To add a user to a security group in PowerShell, you can use the `Add-ADGroupMember` cmdlet to efficiently include a specified user in the desired security group.
Add-ADGroupMember -Identity "YourSecurityGroupName" -Members "Username"
Understanding Security Groups in PowerShell
Definition of Security Groups
Security groups are collections of user accounts that enable you to manage user permissions efficiently in Windows environments. Unlike distribution groups, which are primarily used for email distribution lists and do not assign security permissions, security groups can be used to grant access to resources for all members. This means you can easily manage access to files, folders, and applications by simply managing group membership.
Use Cases for Adding Users to Security Groups
Adding users to security groups can streamline permission management. For example:
- Project Teams: For managing access to shared project files.
- Departmental Access: Granting an entire department access to a centralized network folder.
- Resource Management: Quickly implementing security policies as requirements change.
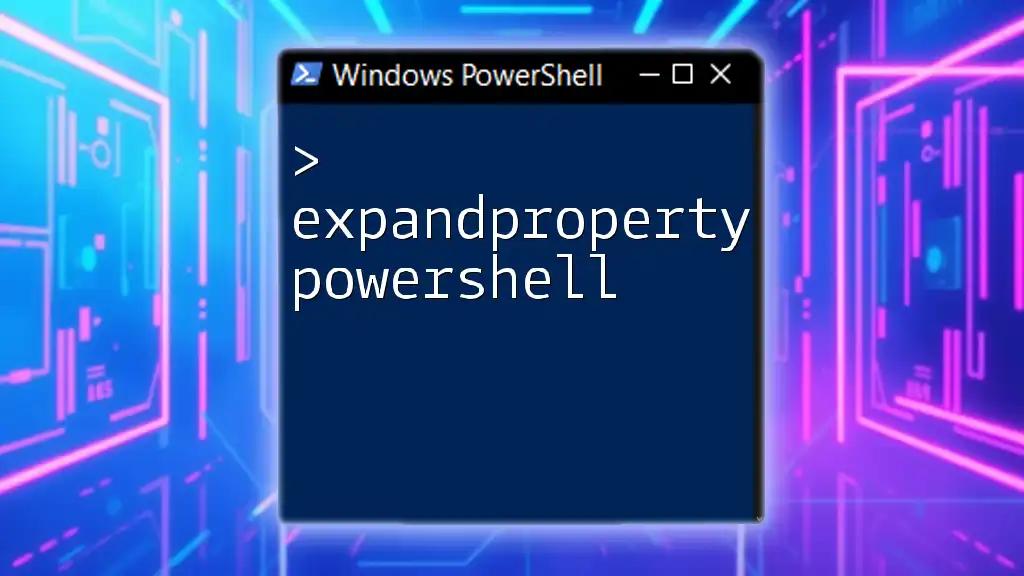
Prerequisites
Required Permissions
To add a user to a security group using PowerShell, you must have administrative permissions in Active Directory (AD). This usually means being part of the Domain Admins or Account Operators groups.
PowerShell Environment Setup
Ensure you have PowerShell installed on your machine. PowerShell typically comes pre-installed on Windows operating systems. To work with security groups, you will need the Active Directory module.
To verify that the Active Directory module is available, run the following command:
Get-Module -ListAvailable
If you don’t see the Active Directory module, you may need to install it via the Remote Server Administration Tools (RSAT).
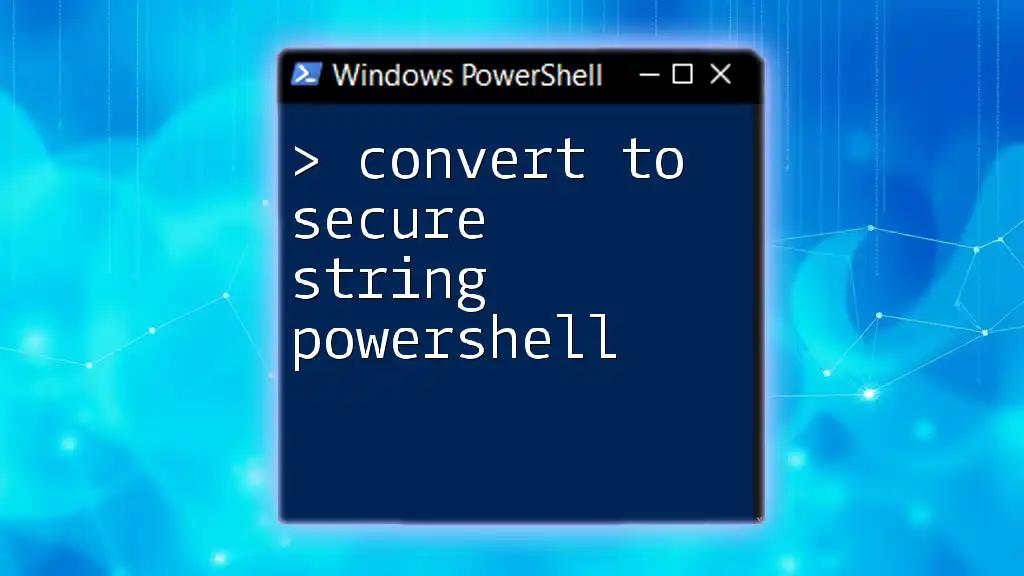
Basic Commands to Add Users to Security Groups
Using `Add-ADGroupMember`
Syntax Explanation
The `Add-ADGroupMember` cmdlet is used to add one or more members to a security group within Active Directory. Here’s the basic syntax:
Add-ADGroupMember -Identity "<GroupName>" -Members "<UserName>"
- `-Identity`: Specifies the security group you're targeting.
- `-Members`: Specifies the user or users you want to add to that group.
Basic Example
Here’s a simple command to add a single user to a security group:
Add-ADGroupMember -Identity "HRGroup" -Members "jdoe"
In this example, we add the user “jdoe” to the "HRGroup."
Adding Multiple Users
Using a Comma-Separated List
To add multiple users at once, simply separate their usernames with commas:
Add-ADGroupMember -Identity "HRGroup" -Members "jdoe", "asmith", "bjones"
In this command, we are adding three users to the "HRGroup."
Using a User List from a CSV File
If you have a large number of users to add, consider using a CSV file. First, your CSV should look something like this:
Username
jdoe
asmith
bjones
Then, you can add these users to a group with the following command:
Import-Csv "C:\path\to\userlist.csv" | ForEach-Object { Add-ADGroupMember -Identity "HRGroup" -Members $_.Username }
This script imports usernames from the CSV file and iteratively adds each user to the specified group.
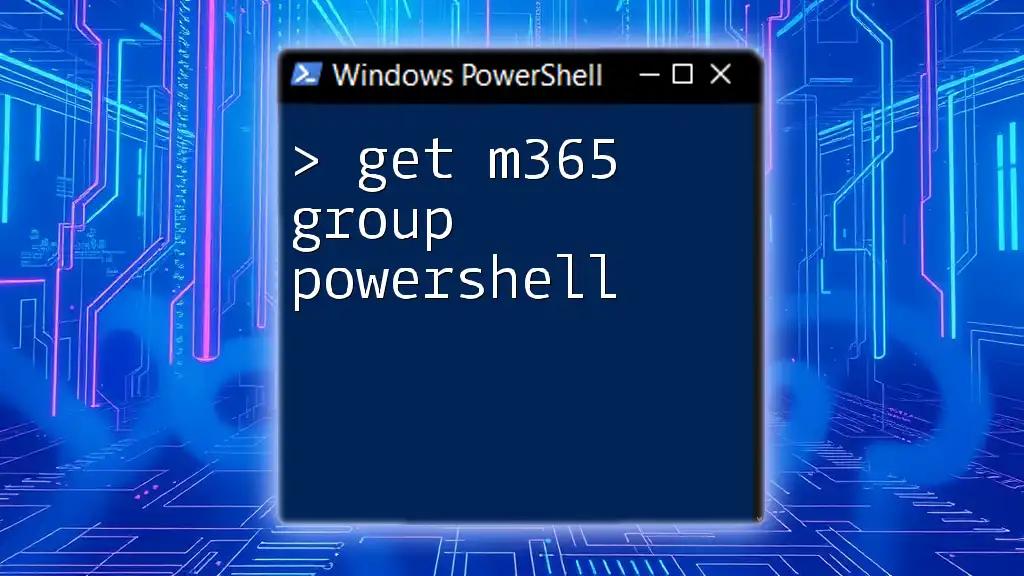
Advanced Techniques
Error Handling
Common Errors When Adding Users
You might encounter errors when adding users to groups, such as the "user not found" or "group not found" error. Diagnosing these errors can be crucial to resolving issues quickly.
Implementing Try-Catch Blocks
To enhance your script's robustness, consider implementing try-catch blocks. Here’s an example:
try {
Add-ADGroupMember -Identity "HRGroup" -Members "jdoe"
} catch {
Write-Host "Error adding user: $_"
}
This command will attempt to add the user to the group and, if it fails, it will display an error message without stopping the entire script.
Verifying User Addition
Checking Group Membership
To confirm that the user has been added to the security group, you can use:
Get-ADGroupMember -Identity "HRGroup"
This command will list all members of the specified group, allowing you to validate the addition.
Generating Reports
If you need a report of the users in a security group, you can export that list to a CSV file with the following command:
Get-ADGroupMember -Identity "HRGroup" | Select-Object Name, SamAccountName | Export-Csv "C:\path\to\groupReport.csv" -NoTypeInformation
The exported CSV will contain the names and SAM account names of the users, making it easy for documentation and audits.

Best Practices
Managing User Permissions
When adding users to security groups, adhere to the principle of least privilege. Only grant users access to the resources they need for their job roles. This practice minimizes security risks and keeps your environment safe.
Documenting Changes
It's important to keep a log of changes made to security group memberships, detailing who was added or removed and when. Maintain fields like:
- Username
- Group Name
- Date of Change
- Administrator Name
Leveraging PowerShell Scripts
For repetitive tasks, leveraging PowerShell scripts can save significant time and effort. Consider creating a simple script that defines the security group and user list, and then executes the addition automatically.
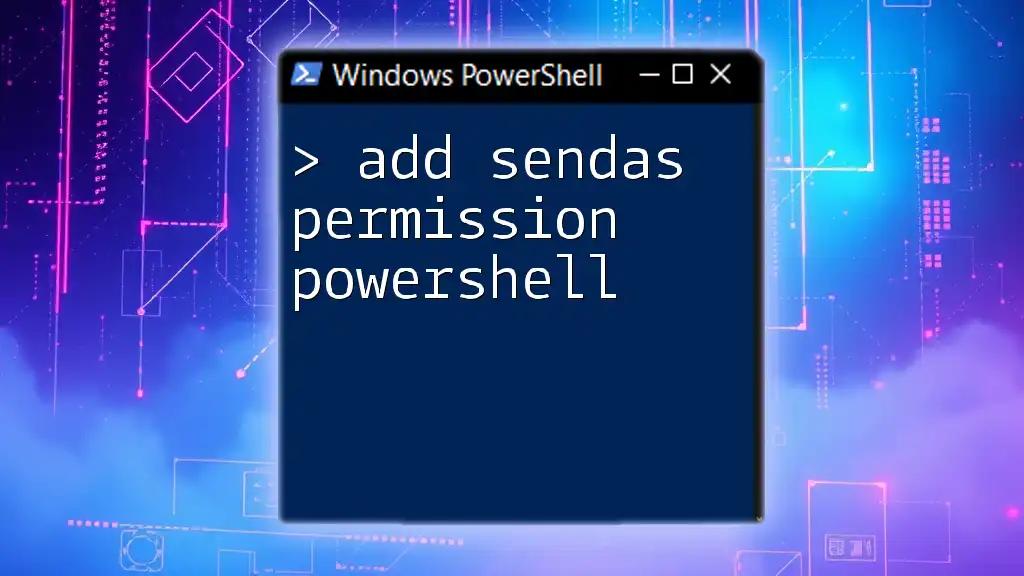
Conclusion
In this guide, we covered how to efficiently add users to security groups using PowerShell. Proper management of user permissions in a Windows environment is vital for maintaining security. Practicing these commands and exploring additional PowerShell techniques will enhance your ability to manage Active Directory effectively.
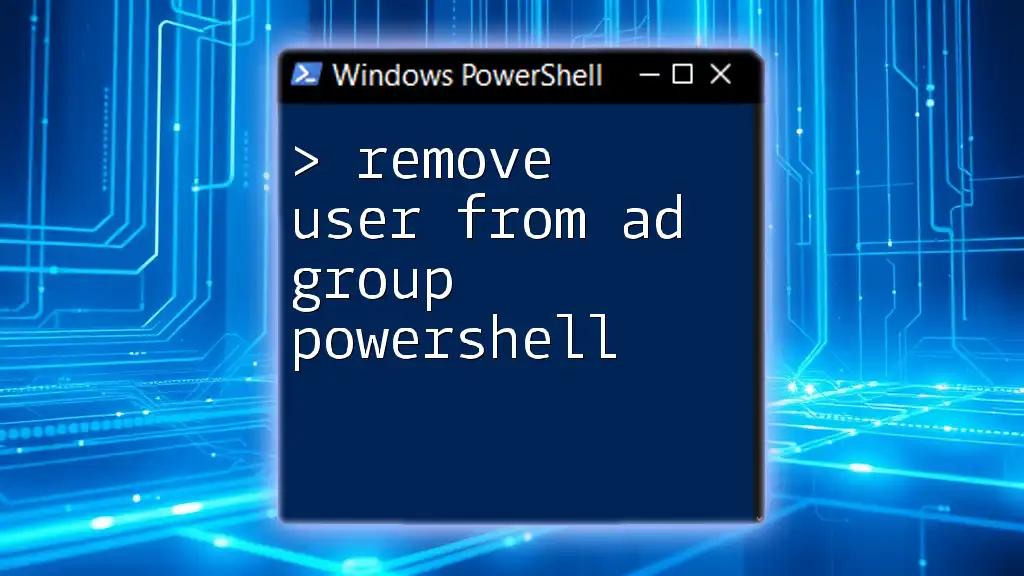
Additional Resources
Recommended Reading
For those looking to broaden their understanding of PowerShell and Active Directory, consider reviewing the official Microsoft documentation and investing in books or online courses that specialize in PowerShell management.
Community Support
Engaging with online forums and communities can provide invaluable support as you delve deeper into PowerShell. Platforms like Stack Overflow and the Microsoft Tech Community are excellent for finding advice, sharing experiences, and expanding your networking opportunities.