To delete a user from Active Directory using PowerShell, you can use the `Remove-ADUser` cmdlet followed by the username or user object.
Remove-ADUser -Identity "username"
Make sure to replace `"username"` with the actual username of the user you wish to delete.
Understanding Active Directory User Accounts
What is an Active Directory User Account?
An Active Directory (AD) user account is a digital identity that enables users to access network resources. Each user account consists of various components, including the username, password, and group memberships. These accounts are critical for maintaining security, ensuring users have the appropriate permissions based on their roles within an organization.
Why You May Need to Delete a User Account
User accounts may need to be deleted for several reasons, such as when an employee leaves the company, a temporary account is no longer needed, or when accounts become outdated. Before committing to the deletion of a user account, it is essential to consider the implications, such as group memberships or shared resources, to avoid disrupting business operations.
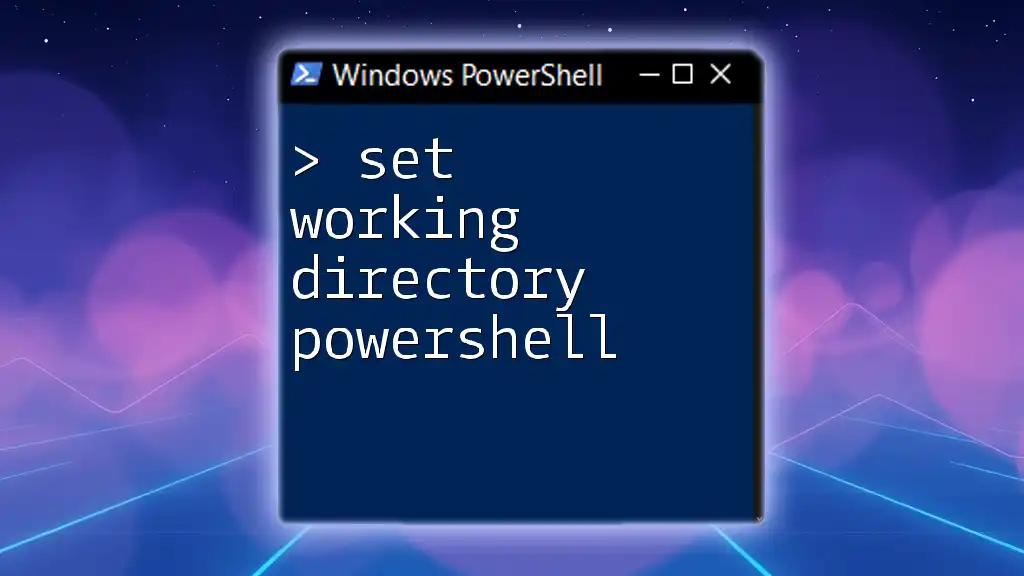
Preparing Your Environment
Prerequisites for Using PowerShell with Active Directory
Before proceeding with the deletion of user accounts using PowerShell, ensure that the Active Directory module is installed and that you have the necessary permissions to delete user accounts in your organization. Admin-level access is typically required.
Installing the Active Directory Module
To install the Active Directory module, you can use the following steps:
- Open PowerShell as an administrator.
- Run the following command to install the module:
Install-WindowsFeature RSAT-AD-PowerShell
- To verify the installation, check if the cmdlet is available by running:
Get-Module -ListAvailable
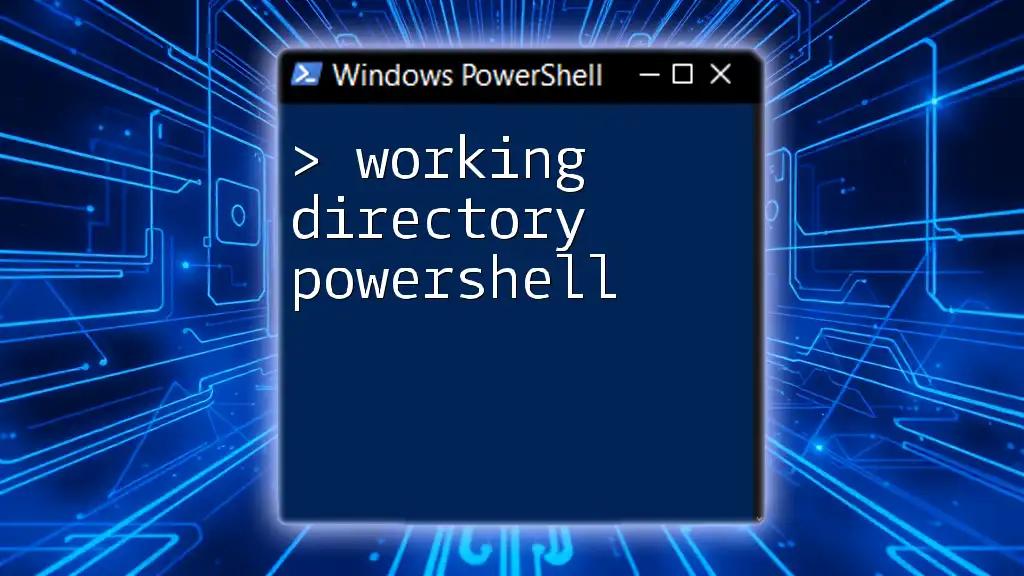
Basic PowerShell Commands for User Management
Introduction to PowerShell Cmdlets
Cmdlets are lightweight commands used in the PowerShell environment, often consisting of a verb-noun structure. The Active Directory module includes various cmdlets specifically designed for user management tasks, making it easier to manage user accounts programmatically.
Listing User Accounts
To view existing user accounts in Active Directory, employ the following command:
Get-ADUser -Filter *
This command retrieves all user accounts, allowing you to inspect them before making any deletions. It’s essential to know which accounts are active to avoid accidental deletion of necessary users.
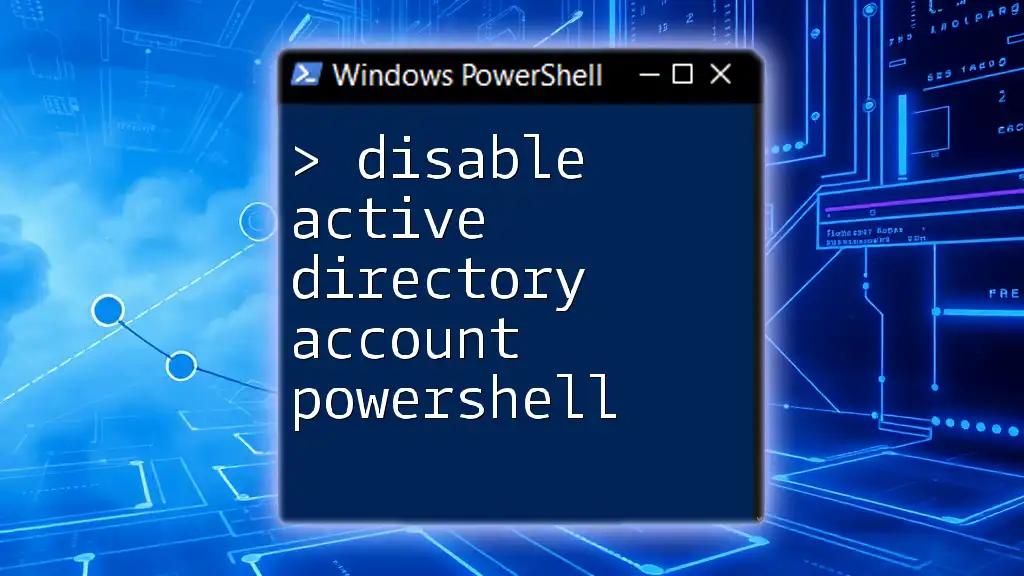
Deleting a User Account in Active Directory
Step-by-step Guide to Deleting a User
To delete a user account, you can utilize the Remove-ADUser cmdlet. The basic syntax for deleting a user account is as follows:
Remove-ADUser -Identity "username"
Replace `"username"` with the actual username of the account you wish to delete. You can also enhance the command with additional options, like:
- -Confirm: Prompts for confirmation before executing the deletion.
- -PassThru: Displays the object that was deleted.
Handling Common Issues
When attempting to delete a user account, you may encounter various errors. Some common issues include:
- User does not exist: Double-check the username for typos.
- Permission denied: Ensure you have the required permissions to perform deletions.
To troubleshoot, utilize PowerShell's error messages to identify the root of the problem.
Verifying Deletion Success
After issuing the deletion command, it is prudent to confirm that the account has been successfully removed from Active Directory. Execute:
Get-ADUser -Identity "username"
If the command returns an error indicating that the user does not exist, the deletion has been successful.
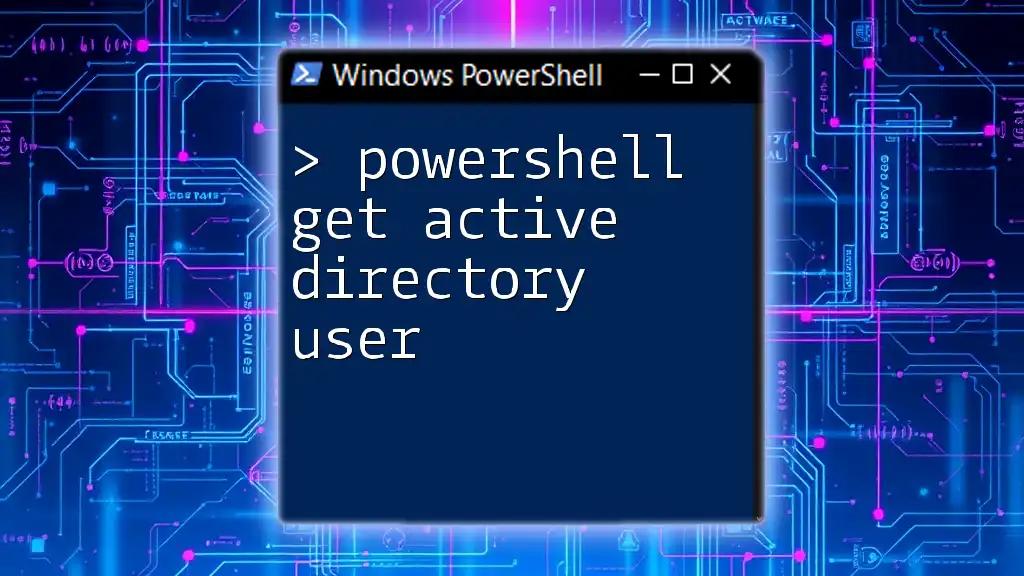
Advanced Techniques
Bulk Deletion of User Accounts
In scenarios where multiple accounts need to be deleted, consider using bulk deletion. First, prepare a CSV file containing the usernames of accounts to be deleted. The example below demonstrates how to perform bulk deletions:
Import-Csv "C:\path\to\users.csv" | ForEach-Object { Remove-ADUser -Identity $_.Username }
This approach is efficient and minimizes the time spent on repetitive tasks.
Adding Safety Measures
Before executing deletion commands, it’s wise to utilize the -WhatIf parameter. This parameter simulates the deletion process without actually removing any accounts:
Remove-ADUser -Identity "username" -WhatIf
This command allows you to verify the action's effects, ensuring you don't delete unintended accounts.
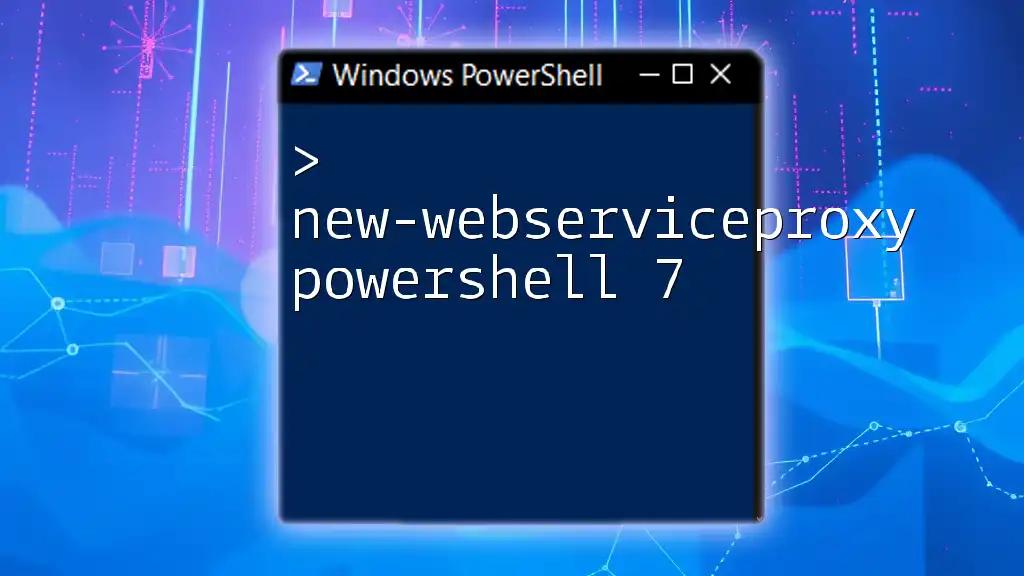
Best Practices for User Management in Active Directory
Ensuring Data Integrity
When managing user accounts, data integrity must be prioritized. Always maintain backups of user information to prevent accidental data loss. Performing regular audits can also help identify outdated or unnecessary accounts that may require deletion.
Documentation and Change Management
Establish a system for documenting changes made to user accounts. Keeping track of who deleted which accounts and why fosters accountability and transparency within your organization. Additionally, consider using a change management system to manage user account updates and deletions effectively.

Conclusion
In summary, utilizing PowerShell to delete user accounts in Active Directory offers a robust and efficient method for managing user access. Familiarizing yourself with the necessary commands and best practices ensures the process is both effective and safe. As you continue your PowerShell journey, don’t hesitate to practice and explore new commands within a secure environment to enhance your skills. Continuous learning in IT management is essential for adapting to ever-evolving technologies.

Additional Resources
For further learning, consider checking out the official Microsoft documentation on PowerShell and Active Directory, as well as engaging with online forums or communities for additional insights and support.