To disable a user account in PowerShell, you can use the `Disable-LocalUser` cmdlet followed by the username you wish to disable.
Disable-LocalUser -Name "username"
Understanding User Accounts in PowerShell
What is a User Account?
A user account acts as a digital identity for individuals accessing a computer system or network. It allows users to log in, access resources, and perform tasks based on assigned permissions. In Windows environments, user accounts come in different types, including local accounts, which are specific to a single machine, and domain accounts, which operate within a broader network environment.
The Role of PowerShell in User Management
PowerShell is an efficient and robust command-line shell and scripting language designed for automation and configuration management. Its capabilities allow system administrators to manage user accounts seamlessly, streamlining processes that can be time-consuming when performed manually. Using PowerShell to disable user accounts can significantly enhance your productivity, especially in larger environments where multiple accounts may need to be disabled simultaneously.
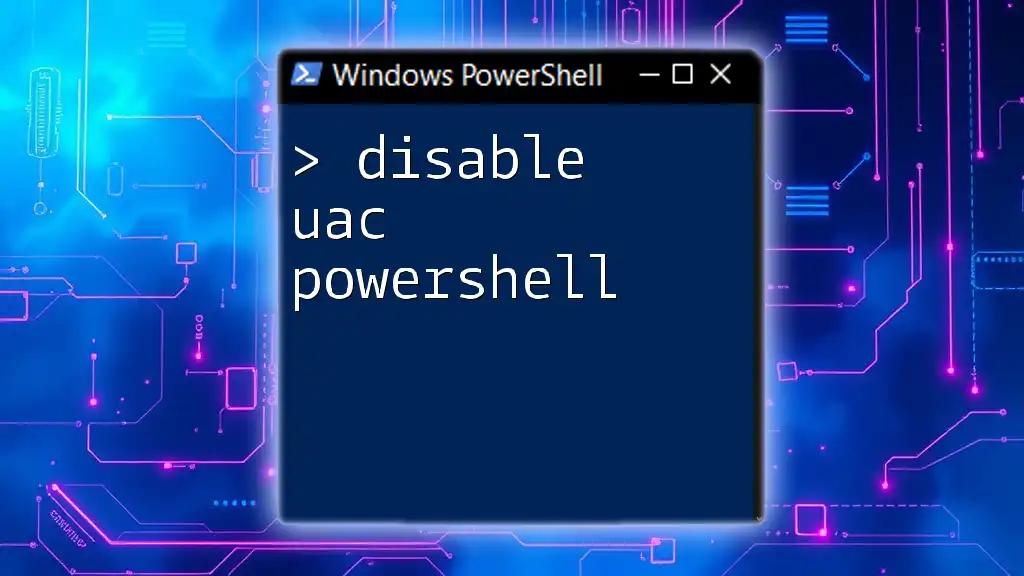
Preparing to Disable User Accounts
Prerequisites for Using PowerShell
Before you can disable a user account using PowerShell, ensure you have the appropriate permissions. You’ll need to be a member of a group that has the rights to modify user accounts, typically the Domain Admins group in a domain environment.
Make sure PowerShell is installed and available on your system. You can access PowerShell by typing "PowerShell" in the Start menu search bar.
Understanding the Get-ADUser cmdlet
The Get-ADUser cmdlet is essential for retrieving information about user accounts in Active Directory (AD). This cmdlet provides vital information about users, making it easier to identify which accounts need to be disabled.
To retrieve all user accounts, you can use the following command:
Get-ADUser -Filter *
This command lists all user accounts in your Active Directory environment, giving an overview of available accounts.
Identifying User Accounts to Disable
To efficiently disable user accounts, it's crucial to identify which accounts are inactive or no longer require access. Implement filters in PowerShell to refine your search. For example, to view all currently enabled user accounts, use:
Get-ADUser -Filter {Enabled -eq $true} | Select-Object Name, SamAccountName
This command filters out enabled accounts, helping you make informed decisions about which accounts to disable.
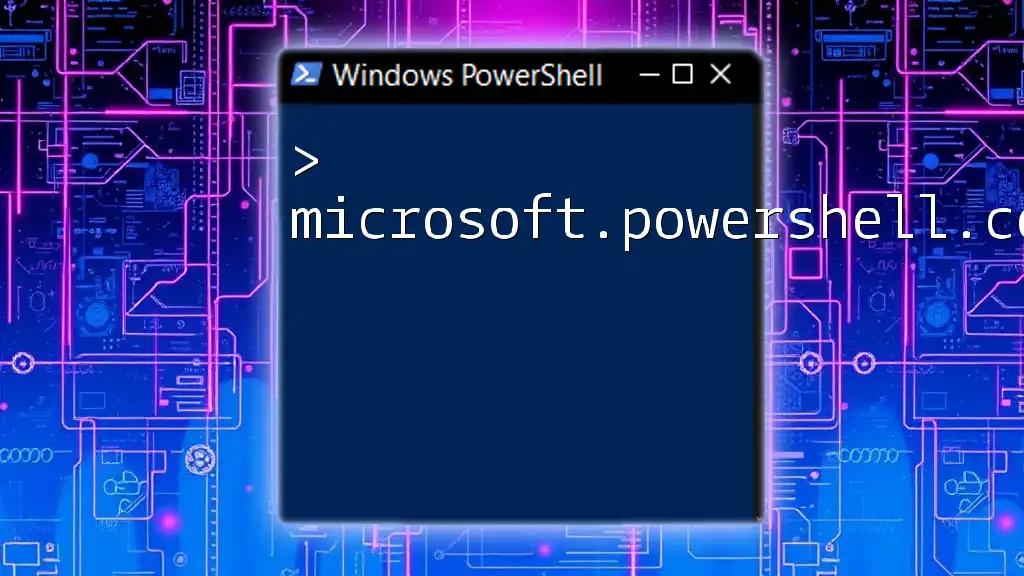
Disabling a User Account with PowerShell
Basic Command to Disable a User Account
To disable a user account, you can use the Disable-ADAccount cmdlet. The basic structure for this command is straightforward:
Disable-ADAccount -Identity 'username'
Replace `'username'` with the actual username of the account you wish to disable. Executing this command immediately disables the specified user account, preventing any further logins.
Disabling Multiple User Accounts
In scenarios where you need to disable several accounts at once, you can utilize a loop. Here’s an example that shows how to disable multiple user accounts efficiently:
$users = @('User1', 'User2', 'User3')
foreach ($user in $users) {
Disable-ADAccount -Identity $user
}
This script creates an array with the usernames of accounts to disable. The `foreach` loop iterates through each name, applying the Disable-ADAccount cmdlet to each one.
Confirmation of Disabled Accounts
After executing the disable command, it's vital to confirm that the accounts are indeed disabled. You can check the status of a user account with this command:
Get-ADUser -Identity 'username' | Select-Object Name, Enabled
This will display the name and enabled status of the account, ensuring that it has been successfully disabled.
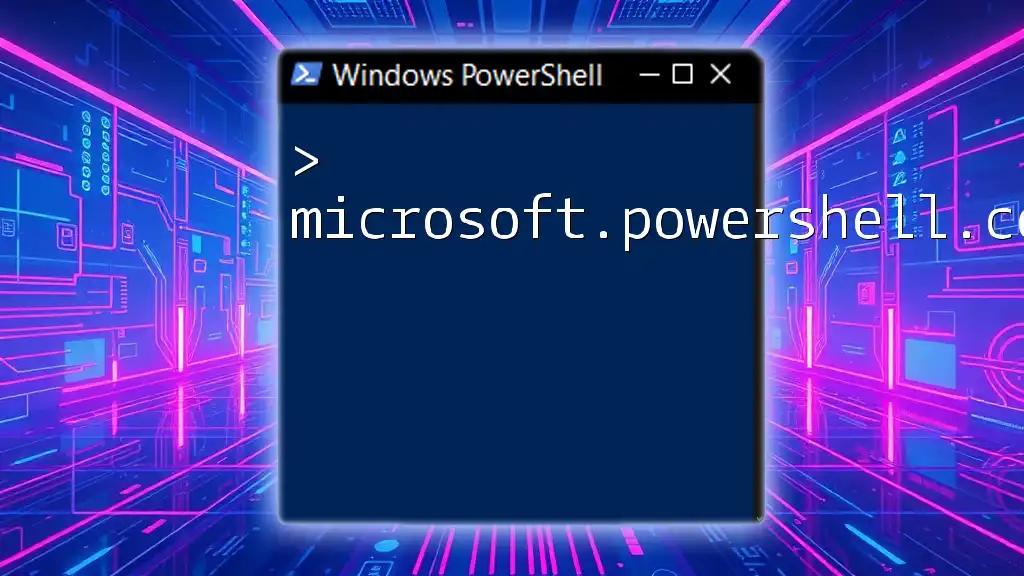
Error Handling and Troubleshooting
Common Errors When Disabling Accounts
While disabling user accounts with PowerShell is generally straightforward, you may encounter some common errors. For instance, you might experience permissions issues or errors due to non-existent accounts. These errors can disrupt your ability to manage user accounts effectively.
Best Practices for Managing Errors
Implementing error handling in your PowerShell scripts can save you time and frustration. Use Try-Catch blocks to catch and handle errors gracefully. Here’s an example of how to implement this:
Try {
Disable-ADAccount -Identity 'username'
} Catch {
Write-Host "Error occurred: $_"
}
This structure attempts to disable the user account, and if it fails, it captures the error and displays a message, allowing you to troubleshoot effectively.
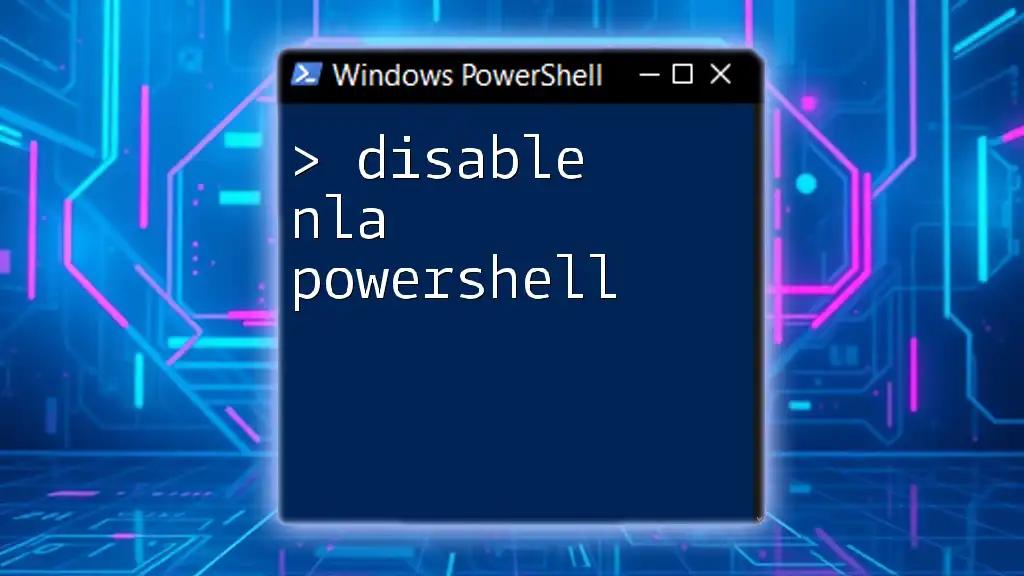
Alternative Methods to Disable User Accounts
Using Active Directory Users and Computers (ADUC)
While PowerShell is a powerful tool for disabling user accounts, the Active Directory Users and Computers (ADUC) management console offers a graphical interface. This method may be preferable for users who are less familiar with command-line tools or when managing a small number of accounts.
However, for larger organizations or tasks requiring automation, using PowerShell remains a more efficient choice.
Using PowerShell to Disable Accounts on Local Machine
If you're working with local user accounts (not domain accounts), you can use a different cmdlet, Set-LocalUser. To disable a local user account, use the following command:
Set-LocalUser -Name 'username' -Enabled $false
This command effectively disables the specified local account, enabling you to manage user accounts both on domain and local levels.
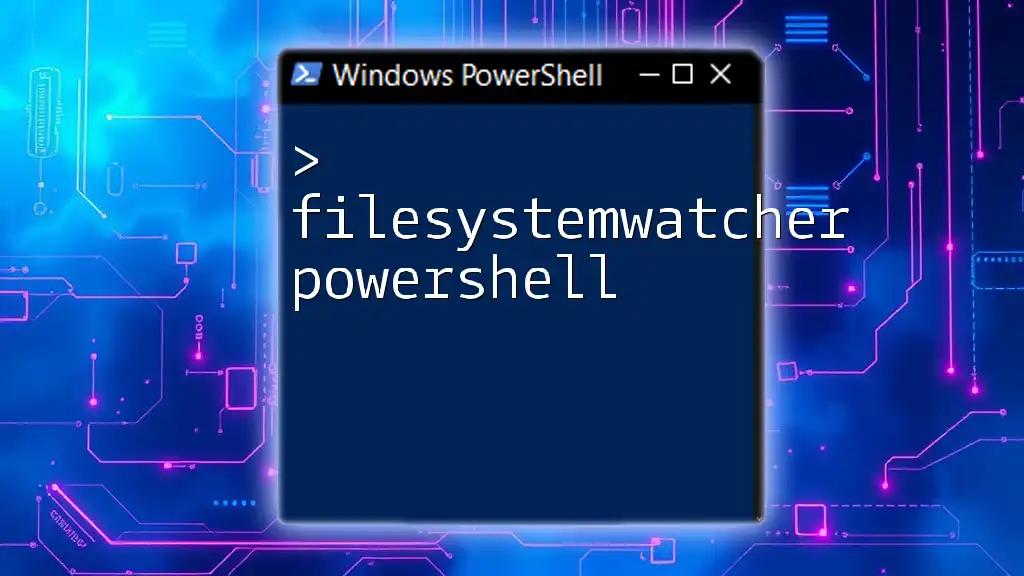
Conclusion
In this guide, we explored the various facets of using PowerShell to disable user accounts efficiently. By understanding the underlying principles, utilizing the right cmdlets, and implementing effective error handling, you can streamline your user management tasks significantly. As you practice these commands and techniques, you’ll find that PowerShell not only saves time but also enhances your capabilities as a system administrator.
For further learning, explore more PowerShell commands on our website and continue refining your skills in user management and automation.