The `LastLogonTimestamp` attribute in PowerShell retrieves the last time a user logged onto a domain, which can be useful for auditing and monitoring user accounts.
Here’s a simple command to retrieve the `LastLogonTimestamp` for a specific user:
Get-ADUser username -Properties LastLogonTimestamp | Select-Object Name, LastLogonTimestamp
Replace `username` with the actual username you want to query.
Understanding lastLogontimestamp
lastLogontimestamp is an attribute in Active Directory that provides the last time a user successfully logged onto a domain. This value is crucial for administrators in tracking user activity, monitoring security, and maintaining compliance with organizational policies. Unlike other logon timestamp attributes, the lastLogontimestamp does not update every time the user logs in; instead, it updates only when the user logs in after more than 14 days, which reduces the replication traffic in a multi-domain controller environment.
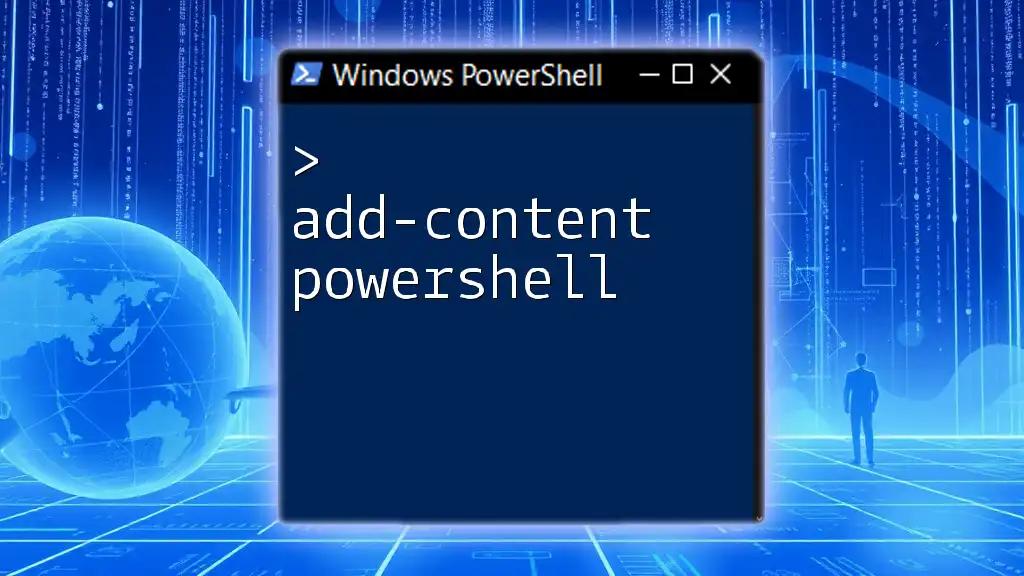
Understanding Active Directory Timestamps
What are Active Directory Timestamps?
Active Directory (AD) makes use of several timestamp attributes to track user logon activities, manage permissions, and facilitate auditing efforts. The main timestamp attributes include:
- lastLogon: This attribute records the last logon time for a user but does not replicate between domain controllers, making it accurate only for the specific DC where the logon occurred.
- lastLogonTimestamp: This attribute is replicated across domain controllers, primarily used for reporting purposes, and it provides a generalized view of user activity.
- lastLogoff: This attribute stores the last time the user logged off from the domain but is less commonly used in practical scenarios.
Different Logon Timestamps in Active Directory
Understanding the difference between these timestamps is vital for system administrators as it impacts how they're used for reporting and monitoring user activity. The lastLogon attribute can provide precise, but unreplicated, information, while lastLogonTimestamp offers a broader view due to its replication features. This replication is key in environments where multiple domain controllers are in use, ensuring that login information is consistent across the network.
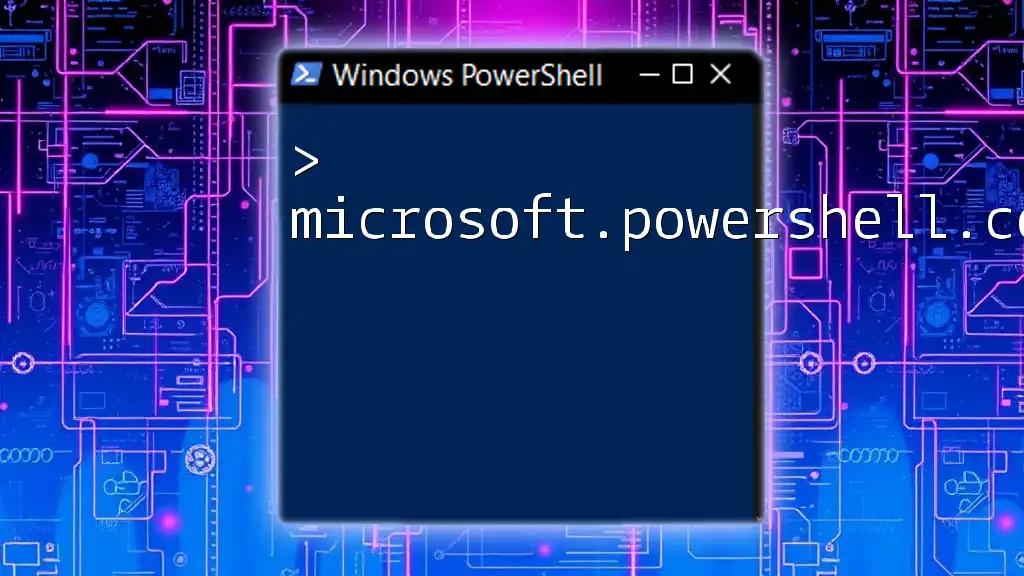
Retrieving lastLogontimestamp Using PowerShell
Initial Requirements
Before interacting with the lastLogontimestamp using PowerShell, you'll need to ensure you have the appropriate permissions. Access to Active Directory data typically requires at least read permissions on the user objects. Additionally, the Active Directory module must be installed in your PowerShell environment, which is generally available in Windows Server environments or can be installed via Remote Server Administration Tools (RSAT) on Windows client systems.
Basic Command to Retrieve lastLogontimestamp
To query the last logon timestamp for a specific user, you can use the following command:
Get-ADUser -Identity username -Properties lastLogonTimestamp
In this command, replace `username` with the actual username of the account you want to investigate. This straightforward query will return the user object and all its properties, including lastLogonTimestamp.
Example: Fetching User's lastLogontimestamp
To convert the timestamp to a human-readable format, you can extend the command as follows:
$user = Get-ADUser -Identity 'jdoe' -Properties lastLogonTimestamp
[DateTime]::FromFileTime($user.lastLogonTimestamp)
In this example:
- The command retrieves the user object for `jdoe`.
- The last logon timestamp is converted from a file time format into a standard DateTime format, making it easier to read and interpret.
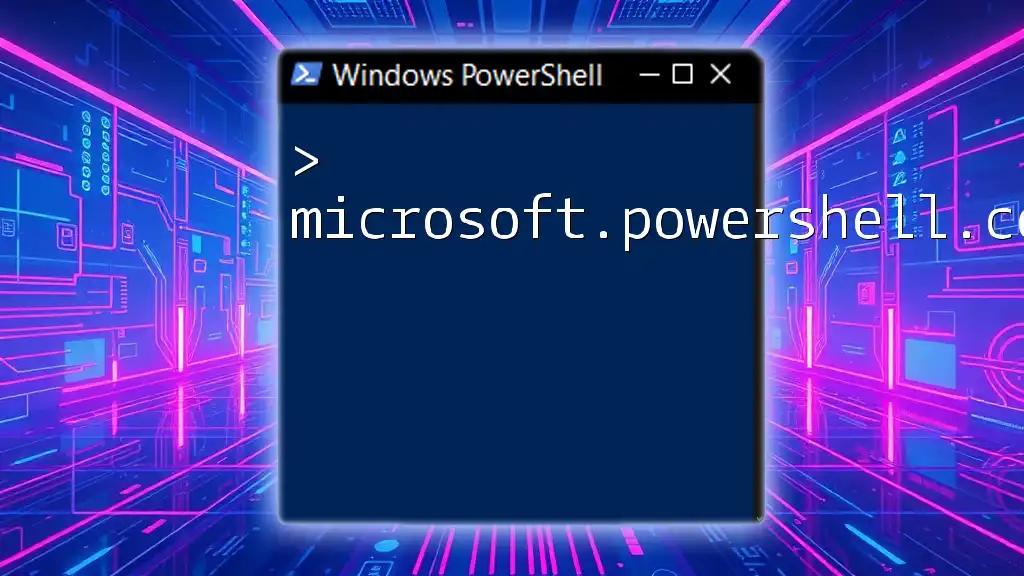
Interpreting the lastLogontimestamp Value
Understanding the Output Format
The lastLogonTimestamp is stored in a format that may not be immediately understandable. It stores the value as a Windows file time, which counts the number of 100-nanosecond intervals since January 1, 1601. This means that simply outputting the lastLogonTimestamp value will provide a numeric response.
Using FromFileTime() Method
To convert the numeric representation into a DateTime object, you leverage the .NET FromFileTime() method, like so:
$timestamp = [DateTime]::FromFileTime($user.lastLogonTimestamp)
This PowerShell one-liner will allow you to see the last logon time in a human-readable format, further contributing to your understanding of user activity.
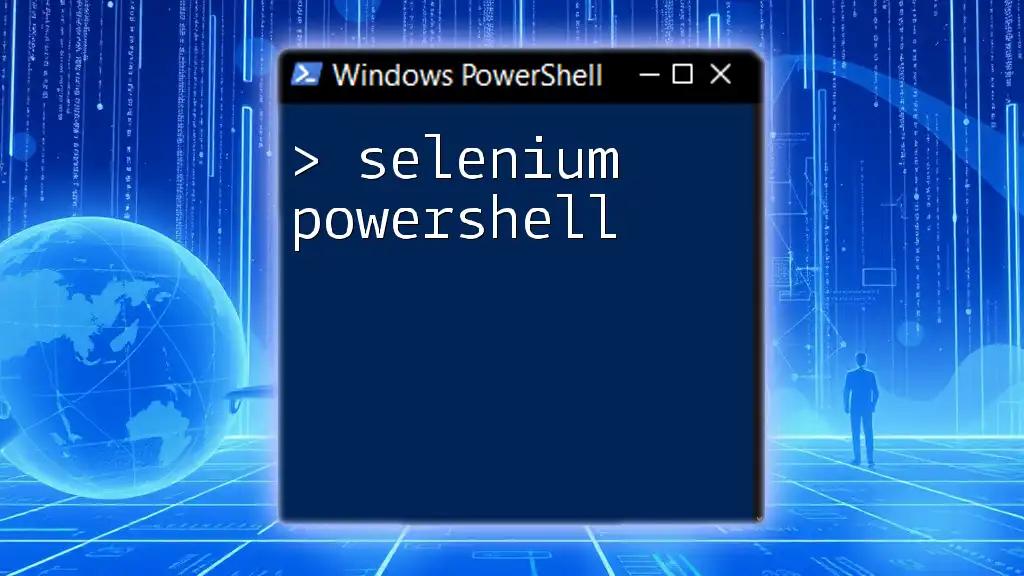
Filtering and Sorting User Logon Information
Searching for Users with a Specific lastLogontimestamp
You may want to find users who have logged on within a specific timeframe. Here is how you can filter users based on their lastLogonTimestamp:
Get-ADUser -Filter {lastLogonTimestamp -gt (Get-Date).AddDays(-30)} -Properties lastLogonTimestamp
This command finds all users whose last logon timestamp is within the last 30 days, allowing you to identify active users effectively.
Sorting Users by lastLogontimestamp
Sorting user accounts based on their last logon timestamp can be useful for identifying inactive accounts or for reporting purposes. You can achieve this with the following command:
Get-ADUser -Filter * -Properties lastLogonTimestamp |
Sort-Object -Property lastLogonTimestamp -Descending
This command retrieves all user accounts, sorts them by the last logon timestamp in descending order (most recent logons first), and can provide an overview of user activity trends over time.
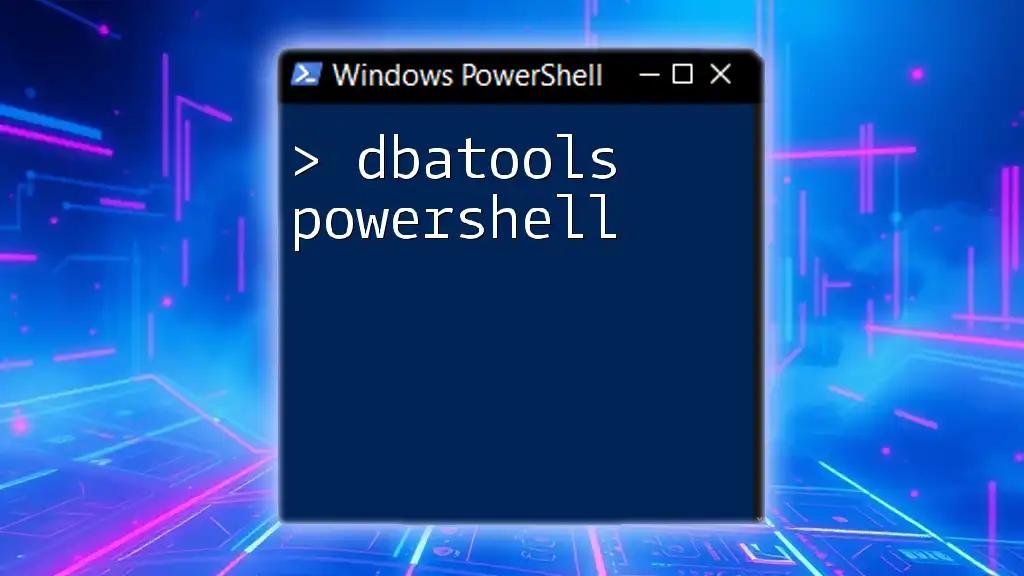
Automating Reports Using lastLogontimestamp
Creating a Custom Report Script
To streamline reporting, you can create a PowerShell script that automatically generates a report of user logon activities based on their lastLogonTimestamp. Here's how you might structure such a script:
$users = Get-ADUser -Filter * -Properties lastLogonTimestamp
$output = $users | ForEach-Object {
[PSCustomObject]@{
UserName = $_.SamAccountName
LastLogon = [DateTime]::FromFileTime($_.lastLogonTimestamp)
}
}
$output | Export-Csv -Path 'LastLogonReport.csv' -NoTypeInformation
In this script:
- All user accounts are fetched with the last logon timestamp.
- Each user's username and converted last logon timestamp are formatted into a custom object for easy readability.
- The output is then exported to a CSV file, allowing for convenient sharing and analysis.
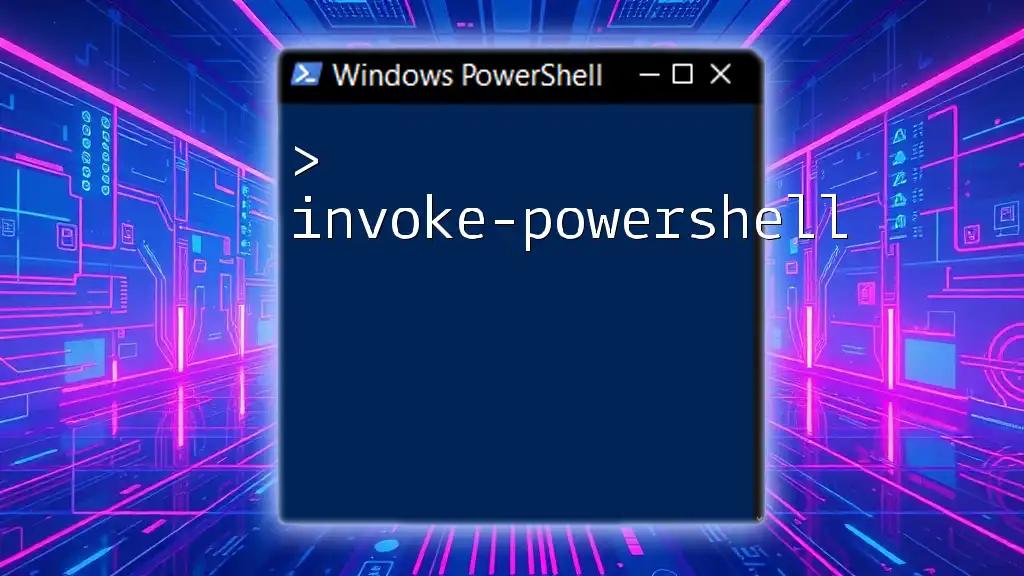
Common Pitfalls and Troubleshooting
Permissions Issues
Accessing user logon data can result in errors due to insufficient permissions. It's essential to ensure that your account has at least read access to user attributes within Active Directory. If you encounter errors, consult your system administrator for the necessary permissions.
Outdated or Inaccurate Data
While the lastLogonTimestamp offers a replicated view of user activity, factors such as user inactivity and replication delays can lead to outdated data. To maintain accuracy, ensure regular reviews and updates of user accounts and consider implementing policies to keep track of inactive users over prolonged periods.
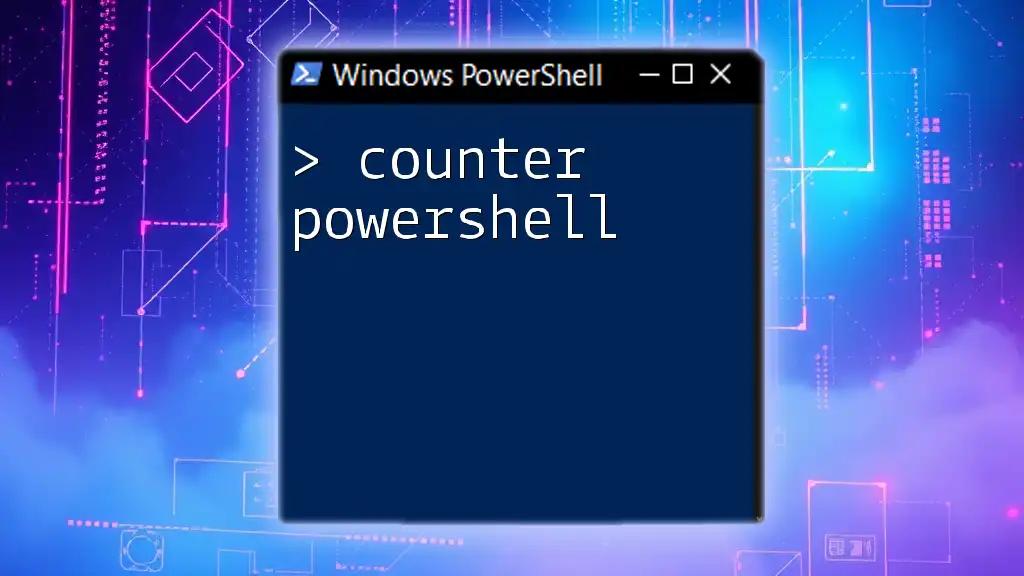
Conclusion
Understanding and utilizing the lastLogontimestamp attribute in PowerShell is vital for effective Active Directory management. By knowing how to retrieve, filter, and interpret logon data, administrators can maintain a healthy security posture, ensuring compliance and identifying potential risks within their organizations.
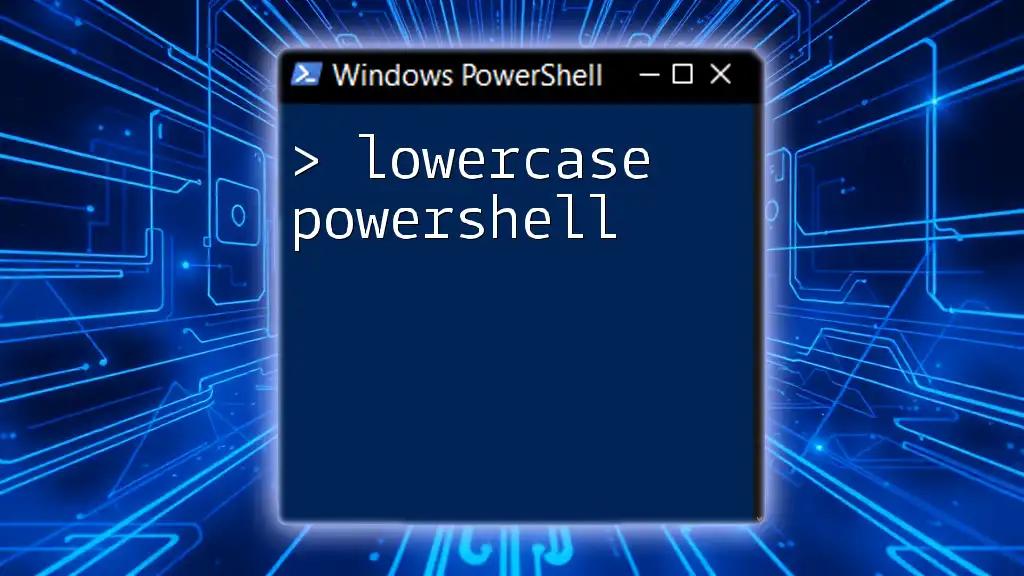
Call to Action
Practice executing these PowerShell commands related to lastLogontimestamp to enhance your skills. Follow us for more tips and tutorials on PowerShell usage, and stay ahead in your administrative journey!