.
Return codes should not be confused with error messages. While an error message may describe an issue that occurred during execution, the return code provides a numeric representation of the execution state.
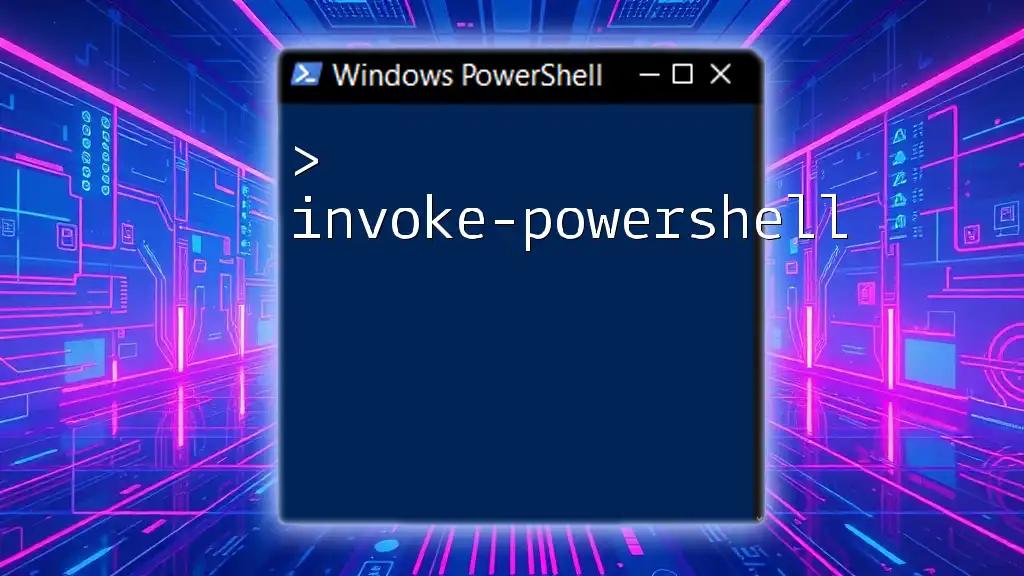
Understanding Exit Codes in PowerShell
Exit codes in PowerShell are derived from the underlying Windows operating system. When a process completes, it returns an exit code to its parent process. This code indicates the status of the process:
- Zero (0) typically indicates success.
- Non-zero values indicate different types of errors or conditions.
Using exit codes allows for easier debugging and control flow within scripts.
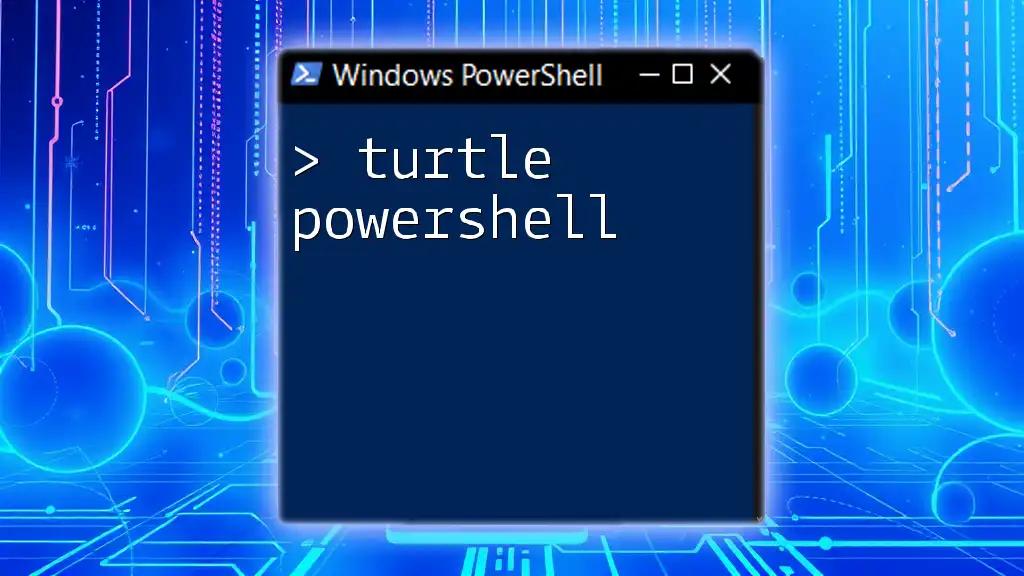
How to Retrieve Return Codes in PowerShell
To retrieve the last exit code from a command in PowerShell, you can simply use the automatic variable `$LASTEXITCODE`. This variable stores the exit code of the last Windows-based program that was added to the pipeline.
For example, after executing a command, you can check the exit code as follows:
$LASTEXITCODE
This command will display the exit code, helping you determine if the prior command executed successfully or if an error occurred.
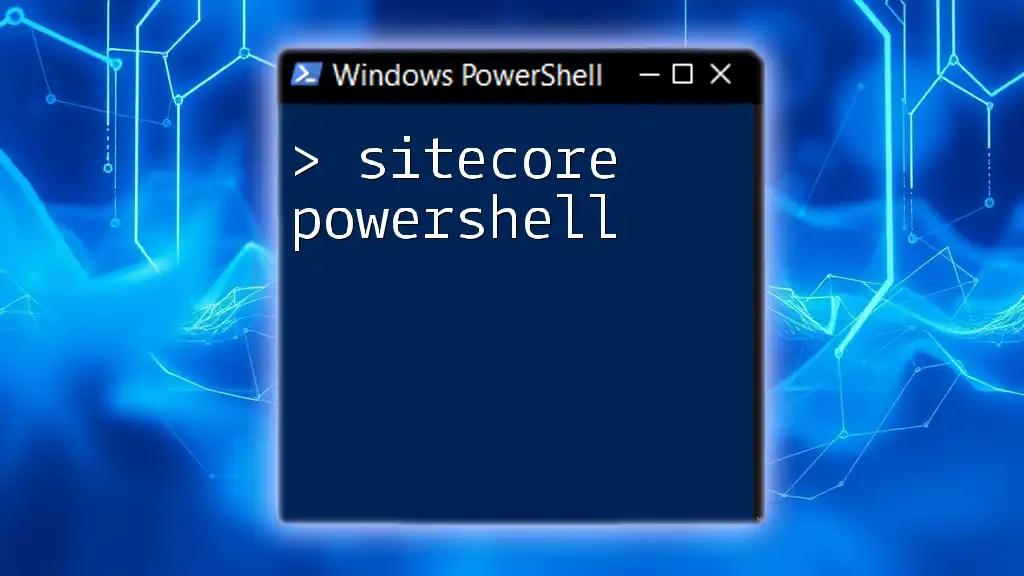
Common Exit Codes in PowerShell
PowerShell commands and scripts can yield various exit codes. Here are some of the standard exit codes you should be aware of:
- 0: Success - The command completed successfully without any errors.
- 1: General error - The command encountered a generic error.
- Other codes: Non-standard exit codes may indicate specific errors related to particular commands or applications.
Being aware of these common exit codes can greatly aid in troubleshooting issues when scripting.
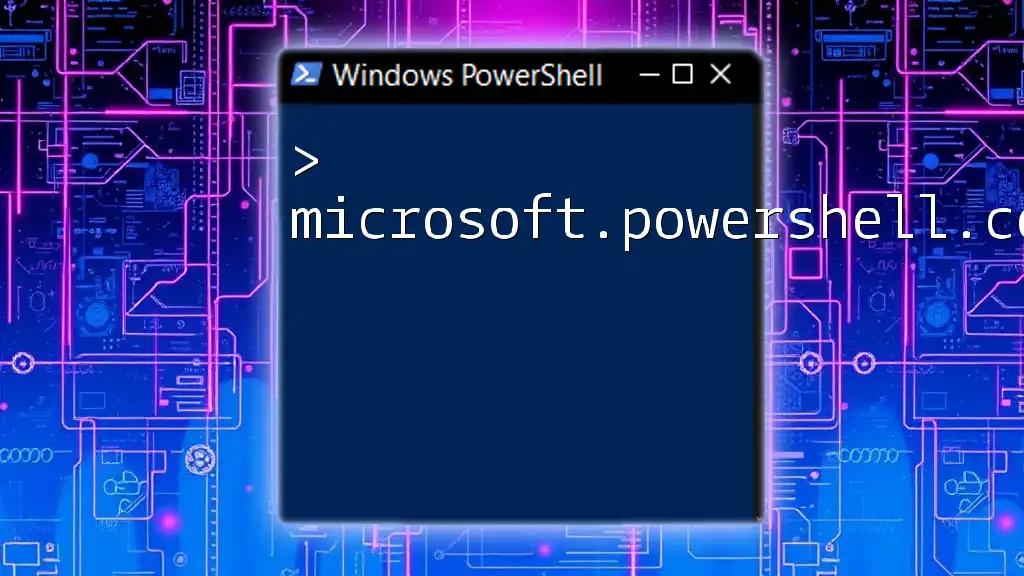
Using Return Codes for Error Handling
Return codes play a pivotal role in error handling. Leveraging these codes allows your scripts to react appropriately based on the success or failure of a command.
Consider the following example, where we check the exit code after executing a command:
Start-Process "SomeCommand" -NoNewWindow
if ($LASTEXITCODE -ne 0) {
Write-Host "Error detected with exit code: $LASTEXITCODE"
} else {
Write-Host "Command executed successfully."
}
In this code snippet, we use the `$LASTEXITCODE` variable to verify whether the command succeeded or failed. This allows for proactive error handling, resulting in more robust scripts.
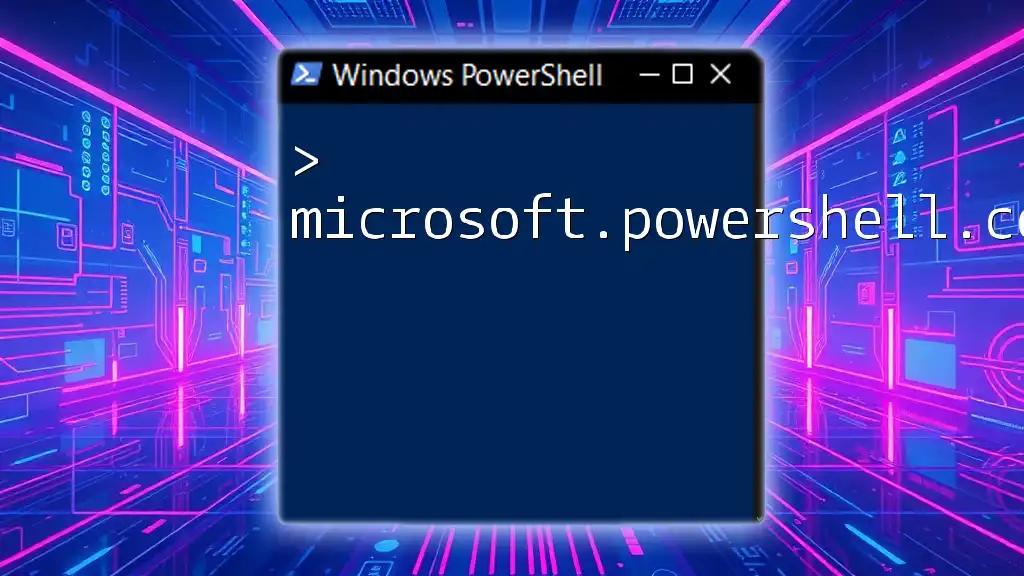
Return Codes in Conditional Statements
Return codes can be effectively integrated into `if` statements, enabling conditional processing based on the outcome of a command.
Here is an example:
$process = Start-Process "SomeCommand" -PassThru
$process.WaitForExit()
if ($process.ExitCode -eq 0) {
Write-Host "Process exited successfully."
} else {
Write-Host "Process failed with exit code: $($process.ExitCode)"
}
In this script, we initiate a process and wait for its completion. Upon completion, we check the `$process.ExitCode`. If it equals zero, the process was successful; otherwise, we display the failure code, facilitating effective error tracking.
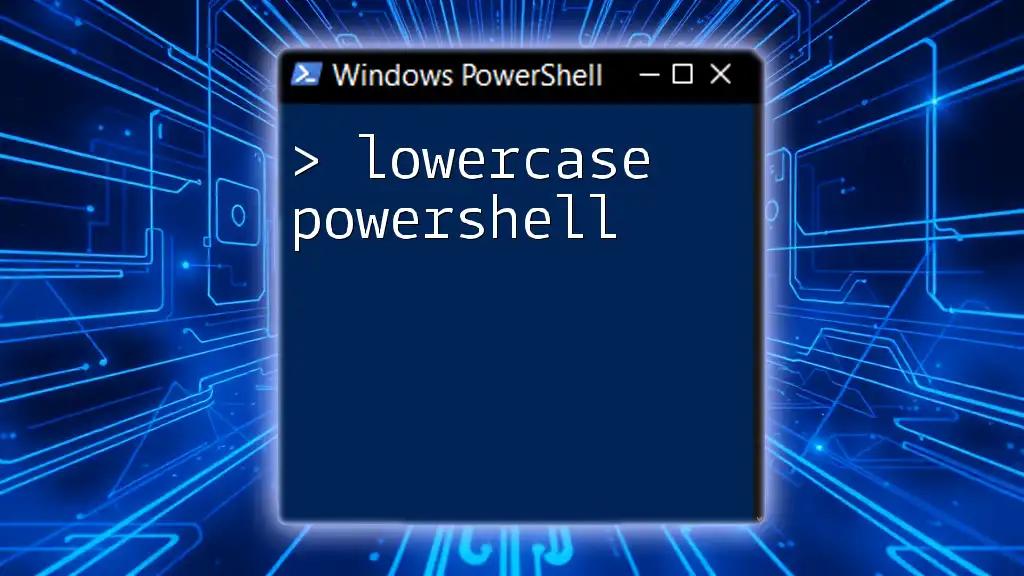
Best Practices for Using Return Codes
When utilizing return codes in PowerShell scripting, consider the following best practices:
- Consistency is Key: Ensure that your scripts follow a consistent approach to exit codes. This helps maintain clarity.
- Document Exit Codes: Clearly document the meaning of custom exit codes in your scripts. Doing so aids others in understanding your code better.
- Use Custom Exit Codes: For more complex scripts, consider using custom exit codes. This can help differentiate between various types of errors.
By adhering to best practices, you can enhance the maintainability and reliability of your PowerShell scripts.
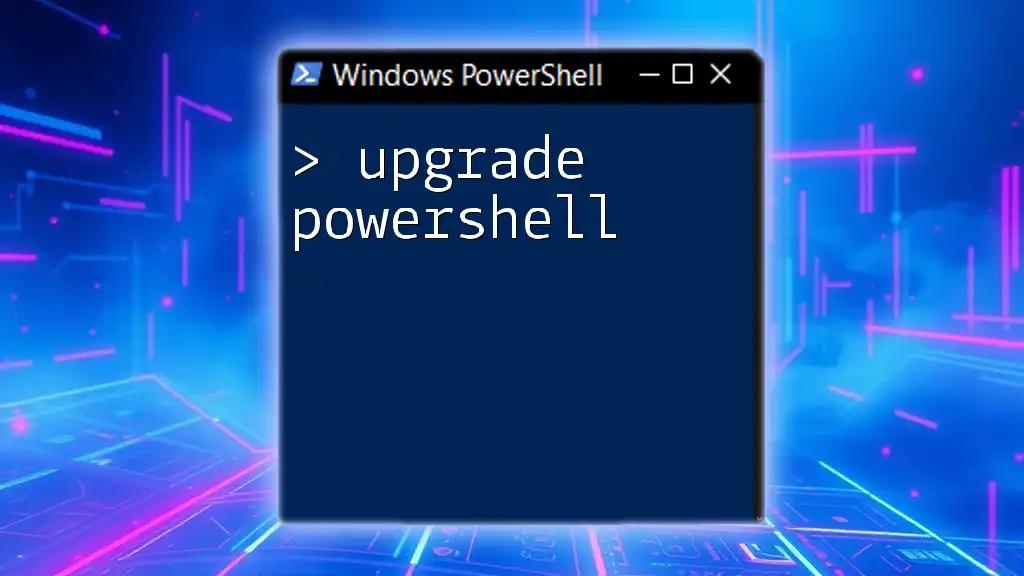
Advanced Topics in PowerShell Return Codes
Using return codes becomes even more interesting when working with functions and scripts that include specific return statements.
You can define custom exit codes within a function like this:
function Test-ReturnCode {
param (
[switch]$Success
)
if ($Success) {
return 0 # Custom success code
} else {
return 1 # Custom error code
}
}
In this example, the function `Test-ReturnCode` takes a parameter that determines whether to return a success or error code. Such custom codes enhance clarity in larger scripts, making it easier to debug and understand outcomes.
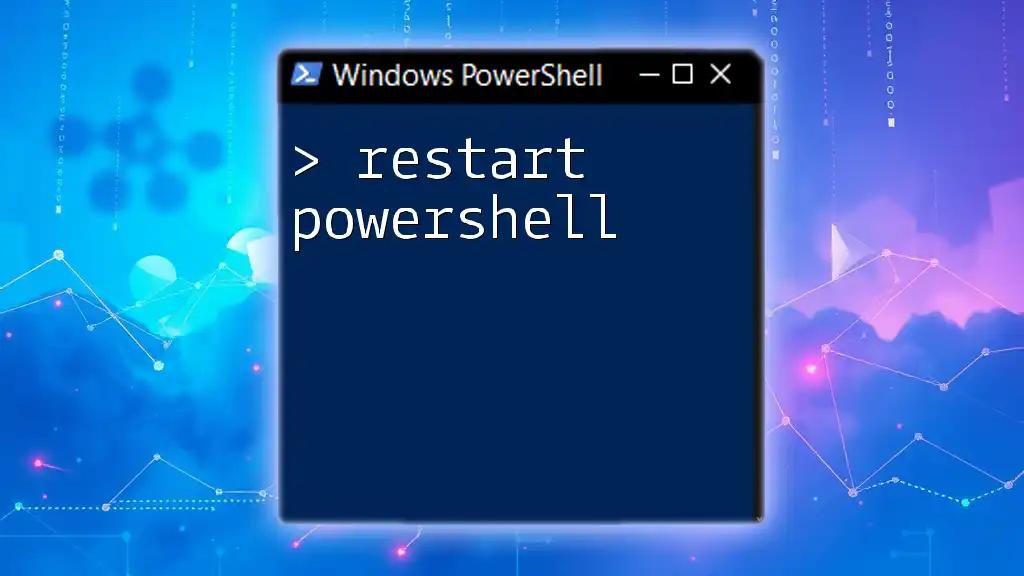
Debugging Using Return Codes
Return codes are invaluable for debugging PowerShell scripts. By monitoring the status of commands, you can more easily pinpoint issues and identify solutions.
Common pitfalls include neglecting to check return codes or misunderstanding their meanings. Always ensure that you consistently check for return codes in your scripts, as this simplifies the debugging process.
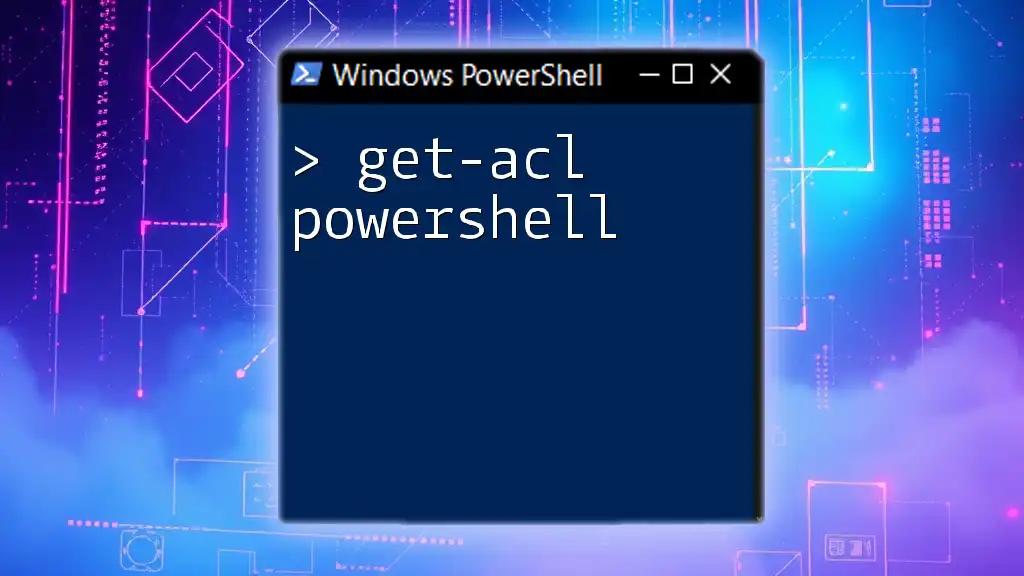
Conclusion
Understanding the return code PowerShell is fundamental for effective script writing. Not only does it facilitate error detection and handling, but it also enhances the overall robustness of your PowerShell applications. By leveraging return codes correctly, you ensure smoother operations and contribute to the integrity of your automated processes.

Call to Action
Stay tuned for more insightful PowerShell tips and tutorials! Subscribe to our newsletter for updates on the latest scripting techniques and best practices.