To create a new directory in PowerShell, you can use the `New-Item` cmdlet followed by the desired path and type of item you want to create.
New-Item -Path 'C:\ExampleDirectory' -ItemType Directory
Understanding Directories
What is a Directory?
A directory is a structure within a file system that contains files and potentially other directories. It acts like a container, allowing users to organize files in a logical hierarchy. This organization is crucial for efficient file management, making it easier to locate and manage data.
Types of Directories
When using PowerShell, it's important to understand the different types of directories:
- Root Directories: This is the top-level directory in a file system. It serves as the starting point for navigating the file system structure.
- Subdirectories: These are directories created within other directories, enabling further categorization of files.
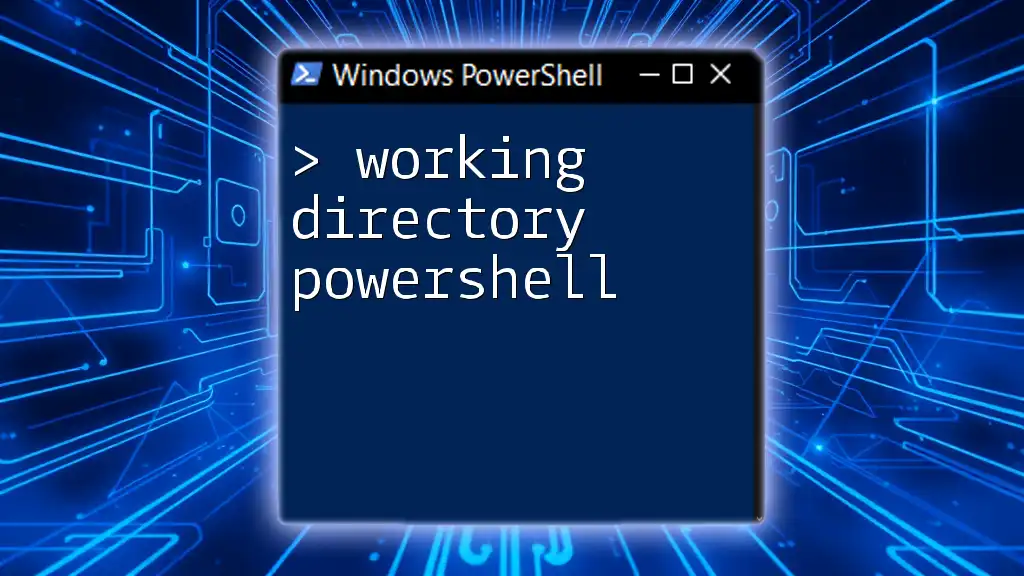
How to Create a Directory in PowerShell
Basic Command Structure
PowerShell commands are known as cmdlets and follow a specific structure. To create a directory, you can make use of the `New-Item` cmdlet. This cmdlet allows users to create various types of items, including directories.
Syntax of the New-Item Cmdlet
The general syntax for creating a directory using `New-Item` is as follows:
New-Item -Path "C:\Path\To\Directory" -ItemType Directory
Each parameter serves a distinct purpose in this command:
- -Path: This specifies the location where the new directory will be created.
- -ItemType: This indicates the type of item being created, which is a directory in this case.
Example of Creating a Directory
To illustrate creating a directory, you might use the following command in PowerShell:
New-Item -Path "C:\Users\YourUserName\Documents\MyNewDirectory" -ItemType Directory
When executed, this command instructs PowerShell to create a new directory named `MyNewDirectory` within the specified path. If successful, PowerShell will provide confirmation.
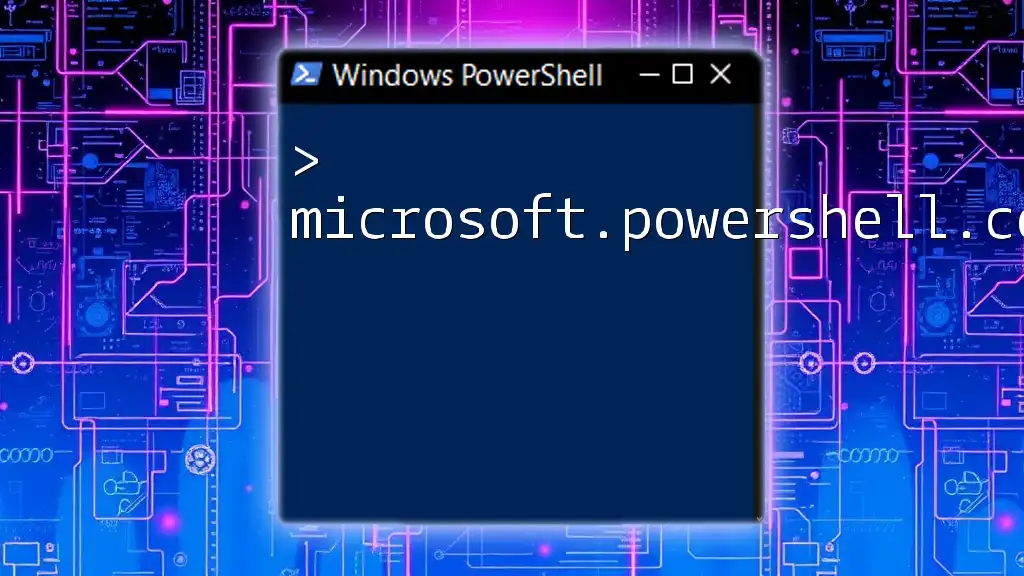
Creating Multiple Directories at Once
Using the mkdir Alias
For those familiar with Unix-like systems, mkdir is commonly used to create directories. In PowerShell, you can use the `mkdir` command as an alias for `New-Item`, allowing for a quick and informal way to create directories.
To create multiple directories simultaneously, you can do the following:
mkdir "C:\Users\YourUserName\Documents\Folder1","C:\Users\YourUserName\Documents\Folder2"
This command allows you to create `Folder1` and `Folder2` at once, saving time if you need several directories.
Example of Nested Directories
If you wish to create a subdirectory within an existing directory, you can do so easily with PowerShell:
New-Item -Path "C:\Users\YourUserName\Documents\ParentFolder\SubFolder1" -ItemType Directory
By executing this command, you create `SubFolder1` inside `ParentFolder`, showcasing how PowerShell can help maintain a clear and organized folder structure.
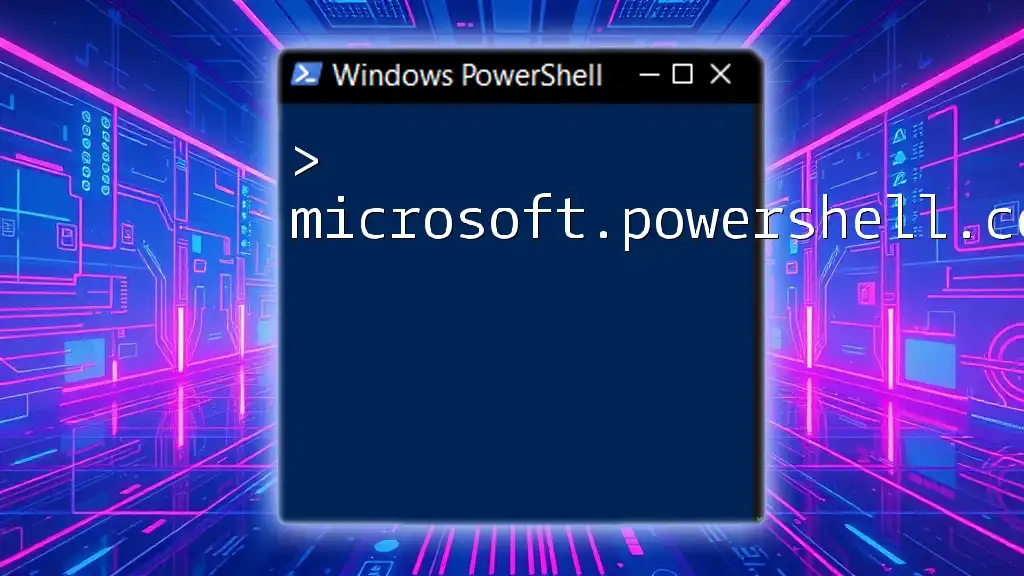
Working with Existing Directories
Checking If a Directory Exists
Before creating a directory, you may want to check if it already exists. This can be done through the `Test-Path` cmdlet:
Test-Path "C:\Users\YourUserName\Documents\MyNewDirectory"
This command returns `True` if the directory exists and `False` if it does not. Such checks are essential to prevent errors and maintain efficient workflows.
Creating a Directory Only If It Doesn't Exist
To ensure that a directory is created only when it does not already exist, you can use an `if` statement combined with `Test-Path`:
if (-Not (Test-Path "C:\Users\YourUserName\Documents\MyNewDirectory")) {
New-Item -Path "C:\Users\YourUserName\Documents\MyNewDirectory" -ItemType Directory
}
This script will check for the existence of `MyNewDirectory` and will create it only if it does not exist, thereby enhancing your script's robustness.
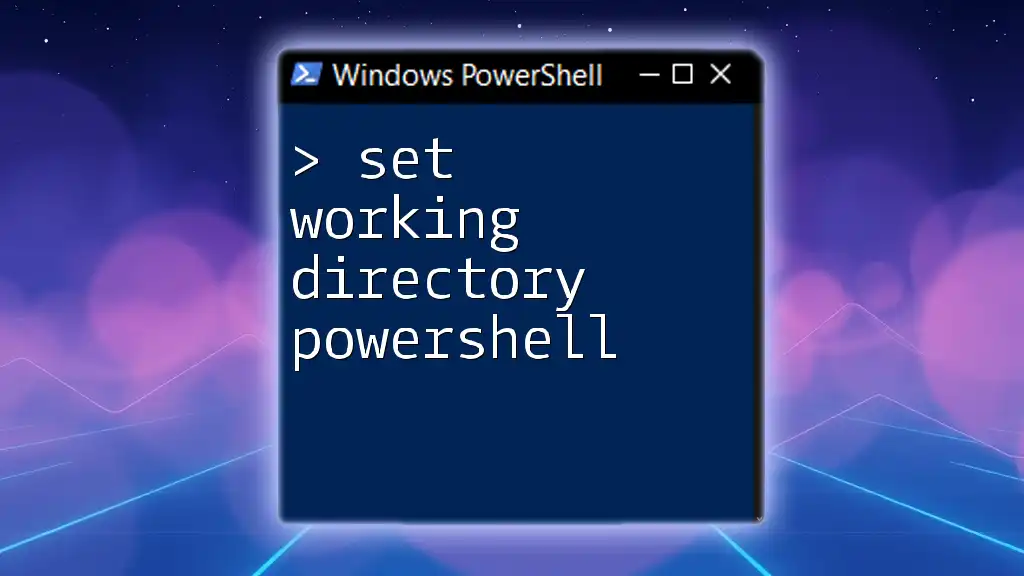
Additional Tips for Using PowerShell
Using PowerShell Scripts for Repetitive Tasks
Creating multiple directories or performing other repetitive tasks can benefit from automation. By creating a simple PowerShell script, you can automate the directory creation process, saving time and reducing manual oversight.
Leveraging Variables
Using variables can simplify your commands and enhance readability. For example:
$directoryPath = "C:\Users\YourUserName\Documents\NewFolder"
New-Item -Path $directoryPath -ItemType Directory
This approach not only makes your command easier to read but also allows for easier adjustments in case the path needs to change. Define the path once, and use the variable wherever necessary.
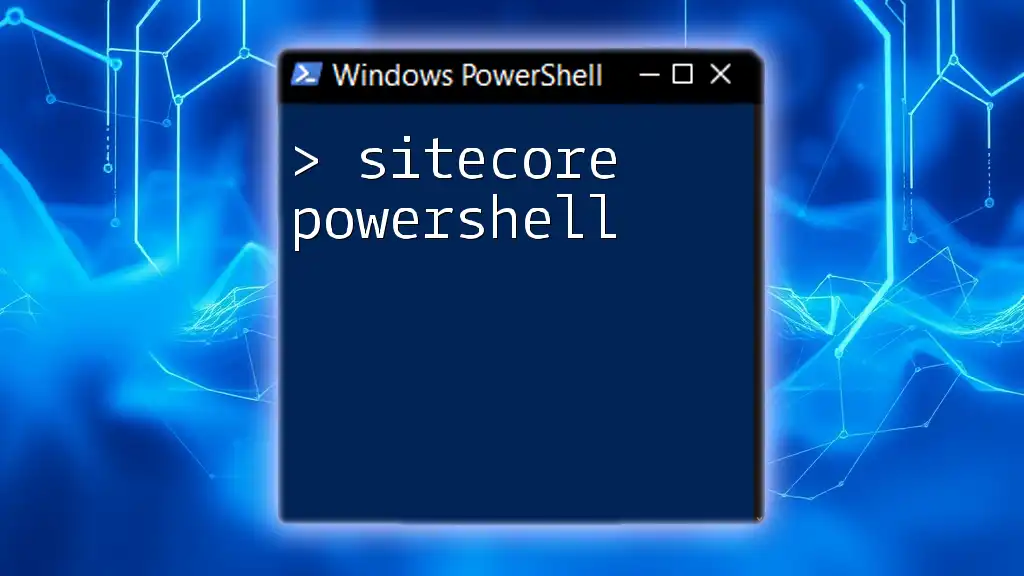
Conclusion
In this guide, we explored various ways to make a directory in PowerShell. We discussed the essential `New-Item` cmdlet, as well as shortcuts like `mkdir`, and demonstrated how to create both single and multiple directories. Additionally, we highlighted checking for existing directories and automating repetitive tasks with scripts and variables.
By mastering these techniques, you can efficiently manage your file structure using PowerShell, enhancing your productivity and organization. Happy scripting!
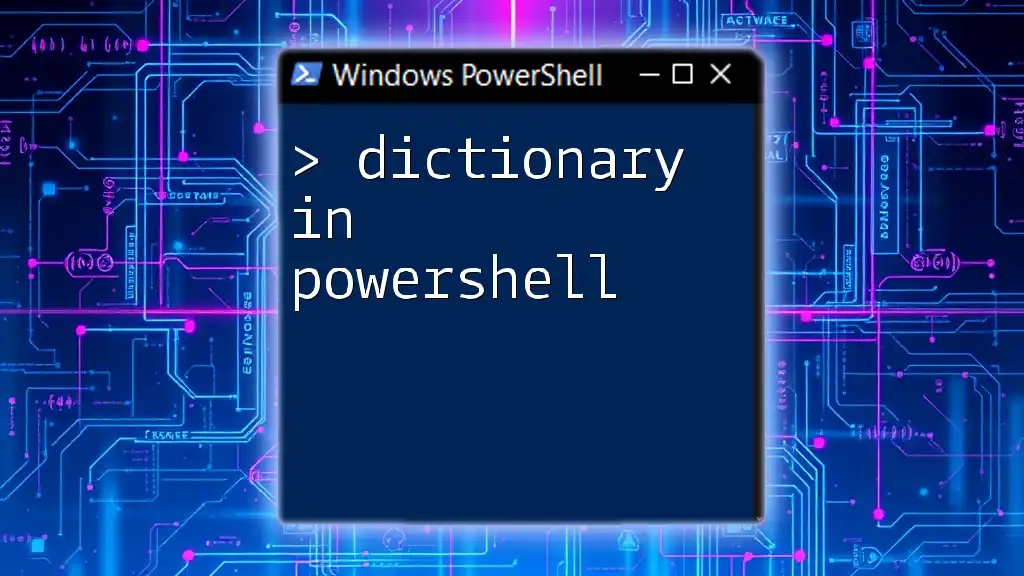
Additional Resources
For further reading and to expand your PowerShell knowledge, consider checking the official PowerShell documentation as well as various tutorials and community forums. Engaging with tutorials will help solidify your understanding while providing real-world context for commands and scripts.