The `-not` operator in PowerShell is used to negate a condition, returning true if the condition is false, and can be utilized in conditional statements to simplify logic.
Here's a code snippet demonstrating its use:
$condition = $false
if (-not $condition) {
Write-Host 'The condition is false.'
}
Overview of PowerShell Operators
PowerShell operators serve as the backbone of scripting and automation tasks within the framework. These operators manipulate data, control flow, and provide conditional evaluations.
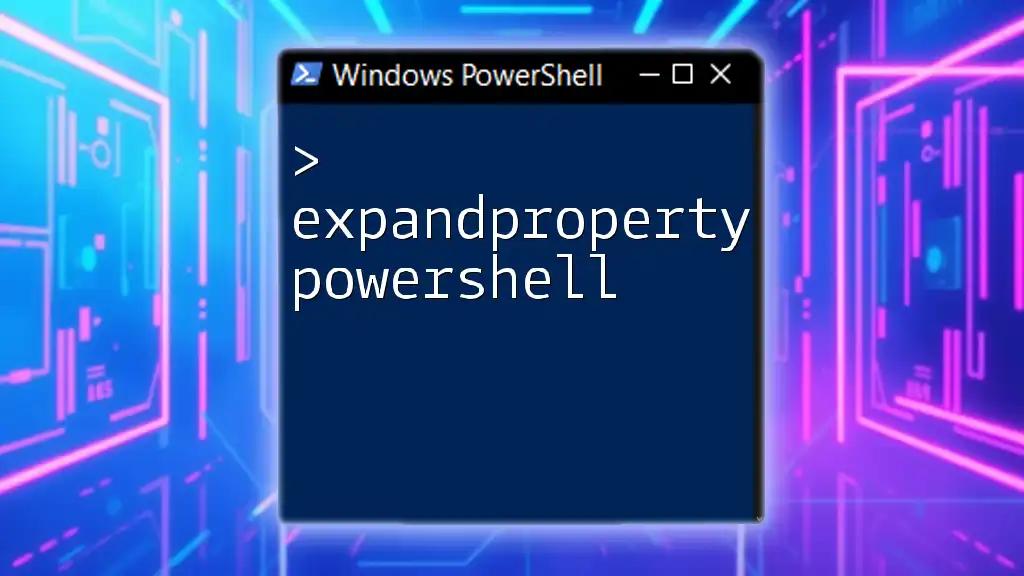
What is the Not Operator?
The Not operator is a logical operator in PowerShell that negates the value of a given expression. Essentially, it allows you to reverse the truth value of an expression, which is crucial in scenarios where you want to test conditions in the opposite manner. Understanding how the Not operator works can greatly enhance your PowerShell scripting capabilities.
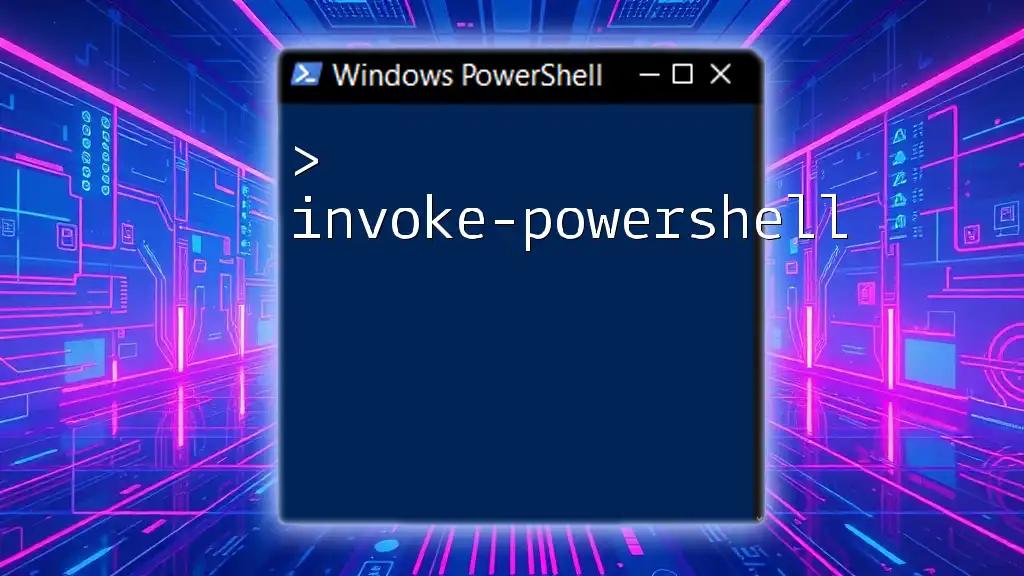
Understanding Logical Operators in PowerShell
PowerShell includes several logical operators that help in evaluating expressions. Here’s a brief overview of the key types:
- AND (`-and`): Returns true only if both expressions are true.
- OR (`-or`): Returns true if at least one of the expressions is true.
- Not (`-not`): Reverses the truth value of the expression.
Combining these operators effectively is fundamental to creating versatile scripts.
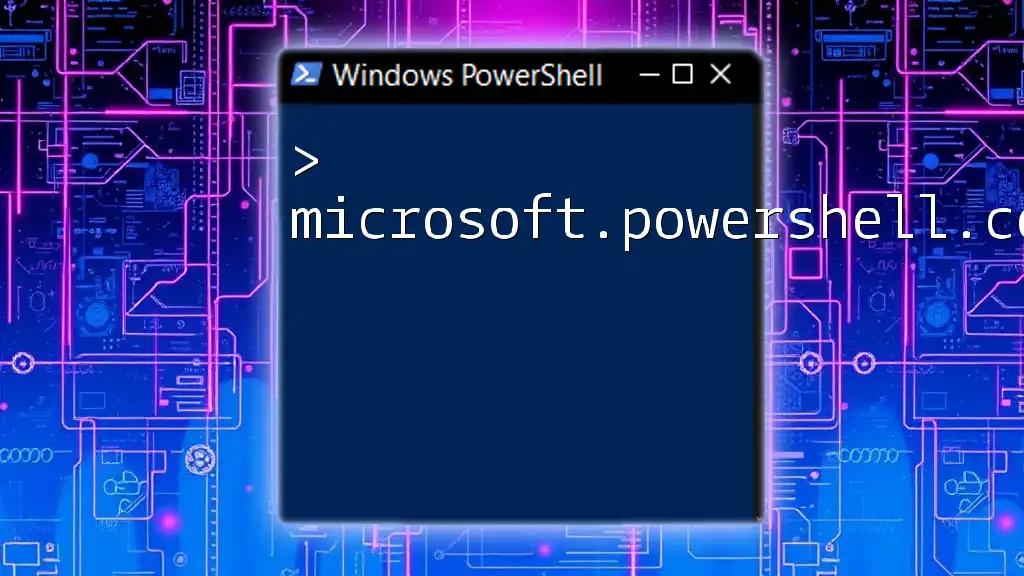
The Not Operator: Functionality and Usage
Syntax of the Not Operator
The syntax of the Not operator is straightforward:
not <expression>
Using this syntax, you can easily check conditions by expressing the opposite of what you would normally expect.
How the Not Operator Works
In PowerShell, the Not operator evaluates expressions to determine their truthiness. Here’s an essential concept: if an expression evaluates to $true, the Not operator will convert it to $false, and vice versa.
Practical Examples of the Not Operator
Example 1: Basic Usage
In this example, the Not operator checks if a variable is null:
$value = $null
if (-not $value) {
"Value is null or false"
}
Output: Value is null or false. This shows how the Not operator successfully detects that `$value` is indeed null.
Example 2: Negating Boolean Values
Here’s how iterating through logical values behaves with the Not operator:
$isTrue = $true
if (-not $isTrue) {
"This will not be displayed."
}
In this case, since `$isTrue` is true, the output will not display anything, demonstrating the negation at work.
Example 3: Using Not with Comparisons
You can apply the Not operator alongside comparison operators too:
if (-not (3 -eq 5)) {
"3 is not equal to 5"
}
The Not operator evaluates the comparison 3 -eq 5 and negates it, confirming the truth of the statement.
Combining the Not Operator with Other Logical Operators
Using Not with Or and And
The effectiveness of the Not operator shines when combined with Or and And:
$a = $false
$b = $true
if (-not ($a -or $b)) {
"Both are false"
}
Here, the Not operator combined with Or determines that both `$a` and `$b` are indeed not true at the same time to return false.
Complex Conditional Statements
You can use the Not operator in more elaborate logical constructs as well:
$userLoggedIn = $false
$isAdmin = $true
if (-not $userLoggedIn -and -not $isAdmin) {
"User is not logged in and not an admin"
}
This checks two conditions and ensures that both are false before executing the statement, showcasing the Not operator’s flexibility.
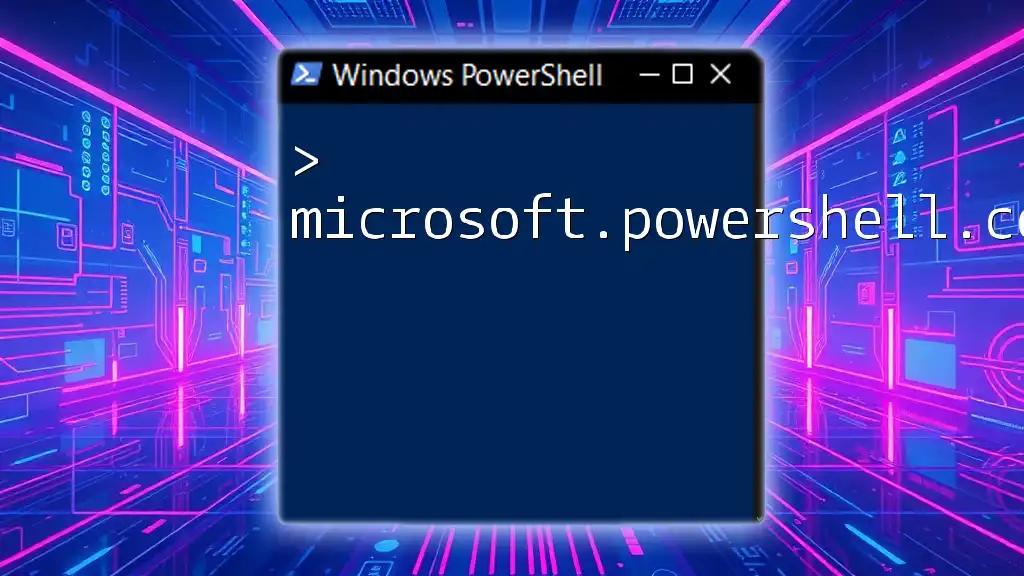
Common Use Cases for the Not Operator in PowerShell
Filtering Data with the Not Operator
One convenient application of the Not operator is in data filtering, especially with commands such as `Where-Object`:
$numbers = 1..10
$filteredNumbers = $numbers | Where-Object { -not ($_ -gt 5) }
This script filters out values greater than 5. The Not operator effectively allows you to reverse the logic and target the values you wish to include.
Error Handling with Not Operator
You can implement error handling scenarios utilizing the Not operator. Here’s a demonstration of a potential failure:
try {
# Assuming this command fails
Get-Item "C:\NonExistentFile.txt"
} catch {
if (-not $_.Exception.Message) {
"No error found."
} else {
$_.Exception.Message
}
}
In this case, the Not operator helps check if an exception message exists, ensuring appropriate error handling.

Best Practices for Utilizing the Not Operator in PowerShell
Readability and Code Maintenance
When writing scripts, clarity is vital. It is crucial to structure your code logically for ease of future reading and maintenance. Always aim for straightforward conditions when using the Not operator to prevent confusion.
Testing and Debugging Logic
Testing your logic before deployment is essential for avoiding errors. Utilize PowerShell's debugging features to test conditional expressions to ensure that your use of the Not operator yields the expected results.
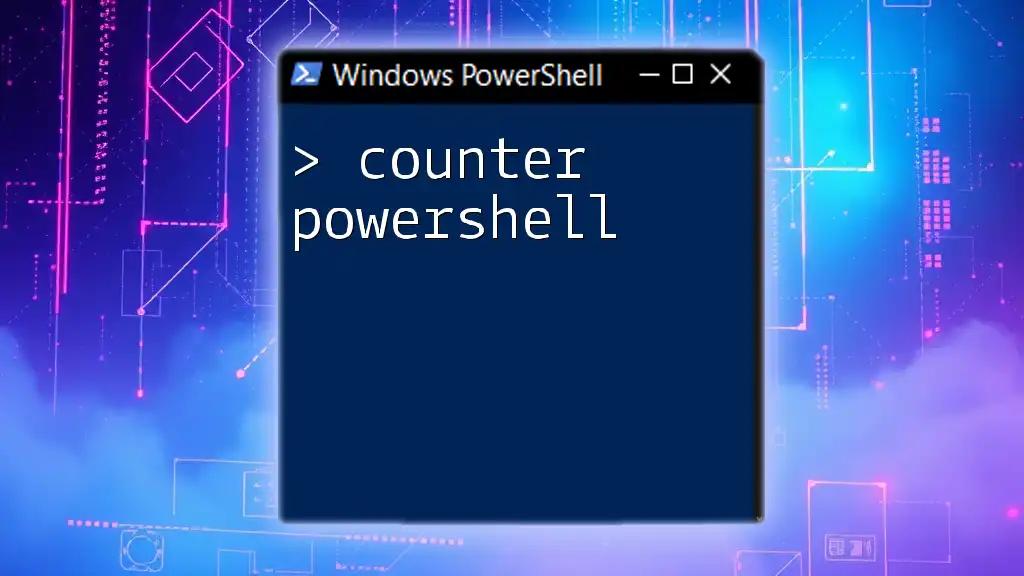
Conclusion
The Not operator in PowerShell is a powerful tool for reversing logical conditions. By mastering its usage, you can write more flexible and dynamic scripts that respond intuitively to varying input conditions. As you delve deeper into PowerShell, continue to explore the Not operator's potential and its application across different scenarios.
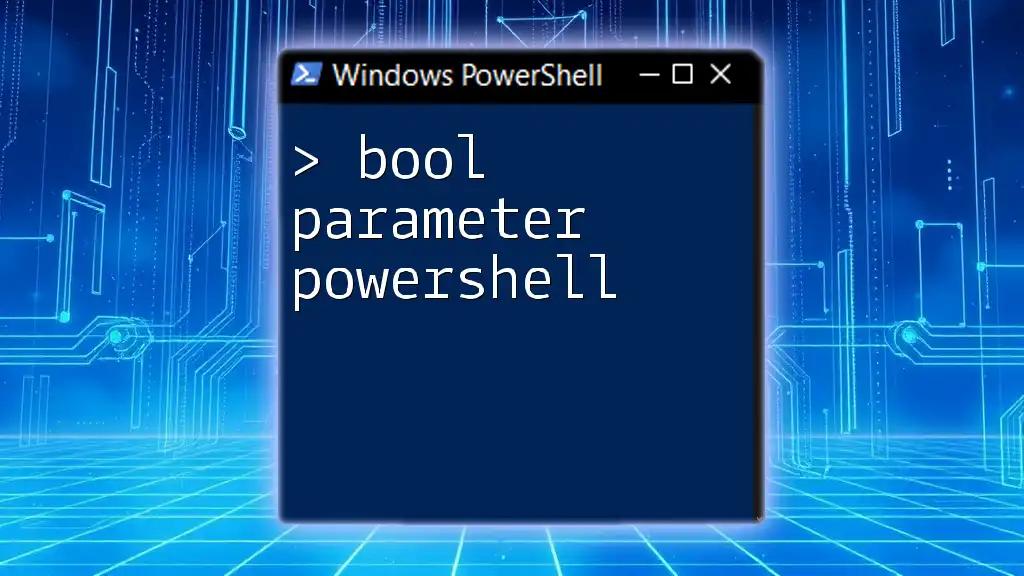
Additional Resources
For further learning, refer to the official PowerShell documentation, which provides comprehensive guidance. Additionally, community forums such as PowerShell.org offer valuable discussions and problem-solving opportunities among enthusiasts. Immerse yourself in both documentation and community to strengthen your understanding and command of the Not operator in PowerShell.