You can send an email from PowerShell using the `Send-MailMessage` cmdlet, allowing you to quickly send messages directly from your script.
Here’s a simple code snippet demonstrating how to do this:
Send-MailMessage -From "yourEmail@example.com" -To "recipient@example.com" -Subject "Test Email" -Body "This is a test email sent from PowerShell." -SmtpServer "smtp.example.com"
Understanding PowerShell Email Sending Capabilities
What is Send-MailMessage?
The `Send-MailMessage` cmdlet is one of the most valuable built-in tools in PowerShell for sending emails. It simplifies the process, allowing users to send emails directly from their scripts or command line. This cmdlet is particularly useful for automating notifications, alerts, and reports on systems where manual email composition would be inefficient.
Prerequisites
Before diving into sending emails, it's essential to ensure your PowerShell environment is ready. Here are the basic prerequisites:
- PowerShell Version: You’ll need PowerShell version 3.0 or later to utilize the `Send-MailMessage` cmdlet effectively.
- SMTP Server Access: You must have access to an SMTP (Simple Mail Transfer Protocol) server. This can be a corporate email server, or you can use a free service like Gmail or Outlook, provided that you configure them for external access correctly.
- Permissions: Ensure that your user account has permission to send emails through the chosen SMTP server.
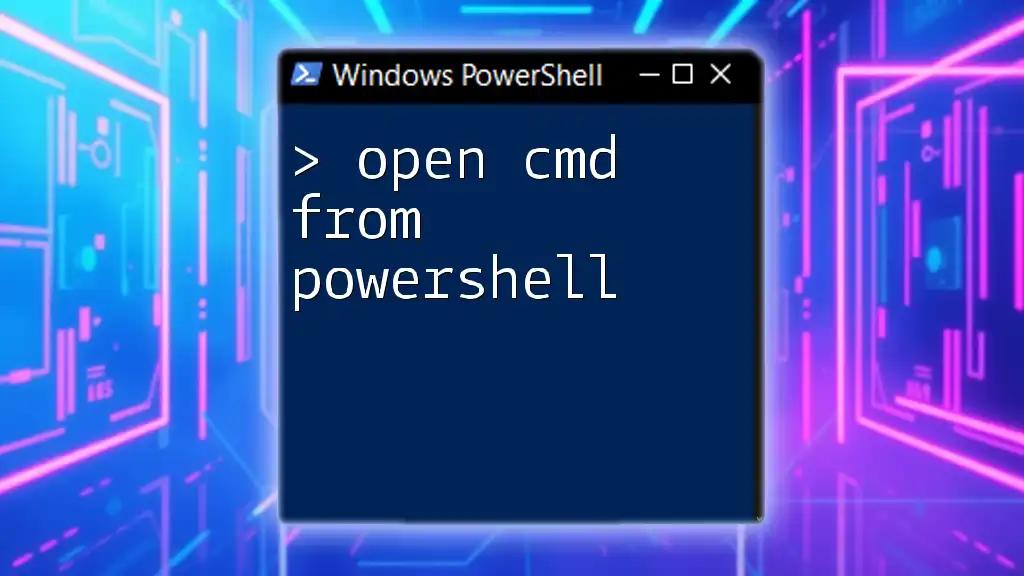
How to Send Email via PowerShell
Basic Syntax of Send-MailMessage
The syntax for the `Send-MailMessage` cmdlet is straightforward but requires a few mandatory parameters to function correctly. The basic format looks like this:
Send-MailMessage -To "recipient@example.com" -From "sender@example.com" -Subject "Email Subject" -Body "Email Body" -SmtpServer "smtp.example.com"
Example: Sending a Simple Email
To illustrate sending an email, let’s consider this simple example. The following command sends a straightforward email:
Send-MailMessage -To "recipient@example.com" -From "sender@example.com" -Subject "Test Email" -Body "This is a test email." -SmtpServer "smtp.example.com"
In this command:
- `-To`: Specifies the recipient's email address.
- `-From`: Indicates the sender's email address.
- `-Subject`: Sets the subject line for the email.
- `-Body`: Contains the main content of the email.
- `-SmtpServer`: Defines the SMTP server used to send the email.
Exploring Additional Parameters
Adding Attachments
One of the powerful features of `Send-MailMessage` is the ability to attach files to your email. This is useful for sending reports, logs, or any necessary documents. Here’s how to include an attachment:
Send-MailMessage -To "recipient@example.com" -From "sender@example.com" -Subject "Email with Attachment" -Body "Please find the attachment." -SmtpServer "smtp.example.com" -Attachments "C:\path\to\file.txt"
In this example, the `-Attachments` parameter is used to specify the path to the file you want to include in the email.
HTML Bodies
For a more engaging email format, you can send HTML content by using the `-BodyAsHtml` switch. This allows you to create visually appealing emails:
Send-MailMessage -To "recipient@example.com" -From "sender@example.com" -Subject "HTML Email" -Body "<h1>Hello</h1><p>This is an HTML email.</p>" -BodyAsHtml -SmtpServer "smtp.example.com"
In this case, the HTML content creates a header and paragraph in the body of the email, making it look more professional.
CC and BCC Options
You can also send copies of the email to other recipients using the `-Cc` (Carbon Copy) and `-Bcc` (Blind Carbon Copy) parameters:
Send-MailMessage -To "recipient@example.com" -Cc "cc@example.com" -Bcc "bcc@example.com" -From "sender@example.com" -Subject "CC and BCC Example" -Body "This email is sent with CC and BCC." -SmtpServer "smtp.example.com"
- `-Cc` allows recipients to see who else received the email.
- `-Bcc` prevents recipients from seeing who else has been included in the email list.
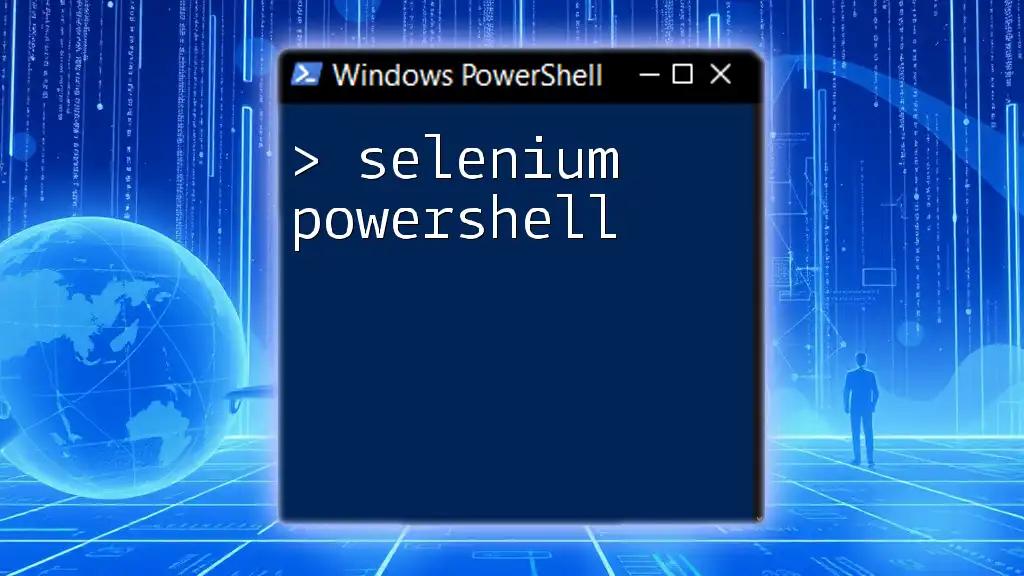
Advanced Sending Methods
Sending Emails with PowerShell Scripts
To take advantage of the `Send-MailMessage` cmdlet in a more automated fashion, you can create PowerShell scripts. This is especially beneficial for sending routine emails, such as daily reports or notifications.
Here’s an example of a simple script that sends an email:
$EmailFrom = "sender@example.com"
$EmailTo = "recipient@example.com"
$EmailSubject = "Automated Email"
$EmailBody = "This is an email sent from a PowerShell script."
$SmtpServer = "smtp.example.com"
Send-MailMessage -From $EmailFrom -To $EmailTo -Subject $EmailSubject -Body $EmailBody -SmtpServer $SmtpServer
This script defines variables for critical components like sender and recipient details, subject, and body content. It allows for easier customization and repeated use.
Using External Libraries (Optional)
If you need more advanced emailing capabilities, consider using .NET libraries, such as `System.Net.Mail`. This gives you more control over the email sending process, including MIME types, encoding, and more.
Add-Type -AssemblyName System.Net.Mail
$mailMessage = New-Object System.Net.Mail.MailMessage
$mailMessage.From = "sender@example.com"
$mailMessage.To.Add("recipient@example.com")
$mailMessage.Subject = "Advanced Email"
$mailMessage.Body = "This email uses System.Net.Mail"
$smtpClient = New-Object System.Net.Mail.SmtpClient("smtp.example.com")
$smtpClient.Send($mailMessage)
This method allows you to manage complex email scenarios and is great for developers who prefer more advanced configurations.
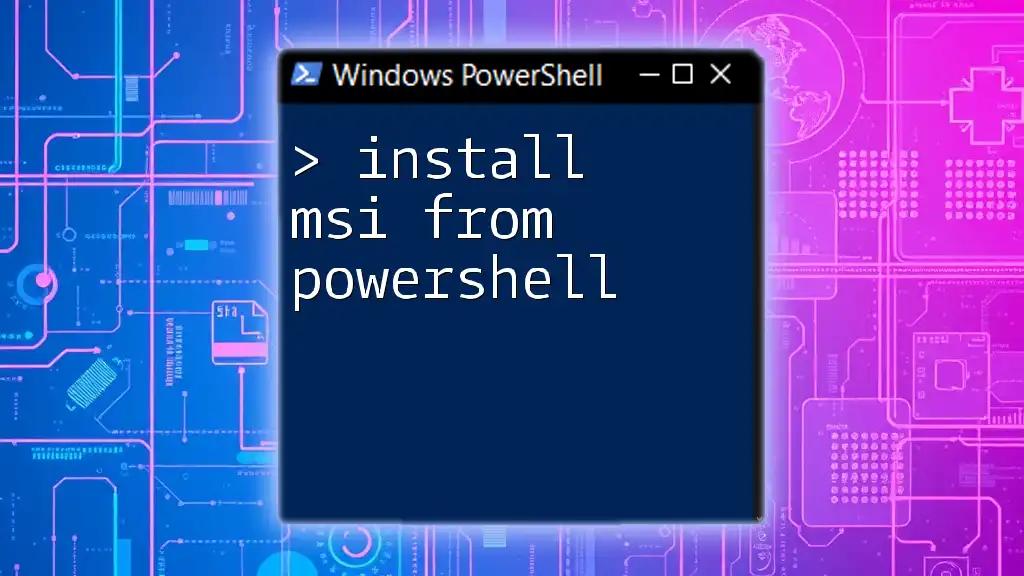
Testing Your Email Sending Capability
Troubleshooting Common Issues
While using `Send-MailMessage`, you might encounter several common problems:
- SMTP Authentication Errors: Ensure that you have provided proper authentication, especially when using third-party email services.
- Firewall and Security Implications: Be aware that some environments may restrict outgoing SMTP traffic, necessitating firewall configurations.
Logging and Error Handling in PowerShell
To effectively manage and diagnose issues, it’s beneficial to implement error handling in your PowerShell scripts. Here's how to log errors when sending emails:
try {
Send-MailMessage -To "recipient@example.com" -From "sender@example.com" -Subject "Test Email" -Body "This is a test email." -SmtpServer "smtp.example.com"
} catch {
Write-Error "Email sending failed: $_"
}
Using a `try-catch` block, you can capture errors and log them for further investigation.
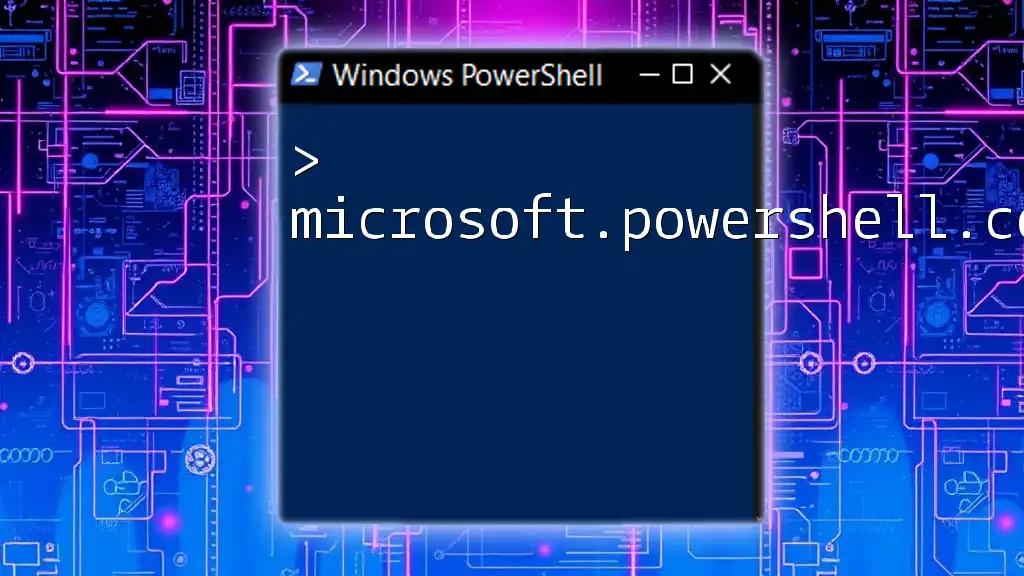
Conclusion
In summary, learning how to send email from PowerShell opens up a myriad of possibilities for automation and communication. By utilizing the `Send-MailMessage` cmdlet, you can create scripts that significantly reduce the time spent on repetitive emailing tasks. As you practice with the examples provided, you’ll gain confidence in using PowerShell for this purpose, making it an invaluable tool in your automation arsenal.
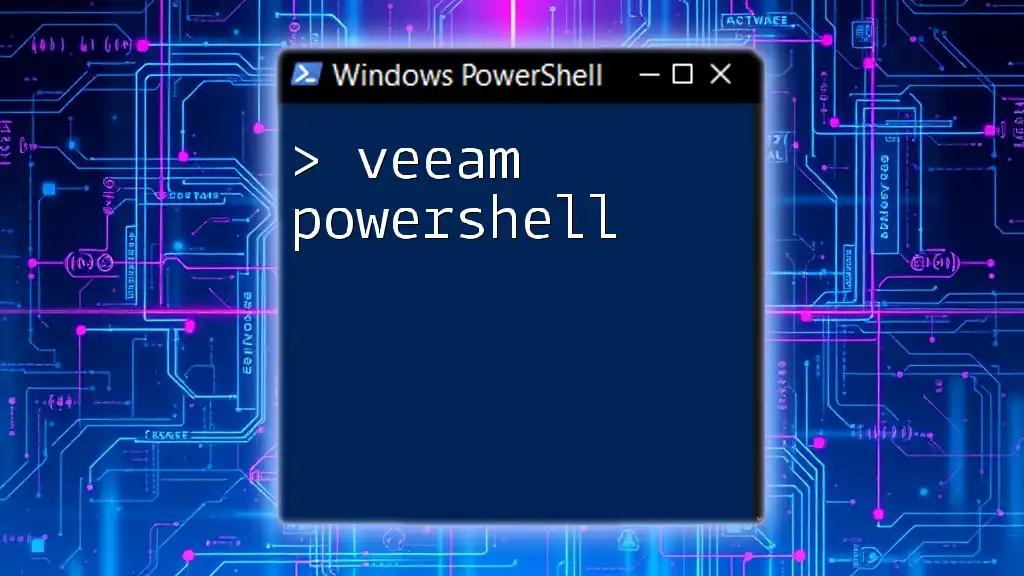
Frequently Asked Questions
Can I send emails from PowerShell without a working SMTP server?
Unfortunately, you cannot send emails from PowerShell without access to a functioning SMTP server. It is a crucial component of the email delivery process.
Is it secure to send emails from PowerShell?
When using PowerShell to send emails, always consider security implications. Ensure you're using secure and trusted SMTP servers, and implement authentication when possible to safeguard sensitive data.

Call to Action
Now that you’re equipped with the knowledge to send emails from PowerShell, consider signing up for our comprehensive courses that cover PowerShell scripting in detail. Practice these commands and share your progress—let’s open new doors in your scripting journey!