In PowerShell, you can display a simple message to the user by using the `Write-Host` command, as demonstrated in the code snippet below:
Write-Host 'Hello, World!'
Understanding PowerShell Messaging
What is Messaging in PowerShell?
Messaging in PowerShell refers to the methods and commands used to communicate information to the user. Whether it's a simple notification to indicate that a script has completed successfully or an error alert to inform the user of a failure, messaging is crucial for enhancing script interaction and user experience. For example, displaying messages can guide users through automated processes, alert them to potential issues, or provide essential feedback.
Why Use Message Alerts?
Utilizing message alerts in PowerShell serves several significant purposes:
- Enhancing user experience: Notifications keep users informed, making scripts more user-friendly.
- Facilitating automation: When scripts run without direct oversight, messages help in knowing the progress and outcomes.
- Communication about issues: Messages can promptly inform users about errors or completion statuses, enhancing overall script effectiveness.
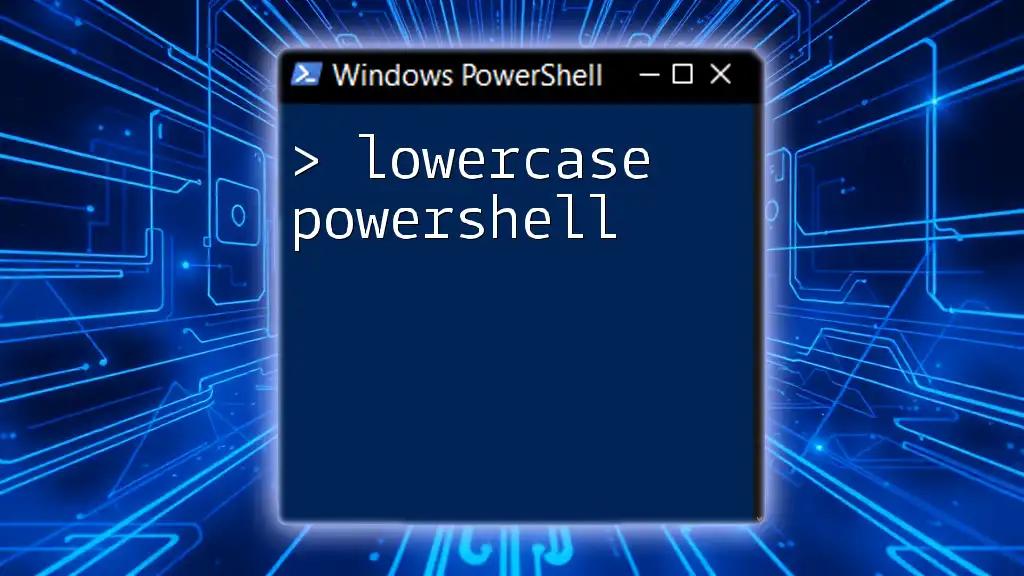
Different Methods to Show Messages in PowerShell
Using Write-Host
Definition and Usage
`Write-Host` is a command used to display output directly to the console. It's best suited for displaying information that does not need to be processed further in a pipeline. The syntax is straightforward:
Write-Host "This is a message displayed in the console"
This command can communicate simple messages effectively. However, it's essential to understand its limitations; `Write-Host` does not output data to the pipeline, which means that capturing the message for additional processing within the script is impossible.
Using Write-Output
Definition and Usage
`Write-Output` is an alternative to `Write-Host`. It sends the output to the pipeline, making it more versatile for use in scripts where further data manipulation might be needed. Here's how you can use it:
Write-Output "This is a message returned for further processing"
This command is particularly beneficial when passing information down the pipeline or when using it within functions. By using `Write-Output`, the message can be captured and processed as part of script logic.
Utilizing Message Box with Windows Forms
Creating a Simple Message Box
PowerShell can leverage Windows Forms to display message boxes, which provide a more interactive experience for users. To create a simple message box, you must first add the necessary assembly:
Add-Type -AssemblyName System.Windows.Forms
[System.Windows.Forms.MessageBox]::Show("Hello, this is a message box!")
This code snippet will display a standard message box with a basic message. Message boxes can also be customized to enhance user interaction.
Customizing Message Boxes
PowerShell allows you to modify your message boxes to include different buttons, icons, and titles. For instance, if you wish to provide a choice between "Yes" and "No", you can do so as follows:
[System.Windows.Forms.MessageBox]::Show("Choose an option", "My Message Box", [System.Windows.Forms.MessageBoxButtons]::YesNo, [System.Windows.Forms.MessageBoxIcon]::Warning)
This code will generate a message box with a title "My Message Box," showing a warning icon and including "Yes" and "No" buttons, allowing for a more engaging user experience.
Using Toast Notifications
What are Toast Notifications?
Toast notifications are brief messages that pop up in the corner of the user’s screen. These notifications are less intrusive than message boxes and can be customized for various tasks. They provide users with alerts while allowing scripts to continue running in the background.
Creating a Toast Notification in PowerShell
You can create toast notifications by utilizing external modules such as BurntToast. Here’s a simple example:
New-BurntToastNotification -Text "Hello from PowerShell", "This is a toast notification!"
This command will trigger a toast notification that informs users of an event or status update. Toasts can be enhanced with images and additional text for greater impact.
Customizing Toast Notifications
Adding elements like images or custom expiration times can improve the relevance of your notifications. The BurntToast module allows flexibility in crafting a fitting message.
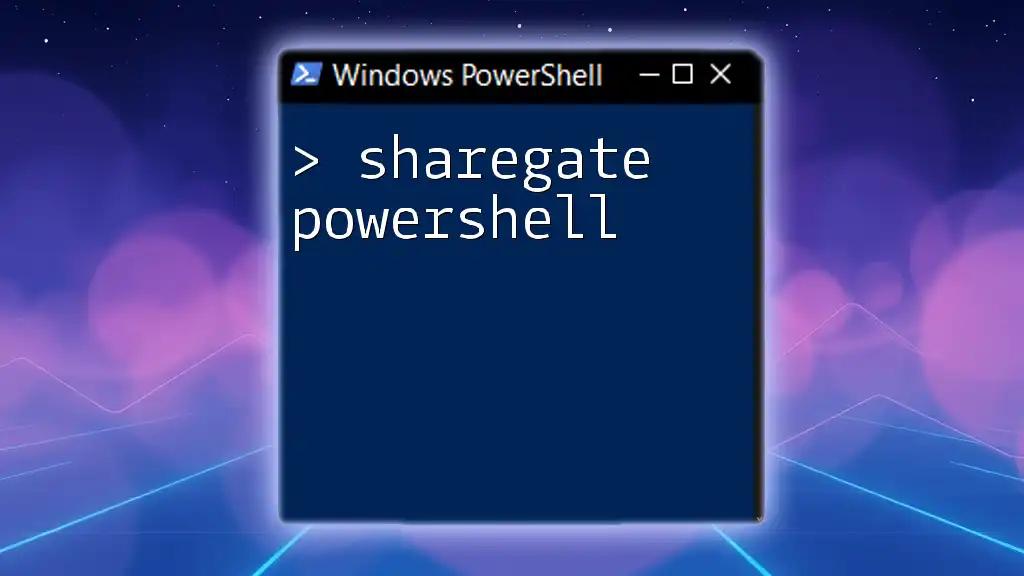
Advanced Techniques
Conditional Messaging
How to Display Messages Based on Conditions
You can enhance user interaction by utilizing conditional statements in your scripts. For example, you might want to provide feedback based on the success or failure of a task.
$status = "success"
if ($status -eq "success") {
[System.Windows.Forms.MessageBox]::Show("Operation completed successfully!")
} else {
[System.Windows.Forms.MessageBox]::Show("Operation failed.", "Error", [System.Windows.Forms.MessageBoxButtons]::OK, [System.Windows.Forms.MessageBoxIcon]::Error)
}
This code snippet demonstrates how to display a success or error message based on a variable's value. Such conditional messaging can guide users based on the situation.
Logging and Messaging
Importance of Logging Alongside Messaging
Tracking messages through logs is essential for auditing and troubleshooting. By logging messages while notifying users, you can create a robust feedback loop. Here’s an example of how to combine message logging with user notifications:
$logPath = "C:\logs\scriptLog.txt"
$message = "Task completed successfully."
Write-Output $message | Out-File -Append -FilePath $logPath
[System.Windows.Forms.MessageBox]::Show($message)
In this example, the script logs the message to a specified log file while also displaying it to the user, maintaining transparency and ease of tracking script progress.
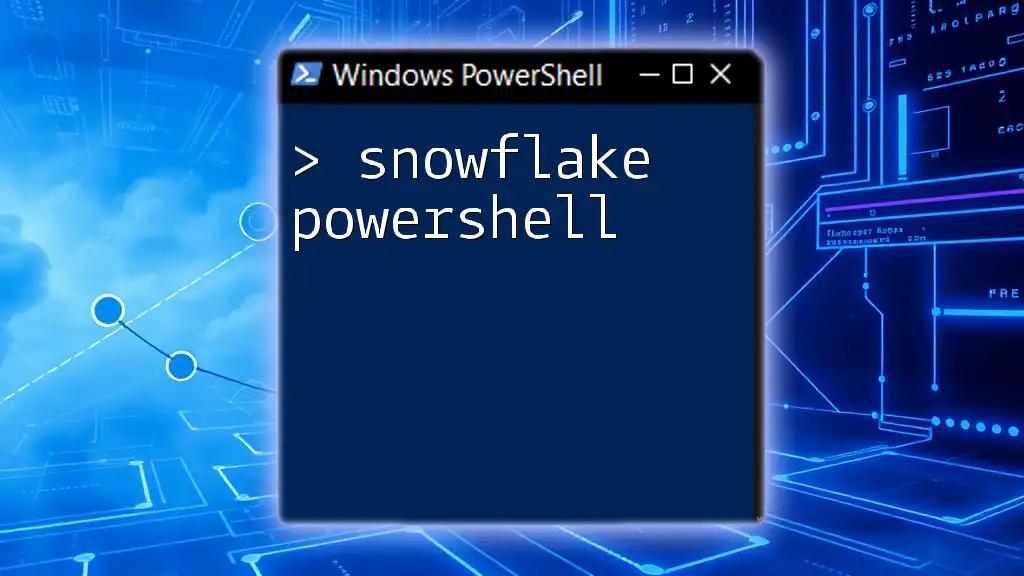
Best Practices for Messaging in PowerShell
Tips for Creating Effective Messages
- Clarity and Conciseness: Keep messages brief and to the point to ensure understanding.
- Appropriate Notification Types: Choose between message boxes, write-host, or toast notifications based on context and user needs.
- Avoid Overloading Users: Limit the frequency of messages to prevent overwhelming the user.
Common Pitfalls to Avoid
- Misusing Write-Host: Avoid using `Write-Host` where output can be captured for processing, as this limits your script's capabilities.
- Neglecting User Experience: Ensure that notifications are not disruptive to user workflows and are relevant to the context.
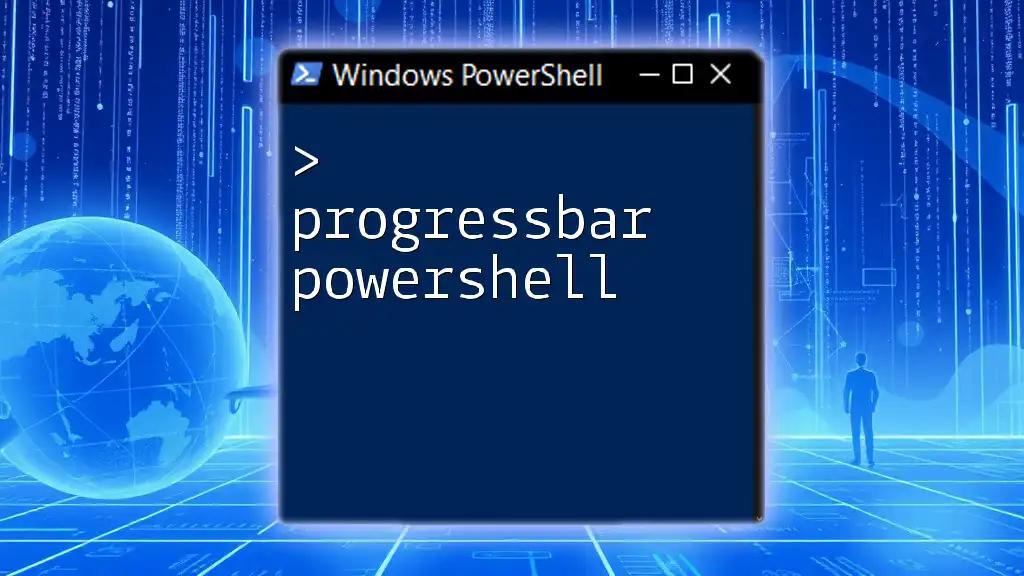
Conclusion
Show Message PowerShell techniques significantly enhance user interaction and feedback throughout scripting processes. These methods ensure users stay informed, whether through console outputs or more interactive message boxes and notifications. Test different techniques and adapt them to your scripts to improve the overall experience. By practicing and exploring these messaging options, you will not only streamline your scripts but also elevate your scripting projects to a more professional level.
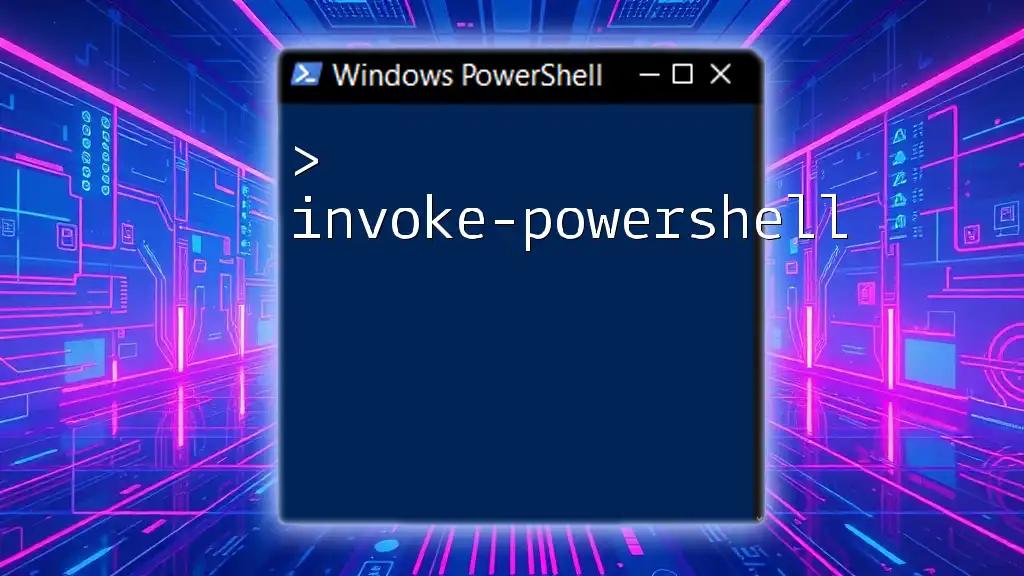
Additional Resources
Refer to the official PowerShell documentation and community tutorials to expand your knowledge and skills associated with messaging in PowerShell.